21
NovC# Destructor
C# Destructor- An Overview
A C# destructor is a critical memory management component in C# programming. This article explores the role and significance of destructors in C# applications, providing insights into their usage, best practices, and how they differ from constructors. Understanding destructors is essential for writing efficient and resource-friendly C# code.
What is a Destructor in C#?
In C#, a destructor is a special method used to clean up resources and perform finalization tasks before an object is destroyed. It is defined using the tilde (~) symbol followed by the class name without any parameters. Destructors are automatically called by the garbage collector when an object is no longer in use, allowing developers to release unmanaged resources and free memory.
Syntax
class Example
{
// Rest of the class
// members and methods.
// Destructor
~Example()
{
// Your code
}
}
Important points to remember about C# Destructor
- Name: Destructors have the same name as the class prefixed with a tilde (~).
- No Parameters: Destructors don't take any parameters and can't be explicitly called.
- Finalization: They are used for finalization, automatically called by the garbage collector when an object is about to be destroyed.
- Resource Cleanup: Ideal for releasing unmanaged resources like files, database connections, etc.
- Implicitly Defined: If not explicitly defined, C# provides a default destructor that performs basic cleanup.
- No Overloading: Destructors can't be overloaded or inherited. Each class can have only one destructor.
- Limited Use: With C# relying on automatic memory management, explicit destructors are rarely necessary. Consider implementing IDisposable interface and Dispose() method for resource management instead.
Need of Destructor in C#
In C#, destructors are essential for managing resources and ensuring proper cleanup within an object-oriented program. Here's why they are needed:
- Resource Management: Destructors help release unmanaged resources such as file handles, database connections, and network sockets when an object is no longer needed.
- Memory Cleanup: They are crucial for releasing managed resources like objects and memory, preventing memory leaks, and improving overall application performance.
- Garbage Collection: Destructors facilitate automatic garbage collection in C# by allowing objects to be finalized before being removed from memory, preventing memory leaks and enhancing system efficiency.
- Custom Cleanup Logic: Destructors allow developers to implement custom cleanup logic, ensuring that specific operations are performed before an object is destroyed, leading to more predictable and reliable code behavior.
- Class Hierarchies: Inheritance chains often involve resource management. Destructors enable proper cleanup throughout the hierarchy, promoting clean and maintainable code.
In summary, destructors in C# are indispensable for efficient resource management, memory cleanup, and ensuring the overall stability and performance of applications.
Example 1 of destructor in C#
using System;
namespace CsharpDestructor {
class Person {
public Person() {
Console.WriteLine("Constructor called.");
}
// destructor
~Person() {
Console.WriteLine("Destructor called.");
}
public static void Main(string [] args) {
//creates object of Person
Person p1 = new Person();
}
}
}
Explanation
The provided C# code defines a Person class with a constructor and a destructor. When the Main method is executed, it creates an object of the Person class. The Person object p1 is created in the Main method, triggering the constructor, which prints "Constructor called." When the program exits, the destructor of the Person class is automatically called, printing "Destructor called." This happens during the cleanup process when the program exits, indicating that the object is being finalized and resources are being released.
Output
Constructor called.
Destructor called.
Example 2 of destructor in C#
// C# Program to illustrate how
// a destructor works
using System;
namespace DotnetTricks {
class Complex {
// Class members, private
// by default
int real, img;
// Defining the constructor
public Complex()
{
real = 0;
img = 0;
}
// SetValue method sets
// value of real and img
public void SetValue(int r, int i)
{
real = r;
img = i;
}
// DisplayValue displays
// values of real and img
public void DisplayValue()
{
Console.WriteLine("Real = " + real);
Console.WriteLine("Imaginary = " + img);
}
// Defining the destructor
// for class Complex
~Complex()
{
Console.WriteLine("Destructor was called");
}
} // End class Complex
// Driver Class
class Program {
// Main Method
static void Main(string[] args)
{
// Creating an instance of class
// Complex C invokes constructor
Complex C = new Complex();
// Calling SetValue method using
// instance C Setting values of
// real to 5 and img to 6
C.SetValue(5, 6);
// Displaying values of real
// and imaginary parts
C.DisplayValue();
// Instance is no longer needed
// Destructor will be called
} // End Main
} // End class Program
}
Explanation
An instance of the Complex class is created using Complex C = new Complex();, invoking the constructor, which initializes real and img to 0. The SetValue(5, 6); method sets the values of real to 5 and img to 6. DisplayValue(); method prints the values of real and img. When the program exits, the destructor of the Complex class is automatically called, printing "Destructor was called." This demonstrates the cleanup process when the object goes out of scope.
Output
Real = 5
Imaginary = 6
Destructor was called
Conclusion
In conclusion, C# destructors are vital for resource cleanup, ensuring efficient memory management, and preventing leaks in object-oriented programs. They facilitate the automatic disposal of resources, enhancing the language's robustness and reliability, making C# a powerful choice for developers seeking effective memory management solutions.
Take our Csharp skill challenge to evaluate yourself!
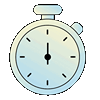
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.