24
SepInheritance in C#
Inheritance in C#- An Overview
Inheritance in C# is a fundamental object-oriented programming concept that allows classes to inherit properties and behaviors from other classes. This powerful mechanism enables code reuse, promotes modularity, and simplifies complex software designs. Explore how inheritance works in C# and its vital role in building efficient and scalable applications.
What is Inheritance in C# ?
Inheritance in C# is a fundamental feature of object-oriented programming, allowing a class (derived or child class) to inherit properties and behaviors from another class (base or parent class). It promotes code reusability, enables the creation of hierarchical relationships between classes, and supports the concept of polymorphism, enhancing the organization and efficiency of C# programs.
Advantages of Inheritance in C#
- Code Reusability: Inheritance allows you to create a new class by reusing properties and methods of an existing class.
- Extensibility: You can create new classes based on existing ones, extending functionality without modifying the original class.
- Polymorphism: Inheritance enables polymorphism, allowing objects of derived classes to be treated as objects of the base class.
- Organized Code: Inheritance helps in organizing classes into a hierarchy, making it easier to manage and understand complex systems.
- Time Efficiency: Reusing existing code through inheritance saves development time and effort.
- Maintenance: Changes in the base class automatically reflect in derived classes, ensuring consistency.
Disadvantages of Inheritance in C#
- Tight Coupling: Inheritance can create tight coupling between classes, making it harder to modify base classes without affecting derived classes.
- Limited Reusability: Subclasses are bound to the implementation details of their base class, limiting their reusability in different contexts.
- Complexity: Inheritance hierarchies can become complex and difficult to understand, leading to maintenance challenges.
- Fragile Base Class Problem: Modifying the base class can inadvertently affect the derived classes, causing unexpected behavior.
- Difficulty in Testing: Testing-derived classes can be challenging due to the inherited behavior from the base class, making it harder to isolate issues.
Types of Inheritance in C#
In C#, inheritance is a fundamental object-oriented programming concept. There are several types of inheritance in C#:
- single inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
- Multiple inheritance
- Hybrid inheritance
1. Single inheritance in C#
Single inheritance in C# refers to the capability of a class to inherit properties and behavior from only one base class. It means a derived class can have only one parent class, ensuring a simple and clear class hierarchy, enhancing code readability, and maintaining a manageable object-oriented structure.
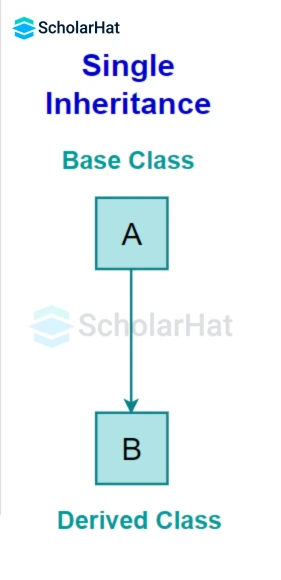
Example
using System;
class Animal
{
public void Eat()
{
Console.WriteLine("The animal is eating.");
}
}
class Dog : Animal
{
public void Bark()
{
Console.WriteLine("The dog is barking.");
}
}
class Program
{
static void Main(string[] args)
{
Dog myDog = new Dog();
myDog.Eat(); // Inherited from Animal class
myDog.Bark(); // Defined in Dog class
Console.ReadKey(); // Keep the console window open
}
}
Explanation
In this example, the Dog class inherits from the Animal class, demonstrating single inheritance. The Dog class inherits the Eat method from the Animal class and adds its own method Bark.
Output
The animal is eating.
The dog is barking.
2. Multilevel Inheritance in C#
Multilevel Inheritance in C# refers to a scenario where a class derives from another class, and then another class is derived from this derived class. It creates a hierarchical chain of inheritance, allowing properties and methods to be passed down through multiple levels of classes, forming a parent-child-grandchild relationship.
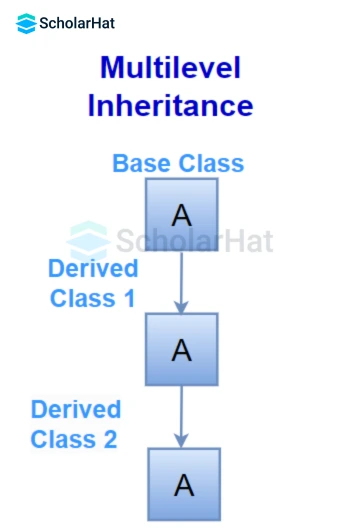
Example
using System;
class Animal
{
public void Eat()
{
Console.WriteLine("Animal is eating.");
}
}
class Mammal : Animal
{
public void Drink()
{
Console.WriteLine("Mammal is drinking.");
}
}
class Dog : Mammal
{
public void Bark()
{
Console.WriteLine("Dog is barking.");
}
}
class Program
{
static void Main(string[] args)
{
Dog myDog = new Dog();
myDog.Eat();
myDog.Drink();
myDog.Bark();
}
}
Explanation
In this example, the Dog class inherits from the Mammal class, which in turn inherits from the Animal class, demonstrating multilevel inheritance. When you run this code, it will produce the specified output.
Output
Animal is eating.
Mammal is drinking.
Dog is barking.
3. Hierarchical Inheritance in C#
In C#, Hierarchical Inheritance refers to a scenario where multiple derived classes inherit from a single base class. Each derived class shares common properties and behaviors defined in the base class, forming a hierarchy. This allows for code reusability and structuring related classes in a logical manner.
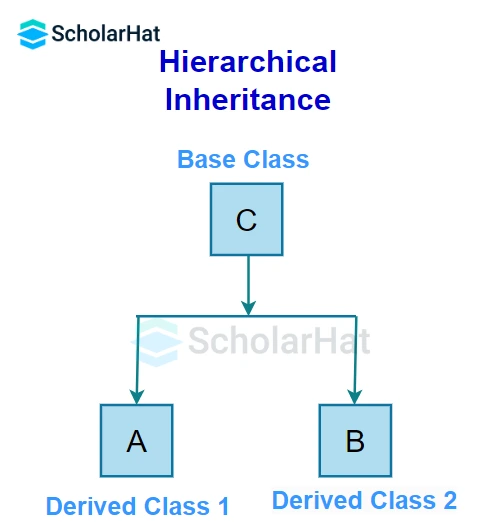
Example
using System;
class Animal
{
public void Eat()
{
Console.WriteLine("Animal is eating.");
}
}
class Dog : Animal
{
public void Bark()
{
Console.WriteLine("Dog is barking.");
}
}
class Cat : Animal
{
public void Meow()
{
Console.WriteLine("Cat is meowing.");
}
}
class Program
{
static void Main(string[] args)
{
Dog myDog = new Dog();
myDog.Eat();
myDog.Bark();
Cat myCat = new Cat();
myCat.Eat();
myCat.Meow();
}
}
Explanation
In this example, Dog and Cat classes inherit from the Animal class, demonstrating hierarchical inheritance. The Eat method is inherited by both Dog and Cat classes, while they each have their specific methods (Bark and Meow respectively).
Output
Animal is eating.
Dog is barking.
Animal is eating.
Cat is meowing.
4. Multiple inheritance in C#
Multiple inheritance in C# allows a class to inherit properties and behavior from more than one base class. Unlike some other programming languages, C# does not support multiple inheritance directly for classes. However, it can achieve similar functionality through interfaces, where a class can implement multiple interfaces to inherit behavior from different sources.
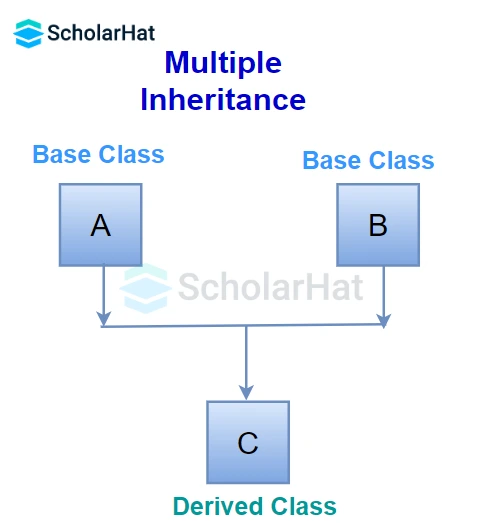
Example
using System;
interface IShape
{
void Draw();
}
interface IColor
{
void Color();
}
class Circle : IShape, IColor
{
public void Draw()
{
Console.WriteLine("Drawing a circle");
}
public void Color()
{
Console.WriteLine("Applying color to the circle");
}
}
class Program
{
static void Main()
{
Circle circle = new Circle();
circle.Draw();
circle.Color();
}
}
Explanation
In this example, Circle class implements both IShape and IColor interfaces, achieving the effect of multiple inheritance.
Output
Drawing a circle
Applying color to the circle
5. Hybrid inheritance in C#
Hybrid inheritance in C# refers to a combination of multiple and multilevel inheritance. It allows a class to inherit properties and behaviors from multiple base classes (multiple inheritance) and create a hierarchy of derived classes (multilevel inheritance), providing a flexible way to structure classes and promote code reusability.
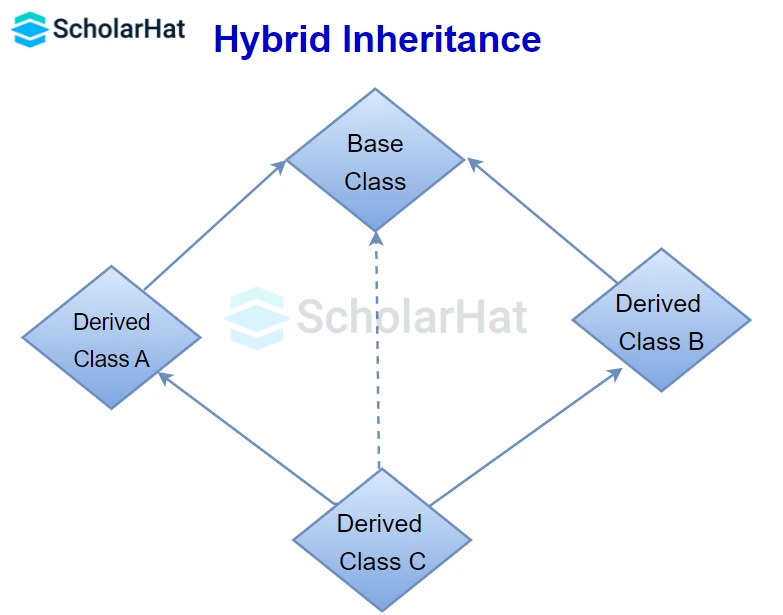
Example
using System;
interface IShape
{
void Draw();
}
class Shape
{
public void Display()
{
Console.WriteLine("Shape is displayed.");
}
}
class Circle : Shape, IShape
{
public void Draw()
{
Console.WriteLine("Circle is drawn.");
}
}
class Program
{
static void Main(string[] args)
{
Circle circle = new Circle();
circle.Display(); // Output: Shape is displayed.
circle.Draw(); // Output: Circle is drawn.
}
}
Explanation
In this example, Shape is a base class, IShape is an interface, and Circle class inherits from both the Shape class and implements the IShape interface, demonstrating hybrid inheritance.
Output
Shape is displayed.
Circle is drawn.
Important facts about inheritance in C#
- Inheritance allows a class (derived/child) to inherit properties and methods from another class (base/parent).
- Promotes code reusability and modularity.
- Supports single inheritance (one class can inherit from one parent) and multiple interfaces.
- Enables polymorphism, allowing objects of derived classes to be treated as objects of the base class.
- Base class members can be accessed using the base keyword in the derived class.
Conclusion
In conclusion, understanding inheritance in C# is essential for building efficient, modular, and maintainable object-oriented programs. It promotes code reusability, enables polymorphism, and enhances the overall design structure. Mastering inheritance empowers developers to create robust applications by leveraging the power of class hierarchy and abstraction.
Take our Csharp skill challenge to evaluate yourself!
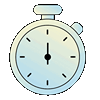
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.