11
JulC Sharp Generic delegates Func, Action and Predicate with anonymous method
Generic delegates in C#: An Overview
In .net 3.5 some new generic delegates -Func<T>, Action<T>, and Predicate<T> were introduced. Using generic delegates, it is possible to concise delegate type which means you don’t have to define the delegate statement. These delegates are the Func<T>, Action<T>, and Predicate<T> delegates and are defined in the System namespace.
In this C# Tutorial, we will explore more about generic delegates which will include generic delegates with examples, func action predicates with examples, and Generic delegates using an anonymous method.
To gain a deeper understanding of exception handling and other essential C# concepts, you can enroll in a C Sharp Online Course Free. This course will provide you with the knowledge and hands-on experience needed to master exception handling and other important C# topics.
What are the generic delegates?
- Delegates in C# are nothing but pointers to function.
- They are used for implementing events and call-back methods.
- Func, Action, and Predicate are the delegates defined in C# 3.0
- Func, Action, and Predicate are generic inbuilt delegates.
- Let's see the types of delegates with its example in C# Compiler.
1.Func Delegate in C#:
- Func is a generic delegate present in the System namespace.
- Func takes one or more input parameters and returns one out parameter and the last parameter is considered as a return value.
- It can include 0 to 16 input parameters of different types but it must have one return type.
Example:
Func delegate with two input parameters
Func func1 = DelegateClass.Add;
int value = func1(22, 44);
TParameter = 22,44;
TOutput = value = 66;
Func delegate with Anonymous Methods
Func with Anonymous methods:
Func func= delegate(intx,int y){ return (x+y); };
int result = func4(2,3);
2. Action Delegate in C#
- Action present in the System namespace.
- It takes one or more than one input parameter and returns nothing.
- It does not return any value.
Example:
Action delegate with two input parameters
Action action1=DelegateClass.ShowEmploye;
action1(44,"Jayanti");
TParameter = 44,Jayanti;
TOutput = Not available (No return value)
Action delegate with Anonymous Methods
Action action = delegate(String msg)
{
Console.WriteLine(msg);
};
action("Jayanti");
3. Predicate Delegate in C#
- A predicate delegate is also an inbuilt generic delegate and is present in the System namespace.
- predicate is used to verify certain criteria of the method and returns output as Boolean, either True or False.
- The predicate can be used with the method, anonymous, and lambda expressions.
Example:
Predicate delegate with two input parameters
Predicate predicate = DelegateClass.IsNumeric;
bool number = predicate("987654");
Predicate delegate with Anonymous Methods
Predicate predicate = delegate(string str)
{
double retNum;
bool isNum = Double.TryParse(Convert.ToString(str), System.Globalization.NumberStyles.Any, System.Globalization.NumberFormatInfo.InvariantInfo, out retNum);
return isNum;
};
bool found = predicate("987654");
Generic delegates in a nutshell
Action<T> operates on the generic arguments. Func<T> performs an operation on the argument(s) and returns a value, and Predicate<T> is used to represent a set of criteria and determine if the argument matches the criteria.
delegate TResult Func ();
delegate TResult Func (T arg);
delegate TResult Func (T1 arg1, T2 arg2);
... up to T16
delegate void Action ();
delegate void Action (T arg);
delegate void Action (T1 arg1, T2 arg2);
... up to T16
Here "in" shows the input parameters and "out" shows the return value by the delegate.
Read More - C# Interview Questions For Freshers
Generic delegate example
using System;
class demo
{
delegate void MyDelegate(string str);
static void Main(string[] args)
{
MyDelegate d = show;
d("Hello World!");
Console.ReadLine();
}
static void show(string str)
{
Console.WriteLine(str);
}
}
The above code can be written using a generic delegate.
using System;
class demo
{
static void Main(string[] args)
{
Action<string> d = show;
d("Hello World!");
Console.ReadLine();
}
static void show(string str)
{
Console.WriteLine(str);
}
}
Generic delegate using an anonymous method
using System;
class demo
{
static void Main(string[] args)
{
Action<string> d = s => Console.WriteLine(s);
d("Hello World!");
}
}
Summary
In this article, I try to explain the generic delegates with examples. I hope after reading this article you will be able to understand the use of generic delegates. I would like to have feedback from my blog readers. Please post your feedback, questions, or comments about this article. Also, Consider our C# Programming Course for a better understanding of all C# concepts.
FAQs
Take our Csharp skill challenge to evaluate yourself!
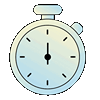
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.