30
DecArrays in C#: Create, Declare and Initialize the Arrays
Arrays in C#
C# arrays are useful for developers who need to store and manage large amounts of data efficiently. Imagine being able to arrange several items of the same kind, such as integers or strings, into a single data structure, resulting in better organized, maintainable, and efficient code. Arrays enable you to work with ordered elements, providing convenient access and manipulation of data in a sequential manner without the need for extra collection frameworks.
In this C# tutorial, I’ll explain C# Arrays, how they simplify data management by storing multiple values in one structure, and why mastering them is crucial for writing efficient, organized C# code.
What Are Arrays in C#?
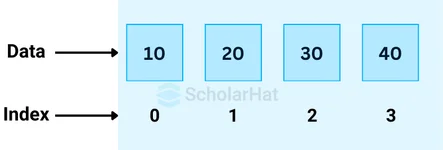
- Arrays in C# are a special type of collection that allows you to store multiple elements of the same type in a single, fixed-size structure.
- They can be used with primitive types like int, float, or complex objects and even with multidimensional arrays.
- When you use an array, it behaves as a sequential container, making your data organized and easier to access using indexed positions.
- Arrays are defined by specifying the type of elements they will hold, followed by square brackets [ ].
- For example, you can create an array of integers or strings to hold multiple values in a single variable.
When to Use Arrays in C#
There are specific scenarios where arrays in C# are especially useful:
- Fixed-Size Data Collection: If you know the exact number of elements beforehand, arrays are ideal for holding a fixed-size data set.
- Efficient Access: Arrays allow for fast access to elements using their index, making them perfect when performance is critical.
- Multi-dimensional Data: Arrays are useful when working with grids, tables, or any data that can be structured in multiple dimensions.
Read More: Types of Arrays in C#: Single-dimensional, Multi-dimensional, and Jagged Array |
Characteristics of Arrays
- Fixed Size: When you create an array, you decide its size upfront. After that, you cannot change its size. This is like renting an apartment; you can only have the number of rooms that were available when you signed the lease.
- Zero-Based Indexing: In C#, the first element of an array is at index 0. This means that if you want to access the first item, you will use index 0, the second item at index 1, and so forth. Understanding this is crucial for correctly accessing array elements.
- Homogeneous Elements: All elements in an array must be of the same type. If you create an integer array, you can only store integers in it. This is similar to having a box that can only hold apples. You can’t put oranges in it.
- Memory Allocation: Arrays are stored in contiguous memory locations, which means they are allocated next to each other in memory. This allows for quick access to each element.
Differences Between Arrays in C# and C/C++
- C# arrays are objects derived from the
System.Array
class, which means they come with built-in properties and methods. You get additional features that make handling arrays easier. - In contrast, C/C++ arrays are simpler and lack these features. You don’t have methods or properties, which means you need to manage everything manually.
Important Points to Remember About Arrays in C#
- Dynamic Allocation: While you need to specify the size of an array when you create it, the memory for arrays is allocated on the heap. This means that arrays can be created dynamically during runtime, giving you flexibility.
- Default Values: Each element in an array is automatically assigned a default value. For example, integers are set to 0, strings are set to
null
, and booleans are set to false. This helps prevent errors when accessing elements you haven’t explicitly set. - Multi-Dimensional Arrays: You can create arrays that have more than one dimension, like a table with rows and columns. This is useful for handling complex data structures, such as matrices in mathematics.
- Jagged Arrays: These are arrays of arrays, allowing for flexibility in storing data. For example, you might have an array that holds multiple arrays of different lengths, like class scores from various subjects.
Array Declaration and Initialization
Array Declaration
- Before you can use an array, you need to declare it. This tells C# what type of data the array will hold.
- Basic syntax:
<Data Type>[] <Name_Array>;
- For example, if you want to create an array of integers named
numbers
, you would declare it like this:
int[] numbers;
Array Initialization
- After declaring an array, you must initialize it. This means you are allocating memory and assigning values to the array elements.
- Initialization can be done in several ways, making it flexible based on your needs.
Syntax for Initialization:
< Data Type >[] < Name_Array > = new < Data Type >[size];
- For instance, if you want to initialize an integer array with 5 elements, you can do it like this:
int[] numbers = new int[5];
Initializing with Values:
- You can also initialize an array directly with values. This is a convenient way to set your array at the time of creation.
- Here’s an example of initializing an integer array with values:
int[] numbers = new int[] { 1, 2, 3, 4, 5 };
Using Shorthand Initialization:
- You can use shorthand initialization to create and initialize an array in one line.
- For example, you can declare and initialize an array of integers like this:
int[] numbers = { 1, 2, 3, 4, 5 };
Creating an Array
- To create an array, you can use the
new
keyword, along with the type of data you want to store. - Here’s how to create a string array containing Indian names:
- This creates an array that can hold three names, and you’ve initialized it with three popular Indian names.
string[] names = new string[3] { "Aarav", "Vivaan", "Aditya" };
Accessing Array Elements
Access by Index: To retrieve or assign values to an array, you can use the index of the element you want to access. Remember that array indices start at 0.
// Accessing and updating array elements
int firstNumber = numbers[0]; // Access the first element
numbers[1] = 25; // Update the second element
Looping Through Arrays
It’s common to use loops to access each element of an array, especially when you want to perform an operation on every item in the array.
Using a for Loop:
- The for loop is ideal when you need to access array elements by their index.
for (int i = 0; i < numbers.Length; i++) {
Console.WriteLine(numbers[i]);
}
Using a foreach Loop:
- If you don’t need the index, a foreach loop provides a simpler way to iterate over the array.
foreach (int number in numbers) {
Console.WriteLine(number);
}
Types of Arrays in C#
1. One-Dimensional Arrays
- The simplest type of array is one-dimensional. It’s a list of items stored in a single row.
string[] cities = { "Mumbai", "Delhi", "Chennai", "Kolkata" };
2. Multi-Dimensional Arrays
- Multi-dimensional arrays let you store data in a grid-like format. For example, a two-dimensional array represents a matrix.
int[,] matrix = { { 1, 2 }, { 3, 4 }, { 5, 6 } };
// Accessing an element in a two-dimensional array
int value = matrix[1, 1]; // Returns 4
3. Jagged Arrays
A jagged array is an array of arrays. Unlike multi-dimensional arrays, the inner arrays can have different lengths.
int[][] jaggedArray = new int[3][];
jaggedArray[0] = new int[] { 1, 2 };
jaggedArray[1] = new int[] { 3, 4, 5 };
jaggedArray[2] = new int[] { 6 };
Array Class Properties
- The
Array
class in C# provides several useful properties that make working with arrays easier. - Length: This property gives you the total number of elements in the array. It’s handy when you need to know how many items you’re working with.
- Rank: This property tells you the number of dimensions in the array. For instance, a one-dimensional array has a rank of 1, while a two-dimensional array has a rank of 2.
- Here’s how to access these properties:
int length = names.Length; // Gets the length of the names array
int rank = names.Rank; // Gets the rank of the names array
1. Search an Element in an Array
- Searching for an element in an array can be done using the
Array.IndexOf
method. - This method returns the index of the first occurrence of the specified value.
- Here’s an example of how to search for an element in the names array:
- If the name is found,
index
it will hold its position; if not, it will return -1.
int index = Array.IndexOf(names, "Aarav"); // Searches for "Aarav"
2. Sorting an Array
- Sorting an array in C# can be done easily using the
Array.Sort
method. - This method sorts the elements in ascending order by default.
- Here’s how you can sort the names array:
- After sorting, the names will be arranged alphabetically.
Array.Sort(names); // Sorts the names array in ascending order
3. Getting and Setting Values
- You can access and modify the elements of an array using their index.
- This allows you to get or set values easily.
- To get a value, simply refer to its index. Here’s an example:
- If you want to change a value, use the index to set a new value:
string firstName = names[0]; // Gets the first name in the array
names[1] = "Rohan"; // Sets the second name to "Rohan"
4. Reverse an Array in C#
- If you need to reverse the order of elements in an array, you can use the
Array.Reverse
method. - This is helpful when you want to display the elements in reverse order.
- Here’s how to reverse the names array:
- After this operation, the last name becomes the first, and so on.
Array.Reverse(names); // Reverses the order of the names array
5. Clear an Array
- Sometimes, you might want to reset the values of an array without creating a new one.
- You can achieve this using the
Array.Clear
method. - This method sets a range of elements in the array to their default value. Here’s an example:
- After executing this, all the names will be set to, effectively clearing the array.
Array.Clear(names, 0, names.Length); // Clears the names array
6. Get the Size of an Array
- To find out how many elements are in an array, you can use the
Length
property. - This tells you the total number of elements currently stored in the array.
- Here’s how to get the size of the numbers array:
- This is useful for loops or when you need to know how many items you're working with.
int size = numbers.Length; // Gets the size of the numbers array
7. Copying an Array
- You can use this method to duplicate an array.
- This allows you to create a copy of the original array into another array.
- Here’s how to copy the numbers array:
- After this operation,
copiedArray
will hold the same elements asnumbers
.
int[] copiedArray = new int[5];
Array.Copy(numbers, copiedArray, numbers.Length); // Copies the numbers array to copiedArray
8. Clone an Array
- You can create a clone of an array using the
Clone
method. - This creates a new array that is a copy of the original array.
- Here’s how to clone the numbers array:
- After cloning,
clonedArray
contains the same elements asnumbers
, but they are two separate arrays.
int[] clonedArray = (int[])numbers.Clone(); // Clones the numbers array
9. Convert an Array to a List
- You can convert an array to a
List
using theToList
method fromSystem.Linq
. - This is useful when you want to take advantage of the dynamic nature of lists.
- Here’s how to convert the numbers array to a list:
- Now,
numberList
can grow or shrink as you add or remove elements, unlike the fixed-size array.
using System.Linq;
List numberList = numbers.ToList(); // Converts the numbers array to a List
Practical Examples and Use Cases
Example 1: Storing Student Grades
- Imagine you are building a simple application to store student grades. You can use an array to store these grades.
using System;
class Program
{
static void Main(string[] args)
{
int[] studentGrades = new int[5];
// Assign grades
studentGrades[0] = 85;
studentGrades[1] = 90;
studentGrades[2] = 78;
studentGrades[3] = 88;
studentGrades[4] = 92;
// Calculate the average grade
int sum = 0;
foreach (int grade in studentGrades)
{
sum += grade;
}
double average = sum / (double)studentGrades.Length;
Console.WriteLine("The average grade is: " + average);
}
}
Example 2: Managing Employee Salaries
- You might need to keep track of employee salaries in an organization. Here’s how you can do that using an array:
using System;
class Program
{
static void Main(string[] args)
{
decimal[] salaries = { 30000m, 45000m, 50000m, 60000m };
// Increase each salary by 10%
for (int i = 0; i < salaries.Length; i++)
{
salaries[i] *= 1.1m;
}
Console.WriteLine("Updated Salaries:");
foreach (decimal salary in salaries)
{
Console.WriteLine(salary);
}
}
}
Example 3: Processing Sales Data
- If you’re handling sales data, you can use an array to store sales amounts for a month and calculate total sales.
using System;
class Program
{
static void Main(string[] args)
{
decimal[] sales = { 1000m, 1500m, 2000m, 2500m, 3000m };
decimal totalSales = 0;
foreach (decimal sale in sales)
{
totalSales += sale;
}
Console.WriteLine("Total Sales for the month: " + totalSales);
}
}
Summary
Arrays in C# are a fixed-size data structure that allows storing multiple elements of the same type, such as integers or strings, in a sequential manner. They enable efficient access to elements via indexing and are best suited for cases in which the number of elements is known in advance. C# offers one-dimensional, multi-dimensional, and jagged arrays, as well as useful attributes like length and methods for sorting, reversing, and searching elements. Understanding arrays is critical for building efficient and ordered code in C#.FAQs
Take our Csharp skill challenge to evaluate yourself!
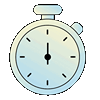
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.