11
JulC Sharp Delegates and Plug-in Methods with Delegates
Delegate in C# is a reference type that holds the reference of a class method. Any method that has the same signature as a delegate can be assigned to a delegate. A delegate is like a function pointer. There are three aspects to a delegate: Declaration, Instantiation, and Invocation.
In this C# Tutorial, we will explore more about C Sharp Delegates and Plug-in Methods which will include the Use of delegate, delegate chaining with an example, types of delegates, single cast, and multicast delegates, properties of delegates, delegate with plugin methods.
To deepen your understanding of delegates and other C# concepts, be sure to enroll in our Free C Sharp Course for a comprehensive learning experience.
Declaration:
A delegate is declared by using the keyword delegate, otherwise it resembles a method declaration.
delegate int delegateAdd (int x,int y);
Instantiation:
To create a delegate instance, we need to assign a method (which has the same signature as a delegate) to delegate.
static int Add (int x,int y)
{
return x+y;
}
..
//create delegate instance
delegateAdd objAdd= new delegateAdd (Add);
//short hand for above statement
delegateAdd objAdd=Add;
Invocation:
Invoking a delegate is like invoking a regular method.
// Invoke delegate to call method
int result = objAdd.Invoke (3,6);
//short hand for above statement
int result = objAdd (3,6)
Read More - C# Interview Questions For Freshers
Plug-in Methods with Delegates
A method can be assigned to a delegate instance dynamically. This is useful for writing plug-in methods. In the below example, The SquareData method has a delegate parameter, for specifying a plug-in SquareData.Let's elaborate on this in C# Compiler.
public delegate int delegateSquare(int x);
class Util
{
public static void SquareData(int[] arr, delegateSquare obj)
{
for (int i = 0; i < arr.Length; i++)
arr[i] = obj(arr[i]);
}
}
class demo
{
static void Main()
{
int[] arr = { 1, 2, 3 };
Util.SquareData(arr, Square); // Dynamically hook in Square
foreach (int i in arr)
Console.Write(i + " "); // 1 4 9
}
static int Square(int x)
{
return x * x;
}
}
Types of Delegates
Single cast Delegate
A single cast delegate holds the reference of only a single method. The above-created delegates are single-cast delegates.
Multicast Delegate
A delegate that holds the reference of more than one method is called a multicast delegate. A multicast delegate only contains the reference of methods which return type is void. The + and += operators are used to combine delegate instances.
MyDelegate d = Method1;
d = d + Method2;
//short hand for above statement
d += Method2;
Now, Invoking d will call both methods - Method1 and Method2. Methods are invoked in the order in which they are added.
The - and -= operators are used to remove a method from the delegate instances.
d -= Method1;
Now, Invoking d will invoke only Method2.
Multicast delegate example
delegate void Delegate_Multicast(int x, int y);
class demo
{
static void Method1(int x, int y)
{
int z=x+y;
Console.WriteLine("Method1 is called");
Console.WriteLine("\n Sum is : {0}",z);
}
static void Method2(int x, int y)
{
int z=x+y;
Console.WriteLine("\n Method2 is called");
Console.WriteLine("\n Sum is : {0}",z);
}
public static void Main()
Delegate_Multicast dmulti = Method1;
dmulti += Method2;
dmulti(1, 2); // Method1 and Method2 are called
dmulti -= Method1;
dmulti(2, 3); // Only Method2 is called
}
}
Output:
Method1 is called
Sum is : 3
Method2 is called
Sum is : 5
Note
Delegates are immutable in nature, so when you call += or -=, a new delegate instance is created and it is assigned to the existing delegate instance.
All delegates are implicitly derived from the System.MulticastDelegate, a class that is inherited from System.Delegate class.
Delegate types are all incompatible with each other, even if their signatures are the same.
delegate void D1(); delegate void D2(); ... D1 d1 = Method1; D2 d2 = d1; // Compile-time error D2 d2 = new D2 (d1); // correct
Delegate instances are considered equal if they have the reference of the same method.
delegate void D(); ... D d1 = Method1; D d2 = Method1; Console.WriteLine (d1 == d2); // True
Multicast delegates are considered equal if they reference the same methods in the same order.
Delegates are used in event handling.
What do you think?
In this article, I try to explain the delegates and their types with examples. I hope after reading this article you will be able to understand delegates. I would like to have feedback from my blog readers. Please post your feedback, questions, or comments about this article. Also, Consider our C# Programming Course for a better understanding of all C# concepts
FAQs
Take our Csharp skill challenge to evaluate yourself!
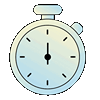
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.