21
NovC# Properties
C# Propeties- Overview
Welcome to our article on properties in C#! Properties provide a way to encapsulate fields in C# classes, controlling their access and ensuring data integrity. In this article, we'll explore the fundamentals of properties, their types, and how they enhance the security and usability of your C# programs.
What is properties in C#?
In C#, properties are class members that encapsulate private fields, providing a way to read or write their values. They allow controlled access to an object's state by defining get and set accessors, enabling data abstraction and encapsulation while maintaining the flexibility of accessing class variables.
Example
// C# program to illustrate the problems
// with public and private members
using System;
// public class
public class C1
{
// public data members
public int rn;
public string name;
// private field
// private int marks = 35;
}
// another public class
public class C2
{
// Main Method
public static void Main(string[] args)
{
// Creating object of C1 class
C1 obj = new C1();
// setting values to public
// data members of class C1
obj.rn = 10000;
obj.name = null;
// setting values to private
// data members of class C1
// obj.mark = 0;
// display result
Console.WriteLine("Name: {0} \nRoll No: {1}", obj.name, obj.rn);
}
}
Explanation
In the given code, the name variable is not initialized, so its default value is null. The rn variable is set to 10000. The private field marks is commented out and not used, so it does not affect the output.
Output
Name:
Roll No: 10000
Using Properties in C#
- Encapsulation: Properties enable data encapsulation by providing a controlled way to access class fields.
- Get and Set Methods: Properties have to get and set methods, allowing read and write access to private fields while maintaining data integrity.
- Syntax: Declare properties using get and set accessors, defining the logic for retrieving and setting values.
- Access Modifiers: Properties can have access modifiers like public, private, or protected, controlling their visibility.
- Data Validation: Properties allow validation logic, ensuring only valid data is stored in class fields.
- Simplified Syntax: Properties simplify the syntax of accessing class members, enhancing code readability and maintainability.
What is Accessor in C#?
In C#, an accessor refers to a method or code block that is used to read or write the value of a private field in a class. Accessors are commonly used in properties to control the access to the underlying data, allowing encapsulation and ensuring data integrity in object-oriented programming. There are two types of accessors in C# those are,
- Get Accessor
- Set Accessor
Syntax
<access_modifier> <return_type> <property_name>
{
get { // body }
set { // body }
}
Get Accessor in C#
In C#, a get accessor retrieves the value of a private field in a class. It is part of a property declaration and returns the property value. Get accessors to allow controlled access to class properties, enabling encapsulation and data abstraction in object-oriented programming.
Example
class Scholar {
// Declare roll_no field
private int roll_no;
// Declare roll_no property
public int Roll_no
{
get
{
return roll_no;
}
}
}
Explanation
The given code defines a class Scholar with a private field roll_no and a public property Roll_no that allows access to the private field. Since the Roll_no property only has a get accessor, you can retrieve the value of the roll_no field but cannot set it from outside the class. There is no output in the code as it does not contain any logic to demonstrate the property usage.
Set Accessor in C#
In C#, a set accessor is a method used within a property to assign a value to that property. It allows you to control the behavior when a property's value is set. You define it using the "set" keyword followed by a code block that specifies how the value should be stored or processed.
Example
class Scholar {
// Declare roll_no field
private int roll_no;
// Declare roll_no property
public int Roll_no
{
get
{
return roll_no;
}
set
{
roll_no = value;
}
}
}
Explanation
This C# code defines a class Scholar with a private field roll_no and a public property Roll_no. The property has both get and set accessors, allowing external code to read and modify the private roll_no field. This encapsulation ensures controlled access to the roll_no data within the class.
Accessor Accessibility in C#
- Accessor modifiers are not permitted on an interface or an explicit interface member implementation.
- Only when a property possesses both set and get accessors can we employ accessor modifiers.
- The accessor modifier for a property that is an override modifier has to match the overriding accessor's accessor.
- The accessor modifier for a property that is an override modifier has to match the overriding accessor's accessor.
Static Properties in C#
In C#, static properties are associated with the class itself rather than specific instances. They are declared using the static keyword and allow access to data or behavior without creating an object of the class. Static properties are accessed using the class name, not through instances, providing shared data across all instances of the class.
Example
using System;
class MyClass
{
private static int _x;
public static int X
{
get
{
return _x;
}
set
{
_x = value;
}
}
}
class MyClient
{
public static void Main()
{
MyClass.X = 30;
int xVal = MyClass.X;
Console.WriteLine(xVal);
}
}
Explanation
MyClass.X = 30; sets the static property X in the MyClass class to the value 30.
int xVal = MyClass.X; retrieves the value of the static property X (which is 30) and assigns it to the variable xVal.
Console.WriteLine(xVal); prints the value of xVal (which is 30) to the console.
Output
30
Example of Properties and Inheritance in C#
using System;
class Base
{
public virtual int X
{
get
{
Console.Write("Base GET");
return 40;
}
set
{
Console.Write("Base SET");
}
}
}
class Derived : Base
{
}
class MyClient
{
public static void Main()
{
Derived d1 = new Derived();
d1.X = 40;
Console.WriteLine(d1.X);
}
}
Explanation
d1.X = 40; calls the set accessor of the X property from the Base class, printing "Base SET" to the console.
Console.WriteLine(d1.X); calls the get accessor of the X property from the Base class, printing "Base GET" to the console, and returns the value 40, which is then printed to the console.
Output
Base SET
Base GET
40
Example of Properties and Polymorphism in C#
using System;
class Base
{
public virtual int X
{
get
{
Console.Write("Base GET");
return 70;
}
set
{
Console.Write("Base SET");
}
}
}
class Derived : Base
{
public override int X
{
get
{
Console.Write("Derived GET");
return 70;
}
set
{
Console.Write("Derived SET");
}
}
}
class MyClient
{
public static void Main()
{
Base b1 = new Derived();
b1.X = 70;
Console.WriteLine(b1.X);
}
}
Explanation
Base b1 = new Derived(); creates a new object of the Derived class and assigns it to a variable of type Base.
b1.X = 70; calls the set accessor of the X property from the Derived class, printing "Derived SET" to the console.
Console.WriteLine(b1.X); calls the get accessor of the X property from the Derived class, printing "Derived GET" to the console, and returns the value 70, which is then printed to the console. The behavior is determined by the overridden property in the Derived class, even though the variable is declared as Base.
Output
Derived SET
Derived GET
70
Conclusion
In conclusion, understanding properties in C# is crucial for encapsulation and data abstraction. They provide controlled access to class fields, enhancing security and maintainability. Properties simplify the syntax for accessing class members, making code more readable and efficient. Mastering properties empowers developers to create robust, well-organized C# applications.
Take our Csharp skill challenge to evaluate yourself!
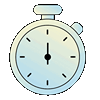
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.