21
FebDifference between int, Int16, Int32 and Int64
The Int DataType: An Overview
In the learning phase, developers are not very aware of the difference between primitive, FCL (framework class library), reference, and value types. This causes bugs and performance issues in the code. there are different behaviors of integer types. In this C# Tutorial, we will explore more about C Sharp int datatypewhich will include C# int Int16 Int32 Int64, int vs Int16 vs Int32 vs Int64.
To deepen your understanding of C# data types and improve your coding skills, you can enroll in a C Sharp Course Free and start learning today!
int
It is a primitive data type defined in C#.
It is mapped to Int32 of FCL type.
It is a value type and represents a System.Int32 struct.
It is signed and takes 32 bits.
It has a minimum of -2147483648 and a maximum of +2147483647 capacity.
Example
Let's elaborate more about int datatype in C# Compiler
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
namespace HelloWorld
{
public class Program
{
public static void Main(string[] args)
{
{
// Integer value
int a = 9;
Console.WriteLine("Integer value="+ a);
}
}
}
}
Output
Integer value=9
Read More - C# Programming Interview Questions
Int16
It is a FCL type.
In C#, the short is mapped to Int16.
It is a value type and represents the System.Int16 struct.
It is signed and takes 16 bits.
It has a minimum -32768 and maximum +32767 capacity.
Example:
using System;
using System.Text;
public class Scholarhat
{
static void Main(string[] args)
{
Console.WriteLine("Int16 MinValue: "+ Int16.MinValue);
Console.WriteLine("Int16 MaxValue: "+ Int16.MaxValue);
Console.WriteLine();
Int16[] arr1 = {-5, 1, 4, 4, 8};
foreach (Int16 i in arr1)
{
Console.WriteLine(i);
}
}
}
Output
Int16 MinValue: -32768
Int16 MaxValue: 32767
-5
1
4
4
8
Int32
It is an FCL type.
In C#, int is mapped to Int32.
It is a value type and represents the System.Int32 struct.
It is signed and takes 32 bits.
It has a minimum -2147483648 and a maximum +2147483647 capacity.
Example:
using System;
using System.Text;
public
class Scholarhat {
static void Main(string[] args) {
Console.WriteLine("Int32 MinValue: "+ Int32.MinValue);
Console.WriteLine("Int32 MaxValue: " + Int32.MaxValue);
Console.WriteLine();
Int32[] arr1 = {-4, 0, 3, 2, 9};
foreach (Int32 i in arr1)
{
Console.WriteLine(i);
}
}
}
Output
int32 MinValue: -2147483648
Int32 MaxValue: 2147483647
-4
0
3
2
9
Int64
It is a FCL type.
In C#, the long is mapped to Int64.
It is a value type and represents the System.Int64 struct.
It is signed and takes 64 bits.
It has a minimum of 9,223,372,036,854,775,808 and a maximum of 9,223,372,036,854,775,807 capacity.
Example:
using System;
using System.Text;
public class Scholarhat {
static void Main(string[] args) {
Console.WriteLine("Int64 MinValue: "+ Int64.MinValue);
Console.WriteLine("Int64 MaxValue: " + Int64.MaxValue);
Console.WriteLine();
Int64[] arr1 = {-4, 0, 3, 5, 9};
foreach (Int64 i in arr1)
{
Console.WriteLine(i);
}
}
}
Output
Int64 MinValue: -9223372036854775808
Int64 MaxValue: 9223372036854775807
-4
0
3
5
9
Note
A number of developers think that int represents a 32-bit integer when the application is running on a 32-bit OS and it represents a 64-bit integer when the application is running on a 64-bit OS. This is absolutely wrong.
In C# int is a primitive data type and it is always mapped to System.Int32 whether the OS is 32-bit or 64-bit.
Difference between int, Int16, Int32 and Int64:
Conclusion:
I hope you will enjoy the tips while programming with C#. I would like to have feedback from my blog readers. Your valuable feedback, questions, or comments about this article are always welcome. Also, Consider our C# Programming Course for a better understanding of all C# concepts.
FAQs
Take our Csharp skill challenge to evaluate yourself!
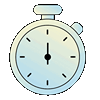
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.