18
JulTypes of Classes in C#
Understanding Types of Classes in C#
In C#, classes are the foundation of object-oriented programming, and understanding their types is key to writing efficient code. Different types of classes, such as static, sealed, and abstract, serve specific purposes, allowing you to structure your programs effectively and reuse code where needed.
In this Csharp tutorial, I will walk you through the different types of classes in C#, explaining how they work and when to use them. Do not worry. We will simplify everything so it is easy to understand and apply. Ready to explore the types of classes in C#? Let us get started!
What Are Classes in C#?
In C#, a class is a user-defined data type that describes the state and behavior of an object. The state is represented by the properties, and the behavior refers to the actions or methods the object can perform. Doesn't that sound simple?
Access Specifiers for Classes
You can use access specifiers to control how a class interacts with other parts of your program. Here are the common ones:
- Public: Allows access from anywhere in your code.
- Private: Restricts access to within the class only.
- Protected: Grants access to the class and its derived classes.
- Internal: Limits access to within the same assembly.
- Protected Internal: Combines protected and internal access.
Example of a Class
Here’s a quick example to get you started:
public class Accounts
{
// Properties and methods go here
}
Key Points About Classes
- Reference Types: Classes are reference types, and objects created from them are stored in the heap.
- Base Type: All classes inherit from the
System.Object
class by default. - Default Access: Classes default to
Internal
access, while methods and variables default toPrivate
. - Private Restrictions: You can't declare private classes directly inside a namespace.
Types of Classes in C#
C# provides various types of classes to cater to different programming scenarios. Each class type serves a specific purpose, enabling efficient code management and functionality.
- Abstract Class:Abstract Class acts as a blueprint for other classes. It cannot be instantiated and is designed to be inherited.
- Concrete Class: A fully implemented class that can be instantiated and used directly in your programs.
- Sealed Class: Prevents inheritance, ensuring that the class's functionality cannot be extended.
- Static Class: Designed to hold only static members, it cannot be instantiated or inherited.
- Partial Class: Splits a single class definition into multiple files for better code organization and readability.
- Nested Class: A class defined within another class, useful for logically grouping related functionality.
- Generic Class: A class that allows the use of type parameters, enabling code reusability with different data types.
Read More: Partial Class, Interface, or Struct in C# with Example |
Types of Classes in C# with Examples
1. Abstract Classes
These classes are designed to serve as base classes for others. You can't create objects from them, but you can define shared properties or methods that derived classes must implement.
Use Cases:
- Defining common behavior for related classes.
- Enforcing implementation of specific methods in derived classes.
- Providing a template for multiple inheritance scenarios.
Example
using System;
abstract class Shape
{
public abstract void Draw();
}
class Circle : Shape
{
public override void Draw()
{
Console.WriteLine("Drawing a Circle.");
}
}
class Program
{
static void Main()
{
Shape myShape = new Circle();
myShape.Draw();
}
}
Output
Drawing a Circle.
2. Concrete Classes
These are the most common classes in C#. They can be instantiated and used directly to create objects and perform operations.
Use Cases:
- Implementing real-world objects like cars or users.
- Building custom classes for business logic.
- Encapsulating data and methods.
Example
using System;
class Car
{
public string Brand { get; set; }
public void Drive()
{
Console.WriteLine($"{Brand} is driving.");
}
}
class Program
{
static void Main()
{
Car myCar = new Car { Brand = "Tesla" };
myCar.Drive();
}
}
Output
Tesla is driving.
3. Sealed Classes
These classes cannot be inherited, making them ideal for scenarios where you want to restrict modifications through inheritance.
Use Cases:
- Securing sensitive functionality.
- Ensuring the integrity of a specific implementation.
- Providing a final implementation for utility or helper classes.
Example
using System;
sealed class Logger
{
public void Log(string message)
{
Console.WriteLine($"Log: {message}");
}
}
class Program
{
static void Main()
{
Logger logger = new Logger();
logger.Log("Application started.");
}
}
Output
Log: Application started.
4. Static Classes
These classes cannot be instantiated and are used for utility functions. They contain only static members.
Read More: C# Extension Method |
Use Cases:
- Creating reusable utility methods.
- Encapsulating global logic like configuration or validation.
- Providing mathematical operations.
Example
using System;
static class MathHelper
{
public static int Add(int a, int b)
{
return a + b;
}
}
class Program
{
static void Main()
{
Console.WriteLine($"Sum: {MathHelper.Add(5, 7)}");
}
}
Output
Sum: 12
5. Partial Classes
These classes allow you to split the definition into multiple files, making it easier to manage large projects.
Use Cases:
- Organizing code in large classes.
- Supporting auto-generated and manual code together.
- Improving readability and maintainability.
Example
// File 1
public partial class Employee
{
public void DisplayName()
{
Console.WriteLine("Employee Name: Raj.");
}
}
// File 2
public partial class Employee
{
public void DisplayID()
{
Console.WriteLine("Employee ID: 101.");
}
}
// Main Program
using System;
class Program
{
static void Main()
{
Employee emp = new Employee();
emp.DisplayName();
emp.DisplayID();
}
}
Output
Employee Name: Raj.
Employee ID: 101.
6. Nested Classes
These are classes defined within another class, enabling encapsulation and logical grouping of related classes.
Read More: OOPs Concepts in C# |
Use Cases:
- Encapsulating helper or utility classes within a parent class.
- Providing restricted access to nested class members.
- Organizing related functionality within a single-parent class.
Example
using System;
class OuterClass
{
private string outerMessage = "Outer class message.";
public class NestedClass
{
public void Display()
{
Console.WriteLine("This is the nested class.");
}
}
public void ShowMessage()
{
Console.WriteLine(outerMessage);
}
}
class Program
{
static void Main()
{
OuterClass outer = new OuterClass();
outer.ShowMessage();
OuterClass.NestedClass nested = new OuterClass.NestedClass();
nested.Display();
}
}
Output
Outer class message.
This is the nested class.
7. Generic Classes
These classes are type-safe and reusable, allowing you to define a class with a placeholder for the type it operates on.
Use Cases:
- Creating collections such as lists or dictionaries.
- Implementing algorithms that work with multiple data types.
- Enhancing code reusability and reducing redundancy.
Read More: Generics in C# |
Example
using System;
class GenericClass
{
private T data;
public GenericClass(T value)
{
data = value;
}
public void DisplayData()
{
Console.WriteLine($"Data: {data}");
}
}
class Program
{
static void Main()
{
GenericClass intObj = new GenericClass(10);
intObj.DisplayData();
GenericClass stringObj = new GenericClass("Hello Generics");
stringObj.DisplayData();
}
}
Output
Data: 10
Data: Hello Generics
Summary
In C#, classes define the state and behavior of objects, making your code more organized and efficient. We covered types like abstract classes, sealed classes, static classes, partial classes, nested classes, and generic classes, each designed for specific use cases. Mastering these helps you write better programs.
Ready to boost your skills? Enroll in these free courses:
Did You Know? Quiz - Types of Classes in C#
Q1: "A static class can be instantiated in C#."
- True
- False
Q2: "A partial class allows the splitting of a class definition across multiple files."
- True
- False
Q3: "A sealed class can be inherited in C#."
- True
- False
Q4: "Abstract classes cannot have any implementation of methods in C#."
- True
- False
Q5: "A class defined as `internal` can be accessed only within its own assembly."
- True
- False
FAQs
Take our Csharp skill challenge to evaluate yourself!
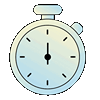
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.