21
FebSwitch Statement in C#: A Complete Guide
Switch Statement in C#
The switch statement in C# is a helpful way to simplify decision-making in your code. Instead of using several if-else conditions, you can use the switch statement to match a value against multiple cases. Each case corresponds to a different block of code, and if the value matches a case, the associated code runs.
This C# tutorial provides you with an easy way to understand what is the switch statement in C#?, why do we use switch statements in C#, the difference between if-else and switch statements, case patterns in switch statement in c#, and many more. So, let's first understand what a switch statement is in C#.
To gain a deeper understanding of switch statements and other C# concepts, be sure to enroll in our Free C Sharp Course for a comprehensive learning experience.
If you are a beginner, this C# Developer Roadmap article will help you understand the complete path for C# programming.
What is the switch statement in C#?
In simple words, you may verify a value and make decisions depending on it by using a switch statement in C#. It's similar to selecting a particular course of action based on the option or number you receive.
Imagine you have a remote control with buttons:
- If you press button "1," it plays music.
- If you press button "2," it plays a movie.
- If you press anything else, it says, "Invalid button."
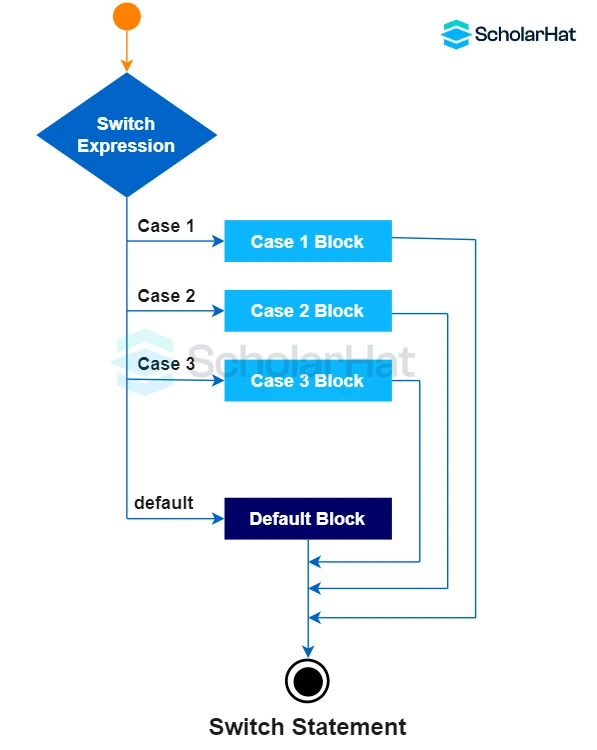
'break' Keyword in the switch Statement
The break keyword in a switch statement stops the program from running the next cases after a match is found. Without a break, the program will keep running the following cases, even if they don't match.
'default' Keyword in the switch Statement
The default keyword in a switch statement is used when no other case matches the value. It acts like an "else" statement and runs when none of the specified cases are true. Including a default case is optional, but it's useful to handle unexpected values.
Syntax
switch (expression) {
case value1: // statement sequence
break;
case value2: // statement sequence
break;
.
.
.
case valueN: // statement sequence
break;
default: // default statement sequence
}
Example
using System;
int dayOfWeek = 3;
switch (dayOfWeek)
{
case 1:
Console.WriteLine("Monday.");
break;
case 2:
Console.WriteLine("Tuesday.");
break;
case 3:
Console.WriteLine("Wednesday.");
break;
case 4:
Console.WriteLine("Thursday.");
break;
case 5:
Console.WriteLine("Friday.");
break;
default:
Console.WriteLine("weekend!");
break;
}
Explanation
In this example, we declare a variable named 'dayOfWeek' and assign a value of 3 to it; when the switch statement executes, it matches with the 3rd case and prints the result in the console.
Output
Wednesday.
Important points to remember about using switch statements in C#
- Switch statements in C# are used for efficient branching in code.
- They evaluate an expression and compare it to case labels.
- Each case label represents a specific value or range of values.
- The default case is executed if no matches are found.
- Cases can fall through without a break statement if desired.
- Switches can handle different data types, including enums and strings.
- Avoid nesting switch statements excessively for readability.
- C# 8.0 introduced pattern matching in switch expressions for more powerful handling.
- Use switch for multiple branching options and if-else for complex conditions.
- Consider using switch expressions when returning values instead of statements for concise code.
Read More: |
Loops in C#: For, While, Do-While Loops |
Conditional Statements in C#: if, if..else, Nested if, if-else-if |
Why do we use Switch Statements instead of if-else statements?
- Readability: When you have a number of criteria to compare to a single value or expression, the switch statements can help make the code easier to understand. Coding understanding and organization are enhanced by the representation of individual branches of logic in each situation.
- Efficiency: Switch statements are often more efficient than long chains of if-else statements because they use a jump table or similar mechanisms, resulting in faster execution for large numbers of cases.
- Intent: Using a switch statement conveys the intention that you are comparing a single value against multiple possible values, making your code more self-explanatory.
- Maintenance: Switch statements are easier to maintain when you need to add or remove cases because you only need to modify the switch block, whereas if-else chains require changes throughout the code.
- Enumerations: Switch statements work well with enumerated types, making code cleaner and less error-prone when dealing with predefined sets of values.
- Code Quality: Code analyzers and compilers can better optimize switch statements, leading to potentially more efficient and error-free code.
However, if-else statements are still valuable for handling more complex conditions or when you need to evaluate expressions or conditions that cannot be easily represented by simple value comparisons. The choice between switch and if-else depends on the specific requirements of your code and readability considerations.
Using goto in the Switch Statement
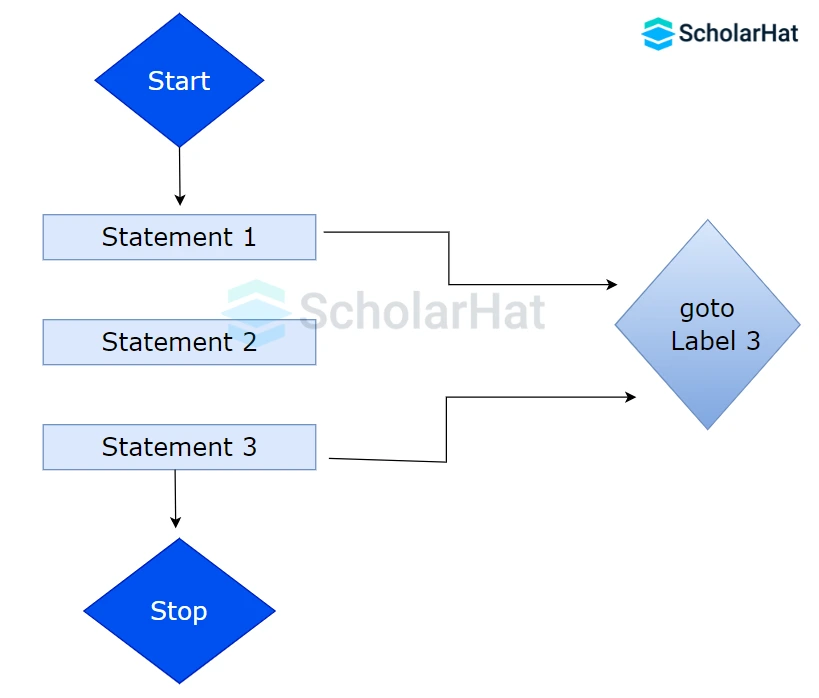
In C#, you may use goto inside a switch statement, but you should use it carefully. The goto statement is often avoided since it might make code harder to comprehend and maintain. On the other hand, it could be a sensible strategy to manage the program's flow under some uncommon circumstances.
Example
using System;
class Program
{
static void Main()
{
int option = 2;
string result = "Undefined";
switch (option)
{
case 1:
result = "Option 1 is selected.";
break;
case 2:
goto case 1; // Jump to case 1
case 3:
result = "Option 3 is selected.";
break;
}
Console.WriteLine(result);
}
}
Explanation
In this program, We created two variables, 'option' and 'result, 'and assigned a '2' number. When the switch statement executes, it matches with the second case, where it jumps to the first case and prints the results.
Output
Option 1 is selected.
Some Other Examples of Switch Statements in C#
Here, we will provide you with some more examples to help you understand the switch statement in C#.
Example-1. Grading System
using System;
class Program
{
static void Main()
{
char grade = 'B';
switch (grade)
{
case 'A':
Console.WriteLine("Excellent!");
break;
case 'B':
Console.WriteLine("Good Job!");
break;
case 'C':
Console.WriteLine("You passed");
break;
case 'D':
Console.WriteLine("You need to work harder");
break;
case 'F':
Console.WriteLine("Failed");
break;
default:
Console.WriteLine("Invalid grade");
break;
}
}
}
Output
Good Job!
Explanation
In this example, we create a variable named grade and assign this value B. When the switch statement executes, it matches with the case and prints 'Good Job!' in the console
Example-2. Using the switch with Strings
using System;
class Program
{
static void Main()
{
string fruit = "Apple";
switch (fruit)
{
case "Apple":
Console.WriteLine("Apples are red or green");
break;
case "Banana":
Console.WriteLine("Bananas are yellow");
break;
case "Orange":
Console.WriteLine("Oranges are orange");
break;
default:
Console.WriteLine("Unknown fruit");
break;
}
}
}
Output
Apples are red or green
Explanation
In this example, declare a string variable and store Apple in it. When the switch statement is executed, it matches the case and prints' Apples are red or green' in the console.
Example-3. Using Enums in Switch
using System;
class Program
{
enum Direction { North, South, East, West }
static void Main()
{
Direction dir = Direction.East;
switch (dir)
{
case Direction.North:
Console.WriteLine("Heading North");
break;
case Direction.South:
Console.WriteLine("Heading South");
break;
case Direction.East:
Console.WriteLine("Heading East");
break;
case Direction.West:
Console.WriteLine("Heading West");
break;
default:
Console.WriteLine("Invalid Direction");
break;
}
}
}
Output
Heading East
Explanation
In this example, we declare an enum of 'direction' and declare a variable of enum 'dir' and store 'East.' when the switch statement executes, it matches with the case and prints 'Heading East' in the console.
Example-4. Using multiple case statements in one switch
using System;
class Program
{
static void Main()
{
int day = 6;
switch (day)
{
case 1:
case 2:
case 3:
case 4:
case 5:
Console.WriteLine("It's a weekday.");
break;
case 6:
case 7:
Console.WriteLine("It's the weekend.");
break;
default:
Console.WriteLine("Invalid day.");
break;
}
}
}
Output
It's the weekend.
Explanation
In this example, we declare a variable 'day' and store the number 6 in it. When the switch statement executes, it matches with the cases where the condition for 6 and 7 numbers are the same, so it prints the It's the weekend result in the console.
Case Patterns in 'Switch' Statement
In C#, the Case pattern is introduced in the C# 7.0 version and is part of pattern matching in switch statements. This case pattern allows you to check the condition depending on the type of the variable, properties of the object, or conditions within the case itself.
1. Constant pattern
A constant pattern in C# means that a switch statement checks if a value is exactly equal to a specific constant value (like a number or string). If the value matches, the code inside that case runs.
Example
using System;
class Program
{
static void Main()
{
int number = 5;
switch (number)
{
case 1:
Console.WriteLine("Number is one");
break;
case 5:
Console.WriteLine("Number is five");
break;
default:
Console.WriteLine("Number is unknown");
break;
}
}
}
Output
Number is five
Explanation
In this example, we have declared a variable named 'number' and stored 5 in it. When the switch statement executes, it matches the condition and prints the 'Number is five' result in the console.
2. Type Pattern
A type pattern in C# is used in a switch statement to check if a value is of a specific type (like int, string, etc.). If the value matches the type, the code for that case will run.
Example
using System;
class Program
{
static void Main()
{
object value = 42;
switch (value)
{
case int i:
Console.WriteLine($"It's an integer: {i}");
break;
case string s:
Console.WriteLine($"It's a string: {s}");
break;
default:
Console.WriteLine("Unknown type");
break;
}
}
}
Output
It's an integer: 42
Explanation
In this example, we declare an object name value and store 42 in it. When the switch statement executes, it matches the particular case and its value type and prints the 'It's an integer: 42' result in the console.
Differences Between If-Else and Switch Statements
Parameters | If-else statement | Switch statement |
Expression Comparison | The if-else statement allows you to evaluate any Boolean expression as a condition. You can use relational operators, logical operators, and any valid expression to determine the flow of execution. | The switch statement, on the other hand, evaluates a single expression and compares it against various cases. It only supports equality comparisons (==) and cannot handle more complex conditions like ranges or logical operators. |
Multiple Conditions | With if-else, you can have multiple conditions and use logical operators (e.g., &&, ||) to combine them. | In switch, you can only compare a single expression against different cases. Each case represents a specific value, and the control flow transfers to the matching case. It doesn't support combining multiple conditions directly. |
Execution Flow | In the if-else statement, the conditions are evaluated one by one from top to bottom until a true condition is found. Once a condition is true, the corresponding block of code executes, and the rest of the conditions are skipped. | In the switch statement, the expression is evaluated once, and the control jumps directly to the matching case (or the default case if no matches are found). After executing the code block for the matching case, the switch statement is exited unless you explicitly use break statements to control the flow. |
Flexibility | The if-else statement provides more flexibility in terms of the conditions and actions you can perform. You can have nested if-else statements and handle more complex scenarios. It is suitable for conditions that involve ranges, multiple variables, or custom logic. | The switch statement is more concise and readable when you have a single expression to compare against different cases. It's commonly used for scenarios where you have a fixed set of possible values or enumerations. |
Read More: |
Top 50 C# Interview Questions and Answers To Get Hired |
OOPs Interview Questions and Answers in C# |
Conclusion
In conclusion, the switch statement in C# is a versatile and powerful control structure that offers a cleaner and more efficient alternative to nested if-else statements in many scenarios. This switch statement also helps you to find the complex type of case patterns in C#. For mastering C# programming language, ScholarHat provides you with a Full-Stack .NET Developer Certification Training Course.
FAQs
- Primitive types like int, char, bool
- Strings
- Enums
- Patterns (like type patterns and constant patterns)
- Other types, like float, double, or decimal, cannot be directly used in a switch.
Take our Csharp skill challenge to evaluate yourself!
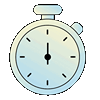
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.