24
JanAbstract Class in C#
Abstract Class in C#
C# Abstract Classes are vital for developers seeking to implement object-oriented principles effectively while promoting code reusability and maintainability. Imagine being able to define a base class with common functionality that other derived classes can inherit and implement, ensuring a clear structure and consistent behavior across related classes. Abstract classes in C# offer a powerful way to establish a blueprint for other classes, enabling the enforcement of method implementation while allowing for shared code.
In this C# tutorial, I’ll explain Abstract Classes in c#, how they facilitate code organization and inheritance, and why mastering them is crucial for writing robust, scalable C# applications.
What is an abstract class?
- Abstraction can be achieved with either abstract classes or interfaces
- An abstract class is a special type of class that cannot be instantiated and acts as a base class for other classes.
- Abstract class members marked as abstract must be implemented by derived classes.
- An abstract class can be used as a base class, and all other derived classes should implement the abstract class definitions.
- All the abstract methods should be implemented in all non-abstract classes using the keyword called an override.
- After overriding, the abstract method is in the non-abstract class.
- We can derive this class in another class, and again, we can ultimately override the same abstract method with it.
- The purpose of an abstract class is to provide basic or default functionality as well as common functionality that multiple derived classes can share and override.
Syntax of Abstract Class:
Let's see the syntax of an abstract class in C# that has one abstract method declared, which is "drive()." Its implementation can be provided by derived classes as required.
using System;
public abstract class Car
{
public abstract void drive();
}
Example
A class library may define an abstract class that is used as a parameter to many of its functions, and programmers may be required to use that library to implement the class by creating a derived class. In C#, System.IO.FileStream is an implementation of the System.IO.Stream abstract class. Let's elaborate on this in C# Compiler.
using System;
namespace MyApplication
{
// Abstract class
abstract class Company
{
// Abstract method (does not have a body)
public abstract void CompanyName();
// Regular method
public void CompanyRevenue()
{
Console.WriteLine(" The Company Revenue is 3 Crore.");
}
}
// Derived class (inherit from Company)
class Employee : Company
{
public override void CompanyName()
{
Console.WriteLine("The Company Name is: DotNetTricks Innovation .");
}
}
class Program
{
static void Main(string[] args)
{
Employee emp = new Employee();
emp.CompanyName();
emp.CompanyRevenue();
}
}
}
Output
The Company Name is: DotNetTricks Innovation .The Company Revenue is 3 Crore.
Explanation
We have taken an abstract class called "Company" and an abstract method called "ComapanyName." We also took one regular method called" CompanyRevenue".We override an abstract method in the Derived class employee. Fetch properties of the base class by creating an object of the derived class. This is how abstraction works.
Read More - C# Programming Interview Questions
What is Abstract methods?
- A method that is declared as an abstract method has no “body,” and it can be declared inside the abstract class only, which is the thumb rule.
- An abstract method should be implemented in all non-abstract classes using the keyword called "override."
- After overriding the methods, the abstract method is in the context of a non-abstract class.
Syntax of abstract method:
public abstract void getData(); // where the method called 'getData()' is a abstract method
Features of Abstract Class
- An abstract class cannot be instantiated.
- An abstract class contains abstract members as well as non-abstract members.
- An abstract class cannot be a sealed class because the sealed modifier prevents a class from being inherited, and the abstract modifier requires a class to be inherited.
- A non-abstract class that is derived from an abstract class must include actual implementations of all the abstract members of the parent abstract class.
- An abstract class can be inherited from a class and one or more interfaces.
- An abstract class can have access modifiers such as private, protected, and internal with class members. However, abstract members cannot have a private access modifier.
- An Abstract class can have instance variables (like constants and fields).
- An abstract class can have constructors and destructors.
- An abstract method is implicitly a virtual method.
- Abstract properties behave like abstract methods.
- An abstract class cannot be inherited by structures.
- An abstract class cannot support multiple inheritances.
Example1:Program Demonstrating the Functionality of an Abstract Class
using System;
// Abstract class 'Animal'
public abstract class Animal
{
// Abstract method 'MakeSound()'
public abstract void MakeSound();
}
// Class 'Dog' inherits from 'Animal'
public class Dog : Animal
{
// Overriding the abstract method 'MakeSound()'
public override void MakeSound()
{
Console.WriteLine("Dog barks");
}
}
// Class 'Cat' inherits from 'Animal'
public class Cat : Animal
{
// Overriding the abstract method 'MakeSound()'
public override void MakeSound()
{
Console.WriteLine("Cat meows");
}
}
// Driver class
public class Program
{
// Main Method
public static void Main()
{
// 'animal' is an object of class 'Animal'
// Animal cannot be instantiated
Animal animal;
// Instantiate class 'Dog'
animal = new Dog();
// Call 'MakeSound()' of class 'Dog'
animal.MakeSound();
// Instantiate class 'Cat'
animal = new Cat();
// Call 'MakeSound()' of class 'Cat'
animal.MakeSound();
}
}
Output:
Dog barks
Cat meows
Explanation
- This C# program demonstrates the use of an abstract class, Animal, which defines an abstract method MakeSound().
- The Dog and Cat classes inherit from Animal and provide their own implementations of the MakeSound() method.
- In the Main method, instances of Dog and Cat are created, and their respective MakeSound() methods are invoked, showcasing polymorphism through method overriding.
Example2:Program to Calculate the Area of a Rectangle Using Abstract Class
using System;
// Declare class 'Shape' as abstract
abstract class Shape
{
// Declare method 'Area' as abstract
public abstract double Area();
}
// Class 'Rectangle' inherits from 'Shape'
class Rectangle : Shape
{
private double length;
private double width;
// Constructor
public Rectangle(double l, double w)
{
length = l;
width = w;
}
// The abstract method 'Area' is overridden here
public override double Area()
{
return length * width;
}
}
class Program
{
// Main Method
public static void Main()
{
Rectangle rect = new Rectangle(5.0, 3.0);
Console.WriteLine("Area of Rectangle = " + rect.Area());
}
}
Output
Area of Rectangle = 15
Explanation
- This C# program shows how to use the abstract Shape class, which defines the abstract function Area().
- The Rectangle class, which derives from Shape, implements the Area() function and determines the area by taking the length and width of the rectangle as input.
- The computed area is printed to the console, and a Rectangle instance is created using the main method.
Here are some key points to note about abstract classes in C#:
Example 1: Abstract Class with Non-Abstract Methods
using System;
// Abstract class 'MathOperations'
abstract class MathOperations
{
// Non-abstract method
public int Add(int num1, int num2)
{
return num1 + num2;
}
// Abstract method
public abstract int Subtract(int num1, int num2);
}
// Derived class 'SimpleMath' inheriting from 'MathOperations'
class SimpleMath : MathOperations
{
// Implementing the abstract method 'Subtract'
public override int Subtract(int num1, int num2)
{
return num1 - num2;
}
}
// Driver Class
class Program
{
// Main Method
public static void Main()
{
// Create an instance of the derived class
SimpleMath math = new SimpleMath();
Console.WriteLine("Addition: " + math.Add(10, 5)); // Output: Addition: 15
Console.WriteLine("Subtraction: " + math.Subtract(10, 5)); // Output: Subtraction: 5
}
}
Output
Addition: 15
Subtraction: 5
Explanation
- This code demonstrates the use of an abstract class in C#.
- The abstract class MathOperations contains a non-abstract method, Add, that performs addition, and an abstract method, Subtract, that must be implemented in any derived class.
- The SimpleMath class inherits from MathOperations and provides an implementation for the Subtract method.
- In the Main method, an instance of SimpleMath is created, allowing both the addition and subtraction operations to be performed and displayed.
Example 2: Abstract Class with Get and Set Accessors
using System;
// Abstract class 'Person'
abstract class Person
{
protected string name;
// Abstract property
public abstract string Name
{
get;
set;
}
}
// Derived class 'Student' inheriting from 'Person'
class Student : Person
{
// Implementing the abstract property 'Name'
public override string Name
{
get { return name; }
set { name = value; }
}
}
// Driver Class
class Program
{
// Main Method
public static void Main()
{
Student student = new Student();
student.Name = "Alice"; // Setting the name using the property
Console.WriteLine("Student Name: " + student.Name); // Output: Student Name: Alice
}
}
Output:
Student Name: Alice
Explanation
- This code illustrates the use of abstract properties in C#.
- The abstract class Person defines an abstract property Name, which is implemented in the derived class Student.
- In the Main method, an instance of Student is created, and the Name property is set and accessed, displaying the student's name as "Alice."
Common design guidelines for Abstract Class
- Don't define public constructors within an abstract class. Since an abstract class cannot be instantiated, constructors with public access modifiers provide visibility to the classes that can be instantiated.
- Define a protected or an internal constructor within an abstract class since a protected constructor allows the base class to do its own initialization when sub-classes are created, and an internal constructor can be used to limit concrete implementations of the abstract class to the assembly that contains that class.
What is the use of abstract class in C#?
- You need to create multiple versions of your component since versioning is not a problem with abstract classes.
- You can add properties or methods to an abstract class without breaking the code, and all inheriting classes are automatically updated with the change.
- Need to provide default behaviors as well as common behaviors that multiple derived classes can share and override.
Advantages of Abstract Classes:
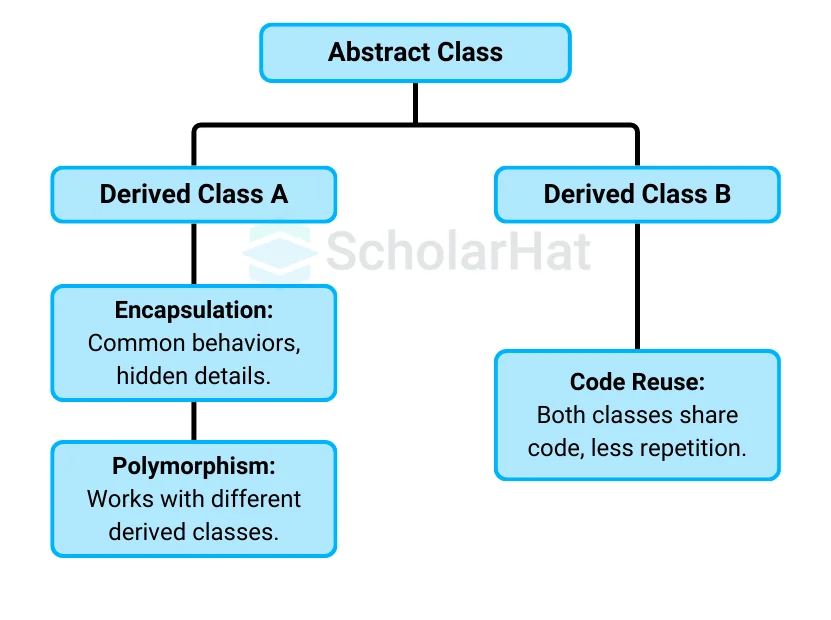
- Encapsulation: Abstract classes let you set common behaviors or properties for derived classes without showing the details of how they work. This makes your code easier to manage and change.
- Code Reuse: You can use an abstract class as a base for several derived classes. This helps reduce repeated code and makes your codebase cleaner.
- Polymorphism: Abstract classes allow you to write code that can work with objects of different derived classes as long as they all inherit from the same abstract base class. This adds flexibility to your code.
Disadvantages of Abstract Classes:
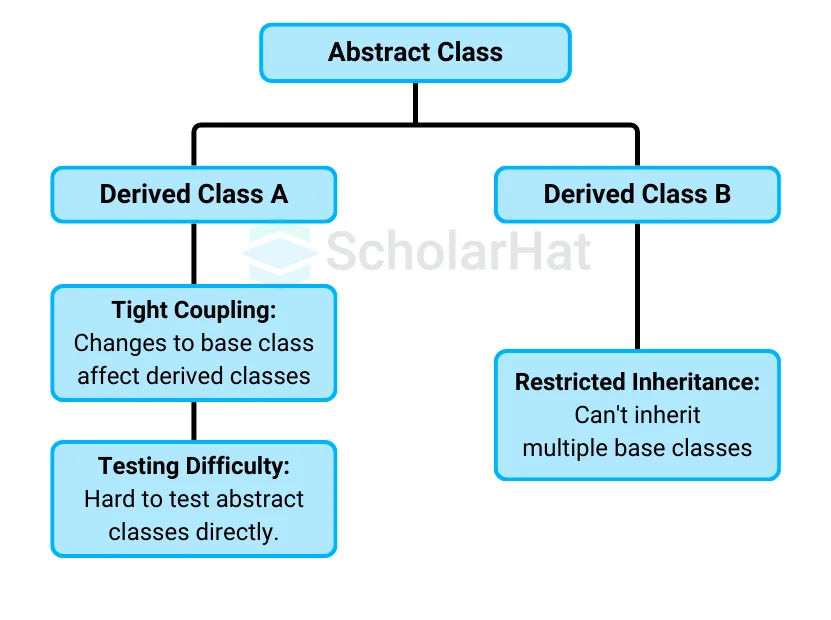
- Tight Coupling: The base class and derived classes can be closely linked by using abstract classes. Because of this, altering the base class without also altering the derived ones may be challenging.
- Restricted Inheritance: A class in C# is limited to inheriting from a single base class. The ability of your derived classes to inherit from other classes is restricted if you use an abstract class.
- Testing Difficulty: Since abstract classes cannot be explicitly created, testing may be more difficult.
Summary
Abstract classes in C# are essential for implementing object-oriented principles, allowing developers to define a base class that cannot be instantiated and serves as a blueprint for derived classes. They facilitate the creation of methods and properties that must be implemented by any non-abstract subclasses, promoting code reusability and maintainability. By providing common functionality, abstract classes enable a structured approach to building applications, making it easier to manage shared behavior while allowing for specific implementations in derived classes. To master the C# concept, enroll now in Scholarhat's C# Programming Course.
FAQs
Take our Csharp skill challenge to evaluate yourself!
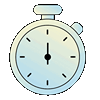
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.