11
JulUnderstanding Factorial Program in C#: With Code Examples
Factorial Program in C#
Factorial program using Csharpis a fun way to practice loops, iteration, and recursion, it will strengthen your basic programming skills and help you to build logic. If we want to get the factorial of the number 5, it is simply 5 multiplied by every number below it like 5 * 4 * 3 * 2 * 1. This is just a simple method, but you can solve a factorial program using iteration or recursion using sharp too.
In this C# Tutorial, we are gonna see how to write a factorial program in C# including what is the logic of the factorial program, Factorial Program Using Iteration in C#, Factorial Program Using Recursion in C#, Factorial of a Number Using for, while, and do-while Loops in C# etc. To deepen your understanding and gain practical skills, you can enroll in a C Sharp Online Course Free. This course will guide you through essential C# programming concepts, including factorial programs, and help you become proficient in implementing such logic effectively.
What is a Factorial concept?
So, Mathematically, the factorial of n is represented as:
n! = n × (n - 1) × (n - 2) × ... × 1
For example:
Factorial Program: Key Concepts in C#
When writing a factorial program in C#, you should understand the following key concepts :
- Iteration: Iteration refers to repeatedly repeating a series of operations until a condition is met.
- Recursion: Recursion is simply a function that calls itself to solve a smaller version of the same problem.
- Loops: You can use different types of loops in C# such as
for
,while
, anddo-while
to implement repetitive calculations. - Base Case: The base case is a condition that prevents unlimited function calls in recursive implementations.
3 Ways to Calculate Factorial Program in C#
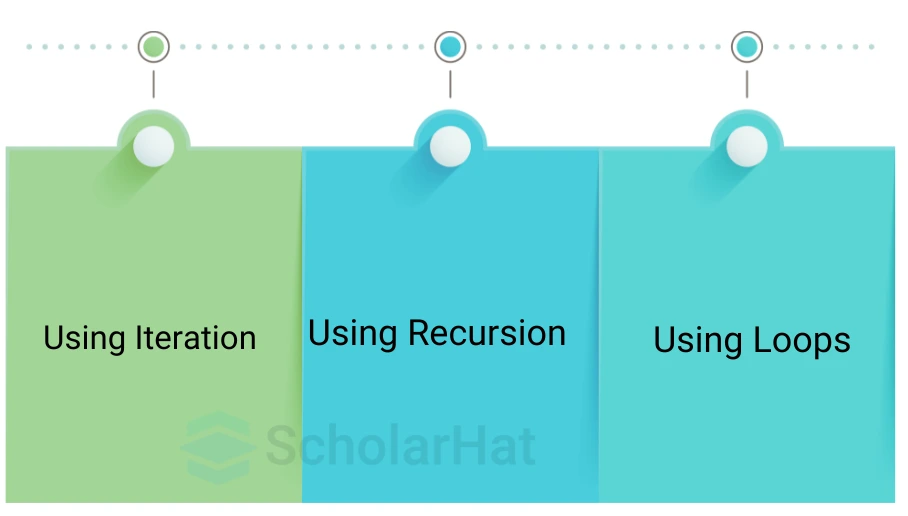
1. Writing a Factorial Program Using Iteration in C#
Iteration is one of the most common methods to calculate the factorial. In this strategy, we utilize a loop to multiply the numbers in decreasing order, from n to 1.
using System;
class FactorialProgram
{
static void Main()
{
int number = Convert.ToInt32(Console.ReadLine());
long result = CalculateFactorial(number);
Console.WriteLine($"Factorial of {number} is {result}");
}
static long CalculateFactorial(int n)
{
long factorial = 1;
for (int i = n; i > 1; i--)
{
factorial *= i;
}
return factorial;
}
}
Output
Factorial of 23 is 8128291617894825984
Explanation:
- The for loop iterates from n down to 1, multiplying the current value of factorial by i in each step.
- The result is then returned and printed.
2. Writing a Factorial Program Using Recursion in C#
- Recursion is an additional common technique to compute factorials.
- A function with recursion calls itself with a smaller issue until it encounters a base case (for example, n = 0 or n = 1).
using System;
class FactorialProgram
{
static void Main()
{
Console.Write("Enter a number: ");
int number = Convert.ToInt32(Console.ReadLine());
long result = FactorialRecursive(number);
Console.WriteLine($"Factorial of {number} is {result}");
}
static long FactorialRecursive(int n)
{
if (n <= 1)
return 1;
else
return n * FactorialRecursive(n - 1);
}
}
Output
Factorial of 23 is 8128291617894825984
Explanation:
- The FactorialRecursive() function calls itself with n-1 until n equals 1.
- The base case ensures that the recursion terminates when n is less than or equal to one.
3. Factorial of a Number Using for, while, and do-while Loops in C#
In C#, loops provide different ways to implement iterative calculations like factorial. Let's explore how we can calculate the factorial using for, while, and do-while loops.
1. Factorial Program Using for Loop
using System;
class Program
{
static void Main()
{
int number, factorial = 1;
// Taking input from the user
Console.Write("Enter a number: ");
number = Convert.ToInt32(Console.ReadLine());
// Calculating factorial using for loop
for (int i = 1; i <= number; i++)
{
factorial *= i;
}
// Output the result
Console.WriteLine($"Factorial of {number} is: {factorial}");
}
}
Output
Factorial of 23 is 8128291617894825984
Explanation
2. Factorial Program Using while Loop
using System;
class FactorialProgram
{
static void Main()
{
Console.Write("Enter a number: ");
int number = Convert.ToInt32(Console.ReadLine());
long result = FactorialWhile(number);
Console.WriteLine($"Factorial of {number} is {result}");
}
static long FactorialWhile(int n)
{
long factorial = 1;
int i = n;
while (i > 1)
{
factorial *= i;
i--;
}
return factorial;
}
}
Output
Factorial of 23 is 8128291617894825984
3. Factorial Program Using do-while Loop
using System;
class FactorialProgram
{
static void Main()
{
Console.Write("Enter a number: ");
int number = Convert.ToInt32(Console.ReadLine());
long result = FactorialDoWhile(number);
Console.WriteLine($"Factorial of {number} is {result}");
}
static long FactorialDoWhile(int n)
{
long factorial = 1;
int i = n;
do
{
factorial *= i;
i--;
} while (i > 1);
return factorial;
}
}
Output
Factorial of 23 is 8128291617894825984
Performance Comparison: Iteration vs Recursion for Factorial in C#
Both iterative and recursive approaches have their advantages and drawbacks when calculating factorials.
1. Iteration
- Efficiency: In terms of efficiency, Iteration is generally more efficient in terms of memory usage because it doesn’t involve the overhead of function calls.
- Stability: Iterative approaches handle large values of
n
without running into issues like stack overflow. - Speed: Iteration tends to be faster for large inputs due to fewer operations.
2. Recursion
- Readability: Recursive solutions are often more intuitive and closely mirror the mathematical definition of factorial.
- Drawbacks: Recursive functions consume more memory because each function call is added to the stack.
For small inputs, both approaches perform well. However, for large values (e.g., n > 5000), the recursive approach may throw an exception due to excessive memory usage, while the iterative approach handles it smoothly.
Read More: If you are preparing for the C# exam or interview "Top 50 C# Interview Questions and Answers "Might help you.
Conclusion
Finally, we explored many ways to calculate a factorial program in C#, including the factorial program using Iteration in C#, the factorial program using recursion in C#, and the use of loops such as for, while, and do-while. While recursion provides an elegant method, iteration is more efficient, especially with large inputs. Understanding these different approaches might assist you in selecting the best solution based on the specific requirements of your challenge. Consider our .NET Certification training because it provides a comprehensive understanding of C# programming language
FAQs
Take our Csharp skill challenge to evaluate yourself!
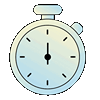
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.