21
FebData Binding in Asp.Net
Data Binding in ASP.Net can be categorized into two: simple data binding and declarative data binding. Some controls support at least simple databinding while others support both. Simple databinding basically involves attaching any collection (item collection) that implements the IEnumerable interface, or the DataSet and DataTable classes to the DataSource property of the control.
In this Asp.Net Tutorial, we will explore data binding in asp.net which will include what is data binding in asp. net, and types of data binding in asp.net.
What is data binding in ASP.NET?
Data binding is the process that establishes a connection between the app UI and the data it displays.
Types of data binding in asp.net.
- Simple data binding
- Declarative data binding
1.Simple Data Binding
We will discuss simple data binding with the help of a simple application. In this application, we have created a booklist class. This class has two string attributes a title (bookname) and author (authorname). On the page, we have used four data-bound controls: a ListBox, a DropDownList, a RadioButtonList, and a CheckButtonList. With each such control, there will be a label to show the selected value from the list. The application is self-explanatory and you can download it.
The class booklist looks like this:
Example:
public class booklist
{
protected String bookname;
protected String authorname;
public booklist(String bname, String aname)
{
this.bookname = bname;
this.authorname = aname;
}
public String Book
{
get
{
return this.bookname;
}
set{
this.bookname = value;
}
}
public String Author
{
get
{
return this.authorname;
}
set
{
this.authorname = value;
}
}
}
The code behind the page:
protected void Page_Load(object sender, EventArgs e)
{
IList bklist = createbooklist();
if (!this.IsPostBack)
{
this.ListBox1.DataSource = bklist;
this.ListBox1.DataTextField = "Book";
this.ListBox1.DataValueField = "Author";
this.DropDownList1.DataSource = bklist;
this.DropDownList1.DataTextField = "Book";
this.DropDownList1.DataValueField = "Author";
this.RadioButtonList1.DataSource = bklist;
this.RadioButtonList1.DataTextField = "Book";
this.RadioButtonList1.DataValueField = "Author";
this.CheckBoxList1.DataSource = bklist;
this.CheckBoxList1.DataTextField = "Book";
this.CheckBoxList1.DataValueField = "Author";
this.DataBind();
}
}
protected IList createbooklist()
{
ArrayList allbooks = new ArrayList();
booklist bl;
bl = new booklist("UNIX CONCEPTS", "SUMITABHA DAS");
allbooks.Add(bl);
bl = new booklist("PROGRAMMING IN C", "RICHI KERNIGHAN");
allbooks.Add(bl);
bl = new booklist("DATA STRUCTURE", "TANENBAUM");
allbooks.Add(bl);
bl = new booklist("NETWORKING CONCEPTS", "FOROUZAN");
allbooks.Add(bl);
bl = new booklist("PROGRAMMING IN C++", "B. STROUSTROUP");
allbooks.Add(bl);
bl = new booklist("ADVANCED JAVA", "SUMITABHA DAS");
allbooks.Add(bl);
return allbooks;
}
protected void ListBox1_SelectedIndexChanged(object sender, EventArgs e)
{
this.lbllistbox.Text = this.ListBox1.SelectedValue;
}
protected void DropDownList1_SelectedIndexChanged(object sender, EventArgs e)
{
this.lbldrpdown.Text = this.DropDownList1.SelectedValue;
}
protected void RadioButtonList1_SelectedIndexChanged(object sender, EventArgs e)
{
this.lblrdlist.Text = this.RadioButtonList1.SelectedValue;
}
protected void CheckBoxList1_SelectedIndexChanged(object sender, EventArgs e)
{
this.lblchklist.Text = this.CheckBoxList1.SelectedValue;
}
Notice the use of the Generic IList class template. An ArrayList class is used to pass the values. This kind of coding is alright when data is not strongly typed. The rendered page looks like
2. Declarative Data Binding
Connecting with the database:
- Odbc Data Provider
- OleDb Data Provider
- OracleClient Data Provider
- SqlClient Data Provider
- SQL Server CE Data Provider
Example:
Class myDBappl
{
static void Main(string[] args)
{
DbProviderFactory providerfactory = DbProviderFactories.GetFactory(“System.Data.SqlClient”);
DbConnection conn = providerfactory.CreateConnection();
using(conn)
{ ConfigurationSettings s = ConfigurationSettings.ConnectionStrings[“Booklist”];
conn.ConnectionString = s.ConnectionString;
conn.Open();
DbCommand cmd = conn.CreateCommand();
cmd.CommandText = “select * from books”;
DbDataReader reader = cmd.ExecuteReader();
//display the data .........
}
}
}
Working with the data
DataReader:
DbDataReader reader = cmd.ExecuteReader();
DataSet:
- Firstly, it helps to overcome concurrency in the database.
- If more than one user tries to write on the same data simultaneously it leads to a concurrency problem.
- But using a dataset each user gets a copy of the required portion of the database as queried by the user.
- And secondly, it increases the scalability of the application, because here the connectivity with the database will not hinder the application’s progress, as each user will be disconnected after retrieving the required data.
- DataSet class objects are built using a DataAdapter.
- A DataSet includes a DataTable array – one for each selection statement in the query.
- The DataSet contains a DataTable collection and creates a DataTable element for each select statement.
- The Tables collection could be accessed by either ordinal or String-type indices.
Example:
Public static void usedataset()
{
DataSet ds = new DataSet();
Try
{
SqlDataAdapter da = new SqlDataAdapter( “select * from customer; select from country”, “server=.; uid=sa; pwd=;database=CUSTOMERS”);
da.Fill(ds, “customer”);
}catch(SqlException e)
{ System.Console.WriteLine(e);
}
Foreach( DataTable t in ds.Tables)
{
Console.WriteLIne(“Table ” + t.TableName + “ is in the dataset” );
Console.WriteLIne(“row 0 , column 1: “ + t.Rows[0][1]);
Console.WriteLIne(“row 1 , column 1: “ + t.Rows[1][1]);
Console.WriteLIne(“row 2 , column 1: “ + t.Rows[2][1]);
}
ds.WriteXml(“(c:\\dataset.xml”);
ds.WriteXmlSchema(“(c:\\dataset.xsd”);
...
...
}
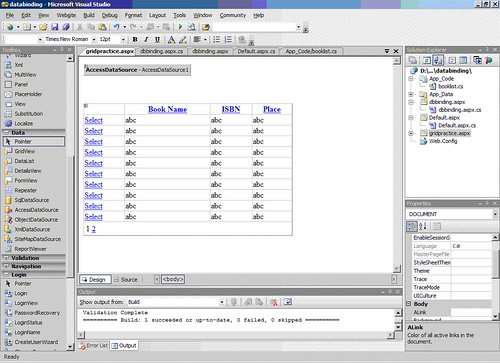
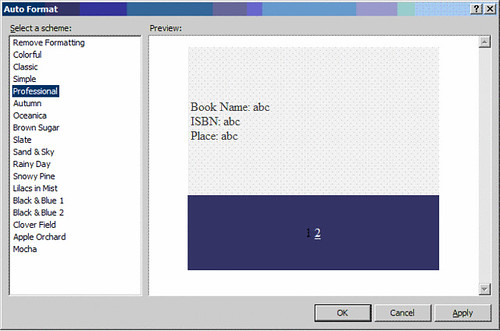
Conclusion:
Take our Aspnet skill challenge to evaluate yourself!
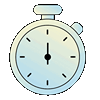
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.