11
JulC# Attributes
Attributes in C#-An Overview
C# Attributes play a crucial role in enhancing code readability, providing metadata, and enabling runtime behaviors. This article explores the fundamental concepts of C# attributes, demonstrating their usage and impact on various aspects of software development, from documentation to customization.
Enroll in the C Sharp Full Course For Free to gain a deeper understanding of C# attributes and their applications!
What is Attributes in C# ?
In C#, attributes provide metadata that can be added to program entities such as classes, methods, or properties. They convey information about the elements they decorate, influencing behaviors or providing additional information for tools and compilers.
Properties of Attributes in C#
- Type: Specifies the data type of the attribute.
- Positional Parameters: Allow passing values to attribute constructor.
- Named Parameters: Provide named values for attribute properties.
- Targets: Define where the attribute can be applied (e.g., class, method).
- Inheritance: Determine if the attribute is inherited by subclasses.
- Usage Restrictions: Set constraints on attribute usage.
- Reflection: Attributes can be accessed and manipulated at runtime.
- AttributeTargets Enumeration: Specifies valid attribute targets.
- Multiple Attributes: Apply multiple attributes to a single target.
- Custom Attributes: Create user-defined attributes for specific behaviors.
Predefined Attributes
Predefined attributes are those that are supported by the C# compiler for a particular use and are a component of the.NET Framework Class Library. The System is the source of several of the preset properties. The following are basic classes for attributes.
Attribute | Description |
AttributeUsageAttribute | This property describes how to use an alternative attribute. |
CLSCompliantAttribute | This property indicates whether or not a specific code element conforms with the Common Language Specification. |
ContextStaticAttribute | This property indicates that different contexts shouldn't share a static field. |
FlagsAttribute | The ability to utilize an enumeration as a collection of flags is indicated by the FlagsAttribute. Bitwise operators are the most typical applications for this. |
LoaderOptimizationAttribute | In the main method, this property determines the optimization policy for the default loader. |
NonSerializedAttribute | This property indicates that serializing the serializable class field is not recommended. |
ObsoleteAttribute | This characteristic designates code components that are out-of-date, or no longer in use. |
SerializableAttribute | This property indicates that the serializable class's field is serializable. |
ThreadStaticAttribute | This property shows that every thread has a different static field value. |
DllImportAttribute | According to the unmanaged DLL, this characteristic shows that the method is a static entry point. |
CLSCompliantAttribute
This property indicates whether or not a specific code element conforms with the Common Language Specification. if a certain piece of code conforms to the Common Language Specification. The compiler will then display a warning message if it doesn't.
Example
// C# program to demonstrate CLSCompliantAttribute
using System;
// CLSCompliantAttribute applied to entire assembly
[assembly:CLSCompliant(true)]
public class DNT {
// Main Method
public static void Main(string[] args)
{
Console.WriteLine("DotNetTricks");
}
}
Explanation
This C# program demonstrates the use of the CLSCompliantAttribute applied to the entire assembly, ensuring adherence to the Common Language Specification (CLS). The attribute is set to true, indicating CLS compliance, and the program prints "DotNetTricks" to the console in the Main method.
Output
DotNetTricks
FlagsAttribute
The ability to utilize an enumeration as a collection of flags is indicated by the FlagsAttribute. Bitwise operators are the most typical applications for this.
Example
// C# program to demonstrate FlagsAttribute
using System;
class DNT {
// Enum defined without FlagsAttribute.
enum Colours { Red = 10,
Blue = 20,
Pink = 40,
Green = 80
}
// Enum defined with FlagsAttribute.
[Flags] enum ColoursFlags { Red = 10,
Blue = 20,
Pink = 40,
Green = 80
}
// Main Method
public static void Main(string[] args)
{
Console.WriteLine((Colours.Red | Colours.Blue).ToString());
Console.WriteLine((ColoursFlags.Red | ColoursFlags.Blue).ToString());
}
}
Explanation
For the Colours enum without FlagsAttribute, the bitwise OR operation results in the sum of the enum values (Red + Blue), which is 30. For the ColoursFlags enum with FlagsAttribute, the ToString() method displays the combined enum values using commas, indicating a bitwise OR operation.
Output
30
Red, Blue
ObsoleteAttribute
Code elements that are no longer in use are designated as obsolete using the ObsoleteAttribute. A compiler error occurs when one calls these out-of-date code parts.
Example
// C# program to demonstrate ObsoleteAttribute
using System;
class DNT {
// The method1() is marked as obsolete
[Obsolete("method1 is obsolete", true)] static void method1()
{
Console.WriteLine("This is method1");
}
static void method2()
{
Console.WriteLine("This is method2");
}
public static void Main(string[] args)
{
method1(); // Compiler error as method1() is obsolete
method2();
}
}
Explanation
The output of this code would not be applicable since it contains a compilation error. The method1() is marked as obsolete with the ObsoleteAttribute, and the second parameter is set to true, indicating it is an error if the obsolete method is used. Therefore, attempting to call method1() will result in a compiler error, and method2() will not be executed due to the error in the code.
Custom Attributes
In C#, custom attributes may be made to connect declarative data in any necessary way to methods, assemblies, properties, types, etc. This makes the.NET framework more extensible. How to Make Custom Attributes:
- Describe a System-derived custom attribute class. Class attributes.
- The suffix Attribute should appear at the end of the custom attribute class name.
- To indicate how the constructed custom attribute class will be used, use the attribute AttributeUsage.
- Construct the custom attribute class's constructor and its available properties.
Conclusion
In conclusion, C# attributes enhance code by providing metadata that influences the behavior of the program. They offer a powerful way to convey additional information, improve readability, and enable advanced features. Utilizing attributes wisely contributes to cleaner, more efficient, and maintainable C# code.
Take our Csharp skill challenge to evaluate yourself!
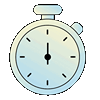
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.