21
NovReverse a String in C# Using Array, For Loop & Reverse Function
Reverse String in C#
Reverse String in C# is a basic programming concept that involves reversing the order of the characters in a given string. This method can be helpful in a number of situations, including processing user input in some programs, modifying text for specialized formatting, and verifying palindromes. Understanding how to reverse a string efficiently also enhances a developer's ability to work with arrays, loops, and built-in methods.
In the C# tutorial, we will learn what is reverse string in C#?, how to reverse strings in C#, common use cases and examples, key features and benefits and many more.
What is the Reverse String in C#?
Reverse a String in C# is a method defined in the C# programming language where the developer can rearrange the position of a given string. It serves as a fundamental exercise in understanding string manipulation, which is a core aspect of programming. By reversing a string, developers can solve problems related to palindromes (strings that read the same forwards and backwards), data encryption, and algorithm challenges.
- Strings in C# are immutable; reversing requires conversion to a mutable structure like a char[] array.
- Use Array.Reverse() or LINQ's Reverse() method for reversing strings.
- Consider the efficiency of the reversal method, especially with large strings.
- Useful in palindrome checking, data processing, and certain algorithm challenges.
- Enhances understanding of string handling, arrays, and loops in C#.
Read More: |
Top 20 C# Features Every .NET Developer Must Know in 2024 |
How do you reverse a string in C#?
There are several ways in C# to go reverse with a string. Here are some typical methods:
1. Using a char[] Array and Array.Reverse()
Using this approach, the string is first converted to a character array, which is then reversed, and finally, the array is converted back to a string.
Example
using System;
class Program
{
static void Main()
{
string original = "hello ScholarHat";
// Convert the string to a char array
char[] charArray = original.ToCharArray();
// Reverse the char array
Array.Reverse(charArray);
// Convert the reversed char array back to a string
string reversed = new string(charArray);
Console.WriteLine(reversed);
}
}
Output
taHralohcS olleh
2. Using LINQ's Reverse() Method
A string can get reversed by converting it to a character array, reversing it, and then constructing a new string from the reversed array using LINQ's Reverse() function.
Example
using System;
using System.Linq;
class Program
{
static void Main()
{
string original = "Welcome to ScholarHat";
// Reverse using LINQ
string reversed = new string(original.Reverse().ToArray());
Console.WriteLine(reversed);
}
}
Output
taHralohcS ot emocleW
3. Using a for Loop
You can also reverse a string by manually iterating over it from the end to the beginning and building a new string by using the for loop.
Example
using System;
class Program
{
static void Main()
{
string original = "Welcome to ScholarHat";
string reversed = "";
// Loop through the string in reverse order
for (int i = original.Length - 1; i >= 0; i--)
{
reversed += original[i];
}
Console.WriteLine(reversed);
}
}
Example
taHralohcS ot emocleW
4. Using a StringBuilder
Using a StringBuilder, you can efficiently reverse a string by appending characters in reverse order within a loop, which is faster and more memory-efficient than repeatedly concatenating strings.
Example
using System;
using System.Text;
class Program
{
static void Main()
{
string original = "CSharp";
StringBuilder reversed = new StringBuilder();
// Append characters in reverse order
for (int i = original.Length - 1; i >= 0; i--)
{
reversed.Append(original[i]);
}
Console.WriteLine(reversed.ToString());
}
}
Output
prahSC
5. Using a while loop
Using a while loop, we can reverse a string by iterating through it from the last character to the first, appending each character to a new string.
Example
using System;
class Program
{
static void Main()
{
string original = "reverse";
string reversed = "";
int index = original.Length - 1;
// Use a while loop to iterate from the end to the beginning of the string
while (index >= 0)
{
reversed += original[index];
index--;
}
Console.WriteLine(reversed);
}
}
Example
esrever
How do you choose the correct method to reverse a string in C#?
The following criteria determine the C# technique to use when reversing a string:
- Performance: We will use StringBuilder or Array.Reverse for large strings; LINQ for smaller strings.
- Readability: We will use LINQ to produce concise and readable code.
- Memory Efficiency: StringBuilder is preferred to avoid multiple string allocations.
- Code Complexity: We will use simple methods like LINQ or Array.Reverse for less complex scenarios; manual loops for specific control.
Read More: |
Understanding Type Casting or Type Conversion in C# |
Arrays in C#: Create, Declare, and Initialize the Arrays |
Loops in C#: For, While, Do While Loops |
Key Features and Benefits of Reversing String in C#
Here are the key features and benefits of reversing string in C#:
Key Features of Reversing String in C#
- Palindrome Checking: Verify if a string is the same forwards and backward.
- Text Processing: Reverse user input or transform data.
- Algorithm Challenges: Solve common coding problems and exercises.
- String Manipulation: Practice handling arrays, loops, and string operations.
Benefits of Reversing String in C#
- Improves Problem-Solving Skills: Enhances understanding of algorithms and data structures.
- Increases Code Efficiency: Learn to optimize string operations and memory usage.
- Boosts Readability: Helps in writing clean and maintainable code.
- Versatile Applications: Useful in various real-world scenarios and applications.
Conclusion
In the above article, we will learn what the reverse string in C# is and multiple methods for easily reversing a string. Effective string reversing is essential whether you're processing text, checking for palindromes, working with data, or resolving algorithmic problems. Based on readability, memory efficiency, and performance requirements, developers can select the best technique by utilizing Array.Reverse, LINQ's Reverse(), or StringBuilder. Also, Consider our C# Programming Course for a better understanding of all C# concepts.
FAQs
Take our Csharp skill challenge to evaluate yourself!
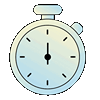
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.