26
OctEfficient File Handling in C#: Techniques and Tips
File Handling in C#
File handling in C# is an important topic that every developer asks for. Suppose you're on an adventure in a world where crucial data is stored in different files. File handling in C# is like having a unique key that helps you open, create, and change these files. By learning how to use File handling in C#, you'll be able to read data, write new information, and manage files, making your programs more valuable and exciting.
This C# Tutorial article will guide you to collect information about what is file handling in C#, including file and directory, why do we require file handling, working with Files in C#, StreamWriter Class, StreamReader Class, methods of file handling in C#, File Handling in C# with example, and many more.
What are Files and Directories in programming?
The Files and Directories in programming are:
- File: A file in programming is a group of data or information that is kept on a computer and that a program may generate, read, or edit.
- Directory: A directory is a folder on a computer used in programming that stores files and other directories and aids in the organized organization of data.
What is Stream?
A stream is a way to transfer or process data continuously, often one piece at a time, instead of handling everything at once. Streams are commonly used in programming to read or write files, process data from networks, or handle input/output operations. There are two types of streams that are explained below:
- Input Stream: Reads data from a source into a program.
- Output Stream:Writes data from a program to a destination.
What is File handling in C#?
Let us understand file handling in C# through some points explained below. Just check this out:
- Thanks to C #'s file-handling functionality, programs may create, read, write, and manage files on the computer.
- To efficiently communicate with files, classes like File, StreamReader, and StreamWriter are used.
- You have the ability to write new data, read data from a file, and add data to an already existing file.
- Numerous file kinds, including binary and text files, are supported via file handling.
- It also offers ways to move, copy, and verify the presence of files.
File Handling Class Hierarchy Diagram
- The Object class is the root of all .NET classes.
- Across application domains, objects can communicate thanks to MarshalByRefObject.
- The fundamental class for managing files and directories, FileSystemInfo, offers common methods and attributes.
- FileInfo provides for file operations such as creation, deletion, and transferring. It represents a file.
- Directories may be created and enumerated using DirectoryInfo, which is a representation of a directory.
- Static methods for file operations, such as reading and writing, are provided by the File class.
- Path offers functions for manipulating directory and file paths.
- Static methods for directory operations are provided by the Directory class.
- DriveInfo provides details on drives, such as their overall size and free space.
Why do we use File Handling in C#?
There are multiple reasons to use file handling in C#, and here, we have explained most important some of them:
- C# file handling saves data eternally, enabling programs to retain data long after they have closed.
- It makes it simple to process or retrieve information as needed by enabling reading and writing data to files.
- File handling allows for the management of large volumes of data outside of memory, which is crucial for effectiveness and performance.
- It offers a means of generating logs, storing user input, and saving configuration settings for later access.
- Creating reports and storing crucial data are two further ways that file management facilitates organized data organization.
Getting Started with Files in C#
Before you can work with files in C#, you need to include the System.IO
namespace:
using System.IO;
Basic File Operations
There are several basic file operations in C#. We have explained each of them step by step:
1. Creating a File in C#
You can use this method to create a new file. Here’s a complete and runnable example:
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = "example.txt";
using (FileStream fs = File.Create(filePath))
{
Console.WriteLine("File created successfully.");
}
}
}
Output
File created successfully.
2. Writing to a File
You can write text to a file using the StreamWriter
class. Here’s how to do it:
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = "example.txt";
using (StreamWriter writer = new StreamWriter(filePath))
{
writer.WriteLine("Hello, this is a test file.");
writer.WriteLine("This file is used for file handling examples in C#.");
}
Console.WriteLine("Data written to file successfully.");
}
}
Output
Data written to file successfully.
3. Reading from a File
To read data from a file, use the StreamReader
class. Here’s a complete example:
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = "example.txt";
using (StreamReader reader = new StreamReader(filePath))
{
string line;
Console.WriteLine("Reading from file:");
while ((line = reader.ReadLine()) != null)
{
Console.WriteLine(line);
}
}
}
}
Output
Reading from file:
Hello, this is a test file.
This file is used for file handling examples in C#.
4. Appending to a File
If you want to add more content to an existing file without deleting the current content, you can use StreamWriter
with append: true
:
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = "example.txt";
using (StreamWriter writer = new StreamWriter(filePath, append: true))
{
writer.WriteLine("Appending this line to the existing file.");
}
Console.WriteLine("Data appended to file successfully.");
}
}
Output
Data appended to file successfully.
5. Deleting a File
To delete a file, use the File.Delete()
method. Here’s a complete example:
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = "example.txt";
if (File.Exists(filePath))
{
File.Delete(filePath);
Console.WriteLine("File deleted successfully.");
}
else
{
Console.WriteLine("File does not exist.");
}
}
}
Output
File deleted successfully.
2. Handling Exceptions in C# Files
It’s important to handle exceptions to avoid crashes when working with files. You can use try-catch blocks for this purpose:
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = "example.txt";
try
{
using (StreamReader reader = new StreamReader(filePath))
{
// Reading file content
string line;
Console.WriteLine("Reading from file:");
while ((line = reader.ReadLine()) != null)
{
Console.WriteLine(line);
}
}
}
catch (FileNotFoundException e)
{
Console.WriteLine("Error: File not found. " + e.Message);
}
catch (IOException e)
{
Console.WriteLine("An IO error occurred: " + e.Message);
}
catch (Exception e)
{
Console.WriteLine("An error occurred: " + e.Message);
}
}
}
Output
Error: File not found. Could not find file 'C:\IIS\CSharpCompiler\example.txt'.
3. Advanced File Operations
Copying and Moving Files
You can also copy and move files using the File.Copy()
and File.Move()
methods. Here’s how to do it:
using System;
using System.IO;
class Program
{
static void Main()
{
string sourceFilePath = "source.txt"; // Original file path
string destinationFilePath = "copy.txt"; // Destination for copying
string movedFilePath = "moved.txt"; // Destination for moving
try
{
// Check if the source file exists before copying
if (File.Exists(sourceFilePath))
{
// Copying the file
File.Copy(sourceFilePath, destinationFilePath, true);
Console.WriteLine("File copied successfully.");
// Moving the copied file to a new location
File.Move(destinationFilePath, movedFilePath);
Console.WriteLine("File moved successfully.");
}
else
{
Console.WriteLine("Source file does not exist.");
}
}
catch (Exception ex)
{
// Handle exceptions such as file not found or permission issues
Console.WriteLine($"An error occurred: {ex.Message}");
}
}
}
Output
Source file does not exist.
File to move does not exist.
StreamWriter Class in C#
The StreamWriter class in C# is used to write text or data into files or streams easily. The following methods are included in the class and are often used.
Method | Explanation |
Write() | Writes data (text, characters, arrays) to the stream without adding a new line. |
WriteLine() | Writes data followed by a newline character (\n). |
Flush() | Clears the buffer and writes any buffered data to the underlying stream/file. |
Close() | Closes the StreamWriter and the underlying stream, freeing up resources. |
Dispose() | Releases all resources used by the StreamWriter. Called automatically when using 'using'. |
WriteAsync() | Asynchronously writes data to the stream without blocking the main program flow. |
WriteLineAsync() | Asynchronously, write a line followed by a new line to the stream. |
StreamReader Class in C#
The StreamReader class in C# is used to read text or data from files or streams efficiently. The following methods are included in the class and are often used.
Method | Explanation |
Read() | It returns an integer after reading the subsequent character from the stream. |
ReadLine() | It reads a character line from the stream and outputs the character line as a string. |
ReadToEnd() | It reads every character in the stream from the current point to the end and outputs them as a string. |
Peek() | the following character from the stream is returned without moving the read position. |
Close() | It releases resources by closing the underlying stream and the StreamReader. |
Dispose() | It releases all of the StreamReader's resources. Called automatically when 'using' is employed. |
Seek() | It allows for random access to the stream's contents by setting the location within the stream to the given value. |
Example: StreamReader and StreamWriter Class
This example shows how to write text to a file and then read it back using both classes.
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = "example.txt";
// Writing to a file using StreamWriter
using (StreamWriter writer = new StreamWriter(filePath))
{
writer.WriteLine("Hello, World!");
writer.WriteLine("Welcome to file handling in C#.");
}
// Reading from a file using StreamReader
using (StreamReader reader = new StreamReader(filePath))
{
string line;
while ((line = reader.ReadLine()) != null)
{
Console.WriteLine(line);
}
}
}
}
Output
Hello, World!
Welcome to file handling in C#.
Example: TextReader Class and TextWriter Class
Here's an example of using these concrete implementations of these abstract classes, TextReader and TextWriter, with StringReader and StringWriter.
using System;
using System.IO;
class Program
{
static void Main()
{
// Using TextWriter with StringWriter
using (TextWriter writer = new StringWriter())
{
writer.WriteLine("Hello, World!");
writer.WriteLine("This is an example of TextWriter.");
string output = writer.ToString(); // Get the written content as a string
Console.WriteLine("Written Output:");
Console.WriteLine(output);
}
// Using TextReader with StringReader
using (TextReader reader = new StringReader("Line 1\nLine 2\nLine 3"))
{
string line;
Console.WriteLine("Reading Output:");
while ((line = reader.ReadLine()) != null)
{
Console.WriteLine(line); // Read and print each line
}
}
}
}
Output
Written Output:
Hello, World!
This is an example of TextWriter.
Reading Output:
Line 1
Line 2
Line 3
Example: BinaryReader Class and BinaryWriter Class
Here's an example showing how to write binary data to a file using BinaryWriter and read it back using BinaryReader:
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = "data.bin";
// Writing binary data using BinaryWriter
using (BinaryWriter writer = new BinaryWriter(File.Open(filePath, FileMode.Create)))
{
writer.Write(42); // Write an integer
writer.Write(3.14); // Write a double
writer.Write("Hello, Binary World!"); // Write a string
}
// Reading binary data using BinaryReader
using (BinaryReader reader = new BinaryReader(File.Open(filePath, FileMode.Open)))
{
int intValue = reader.ReadInt32(); // Read the integer
double doubleValue = reader.ReadDouble(); // Read the double
string stringValue = reader.ReadString(); // Read the string
// Display the read values
Console.WriteLine($"Integer: {intValue}");
Console.WriteLine($"Double: {doubleValue}");
Console.WriteLine($"String: {stringValue}");
}
}
}
Output
Integer: 42
Double: 3.14
String: Hello, Binary World!
Example: StringReader Class and StringWriter Class
An example of using both StringReader and StringWriter is provided here:
using System;
using System.IO;
class Program
{
static void Main()
{
// Using StringWriter to write text to a string
using (StringWriter writer = new StringWriter())
{
writer.WriteLine("Hello, World!");
writer.WriteLine("This is an example of StringWriter.");
writer.WriteLine("StringWriter helps build strings easily.");
// Get the written content as a single string
string output = writer.ToString();
Console.WriteLine("Written Output:");
Console.WriteLine(output);
}
// Using StringReader to read from a string
string input = "Line 1\nLine 2\nLine 3\nLine 4";
using (StringReader reader = new StringReader(input))
{
string line;
Console.WriteLine("Reading Output:");
while ((line = reader.ReadLine()) != null)
{
Console.WriteLine(line); // Read and print each line
}
}
}
}
Output
Written Output:
Hello, World!
This is an example of StringWriter.
StringWriter helps build strings easily.
Reading Output:
Line 1
Line 2
Line 3
Line 4
Read More |
OOPs Interview Questions and Answers in C# |
Top 50 C# Interview Questions and Answers To Get Hired |
Conclusion
Fundamentally, file handling in C# is essential for managing data persistence, enabling applications to read from and write to files efficiently. By leveraging classes from the System.IO namespace, developers can handle both text and binary files with ease. If you are eager to learn more about the C# concept and development, Scholarhat provides you with complete guidance on Advanced Full-Stack .NET Developer Certification Training and the .NET Solution Architect Certification Training program.
FAQs
Q1. What are common exceptions in file handling?
- FileNotFoundException: File doesn’t exist.
- UnauthorizedAccessException: No permission to access the file.
- IOException: General I/O errors.
Q2. What is the purpose of the using statement in file handling?
Q3. How do I rename a file in C#?
Q4. What is the difference between text files and binary files in C#?
- Text files: Store data in a human-readable format (e.g., .txt, .csv).
- Binary files: Store data in a machine-readable format (e.g., .exe, .bin), which is more compact and efficient for certain types of data.
Q5. What happens if I try to read from or write to a file that doesn’t exist?
Take our Csharp skill challenge to evaluate yourself!
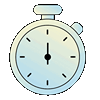
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.