22
DecIntroduction to Adapter Design Pattern
Adapter Design Pattern
The Adapter Design Pattern is crucial for integrating components with incompatible interfaces. It allows objects with different interfaces to work together seamlessly by converting the interface of one class into another that a client expects. This pattern enhances flexibility by enabling interoperability between disparate systems or components.
In this design patterns tutorial, we will explore the Adapter Design Pattern which includes "What is the Adapter Design Pattern?" We’ll also discuss "When should you use the Adapter Design Pattern?" and provide examples to illustrate its use. Let’s start with, "What is the Adapter Design Pattern?"
What is the Adapter Design Pattern?
- The Adapter Design Pattern is a structural design pattern that enables objects with incompatible interfaces to interact.
- It acts as a bridge between two incompatible interfaces by converting one interface into another, which a client expects.
- This pattern helps in integrating new components with legacy systems or third-party libraries without modifying their existing code.
- The Adapter pattern converts one interface into another that a client expects, allowing two incompatible interfaces to work together.
- It involves creating an adapter class that implements the target interface and translates requests to the adaptee (the component with the incompatible interface).
- Promotes code reusability and flexibility by allowing integration between components with different interfaces without altering their source code.
Why do we need the Adapter Design Pattern?
1. Integration with Legacy Systems
- Allows new components to work with legacy systems by adapting their interfaces to match the expected interface of the new components.
- Avoids modifying existing code, which could introduce bugs or require extensive testing.
2. Interoperability
- Enables different systems or components to communicate by providing a standard interface, facilitating integration across diverse platforms.
- Helps in unifying systems that were not designed to work together.
3. Code Reusability
- Allows reuse of existing components without modifying them, promoting cleaner and more maintainable code.
- Avoids code duplication and adheres to the open/closed principle, where code entities are open for extension but closed for modification.
4. Flexibility and Scalability
- Enhances flexibility by allowing systems to be extended or changed without impacting existing code.
- Supports scalability by making it easier to integrate new components with varying interfaces into existing systems.
Simplified Client Code
- Simplifies client code by providing a consistent interface, allowing clients to interact with different systems through a uniform API.
- Clients are unaware of the underlying complexity or differences between interfaces.
Real-world illustration of Adapter Design Pattern
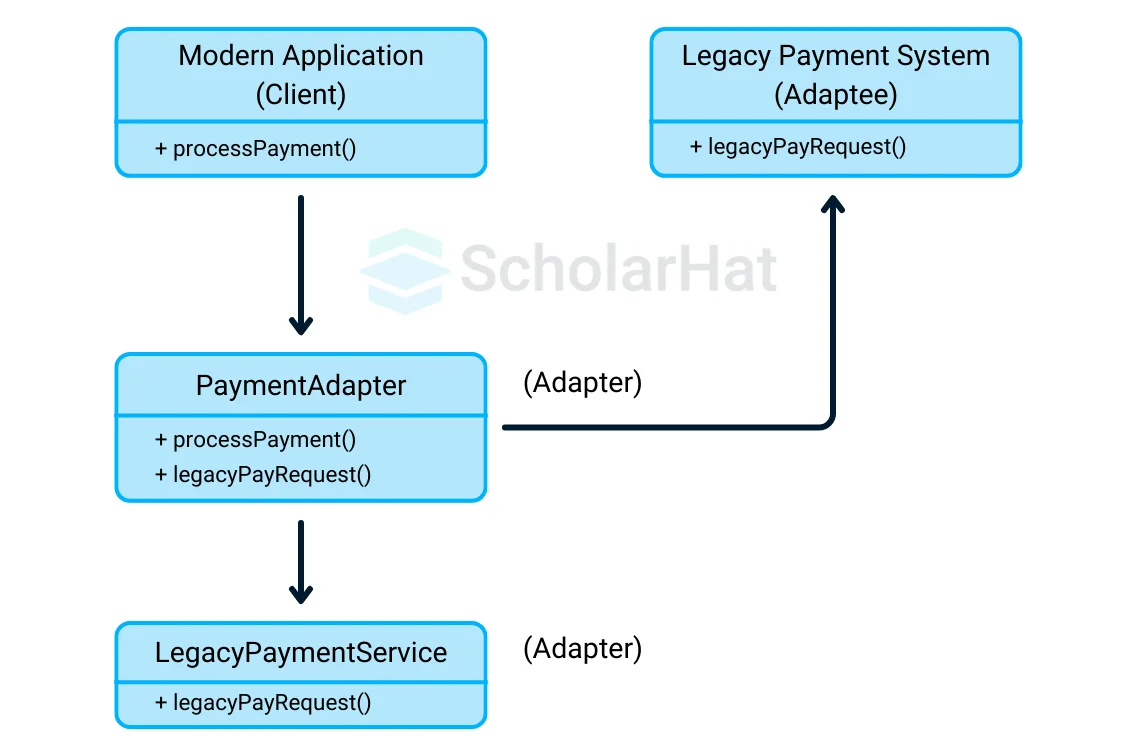
- Imagine a modern application that needs to integrate with a legacy payment gateway system.
- The modern application expects payment processing through a specific API interface, while the legacy system uses a completely different interface.
- An adapter can bridge the gap between the modern API and the legacy system’s API, translating calls and enabling seamless interaction without changing either system.
Adapter Design Pattern Basic Structure and Implementation
The structure for the implementation of the Adapter Design Pattern includes the following key components:
1. Target Interface
- Defines the domain-specific interface that the client uses.
- This interface specifies the methods that clients expect to use.
- For example, a modern application that expects a PaymentProcessor interface defines methods like processPayment().
2. Adaptee
- Represents the existing interface that needs adapting.
- It provides the functionality that the client needs but has an incompatible interface.
- For instance, the legacy payment system has a method like legacyPayRequest() that is different from what the client expects.
3. Adapter
- Implements the target interface and translates client requests to the Adaptee’s interface.
- It delegates calls to the Adaptee and transforms them as necessary to make them compatible.
- For example, the adapter would implement processPayment() but internally call legacyPayRequest() from the legacy system.
Real-Life Example
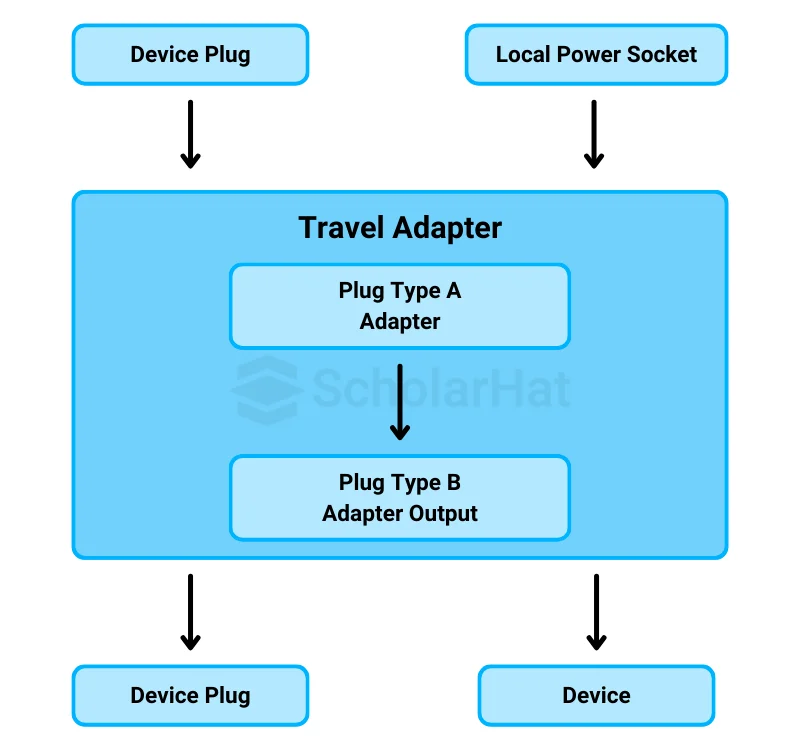
Subsystems (Different Electrical Outlets and Devices)
- Electronic Devices: These are items like laptops, phones, and chargers. They are designed to plug into specific types of outlets and may require different voltages.
- Electrical Outlets: Different countries have different plug types and voltage standards. Each country uses its own type of socket for powering devices.
Adapter (Travel Adapter)
- Travel Adapter: It is used to connect your device's plug to a different type of power outlet. It adapts the plug so that it fits into local sockets and ensures the device gets the correct voltage.
Operation Flow
- Unified Operation: When you use the travel adapter, it allows your device to connect to various power outlets in different countries. You can plug in your laptop or charger, regardless of the local outlet type.
- Plug Type Conversion: The travel adapter changes the plug shape to match local sockets, allowing your device to connect properly.
- Voltage Handling: Some travel adapters also convert voltage so your device receives the correct power level, protecting it from damage.
How the Adapter Helps
- Unified Structure: It allows you to use your electronic devices with different types of power outlets. It is like having one adapter that works anywhere.
- Simplified Management: Using a travel adapter means you do not need to worry about changing your device or finding different plugs for each country. It simplifies your travel.
- Consistent Behavior: Whether you are in Europe, Asia, or the Americas, the travel adapter ensures your device can be used with local power systems. It is straightforward to connect your devices regardless of the location.
Example
// C# Code - Adapter Pattern
using System;
// Target Interface
public interface IDevice {
void Connect();
}
// Adaptee
public class EuropeanPlug {
public void PlugIn() {
Console.WriteLine("European plug connected.");
}
}
// Adapter
public class TravelAdapter : IDevice {
private EuropeanPlug _europeanPlug;
public TravelAdapter(EuropeanPlug europeanPlug) {
_europeanPlug = europeanPlug;
}
public void Connect() {
_europeanPlug.PlugIn();
}
}
// Client Code
public class AdapterExample {
public static void Main(string[] args) {
EuropeanPlug europeanPlug = new EuropeanPlug();
IDevice adapter = new TravelAdapter(europeanPlug);
// Using the adapter to connect the European plug
adapter.Connect();
}
}
# Python Code - Adapter Pattern
# Target Interface
class Device:
def connect(self):
pass
# Adaptee
class EuropeanPlug:
def plug_in(self):
print("European plug connected.")
# Adapter
class TravelAdapter(Device):
def __init__(self, european_plug):
self.european_plug = european_plug
def connect(self):
self.european_plug.plug_in()
# Client Code
european_plug = EuropeanPlug()
adapter = TravelAdapter(european_plug)
# Using the adapter to connect the European plug
adapter.connect()
// Java Code - Adapter Pattern
// Target Interface
interface Device {
void connect();
}
// Adaptee
class EuropeanPlug {
void plugIn() {
System.out.println("European plug connected.");
}
}
// Adapter
class TravelAdapter implements Device {
private EuropeanPlug europeanPlug;
public TravelAdapter(EuropeanPlug europeanPlug) {
this.europeanPlug = europeanPlug;
}
@Override
public void connect() {
europeanPlug.plugIn();
}
}
// Client Code
public class AdapterExample {
public static void main(String[] args) {
EuropeanPlug europeanPlug = new EuropeanPlug();
Device adapter = new TravelAdapter(europeanPlug);
// Using the adapter to connect the European plug
adapter.connect();
}
}
// TypeScript Code - Adapter Pattern
// Target Interface
interface Device {
connect(): void;
}
// Adaptee
class EuropeanPlug {
plugIn(): void {
console.log("European plug connected.");
}
}
// Adapter
class TravelAdapter implements Device {
private europeanPlug: EuropeanPlug;
constructor(europeanPlug: EuropeanPlug) {
this.europeanPlug = europeanPlug;
}
connect(): void {
this.europeanPlug.plugIn();
}
}
// Client Code
const europeanPlug = new EuropeanPlug();
const adapter: Device = new TravelAdapter(europeanPlug);
// Using the adapter to connect the European plug
adapter.connect();
// JavaScript Code - Adapter Pattern
// Target Interface
class Device {
connect() {}
}
// Adaptee
class EuropeanPlug {
plugIn() {
console.log("European plug connected.");
}
}
// Adapter
class TravelAdapter extends Device {
constructor(europeanPlug) {
super();
this.europeanPlug = europeanPlug;
}
connect() {
this.europeanPlug.plugIn();
}
}
// Client Code
const europeanPlug = new EuropeanPlug();
const adapter = new TravelAdapter(europeanPlug);
// Using the adapter to connect the European plug
adapter.connect();
Explanation
- This Java code illustrates the Adapter Pattern. The Device interface defines the expected connect() method.
- The EuropeanPlug class has a different method, plugIn().
- The TravelAdapter implements the Device interface and adapts EuropeanPlug by translating the connect() method to plugIn(), enabling the client to use the European plug seamlessly.
Applications of Adapter Design Pattern
1. Use the Adapter class if you wish to use an existing class, but its interface is incompatible with the rest of your code.
- The Adapter design allows you to define a middle-layer class that acts as a translator between your code and a legacy class, a third-party class, or any other class with an unusual interface.
2. Use the approach when you want to reuse many existing subclasses that lack common functionality that cannot be added to the superclass.
- You could extend each subclass and move missing functionality to new child classes.
- However, you will have to duplicate the code across all of these new classes, which smells very poor.
- The much more elegant solution would be to provide the needed functionality to an adapter class.
- The objects that lack features would then be wrapped inside the adaptor, with the relevant features added dynamically.
- For this to operate, the target classes must share a common interface, and the adapter's field must conform to that interface.
- This approach is quite similar to the Decorator pattern.
Why do we need an Adapter Design Pattern?
1. Integration of Existing Code
- Scenario: Existing code or components have interfaces that are incompatible with those expected by new code or systems.
- Need: The Adapter design pattern enables you to seamlessly incorporate existing components into new systems without changing their original code.
2. Reuse of Existing Functionality
- Scenario: You wish to reuse classes or components that have useful functionality but do not comply with the intended interface.
- Need: The Adapter pattern allows you to reuse old code by constructing an adapter that converts it to the interfaces expected by new code.
3. Interoperability
- Scenario: You need to make different systems or components operate together, especially if their interfaces are different.
- Need: The Adapter pattern functions as a bridge, allowing systems with mismatched interfaces to work together efficiently.
4. Client-Server Communication
- Scenario: When creating client-server applications, the client expects one interface while the server provides another.
- Need: Adapters help to translate requests and responses between client and server, ensuring smooth communication despite interface inconsistencies.
5. Third-Party Library Integration
- Scenario: When using third-party libraries or APIs in a project, their interfaces do not match the rest of the system.
- Need: Adapters enable the use of external components by providing an appropriate interface for the remainder of the program.
When not to use the Adapter Design Pattern?
1. When Interfaces are Stable
- Scenario: If the interfaces between the present system and the new system are stable and unlikely to change frequently.
- Reason: Adapters are very useful when dealing with changing or incompatible interfaces. If the connections are stable, the costs of maintaining adapters may outweigh the advantages.
2. When Direct Modification Is Feasible
- Scenario: You have control over the existing system's source code and can directly adjust its interface to match the target interface.
- Reason: If you can modify the existing code, directly adapting interfaces may be a simpler and more straightforward approach than implementing adapters.
3. When performance is critical
- Scenario: In performance-critical applications when the overhead caused by the Adapter approach is unacceptable.
- Reason: Adapters may add a layer of indirection and abstraction that has a minor impact on performance. In situations where every bit of performance counts, the Adapter pattern may not be the best option.
4. When Multiple Adapters Are Required
- Scenario: If a system requires several adapters for diverse components, the complexity of handling these adapters becomes overwhelming.
- Reason: Managing a large number of adapters may result in increased complexity and maintenance requirements. In such circumstances, rethink the entire design or examine alternatives.
5. When adapters introduce ambiguity
- Scenario: Introducing adapters creates ambiguity or complexity in the overall system design.
- Reason: If the use of adapters makes the system design less apparent or more difficult to grasp, it may be desirable to explore other alternatives that provide a clearer design.
Relationship of Adapter Patterns with Other Patterns
1. Facade design:It is comparable to the Facade design in that both simplify interactions. However, the Adapter pattern concentrates on transforming one interface into another, whereas the Facade provides a simplified interface without modifying the underlying interfaces.
2. Bridge design: It differs from the Bridge design in that the Adapter matches incompatible interfaces, but the Bridge separates abstraction and implementation, allowing both to evolve independently.
3. Decorator Pattern: It differs from the Decorator pattern in that the Adapter modifies interfaces, whereas the Decorator adds new functionality to objects without changing interfaces.
4. Proxy Pattern: It complements the Proxy Pattern by allowing the Adapter to adapt interfaces before the Proxy controls access to the object.
5. Singleton Pattern: It is frequently combined with the Singleton pattern to ensure that just one adapter manages interface conversion consistently throughout the system.
Summary
The Adapter Design Pattern enables incompatible interfaces to communicate by turning one interface into another. It is important for integrating legacy systems, encouraging code reuse, and ensuring adaptability when introducing new components. This style simplifies client code and promotes interoperability across multiple platforms. To master design patterns, enroll in ScholarHat's Master Software Architecture and Design Certification Training.