18
AprUnderstanding Multiple Inheritance in C#
C# Multiple Inheritance
C# Multiple Inheritance may appear complicated at first, but it is actually much simpler than that! In C#, a class can only inherit from one base class, reducing complications such as the "diamond problem." However, interfaces can still provide equivalent capabilities. For example, if you want a FlyingFish class that can fly and swim, you can provide IFlyable and ISwimmable interfaces. This allows FlyingFish to implement both interfaces and get the ability to fly and swim without the complications of traditional multiple inheritance. If you're curious about how multiple inheritance in C# works, I can show you some code samples!
So in this C# Tutorial, we will see what multiple inheritance is. including the diamond problem and how it is solved. To gain a deeper understanding of these concepts and improve your C# skills, be sure to enroll in our Free C Sharp Course for a comprehensive learning experience.
Read More: Before Understanding multiple inheritance in c# you need to know about the following concepts. |
What is Multiple Inheritance in C#?
In simple terms, multiple inheritance in C# is when a class inherits from more than one parent class.
Let' discuss with example, consider the following scenario:
- Class A has some methods and properties.
- Class B also has some methods and properties.
- A new class C inherits from both A and B, gaining access to the methods and properties of both classes.
This can be useful when you want a class to have combined functionalities from multiple sources. However, it can also lead to complications, especially with something called the diamond problem.
The Diamond Problem
The diamond problem occurs when two or more parent classes have methods with the same name, and a derived class inherits from these parent classes. The ambiguity arises because it’s unclear which version of the method the derived class should inherit. Here’s an example:
- Class A and Class B both define a method called Print().
- Class C inherits from both Class A and Class B.
In this case, if ClassCcallsthe Print() method, it’s unclear whether it should execute the method from Class A or Class B. This can lead to bugs and unpredictable behavior.
C# Solution: Using Interfaces for Multiple Inheritance
In C#, a class can only inherit from one base class, but it can implement multiple interfaces. Interfaces are essentially contracts that define a set of methods and properties that the class must implement.
By implementing multiple interfaces, you can achieve the benefits of multiple inheritance without the complexity of inheriting from multiple classes.
Example: Using Interfaces
Let’s say we want to create a SmartDevice the class that inherits characteristics from both a Phone and a Camera. While C# doesn’t allow a class to inherit from both Phone and Camera We can define interfaces to achieve this functionality directly.
using System;
interface IPhone
{
void MakeCall(string number);
}
interface ICamera
{
void TakePhoto();
}
class SmartDevice : IPhone, ICamera
{
public void MakeCall(string number)
{
Console.WriteLine($"Calling {number}...");
}
public void TakePhoto()
{
Console.WriteLine("Taking a photo...");
}
}
class Program
{
static void Main(string[] args)
{
SmartDevice myDevice = new SmartDevice();
// Using IPhone interface method
myDevice.MakeCall("123-456-7890");
// Using ICamera interface method
myDevice.TakePhoto();
}
}
Output
Calling 123-456-7890...
Taking a photo...
Why C# Does Not Support Multiple Inheritance Directly
C# deliberately avoids supporting multiple inheritance for a few reasons:
- Complexity and Ambiguity: Multiple inheritance can create confusion, especially when different base classes have methods with the same signature, leading to ambiguity over which method should be inherited.
- The Diamond Problem: As mentioned earlier, when two base classes have the same method, the derived class might inherit both versions, causing potential errors and making debugging more difficult.
- Design Consideration: The designers of C# wanted to keep the language simple and avoid potential pitfalls that other languages with multiple inheritance (like C++) might face. By using interfaces, C# achieves a cleaner, more predictable way of implementing multiple behaviors.
Abstract Classes vs. Interfaces
While C# doesn’t allow multiple inheritance, it does allow a combination of single inheritance from an abstract class and multiple interface implementations.
- Abstract Class in C#: You can only inherit from one abstract class. Abstract classes can have method implementations as well as abstract methods (methods without implementation).
- Interface: You can implement multiple interfaces in C#. Interfaces only define method signatures, without any implementation.
If you want to provide some common functionality (with actual code) while still enabling multiple behaviors through interfaces, you can use an abstract class and interfaces together.
Example:
abstract class Device
{
public abstract void PowerOn();
}
interface IPhone
{
void MakeCall(string number);
}
interface ICamera
{
void TakePhoto();
}
class SmartDevice : Device, IPhone, ICamera
{
public override void PowerOn()
{
Console.WriteLine("Device is powering on...");
}
public void MakeCall(string number)
{
Console.WriteLine($"Calling {number}...");
}
public void TakePhoto()
{
Console.WriteLine("Taking a photo...");
}
}
class Program
{
static void Main(string[] args)
{
SmartDevice myDevice = new SmartDevice();
myDevice.PowerOn();
myDevice.MakeCall("123-456-7890");
myDevice.TakePhoto();
}
}
Read More: If you are preparing for the C# exam or interview "Top 50 C# Interview Questions and Answers "Might help you.
Conclusion
While C# doesn’t support multiple inheritance directly due to its potential complexity and ambiguity, it provides a clean and effective alternative using interfaces. By implementing multiple interfaces, you can achieve the functionality of multiple inheritance without the downsides. Additionally, combining abstract classes with interfaces allows you to create flexible and well-structured object hierarchies, enabling the development of robust and maintainable code.
C#'s approach ensures that developers can avoid common issues like the diamond problem while still benefiting from the advantages of polymorphism and code reuse. You want to get an idea of other C# concepts like this just enroll in our .NET Certification training.
FAQs
Take our Csharp skill challenge to evaluate yourself!
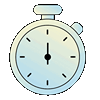
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.