Logging in .NET Core: A Comprehensive Guide
Logging in .NET Core
Logging in .NET Core is all about gathering and documenting important data on the operation of your application. This includes keeping track of events such as warnings, errors, and general data, all of which can be very beneficial for monitoring and debugging.
In this Asp.Net Core Tutorial, We will explore more about Logging in .Net Core which will include What is DBContext? Logging in .net core example, Fundamentals of Logging in .NET Core. Let's see it one by one.
What is DbContext?
- DbContext is the main class in the.NET Core domain that is in charge of communicating with the database via Entity Framework Core.
- You can query and store data using it as a bridge between your domain or entity classes and the database.
- It includes methods to control the lifespan of the entities and all the configurations required to connect to a database.
Different Types of Logs
Any application must include logging since it gives you insight into the program's behavior and helps with monitoring and debugging. An application may produce a variety of log types, including:
- Debug Logs: Detailed data that is usually only useful for problem diagnosis.
- Information Logs: Messages providing coarse-grained details about the application's progress.
- Warning Logs: Dangerous circumstances that could cause problems if left unchecked.
- Error logs: occurrences that might still permit the application to execute are recorded in the error logs.
- Critical Logs: Severe error incidents resulting in the program being terminated.
Introduction to .NET Core Logging Levels and Their Hierarchy
Logging levels in.NET Core specify the level of severity of the messages that are logged. The levels are as follows, in ascending order of severity:
- Trace: The most detailed data, usually utilized to identify issues.
- Debug: Data that can be used to troubleshoot software during development.
- Details: Broad operational occurrences that do not qualify as errors.
- Alert: Draw attention to an odd or surprising application flow event.
- Error: This shows where the current action is failing.
- Important: Explain a system crash or unrecoverable program.
Configuring Logging in ASP.NET Core
1. Setting Up Logging with the ILoggerFactory Interface
A factory interface called ILoggerFactory is used to generate ILogger objects. It is employed for managing and configuring logging providers.
public void Configure(IApplicationBuilder app, ILoggerFactory loggerFactory)
{
loggerFactory.AddConsole();
loggerFactory.AddDebug();
}
2. Registering Logging Providers (Console, File, Debug)
public void ConfigureServices(IServiceCollection services)
{
services.AddLogging(builder =>
{
builder.AddConsole();
builder.AddDebug();
builder.AddFile("Logs/myDemoapp-{Date}.txt"); // Requires additional NuGet package
});
}
3. Determining the Minimum Log Level for Every Provider
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"System": "Warning"
},
"Console": {
"LogLevel": {
"Default": "Debug"
}
}
}
}
Log Messages Writing
1. ILogger Interface: Writing Log Messages at Various Levels
public class HomeController : Controller
{
private readonly ILogger _logger;
public HomeController(ILogger logger)
{
_logger = logger;
}
public IActionResult Index()
{
_logger.LogInformation("This is information log.");
_logger.LogWarning("This is warning log.");
_logger.LogError("This is error log.");
return View();
}
}
2. Adding Contextual Data (such as User IDs and Timestamps) to Log Messages
public void LogWithContext(ILogger logger)
{
using (logger.BeginScope("User ID: {UserId}", 23456))
{
logger.LogInformation("User-specific information.");
}
}
3. Organizing Log Messages to Make Them Readable
_logger.LogInformation("Order {OrderId} created successfully at {Timestamp}.", orderId, DateTime.Now);
Consuming Logs
- It effectively involves setting up a system where logs are collected, aggregated, and analyzed.
- Tools such as Elasticsearch, Kibana, Application Insights, and Serilog are used to integrate for these purposes.
Selecting the Appropriate Log Level in Various Circumstances
- Use trace/debug only for debugging and development; do not use it in production.
- Information: This is used to record the application's overall flow.
- Use only in the event of unusual or unexpected occurrences that won't force the program to terminate.
- Error: This log is used to record errors that impede the continuation of the present task.
- Critical: For serious mistakes that result in the application crashing, use.
Example of Logging in .NET Core
Example
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureLogging(logging =>
{
logging.ClearProviders();
logging.AddConsole();
logging.AddDebug();
logging.AddFile("Logs/myapp-{Date}.txt");
logging.SetMinimumLevel(LogLevel.Information);
})
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup();
});
}
public class HomeController : Controller
{
private readonly ILogger _logger;
public HomeController(ILogger logger)
{
_logger = logger;
}
public IActionResult Index()
{
_logger.LogInformation("Accessed Index page at {Timestamp}.", DateTime.Now);
return View();
}
}
Conclusion
FAQs
Q1. What is logging in .NET Core?
Q2. What is logging in programming C#?
Q3. What is the default logging in .NET Core?
Take our Aspnet skill challenge to evaluate yourself!
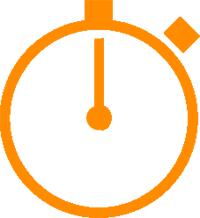
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.