01
FebUnderstanding ASP.NET Page Life Cycle
Asp.Net Page Life Cycle
As the ASP.NET page runs, it goes through a life cycle in which it performs a series of processing steps. Assume you're dealing with an ASP.NET web page, and each time it loads, it goes through a series of events or processes, from the time a request is made to when the final HTML is rendered in the browser. These steps are initialization, loading, validation, and rendering. Understanding this life cycle is critical for appropriately managing events, optimizing page performance, and designing more efficient code. Want to know how these events affect your development workflow? Let us break it down!
In this ASP.NET tutorial, we will exploretheAsp.net page life cycle and its different processing steps more deeply.
Note: If you are preparing for a .Net interview read the following article:
Top 50 .NET Interview Questions and AnswersWhat is the Asp.Net Page Life Cycle?
- The asp.net page life cycle begins, When a page is requested, it is loaded into the server memory, processed, and sent to the given browser.
- After that, it will unloaded from the memory.
- At each of these loading page steps, methods and events are present, which could be overridden according to the needs of the software.
- there are various page life cycle phases such as initialization, Instantiation, Restoration, Execution, and page rendering.
- Understanding the page cycle makes a difference in building codes for making a few particular things happen at any step of the page life cycle.
- It also makes a difference in writing custom controls and initializing them at the proper time.
- It populates their properties with view-state information, and running control behavior code.
Different stages of an ASP.NET page life-cycle:
The following chart shows the various stages of the page life cycle
Different ASP.NET Page Life Cycle Events
We have now seen the different stages of an ASP.NET page life-cycle at each stage, the page raises some events, Let's discuss them one by one.
1.PreInit
- First, check the IsPostBack property to see whether this is the first time the page has been processed or not.
- Then, Create or re-create dynamic controls.
- Now, dynamically set a master page.
- Also, Set the Theme property dynamically.
protected void Page_PreInit (object sender, EventArgs e)
{
}
2. Init
- This event fires after each control has been initialized.
- Each control has its UniqueID set.
- And any skin settings have been applied.
- We Used this event to read or initialize control properties.
- This event is fired first for the bottom-most control in the hierarchy and then fired up the hierarchy until it is fired for the page itself.
protected void Page_Init (object sender, EventArgs e)
{
}
3.InitComplete
- This event allows us to track the view state.
- It's all the controls that turn on view-state tracking.
protected void Page_InitComplete (object sender, EventArgs e)
{
}
4.LoadViewState
- This stage is also called OnPreLoad
- Raised after the page loads view state for itself and all controls and after it processes postback data that is included with the Request instance.
- It loads the view state for itself and all controls and then processes any postback data included with the Request instance,Before the Page instance raises this event,
- The ViewState data are loaded to controls.
- Then the Postback data are now handed to the page controls, This process is called Loads Postback data
protected void OnPreload (object sender, EventArgs e)
{
}
5.PreLoad
- It occurs before the post-back data is loaded in the controls.
- The PreLoad event can be handled by overloading the OnPreLoad method or creating a Page_PreLoad handler.
protected void Page_PreLoad (object sender, EventArgs e)
{
}
6. Load
- The Load event is raised for the page first and then recursively for all child controls.
- Hence, The controls in the control tree are created.
- This event can be managed by overloading the OnLoad method or creating a Page_Load handler.
protected void Page_Load (object sender, EventArgs e)
{
}
7.LoadComplete
- After completing the loading process, control event handlers run, and page validation takes place.
- LoadComplete event can be handled by overloading the OnLoadComplete method or creating a Page_LoadComplete handler.
protected void Page_LoadComplete (object sender, EventArgs e)
{
}
8.PreRender
- This event occurs right before the output is rendered.
- By handling PreRender events, pages, and controls can perform any updates before the output is rendered.
protected void Page_InitComplete (object sender, EventArgs e)
{
}
9.PreRenderComplete
- As this event is recursively fired for all child controls, this event ensures the completion of the pre-rendering phase.
protected void OnPreRender (object sender, EventArgs e)
{
}
10.SaveStateComplete
- At this stage, the State of control of the page is saved.
- During this event, Personalization, control state, and view state information are saved.
- The HTML markup is generated. This stage can be handled by overriding the Render method or creating a Page_Render handler.
protected void OnSaveStateComplete (object sender, EventArgs e)
{
}
11.UnLoad
- This phase is the last phase of the page life cycle.
- It raises the UnLoad event for all controls recursively and lastly for the page itself.
- When Final cleanup is done all resources and references, such as database connections, are freed.
- This event is handled by modifying the OnUnLoad method or creating a Page_UnLoad handler.
protected void Page_UnLoad (object sender, EventArgs e)
{
}
Example 1: Control Values
Now we will demonstrate an example of "How asp.net page lifecycle stages process on every step"
public partial class PageLifeCycle : System.Web.UI.Page
{
protected void Page_PreInit(object sender, EventArgs e)
{
// Work and assign values to label.
lblName.Text += "
" + "PreInit";
}
protected void Page_Init(object sender, EventArgs e)
{
// Work and assign values to label.
lblName.Text += "
" + "Init";
}
protected void Page_InitComplete(object sender, EventArgs e)
{
// Work and assign values to label.
lblName.Text += "
" + "InitComplete";
}
protected override void OnPreLoad(EventArgs e)
{
// Work and assign values to label.
// If the page is post back, then label control values will be loaded from view state.
// E.g.: If you string str = lblName.Text, then str will contain viewstate values.
lblName.Text += "
" + "PreLoad";
}
protected void Page_Load(object sender, EventArgs e)
{
// Work and assign values to label.
lblName.Text += "
" + "Load";
}
protected void btnSubmit_Click(object sender, EventArgs e)
{
// Work and assign values to label.
lblName.Text += "
" + "btnSubmit_Click";
}
protected void Page_LoadComplete(object sender, EventArgs e)
{
// Work and assign values to label.
lblName.Text += "
" + "LoadComplete";
}
protected override void OnPreRender(EventArgs e)
{
// Work and assign values to label.
lblName.Text += "
" + "PreRender";
}
protected override void OnSaveStateComplete(EventArgs e)
{
// Work and assign values to label.
// But "SaveStateComplete" values will not be available during post back, i.e., View state.
lblName.Text += "
" + "SaveStateComplete";
}
protected void Page_UnLoad(object sender, EventArgs e)
{
// Work and it will not affect label control, view state, and post back data.
lblName.Text += "
" + "UnLoad";
}
}
Output
At the very first time, the Page Load is output.
The following output will show When you click on the Submit Button output.
The following output will show when first time the page loads with EnableViewState="false".
When you click on the Submit Button output with EnableViewState="false":
Example 2: View State Values
Please review the code comments and output. It will help you to clearly understand the topics.
public partial class PageLifeCycle : System.Web.UI.Page
{
protected void Page_PreInit(object sender, EventArgs e)
{
// Work and assign values to label.
// Note: If page is post back or first time call and you have not set any values to ViewState["value"], then
// Convert.ToString(ViewState["value"]) is always empty.
ViewState["value"] = Convert.ToString(ViewState["value"]) + "
" + "PreInit";
lblName.Text = Convert.ToString(ViewState["value"]);
}
protected void Page_Init(object sender, EventArgs e)
{
// Work and assign values to label.
// Note: If page is post back or first time call and you have not set any values to ViewState["value"] in previous events, then
// Convert.ToString(ViewState["value"]) is always empty.
ViewState["value"] = Convert.ToString(ViewState["value"]) + "
" + "Init";
lblName.Text = Convert.ToString(ViewState["value"]);
}
protected void Page_InitComplete(object sender, EventArgs e)
{
// Work and assign values to label.
// Note: If page is post back or first time call and you have not set any values to ViewState["value"] in previous events, then
// Convert.ToString(ViewState["value"]) is always empty.
ViewState["value"] = Convert.ToString(ViewState["value"]) + "
" + "InitComplete";
lblName.Text = Convert.ToString(ViewState["value"]);
}
protected override void OnPreLoad(EventArgs e)
{
// Work and assign values to label.
// Note: If the page is post back and you have set or not set any values to ViewState["value"] in previous events, then
// Convert.ToString(ViewState["value"]) will always have post back data.
// E.g.: If you string str = Convert.ToString(ViewState["value"]), then str will contain post back values.
ViewState["value"] = Convert.ToString(ViewState["value"]) + "
" + "PreLoad";
lblName.Text = Convert.ToString(ViewState["value"]);
}
protected void Page_Load(object sender, EventArgs e)
{
// Work and assign values to label.
ViewState["value"] = Convert.ToString(ViewState["value"]) + "
" + "Load";
lblName.Text = Convert.ToString(ViewState["value"]);
}
protected void btnSubmit_Click(object sender, EventArgs e)
{
// Work and assign values to label.
ViewState["value"] = Convert.ToString(ViewState["value"]) + "
" + "btnSubmit_Click";
lblName.Text = Convert.ToString(ViewState["value"]);
}
protected void Page_LoadComplete(object sender, EventArgs e)
{
// Work and assign values to label.
ViewState["value"] = Convert.ToString(ViewState["value"]) + "
" + "LoadComplete";
lblName.Text = Convert.ToString(ViewState["value"]);
}
protected override void OnPreRender(EventArgs e)
{
// Work and assign values to label.
ViewState["value"] = Convert.ToString(ViewState["value"]) + "
" + "PreRender";
lblName.Text = Convert.ToString(ViewState["value"]);
}
protected override void OnSaveStateComplete(EventArgs e)
{
// Work and assign values to label.
// But "SaveStateComplete" values will not be available during post back, i.e., View state.
ViewState["value"] = Convert.ToString(ViewState["value"]) + "
" + "SaveStateComplete";
lblName.Text = Convert.ToString(ViewState["value"]);
}
protected void Page_UnLoad(object sender, EventArgs e)
{
// Work and it will not affect label control values, view state, and post back data.
ViewState["value"] = Convert.ToString(ViewState["value"]) + "
" + "UnLoad";
lblName.Text = Convert.ToString(ViewState["value"]);
}
}
Output
At the very first, the Time Page Load is output.
The following output will show When you click on the Submit Button output
During the first time, the page loads with EnableViewState="false":
The following result will show When you click on the Submit Button, the output with EnableViewState="false":
What is the Application Life Cycle?
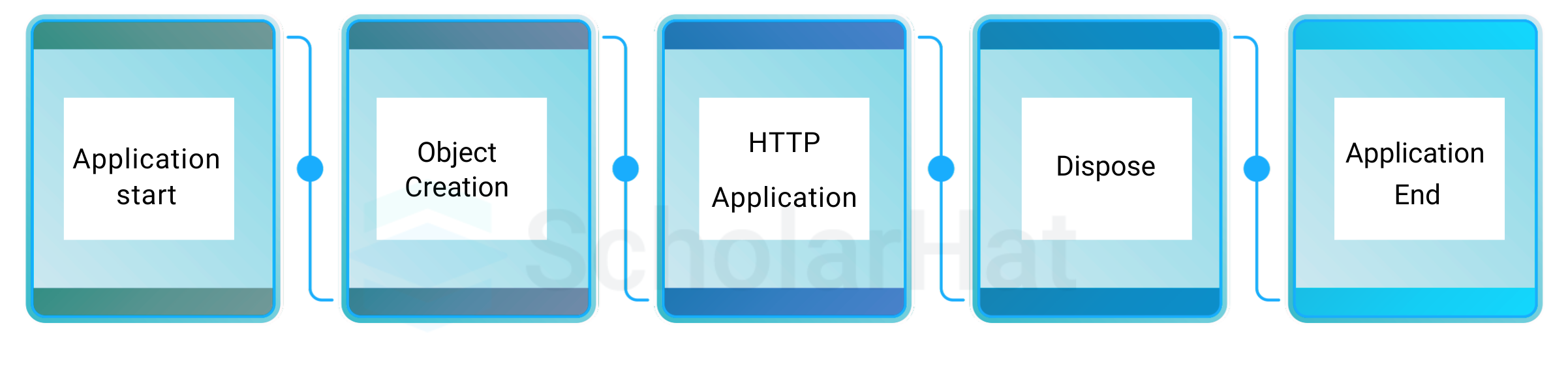
1. Application Start
When a C# application starts, the Application_Start event is called, which initializes global variables, services, and any other configuration required during the application's lifetime.
2. Object Creation.
Objects such as controllers, models, and custom classes are created dynamically during runtime. This technique facilitates the execution of business logic and the handling of HTTP requests.
3. HTTP Application.
In online applications, the HttpApplication class oversees the complete request-response pipeline, which includes handling incoming HTTP requests and creating appropriate responses.
4. Dispose
The Dispose method is used to remove unmanaged resources (such as database connections and file streams) that are no longer required, hence preventing memory leaks.
5. Application Ends
Finally, when the application terminates, the Application_End event is triggered, allowing for cleanup tasks such as disconnecting connections and reporting the shutdown.
Understanding these steps contributes to efficient resource management and seamless application performance.
Conclusion:
So in this article, we have learned about the Asp.net life cycle. I hope you enjoyed learning these concepts while programming with .Net. Feel free to ask any questions from your side. Your valuable feedback or comments about this article are always welcome. Consider our .NET Certification Training to learn .net from scratch. Also, consider learning the ASP.NET Core Course for a better understanding of .net concepts. Now Let's see what the Asp.net page life cycle is.