21
NovAngular Dependency Injection | Types of dependency injection in Angular
Angular Dependency Injection: An Overview
Angular, a popular open-source web application framework developed by Google, incorporates a powerful and flexible concept known as Dependency Injection (DI). It is employed to create the fundamental ideas of Angular. In this Angular tutorial, we'll explore the fundamentals of Angular Dependency Injection, its benefits, and how to leverage it to enhance the structure of your Angular applications. For more in-depth knowledge, consider our Angular Certification Online Training.
Read More: Top 50 Angular Interview Questions & Answers
What Is Dependency Injection?
Dependency Injection is a design pattern that promotes the separation of concerns by decoupling components and their dependencies. In Angular, dependencies are typically services, but they also can be values, such as strings or functions. DI is used to inject instances of services, components, and other objects into classes that depend on them, promoting modularity, reusability, and testability within the application.
There are three types of classes in Dependency Injection:
- Client Class - This is the dependent class, which depends on the service class.
- Service Class - Class that provides the service to the client class.
- Injector Class - Injects the service class object into the client class.
Key Concepts of Angular Dependency Injection
1.) Injector
The Angular Injector creates and manages instances of components, directives, services, and other Angular constructs. It maintains a hierarchy of injectors, with the root injector at the top. When a component requests a dependency, the injector looks for the required service in its hierarchy and provides it.
2.) Providers
Providers tell the injector how to create and configure instances of a particular dependency. They are defined in the metadata of Angular components using the provider's property. Providers can be specified at various levels, such as the component, module, or even the application's root module.
3.) Injectable Decorator
The @Injectable() decorator is used to make a class injectable. This decorator informs Angular that the class may have dependencies that need to be injected. It is typically applied to services, allowing Angular to recognize and manage their dependencies.
Types of Dependency Injection in Angular
In Angular, there are three types of Dependency Injection (DI):
- Constructor injection
- Setter injection
- Interface injection
Let's explore each type in detail:
1. Constructor injection
Constructor injection is the most common type of DI in Angular. Dependencies are injected through the constructor of a class, typically a component or a service. The Angular framework automatically resolves and provides the required dependencies when creating an instance of the class.
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root',
})
export class MyService {
constructor(private dependencyService: DependencyService) {
// ...
}
}
In the example above, DependencyService is injected into the MyService class through its constructor.
2. Setter injection
Setter injection involves injecting dependencies through setter methods. Instead of receiving dependencies through the constructor, the class provides setter methods that can be called to set the dependencies after the instance is created.
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root',
})
export class MyService {
private dependencyService: DependencyService;
setDependencyService(dependencyService: DependencyService): void {
this.dependencyService = dependencyService;
}
}
Setter injection can be useful in scenarios where injecting dependencies through the constructor is not feasible or desirable.
3. Interface injection
Interface-based injection involves creating an interface for a service and injecting the interface rather than the concrete implementation. This allows for greater flexibility and easier substitution of implementations, especially during testing.
import { Injectable } from '@angular/core';
interface DependencyInterface {
// Define methods and properties
}
@Injectable({
providedIn: 'root',
})
export class DependencyService implements DependencyInterface {
// Implementation of the interface
}
@Injectable({
providedIn: 'root',
})
export class MyService {
constructor(private dependency: DependencyInterface) {
// ...
}
}
In this example, MyService depends on the DependencyInterface, which the DependencyService implements. During testing, a mock implementation of the interface can be provided to isolate the unit being tested.
How To Implement Angular Dependency Injection?
1. Create a Service
ng generate service my-service
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root', // Provides the service at the root level
})
export class MyService {
// Implement your service logic here
}
2. Inject the Service into a Component
import { Component } from '@angular/core';
import { MyService } from './my-service.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
constructor(private myService: MyService) {
// Use myService in the component
}
}
3. Register the Service in a Module
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { MyService } from './my-service.service';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
providers: [MyService], // Register the service here
bootstrap: [AppComponent],
})
export class AppModule {}
4. Use the Service in the Component
import { Component } from '@angular/core';
import { MyService } from './my-service.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
constructor(private myService: MyService) {
// Use myService in the component
const data = this.myService.getData();
console.log(data);
}
}
5. Optional: Use Dependency Injection in Templates
<div *ngIf="myService.someCondition()">Content to show based on service condition</div>
Benefits of Angular Dependency Injection
- Modularity: DI promotes modularity by encapsulating the functionality of each component and service. This makes it easier to develop, test, and maintain individual parts of the application independently.
- Testability: With DI, it becomes straightforward to replace real services with mock services during testing. This facilitates unit testing, ensuring that each component can be tested in isolation.
- Maintainability: Dependency injection helps create loosely coupled components, making it easier to modify and extend the application without affecting other parts. This enhances the overall maintainability of the codebase.
- Reusability: Thanks to the decoupling facilitated by dependency injection, components and services can be reused across different parts of the application or even in multiple projects.
Read More:
- What's new in Angular 16: A Developer Guide to Angular 16 New Features
- What's new in Angular 17: A Developer Guide to Angular 17 New Features
- Angular vs. React vs. Blazor: Which Framework is Best?
- Angular Developer Skills
- 10 Angular Dev tools! You Must Know
- React vs. Angular: Which Career Option is More Promising in 2024?
Summary
Angular Dependency Injection is a powerful mechanism that contributes significantly to the framework's architecture. By promoting modularity, testability, maintainability, and reusability, Dependency Injection plays a vital role in building scalable and robust Angular applications. Implementing this design pattern into your development behaviors and understanding its fundamental ideas will surely result in simpler and easily maintained code.
FAQs
Take our Angular skill challenge to evaluate yourself!
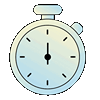
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.