26
OctLINQ in C#: Types of LINQ in C# with Example
LINQ in C#
LINQ(Language Integrated Query) in C# is a beneficial concept that every developer should understand. It is a feature in C# that lets programmers query data collections in a clear and understandable way. A syntax built right into the C# language lets you manipulate complicated data from within your code.
In this C# tutorial, we will get to know about what is LINQ, including why do we use LINQ,types of LINQ in C#, syntax of LINQ, LINQ in C# example, architecture of LINQ, advantages of LINQ, and many more. So, just have a cup of tea and read this article carefully until it is not completed because it will vanish all your confusion related to this article.
What is LINQ?
LINQ (Language Integrated Query) in C# makes working with data easier by helping you to use simple and understandable code to filter, sort, and interact with collections like lists and databases. It offers a uniform approach to managing various data sources using the same techniques. Data queries are made shorter, simpler to build, and more manageable with LINQ.
Architecture of LINQ in C#
Here is the architecture of LINQ in C#:
Types of LINQ
There are basically five types of LINQ that we will learn below:
- LINQ to Objects
- LINQ to SQL
- LINQ to Entities
- LINQ to XML
- LINQ to DataSet
1. LINQ to Objects
- LINQ to Objects makes it simple to query in-memory collections like arrays, lists, and dictionaries using LINQ syntax.
- it is beneficial for you to arrange, filter, and work with data that is already kept in the memory of the software.
2. LINQ to SQL
- Writing queries in LINQ is made possible by LINQ to SQL, which maps database tables to C# objects for SQL Server database querying.
- It streamlines database access by converting LINQ queries into SQL commands that are run on the database.
3. LINQ to Entities
- By representing tables as C# objects and classes, LINQ to Entities interfaces with the Entity Framework to query databases.
- It enables greater object-oriented database interaction by enabling you to construct LINQ queries.
4. LINQ to XML
- LINQ to XML enables the use of LINQ's querying syntax for querying and manipulating XML data.
- It treats XML documents as collections or objects, simplifying the process of working with XML data.
5. LINQ to Dataset
- LINQ to DataSet allows the developer to run LINQ queries on DataSet and DataTable objects, which are used to collect data that has been fetched from a database.
- With LINQ's assistance, you may efficiently manage and retrieve relational data stored in memory in a detached context.
Basic Syntax
There are two ways to write the syntax LINQ in C#:
1. Query Syntax: This syntax resembles SQL and is often easier for those familiar with SQL.
var results = from student in students
where student.Age > 18
select student;
2. Method Syntax: This syntax uses method calls and lambda expressions. It is more flexible and can be more powerful in some cases.
var results = students.Where(student => student.Age > 18);
Example 1: LINQ to Objects
Let's consider a simple example of using LINQ to query a list of students.
using System;
using System.Collections.Generic;
using System.Linq;
public class Student
{
public string Name { get; set; }
public int Age { get; set; }
}
public class Program
{
public static void Main()
{
List<Student> students = new List<Student> {
new Student { Name = "Amit", Age = 17 },
new Student { Name = "Priya", Age = 19 },
new Student { Name = "Raj", Age = 20 },
new Student { Name = "Neha", Age = 18 }
};
// Query Syntax
var adultStudentsQuery = from student in students
where student.Age >= 18
select student;
Console.WriteLine("Adult Students (Query Syntax):");
foreach (var student in adultStudentsQuery)
{
Console.WriteLine(student.Name);
}
// Method Syntax
var adultStudentsMethod = students.Where(student => student.Age >= 18);
Console.WriteLine("\nAdult Students (Method Syntax):");
foreach (var student in adultStudentsMethod)
{
Console.WriteLine(student.Name);
}
}
}
Output
Adult Students (Query Syntax):
Priya
Raj
Neha
Adult Students (Method Syntax):
Priya
Raj
Neha
Example 2: Selecting Specific Properties
You can project specific properties of an object using LINQ.
using System;
using System.Collections.Generic;
using System.Linq;
class Student
{
public int Id { get; set; }
public string Name { get; set; }
public int Age { get; set; }
}
class Program
{
static void Main()
{
// Sample data: List of students
List<Student> students = new List<Student>
{
new Student { Id = 1, Name = "Amit", Age = 20 },
new Student { Id = 2, Name = "Riya", Age = 22 },
new Student { Id = 3, Name = "Raj", Age = 21 }
};
// Selecting specific properties (Name and Age) using LINQ
var selectedProperties = students.Select(s => new { s.Name, s.Age });
// Displaying the selected properties
foreach (var student in selectedProperties)
{
Console.WriteLine($"Name: {student.Name}, Age: {student.Age}");
}
}
}
Output
Name: Amit, Age: 20
Name: Riya, Age: 22
Name: Raj, Age: 21
Example 3: Ordering Results
LINQ can easily sort your data. Here’s how to sort students by age.
using System;
using System.Collections.Generic;
using System.Linq;
class Student
{
public int Id { get; set; }
public string Name { get; set; }
public int Age { get; set; }
}
class Program
{
static void Main()
{
// Sample data: List of students
List<Student> students = new List<Student>
{
new Student { Id = 1, Name = "Amit", Age = 20 },
new Student { Id = 2, Name = "Riya", Age = 22 },
new Student { Id = 3, Name = "Raj", Age = 21 },
new Student { Id = 4, Name = "Neha", Age = 19 }
};
// Ordering students by Age in ascending order
var orderedStudents = students.OrderBy(s => s.Age);
Console.WriteLine("Students ordered by Age (ascending):");
foreach (var student in orderedStudents)
{
Console.WriteLine($"Name: {student.Name}, Age: {student.Age}");
}
// Ordering students by Name in descending order
var orderedByNameDesc = students.OrderByDescending(s => s.Name);
Console.WriteLine("\nStudents ordered by Name (descending):");
foreach (var student in orderedByNameDesc)
{
Console.WriteLine($"Name: {student.Name}, Age: {student.Age}");
}
}
}
Output
Students ordered by Age (ascending):
Name: Neha, Age: 19
Name: Amit, Age: 20
Name: Raj, Age: 21
Name: Riya, Age: 22
Students ordered by Name (descending):
Name: Riya, Age: 22
Name: Raj, Age: 21
Name: Neha, Age: 19
Name: Amit, Age: 20
Example 4: Filtering with Multiple Conditions
You can apply multiple conditions in your queries.
using System;
using System.Collections.Generic;
using System.Linq;
class Student
{
public int Id { get; set; }
public string Name { get; set; }
public int Age { get; set; }
public string Course { get; set; }
}
class Program
{
static void Main()
{
// Sample data: List of students
List<Student> students = new List<Student>
{
new Student { Id = 1, Name = "Amit", Age = 20, Course = "Mathematics" },
new Student { Id = 2, Name = "Riya", Age = 22, Course = "Physics" },
new Student { Id = 3, Name = "Raj", Age = 21, Course = "Mathematics" },
new Student { Id = 4, Name = "Neha", Age = 19, Course = "Biology" },
new Student { Id = 5, Name = "Sita", Age = 23, Course = "Physics" }
};
// Filtering students who are older than 20 and enrolled in Mathematics
var filteredStudents = students.Where(s => s.Age > 20 && s.Course == "Mathematics");
Console.WriteLine("Students older than 20 and enrolled in Mathematics:");
foreach (var student in filteredStudents)
{
Console.WriteLine($"Name: {student.Name}, Age: {student.Age}, Course: {student.Course}");
}
}
}
Output
Students older than 20 and enrolled in Mathematics:
Name: Raj, Age: 21, Course: Mathematics
Example 5: Grouping Data
You can group data using the GroupBy
method. Here’s an example that groups students by age.
using System;
using System.Collections.Generic;
using System.Linq;
class Student
{
public int Id { get; set; }
public string Name { get; set; }
public int Age { get; set; }
public string Course { get; set; }
}
class Program
{
static void Main()
{
// Sample data: List of students
List<Student> students = new List<Student>
{
new Student { Id = 1, Name = "Amit", Age = 20, Course = "Mathematics" },
new Student { Id = 2, Name = "Riya", Age = 22, Course = "Physics" },
new Student { Id = 3, Name = "Raj", Age = 21, Course = "Mathematics" },
new Student { Id = 4, Name = "Neha", Age = 19, Course = "Biology" },
new Student { Id = 5, Name = "Sita", Age = 23, Course = "Physics" }
};
// Grouping students by Course
var groupedStudents = students.GroupBy(s => s.Course);
// Displaying grouped results
foreach (var group in groupedStudents)
{
Console.WriteLine($"Course: {group.Key}");
foreach (var student in group)
{
Console.WriteLine($" Name: {student.Name}, Age: {student.Age}");
}
}
}
}
Output
Course: Mathematics
Name: Amit, Age: 20
Name: Raj, Age: 21
Course: Physics
Name: Riya, Age: 22
Name: Sita, Age: 23
Course: Biology
Name: Neha, Age: 19
Example 6: Joining Two Collections
LINQ allows you to join two collections. For example, let’s join students with their corresponding grades.
using System;
using System.Collections.Generic;
using System.Linq;
class Student
{
public int Id { get; set; }
public string Name { get; set; }
public int CourseId { get; set; }
}
class Course
{
public int Id { get; set; }
public string CourseName { get; set; }
}
class Program
{
static void Main()
{
// Sample data: List of students
List<Student> students = new List<Student>
{
new Student { Id = 1, Name = "Amit", CourseId = 1 },
new Student { Id = 2, Name = "Riya", CourseId = 2 },
new Student { Id = 3, Name = "Raj", CourseId = 1 },
new Student { Id = 4, Name = "Neha", CourseId = 3 },
new Student { Id = 5, Name = "Sita", CourseId = 2 }
};
// Sample data: List of courses
List<Course> courses = new List<Course>
{
new Course { Id = 1, CourseName = "Mathematics" },
new Course { Id = 2, CourseName = "Physics" },
new Course { Id = 3, CourseName = "Biology" }
};
// Joining students and courses on CourseId
var joinedData = from student in students
join course in courses on student.CourseId equals course.Id
select new
{
StudentName = student.Name,
CourseName = course.CourseName
};
// Displaying the joined results
Console.WriteLine("Students and their Courses:");
foreach (var item in joinedData)
{
Console.WriteLine($"Student: {item.StudentName}, Course: {item.CourseName}");
}
}
}
Output
Students and their Courses:
Student: Amit, Course: Mathematics
Student: Riya, Course: Physics
Student: Raj, Course: Mathematics
Student: Neha, Course: Biology
Student: Sita, Course: Physics
Example 7: Checking Existence with Any and All
You can check if any or all elements in a collection meet a certain condition.
using System;
using System.Collections.Generic;
using System.Linq;
class Student
{
public int Id { get; set; }
public string Name { get; set; }
public int Age { get; set; }
public string Course { get; set; }
}
class Program
{
static void Main()
{
// Sample data: List of students
List<Student> students = new List<Student>
{
new Student { Id = 1, Name = "Amit", Age = 20, Course = "Mathematics" },
new Student { Id = 2, Name = "Riya", Age = 22, Course = "Physics" },
new Student { Id = 3, Name = "Raj", Age = 21, Course = "Mathematics" },
new Student { Id = 4, Name = "Neha", Age = 19, Course = "Biology" },
new Student { Id = 5, Name = "Sita", Age = 23, Course = "Physics" }
};
// Check if any student is enrolled in "Mathematics"
bool hasMathematicsStudent = students.Any(s => s.Course == "Mathematics");
Console.WriteLine($"Is there any student enrolled in Mathematics? {hasMathematicsStudent}");
// Check if all students are older than 18
bool allStudentsOlderThan18 = students.All(s => s.Age > 18);
Console.WriteLine($"Are all students older than 18? {allStudentsOlderThan18}");
}
}
Output
Is there any student enrolled in Mathematics? True
Are all students older than 18? False
Advantages of LINQ in C#
Several advantages provided by LINQ in C# are explained below. You can check this out:
- By enabling you to construct shorter, easier-to-read queries, LINQ streamlines your code by eliminating the need for intricate loops.
- It offers a standardized method for utilizing the same syntax to query many data sources, including lists, databases, and XML.
- Because LINQ is tightly typed, it verifies that your queries are valid before executing the program by looking for mistakes during compilation.
- LINQ queries' readability enhances code maintainability by making it simpler to update or alter the code as needed.
- LINQ contributes to the reduction of runtime errors by identifying mistakes during compilation, resulting in more dependable programs.
Conclusion
To sum up, Working with data is significantly simpler and more effective in C# thanks to LINQ. LINQ in C#, Types of LINQ in C#, and LINQ in C# example enable you to use clear and understandable queries to carry out operations like sorting, filtering, and choosing data. If you are eager to learn more about the C# concept and development, Scholarhat provides you with complete guidance on Advanced Full-Stack .NET Developer Certification Training and the .NET Solution Architect Certification Training program.
FAQs
Q1. What is the difference between IEnumerable<T> and IQueryable<T>?
- IEnumerable<T>: Represents a collection of objects that can be enumerated. It is used for in-memory collections.
- IQueryable<T>: Represents a collection that can be queried against a specific data source. It is typically used for querying databases and can build expression trees for translation to SQL.
Q2. Can LINQ be used with different data sources?
Q3. What is deferred execution in LINQ?
Q4. What is the purpose of the Select method in LINQ?
Q5. Can I modify the data in a collection using LINQ?
Take our Csharp skill challenge to evaluate yourself!
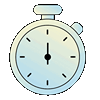
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.