22
DecUnderstanding Startup Class In ASP.NET Core
ASP.NET Core - Startup Class
The phrase ‘Startup class’ will come across regularly as we start working on our first web projects using ASP.NET Core. A Startup Class is the place at which you set up services and the app's request pipeline.
In this ASP.NET Core Tutorial, we will be discussing the Startup Class, its significance, and its relevance for assisting an application in starting and running. Do you want to know more about ASP.NET concepts? Then hurry up to join our Advanced ASP.NET Core Certification Training!
Why Startup Class?
The Startup class in ASP.NET Core is like the control center of your application. It's the place where you configure services and the app's request pipeline. Imagine it as the design for your application launch. This class is important because it guarantees that your app was correctly configured before that point where it begins serving HTTP requests.
Program Class for Applications Infrastructure
Before we go deeper into the Startup class, it's essential to understand its companion: the Program class. The Program class is where your application begins its execution. It contains the Main method, which is the entry point of your app. In the Main method, an instance of the web host is created, which then uses the Startup class to configure the application.
This is a simple example of how the Program class appears:
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
}
Thus, the CreateHostBuilder method specifies that the app configuration process should use the Startup class.
Read More: Salary Offered to ASP.NET Developers |
Exploring the Startup Class
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
public void ConfigureServices(IServiceCollection services)
{
// Register services here
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// Configure the HTTP request pipeline here
}
}
Initializing with the Startup Constructor
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
}
This setup ensures that you can easily access configuration settings throughout the Startup class.
Registering Services with ConfigureServices
public void ConfigureServices(IServiceCollection services)
{
// Register services here
services.AddControllers();
services.AddDbContext<MyDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
}
This method allows you to add services, for example, MVC controllers, authentication, Entity Framework contexts etc.
Setting Up the Request Pipeline with Configure
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthentication();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
}
In this method, you configure middleware components like exception handling, HTTPS redirection, static files, routing, and more.
Where to Find the Startup Class?
Key Services in the Startup Class
- Configuration-Through dependency injection, you can access configuration settings.
- Logging-Set up logging to monitor your application's behavior.
- Dependency Injection-Register services and make them available throughout your application.
- Environment-Determine the current environment (Development, Staging, Production) and configure your app accordingly.
These services are crucial for building robust and scalable applications.
Conclusion
Read More: Top 50 ASP.NET Core Interview Questions and Answers for 2024 |
FAQs
Take our Aspnet skill challenge to evaluate yourself!
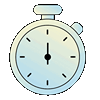
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.