21
FebC Sharp Var data type and Anonymous Type: An Overview
Var data type and Anonymous Type in C#: An Overview
We know that datatypes in c# play a vital role in programming. But what if it is difficult to declare a variable's datatype when the type is not known? and also the code is too complex. To handle this problem, we have var data type and anonymous type in C#.
In this C# tutorial, we will learn when to use var data type and anonymous type and how to use var data type and anonymous type.
To dive deeper into the use of var and anonymous types and enhance your C# skills, you can enroll in a C Sharp Online Course Free. This course will provide you with hands-on experience and practical knowledge to master these data types and other important C# concepts.
What is the Var data type?
Declaring Valid var statements:
Let's elaborate on this in C# Compiler:
using System;
using System.Text;
using System.Collections;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
var str = "1";
var num = 0;
string s2 = null;
string s3 = null;
var s4 = s3;
Console.WriteLine("{0}\n{1}\n{2}\n{3}\n{4}", str, num , s2 , s3 , s4);
Console.Read();
}
}
}
Output
10
Compiled statements
string str = "1";0;
string s4 = s3;
Read More - C# Interview Questions For Freshers
Declaring the array with the var keyword in C#
using System;
using System.Text;
using System.Collections;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
var aInt = new int[] { 5, 6, 3, 8,41, 2 };
foreach (var item in aInt)
{
Console.Write( item.ToString()+ Environment.NewLine);
}
Console.Read();
}
}
}
Output
5
6
3
8
41
2
Var application in the treatment of ArrayList
using System;
using System.Linq;
using System.Text;
using System.Collections;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
ArrayList list = new ArrayList();
list.Add("One");
list.Add("Two");
list.Add(3);
list.Add(4);
Console.WriteLine("Total: {0}\n", list.Count);
foreach(var a in list)
{
Console.WriteLine(a);
}
Console.Read();
}
}
}
Output
Total: 4
One
Two
3
4
The example above shows the advantage of var when no predefined data types for variables are known. Firstly, we created one list and in turn assigned the data as One, Two, 3, and 4. Then use a foreach loop to browse the elements in the list, but this list is initialized with two types of values, hence it is difficult to browse the list. But if we use the var then everything becomes very easy, because no matter what the data type is, the program will automatically cast.
What is Anonymous Types?
Anonymous types allow you to create new types without defining them.This is an easy way to define read-only properties on a single object without explicitly defining the type. An anonymous type is a simple class generated by the compiler in IL (Intermediate Language) to store a set of values.Create an anonymous type with data type 'var' and the keyword 'new'. We have seen how the var data type works.Now let's see how anonymous types work with the "new" keyword.
Implementing Anonymous Types
using System;
using System.Text;
using System.Collections;
namespace DemoApplication1
{
class __Anonymous1
{
static void Main(string[] args)
{
var emp = new {
Name = "Deepak", Address = "Noida", Salary=21000
};
Console.WriteLine("First Name : " + emp.Name);
Console.WriteLine("Local Address : " + emp.Address);
Console.WriteLine("salary : " + emp.Salary);
Console.Read();
}
Output:
First Name: Deepak
Local Address: Noida
salary: 21000
Implementing Nested Anonymous Type
using System;
public class Employee_info {
// Main method
static public void Main()
{
// Creating and initializing nested anonymous object
var emp_object = new {e_id = 11, s_name = "Pragya", profile = "C# Developer",
emp_ob = new { age = 27}};
// Accessing the object properties
Console.WriteLine("Employee id: " + emp_object.e_id);
Console.WriteLine("Employee name: " + emp_object.s_name);
Console.WriteLine("Profile: " + emp_object.profile);
Console.WriteLine("age: " + emp_object.emp_ob.age);
}
Output
Employee id: 11
Employee name: Pragya
Profile: C# Developer
age: 27
Implementing Anonymous type in LINQ
using System;
using System.Collections.Generic;
using System.Linq;
class Employee {
public int A_no;
public string Aname;
public string language;
public int age;
}
class GFG {
static void Main()
{
List<Employee> g = new List<Employee>
{
new Employee{ A_no = 22, Aname = "Mahesh",
language = "C#", age = 23 },
new Employee{ A_no = 12, Aname = "Kunal",
language = "Java", age = 20 },
new Employee{ A_no = 34, Aname = "Ayog",
language = "Python", age = 22 },
new Employee{ A_no = 55, Aname = "Preeti",
language = "CPP", age = 25 },
};
var emp_ob = from emp in g select new {emp.A_no, emp.Aname, emp.language};
foreach(var i in emp_ob)
{
Console.WriteLine("Employee id = " + i.A_no + "\nEmployee name = "
+ i.Aname + "\nEmployee Language = " + i.language);
Console.WriteLine();
}
}
}
Output
id = 22
Employee name = Mahesh
Employee Language = C#
Employee id = 12
Employee name = Kunal
Employee Language = Java
Employee id = 34
Employee name = Ayog
Employee Language = Python
Employee id = 55
Employee name = Preeti
Employee Language = CPP
Unlock the next level of C#
Summary
In this article, I tried to explain var data type with examples and also anonymous type with a new keyword and their examples with different aspects. I hope after reading this article you will be able to use these tricks in your code. I would like to have feedback from my blog readers. Please post your feedback, questions, or comments about this article. Also don't forget to enroll in our .Net Training Course for a better understanding of .net concepts. Enjoy coding..!
FAQs
Take our Csharp skill challenge to evaluate yourself!
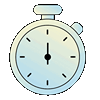
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.