24
JanImplementing SignalR in ASP.NET Core
SignalR in ASP.NET Core
Are you ready to go into the world of real-time web applications? Imagine your web app sending fast notifications without users needing to refresh their pages. This is exactly what SignalR does. It is a library used for adding real-time web features to a web application.
In this ASP.NET Tutorial, we will learn more about SignalR .NET Core, covering everything from setup to implementation and even some advanced features. Alternatively, you can enroll in an ASP.NET Certification Training to explore more about various features and concepts related to ASP.NET.
What is SignalR?
SignalR is a library for ASP.NET developers that makes it easy to add real-time web features to apps without a troublesome process. This makes it possible for the server code to send live updates to the clients immediately after they become accessible. SignalR, for instance, lets you create such things as chat apps, interactive dashboards, as well as notifications among others.
Steps to Implement SignalR in .NET Core
Setting up SignalR in a .NET Core application involves a few key steps. Let's understand them with a SignalR in .NET Core Example:
1. Configuring SignalR on the Server
Before anything else, you must establish your server to support SignalR. This implies setting up your ASP.NET Core application so that it includes the services and middleware it requires.
2. Creating Your ASP.NET Core Application
We will begin by creating a new ASP.NET Core application. Please use your terminal or command prompt for the following instructions:
dotnet new webapp -o SignalRChatApp
cd SignalRChatApp
dotnet add package Microsoft.AspNetCore.SignalR
dotnet add package Microsoft.AspNetCore.SignalR.Client
This sets up a new web application and installs the SignalR packages.
3. Configuring SignalR Services
public void ConfigureServices(IServiceCollection services)
{
services.AddRazorPages();
services.AddSignalR();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapRazorPages();
endpoints.MapHub<ChatHub>("/chatHub");
});
}
4. Setting Up Your SignalR Hub
The hub is the centerpiece of SignalR, defining methods that clients can call and enabling communication between the server and clients. Create a ChatHub class to handle these interactions.
using Microsoft.AspNetCore.SignalR;
using System.Threading.Tasks;
public class ChatHub : Hub
{
public async Task SendMessage(string user, string message)
{
await Clients.All.SendAsync("ReceiveMessage", user, message);
}
}
5. Create SignalR Client Library
<script src="https://cdnjs.cloudflare.com/ajax/libs/microsoft-signalr/5.0.11/signalr.min.js"></script>
<script>
const connection = new signalR.HubConnectionBuilder()
.withUrl("/chatHub")
.build();
connection.on("ReceiveMessage", (user, message) => {
const msg = `${user}: ${message}`;
const li = document.createElement("li");
li.textContent = msg;
document.getElementById("messagesList").appendChild(li);
});
connection.start().catch(err => console.error(err.toString()));
document.getElementById("sendButton").addEventListener("click", event => {
const user = document.getElementById("userInput").value;
const message = document.getElementById("messageInput").value;
connection.invoke("SendMessage", user, message).catch(err => console.error(err.toString()));
event.preventDefault();
});
Read More: Salary Offered to ASP.NET Developers |
How SignalR Works
1. Broadcasting Messages to Clients
2. Managing Groups of Connected Clients
public async Task AddToGroup(string groupName)
{
await Groups.AddToGroupAsync(Context.ConnectionId, groupName);
}
public async Task SendMessageToGroup(string groupName, string user, string message)
{
await Clients.Group(groupName).SendAsync("ReceiveMessage", user, message);
}
Handling Errors in SignalR Applications
public async Task SendMessage(string user, string message)
{
try
{
await Clients.All.SendAsync("ReceiveMessage", user, message);
}
catch (Exception ex)
{
// Handle the exception
}
}
On the client side, ensure you handle connection failures gracefully:
connection.start().catch(err => console.error(err.toString()));
Unit Testing SignalR Hubs and Clients
public class ChatHubTests
{
private readonly Mock<IHubCallerClients> _mockClients;
private readonly Mock<IClientProxy> _mockClientProxy;
private readonly ChatHub _chatHub;
public ChatHubTests()
{
_mockClients = new Mock<IHubCallerClients>();
_mockClientProxy = new Mock<IClientProxy>();
_chatHub = new ChatHub
{
Clients = _mockClients.Object
};
}
[Fact]
public async Task SendMessage_ShouldInvokeReceiveMessage()
{
_mockClients.Setup(clients => clients.All).Returns(_mockClientProxy.Object);
await _chatHub.SendMessage("user", "message");
_mockClientProxy.Verify(client => client.SendCoreAsync("ReceiveMessage", It.Is
Conclusion
Read More: Top 50 ASP.NET Core Interview Questions and Answers for 2024 |
FAQs
dotnet add package Microsoft.AspNetCore.SignalR dotnet add package Microsoft.AspNetCore.SignalR.Client
Take our Aspnet skill challenge to evaluate yourself!
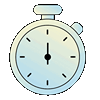
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.