18
AprC# String
String in C#
Strings in C# are a fundamental part of any application, playing a vital role in handling and manipulating text. Imagine you're tasked with creating applications that require efficient text processing, security, and performance optimization. Working with C# strings ensures you have the skills to manage text data effectively while understanding how immutability and memory management impact your applications.
In this C# tutorial, I'll break down the key concepts of working with strings, common methods for string manipulation, and why mastering them is essential for building high-performing, reliable applications. Let’s dive in! Enroll in the C Sharp Full Course For Free to master string manipulation and other essential C# concepts!
What is the C# string?
- In C#, a string is a data type that stores character sequences such as words or sentences.
- It is immutable, which means that once produced, the string's value cannot be modified, and any modification causes a new string to be formed.
- Strings are often used to handle text and may be readily modified with the .NET framework's built-in functions.
Real-world Analogy
C# strings are similar to printed book pages. Once printed, the words on the page cannot be changed immediately. If you need to alter something, you must print a new page with the changes.
Explanation
- The original string is like a book page.
- Modifying the string creates a whole new page, just as modifications to a C# string create a new string.
String vs. System.String
In C#, string is an alias for System. String. They are functionally the same, but understanding this distinction can help clarify the language's syntax and structure.
Immutability of String Objects
- Strings in C# are immutable, meaning that once a string object is created, it cannot be altered.
- This feature has implications for memory management and performance.
- When you manipulate strings, a new string object is created instead of modifying the original.
Properties of the String Class
The String class has several key properties:
- Chars: Gets the Char object at a specified position in the current String object.
- Length: Gets the number of characters in the current String object.
Creating a String Object
Methods of Creating Strings
- Create a string from a literal: This is the most common way to create a string. A user defines a string variable and assigns a value within double quotes.
- Create a string using concatenation: Combine strings using the + operator.
- Create a string using a constructor: Use the String class's constructor to create a string from a character array.
- Create a string using a property or a method: Retrieve a property or call a method that returns a string.
- Create a string using formatting: Use string interpolation or formatting methods to create strings.
Create a string from a literal
This is the most common way to create a string. You define a string variable and assign the value within double quotes. You can use any type of characters within double quotes except some special characters like a backslash (\).
Example: To illustrate the string creation using literals
// C# program to demonstrate the
// string creation using literals
using System;
class Program {
static void Main(string[] args) {
string str1 = "Hello, World!";
Console.WriteLine(str1);
// Using double slash to escape
string str2 = "C:\\Users\\Amit\\Documents\\file.txt";
Console.WriteLine(str2);
}
}
Output
Hello, World!
C:\Users\Amit\Documents\file.txt
Explanation
- This C# program shows how to build strings from literals.
- The str1 variable contains the basic string "Hello, World!" which is printed directly.
- The str2 variable demonstrates the usage of escape sequences (double backslashes \\) to indicate a file path, ensuring that special characters such as backslashes are appropriately handled.
Create a string using concatenation
You can create a string by using the string concatenation operator +
in C#. This operator combines one or more strings.
Example: To illustrate the use of the string concatenation operator
// C# program to demonstrate the use of
// the string concatenation operator
using System;
class Program {
public static void Main() {
string firstName = "Amit";
string lastName = "Kumar";
// Using concatenation operator
string fullName = firstName + " " + lastName;
Console.WriteLine(fullName);
}
}
Output
Amit Kumar
Explanation
- This C# program explains the string concatenation operator (+).
- It combines two string variables, firstName and lastName, with a space between them to form a full name, which is then written to the console as "Amit Kumar".
Create a string using a constructor
The String class has several overloaded constructors that take an array of characters or bytes. You can create a string from a character array.
Example: To illustrate the creation of a string using the constructor
// C# program to demonstrate the creation
// of string using the constructor
using System;
class Program {
public static void Main() {
char[] chars = { 'A', 'M', 'I', 'T' };
// Create a string from a character array.
string str1 = new string(chars);
Console.WriteLine(str1);
}
}
Output
AMIT
Explanation
- This C# programshows how to create a string using the String constructor.
- It generates a string from a character array of chars that contains individual characters ('A', 'M', 'I', and 'T').
- The resulting string, "AMIT," is then displayed on the console.
Create a string using a property or a method
You can create a string by retrieving a property or calling a method that returns a string. For example, using methods of the String class to extract a substring from a larger string.
Example: To illustrate the creation of a string using a property or a method
// C# program to extract a substring from a larger
// string using methods of the String class
using System;
class Program {
public static void Main() {
string sentence = "Amit Kumar Singh";
// Extracting a substring
string firstName = sentence.Substring(0, 4);
Console.WriteLine(firstName);
}
}
Output
Amit
Explanation
- This C# program shows how to extract a substring from a bigger string by calling the String class's Substring function.
- In this example, the program extracts the first four characters ("Amit") of the text "Amit Kumar Singh" and displays the result on the console.
Create a string using formatting
The String.Format
method is used to convert values or objects to their string representation. You can also use string interpolation to create strings.
Example: To illustrate the creation of a string using the format method
// C# program to demonstrate the creation of string using format method
using System;
class Program {
public static void Main() {
int age = 25;
string name = "Amit";
// String creation using string.Format method
string str = string.Format("{0} is {1} years old.", name, age);
Console.WriteLine(str);
}
}
Output
Amit is 25 years old.
Explanation
- This C# programexplains the use of a string.
- The Format method generates a formatted string.
- In this example, the computer inserts the values of the variable's name and age into a sentence, yielding the output "Amit is 25 years old."
- This demonstrates how to dynamically format strings based on variable values.
Common String Methods
Length of a String
- You can find the length of a string using the
Length
property.
Join Two Strings
- Use the
String.Concat
orString.Join
methods to concatenate strings.
Compare Two Strings
- You can compare two strings using the
String.Compare
method.
Contains
- Check if a substring exists within a string using the
Contains
method.
Getting a Substring
- Use the
Substring
method to extract a part of a string.
String Escape Sequences
- Special characters can be included in strings using escape sequences. For example, use
\"
for quotes and\\
for a backslash.
String Interpolation
- String interpolation allows you to embed expressions within string literals, making it easier to format strings.
Example of Common String Methods in C#
using System;
class Program {
public static void Main() {
// Creating strings
string firstName = "Amit";
string lastName = "Kumar";
// 1. Length of a String
int length = firstName.Length;
Console.WriteLine($"Length of '{firstName}': {length}"); // Output: Length of 'Amit': 4
// 2. Join Two Strings
string fullName = String.Concat(firstName, " ", lastName);
Console.WriteLine($"Full Name: {fullName}"); // Output: Full Name: Amit Kumar
// 3. Compare Two Strings
int comparisonResult = String.Compare(firstName, lastName);
Console.WriteLine($"Comparison Result (firstName vs lastName): {comparisonResult}"); // Output: Negative, since 'Amit' is less than 'Kumar'
// 4. Contains
bool contains = fullName.Contains("Amit");
Console.WriteLine($"Does fullName contain 'Amit'? {contains}"); // Output: True
// 5. Getting a Substring
string subString = fullName.Substring(0, 4);
Console.WriteLine($"Substring of fullName (first 4 chars): {subString}"); // Output: Substring of fullName (first 4 chars): Amit
// 6. String Escape Sequences
string filePath = "C:\\Users\\Amit\\Documents\\file.txt";
Console.WriteLine($"File Path: {filePath}"); // Output: File Path: C:\Users\Amit\Documents\file.txt
// 7. String Interpolation
string greeting = $"Hello, {firstName} {lastName}!";
Console.WriteLine(greeting); // Output: Hello, Amit Kumar!
// 8. Immutability of String Objects
firstName = "Raj"; // This does not change the original string; it creates a new string instead
Console.WriteLine($"New firstName: {firstName}"); // Output: New firstName: Raj
}
}
Output
Length of 'Amit': 4
Full Name: Amit Kumar
Comparison Result (firstName vs lastName): -1 (or any negative number, because 'Amit' is lexicographically less than 'Kumar')
Does fullName contain 'Amit'? True
Substring of fullName (first 4 chars): Amit
File Path: C:\Users\Amit\Documents\file.txt
Hello, Amit Kumar!
New firstName: Raj
Explanation
- This C# program demonstrates a variety of string techniques and ideas.
- It covers string creation, length computation, and concatenation using String.
- Concatenation and comparison of two strings using String.
- Compare and use Contains to check for substrings, Substring to extract substrings, handle escape sequences, string interpolation, and string immutability.
Summary
C# strings are an important data type for managing and manipulating text in applications. They are immutable, which means that once generated, they cannot be changed, affecting memory management and performance. This article covers a variety of string creation methods, including literals, concatenation, constructors, and formatting, as well as typical string methods and properties. Understanding C# strings is critical for successful text processing and application development.To master more C# concepts, join Scholarhat'sC# Programming Course.
FAQs
Take our Csharp skill challenge to evaluate yourself!
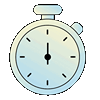
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.