17
JanChain of Responsibility Design Pattern
Chain of Responsibility Design Pattern
Chain of Responsibility Design Pattern is important for creating a flexible way to pass requests along a chain of handlers, where each handler can either process the request or pass it along to the next handler in the chain. This pattern promotes loose coupling between the sender and receiver, allowing for dynamic processing of requests.
In this design patterns tutorial, we will explore the Chain of Responsibility Design Patternincluding, "What is the Chain of Responsibility Design Pattern?" We’ll also discuss "When should I use the Chain of Responsibility Design Pattern?" and provide examples to illustrate its use. Let’s start with, "What is the Chain of Responsibility Design Pattern?"
What is the Chain of Responsibility Design Pattern?
- The Chain of Responsibility Design Pattern is a behavioral design pattern that allows a request to pass through a chain of handlers, where each handler decides either to process the request or forward it to the next handler in the chain.
- It defines a method for linking handlers together, promoting flexibility in how requests are handled without specifying the handler explicitly.
- This approach separates the request sender from the receiver by letting different objects handle the request dynamically.
- The Chain of Responsibility pattern ensures that the client doesn’t need to know which handler will process the request, improving system flexibility and maintainability.
- It encourages the use of chained handlers to manage requests, preventing tight coupling between objects and promoting better scalability.
Why do we need the Chain of Responsibility Design Pattern?
1. Simplified Request Handling
- It provides a structured way to pass requests through a chain of handlers, ensuring that each handler can either process the request or pass it along to the next one without needing to know the entire chain.
- Clients don't need to know which handler will handle the request, simplifying request handling logic.
2. Decouples Request Senders and Handlers
- The Chain of Responsibility Design Pattern decouples the request sender from the specific handler that processes the request.
- Whether the handler is at the beginning or end of the chain, the client doesn't interact with it directly, allowing changes to the chain without affecting client code.
3. Improved Code Flexibility and Scalability
- This pattern promotes reuse by allowing you to add new handlers without modifying the existing request processing logic.
- It supports the open/closed principle, as you can extend the chain by adding new handlers without altering the existing chain structure.
4. Dynamic Request Processing
- Allows you to create flexible, dynamic processing chains where requests can be passed, handled, or rejected at any point.
- It makes it easy to change the order of handlers or add new ones based on the system's needs without disrupting existing functionality.
5. Reduced Coupling Between Objects
- The pattern minimizes tight coupling between request senders and handlers, making the system more maintainable and adaptable to change.
- Handlers are only responsible for processing specific requests, which improves modularity.
6. Consistent Request Flow
- It provides a consistent flow of request handling, ensuring that the client doesn't need to worry about the underlying complexity of how requests are processed or passed along.
- This simplifies client code, as it doesn't need to manage the request flow directly.
7. Support for Complex Processing Chains
- The Chain of Responsibility Pattern supports complex chains of handlers, making it ideal for systems where multiple steps or decision points are needed to process a request.
- It simplifies handling requests in scenarios where processing logic needs to be shared or split across multiple objects.
Real-world Illustration of Chain of Responsibility Design Pattern
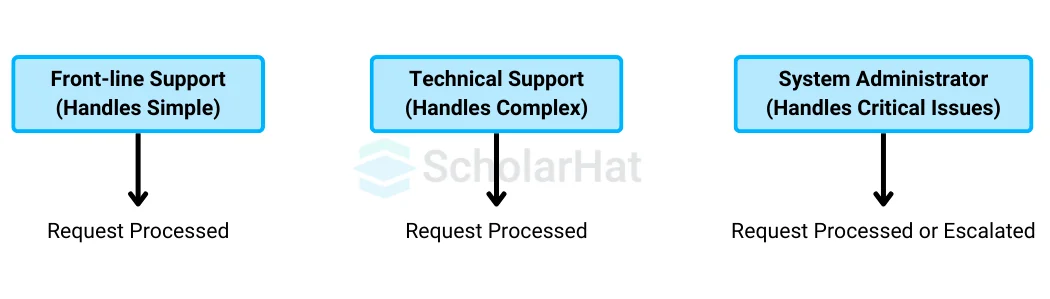
- Imagine a help desk in a company where different levels of support staff handle various types of problems.
- It is designed so that the front-line support team resolves simple issues, more complex issues are forwarded to the technical team, and critical problems are escalated to the system administrator.
- When a user submits a request, it is first reviewed by the front-line support.
- If they cannot solve it, they pass it to the technical team, and if necessary, the system administrator will handle it.
- This is similar to the Chain of Responsibility Pattern, where each level either resolves the issue or forwards it to the next handler until it is addressed.
Chain of Responsibility Design Pattern Basic Structure and Implementation
The structure for the implementation of the Chain of Responsibility Design Pattern includes the following key components:
1. Handler Interface
- Defines the method for handling requests and for passing the request to the next handler in the chain.
- This interface typically includes a handleRequest() method that processes the request or forwards it to the next handler if it cannot be handled.
- For example, in a help desk system, the SupportHandler interface might have a method handleIssue() to address a support ticket.
2. Concrete Handler
- Implements the Handler interface and processes specific types of requests.
- Each concrete handler is responsible for handling a specific part of the request, or it forwards the request to the next handler in the chain if it cannot process it.
- For instance, a TechnicalSupportHandler may address more complex issues, while simpler requests are handled by the FrontLineSupportHandler.
3. Client
- Initiates a request and sends it to the first handler in the chain.
- The client does not need to know the internal logic of how the request is processed.
- It is only passed through a series of handlers until it is addressed.
- For example, a User might submit a support ticket, which is passed through different levels of support (e.g., front-line, technical, admin).
4. Chain Setup
- The sequence of handlers is set up in such a way that each handler forwards the request to the next one if it cannot handle it.
- For example, a FrontLineSupportHandler passes the request to the TechnicalSupportHandler if the issue is too complex, and so on.
Real-Life Example: Customer Support System
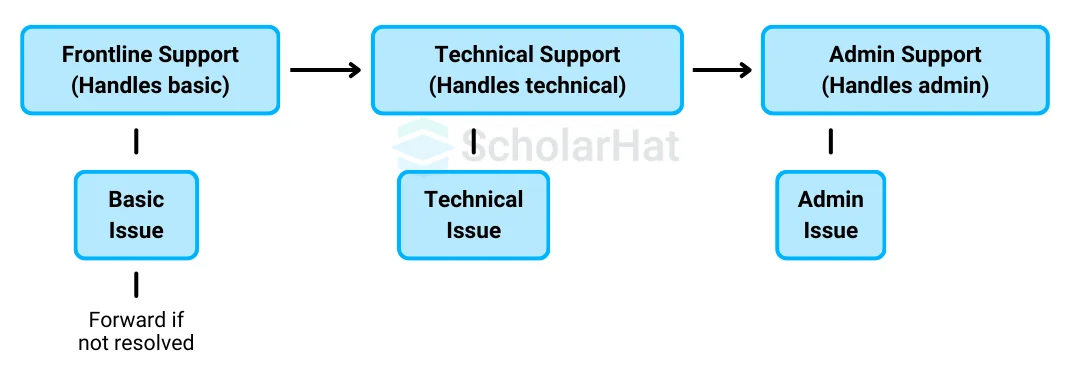
1. Chain of Responsibility (Customer Support Levels)
- Base Component: The customer submits a support request. The request could be a basic query, technical issue, or administrative concern.
2. Support Levels (Handlers)
- FrontLine Support: Handles basic, common queries that can be resolved quickly.
- Technical Support: Deals with technical issues requiring more expertise.
- Admin Support: Manages administrative concerns that frontline or technical support cannot address.
3. Operation Flow
- Frontline Support: The customer’s request is first handled by frontline support. If it’s a basic issue, it is resolved here. If not, it is forwarded to the next support level.
- Technical Support: If the issue requires more technical knowledge, it’s passed to technical support, where more complex issues are handled. If the issue doesn’t match, it is forwarded to admin support.
- Admin Support: If the issue is administrative (e.g., billing, account-related), it is handled at this level. If none of the previous handlers can resolve the issue, admin support will attempt to find a solution.
4. How the Chain of Responsibility Helps
- Efficient Issue Resolution: Each handler in the chain focuses on its area of expertise. The customer’s request is passed through various levels until the appropriate handler is found.
- Scalability: New support levels (like product support, billing support) can be added easily without modifying the existing support structure.
- Separation of Concerns: Each support level only handles issues specific to its domain. Frontline support won’t deal with complex technical issues, and technical support won’t be concerned with administrative tasks.
- Flexible Issue Management: If a handler can’t resolve the issue, it seamlessly forwards it to the next one, ensuring a streamlined support experience.
Example
using System;
// Handler Interface
interface ISupportHandler
{
void SetNextHandler(ISupportHandler nextHandler);
void HandleRequest(string issue);
}
// Concrete Handler 1 - FrontLine Support
class FrontLineSupportHandler : ISupportHandler
{
private ISupportHandler nextHandler;
public void SetNextHandler(ISupportHandler nextHandler)
{
this.nextHandler = nextHandler;
}
public void HandleRequest(string issue)
{
if (issue == "Basic")
{
Console.WriteLine("FrontLine Support: Handling basic issue.");
}
else
{
Console.WriteLine("FrontLine Support: Forwarding to next level.");
nextHandler?.HandleRequest(issue);
}
}
}
// Concrete Handler 2 - Technical Support
class TechnicalSupportHandler : ISupportHandler
{
private ISupportHandler nextHandler;
public void SetNextHandler(ISupportHandler nextHandler)
{
this.nextHandler = nextHandler;
}
public void HandleRequest(string issue)
{
if (issue == "Technical")
{
Console.WriteLine("Technical Support: Handling technical issue.");
}
else
{
Console.WriteLine("Technical Support: Forwarding to next level.");
nextHandler?.HandleRequest(issue);
}
}
}
// Concrete Handler 3 - Admin Support
class AdminSupportHandler : ISupportHandler
{
private ISupportHandler nextHandler;
public void SetNextHandler(ISupportHandler nextHandler)
{
this.nextHandler = nextHandler;
}
public void HandleRequest(string issue)
{
if (issue == "Admin")
{
Console.WriteLine("Admin Support: Handling admin issue.");
}
else
{
Console.WriteLine("Admin Support: No further handler, unable to resolve.");
}
}
}
// Client
class ChainOfResponsibilityDemo
{
static void Main()
{
// Setting up the chain
ISupportHandler frontLineSupport = new FrontLineSupportHandler();
ISupportHandler technicalSupport = new TechnicalSupportHandler();
ISupportHandler adminSupport = new AdminSupportHandler();
frontLineSupport.SetNextHandler(technicalSupport);
technicalSupport.SetNextHandler(adminSupport);
// Client sends requests
Console.WriteLine("Issue: Basic");
frontLineSupport.HandleRequest("Basic");
Console.WriteLine("\nIssue: Technical");
frontLineSupport.HandleRequest("Technical");
Console.WriteLine("\nIssue: Admin");
frontLineSupport.HandleRequest("Admin");
Console.WriteLine("\nIssue: Unknown");
frontLineSupport.HandleRequest("Unknown");
}
}
# Handler Interface
class SupportHandler:
def set_next_handler(self, next_handler):
raise NotImplementedError
def handle_request(self, issue):
raise NotImplementedError
# Concrete Handler 1 - FrontLine Support
class FrontLineSupportHandler(SupportHandler):
def __init__(self):
self.next_handler = None
def set_next_handler(self, next_handler):
self.next_handler = next_handler
def handle_request(self, issue):
if issue == "Basic":
print("FrontLine Support: Handling basic issue.")
else:
print("FrontLine Support: Forwarding to next level.")
if self.next_handler:
self.next_handler.handle_request(issue)
# Concrete Handler 2 - Technical Support
class TechnicalSupportHandler(SupportHandler):
def __init__(self):
self.next_handler = None
def set_next_handler(self, next_handler):
self.next_handler = next_handler
def handle_request(self, issue):
if issue == "Technical":
print("Technical Support: Handling technical issue.")
else:
print("Technical Support: Forwarding to next level.")
if self.next_handler:
self.next_handler.handle_request(issue)
# Concrete Handler 3 - Admin Support
class AdminSupportHandler(SupportHandler):
def __init__(self):
self.next_handler = None
def set_next_handler(self, next_handler):
self.next_handler = next_handler
def handle_request(self, issue):
if issue == "Admin":
print("Admin Support: Handling admin issue.")
else:
print("Admin Support: No further handler, unable to resolve.")
# Client code
if __name__ == "__main__":
# Setting up the chain
front_line_support = FrontLineSupportHandler()
technical_support = TechnicalSupportHandler()
admin_support = AdminSupportHandler()
front_line_support.set_next_handler(technical_support)
technical_support.set_next_handler(admin_support)
# Client sends requests
print("Issue: Basic")
front_line_support.handle_request("Basic")
print("\nIssue: Technical")
front_line_support.handle_request("Technical")
print("\nIssue: Admin")
front_line_support.handle_request("Admin")
print("\nIssue: Unknown")
front_line_support.handle_request("Unknown")
// Handler Interface
interface SupportHandler {
void setNextHandler(SupportHandler nextHandler);
void handleRequest(String issue);
}
// Concrete Handler 1 - FrontLine Support
class FrontLineSupportHandler implements SupportHandler {
private SupportHandler nextHandler;
@Override
public void setNextHandler(SupportHandler nextHandler) {
this.nextHandler = nextHandler;
}
@Override
public void handleRequest(String issue) {
if (issue.equals("Basic")) {
System.out.println("FrontLine Support: Handling basic issue.");
} else {
System.out.println("FrontLine Support: Forwarding to next level.");
if (nextHandler != null) {
nextHandler.handleRequest(issue);
}
}
}
}
// Concrete Handler 2 - Technical Support
class TechnicalSupportHandler implements SupportHandler {
private SupportHandler nextHandler;
@Override
public void setNextHandler(SupportHandler nextHandler) {
this.nextHandler = nextHandler;
}
@Override
public void handleRequest(String issue) {
if (issue.equals("Technical")) {
System.out.println("Technical Support: Handling technical issue.");
} else {
System.out.println("Technical Support: Forwarding to next level.");
if (nextHandler != null) {
nextHandler.handleRequest(issue);
}
}
}
}
// Concrete Handler 3 - Admin Support
class AdminSupportHandler implements SupportHandler {
private SupportHandler nextHandler;
@Override
public void setNextHandler(SupportHandler nextHandler) {
this.nextHandler = nextHandler;
}
@Override
public void handleRequest(String issue) {
if (issue.equals("Admin")) {
System.out.println("Admin Support: Handling admin issue.");
} else {
System.out.println("Admin Support: No further handler, unable to resolve.");
}
}
}
// Client
public class ChainOfResponsibilityDemo {
public static void main(String[] args) {
// Setting up the chain
SupportHandler frontLineSupport = new FrontLineSupportHandler();
SupportHandler technicalSupport = new TechnicalSupportHandler();
SupportHandler adminSupport = new AdminSupportHandler();
frontLineSupport.setNextHandler(technicalSupport);
technicalSupport.setNextHandler(adminSupport);
// Client sends requests
System.out.println("Issue: Basic");
frontLineSupport.handleRequest("Basic");
System.out.println("\nIssue: Technical");
frontLineSupport.handleRequest("Technical");
System.out.println("\nIssue: Admin");
frontLineSupport.handleRequest("Admin");
System.out.println("\nIssue: Unknown");
frontLineSupport.handleRequest("Unknown");
}
}
// Handler Interface
interface SupportHandler {
setNextHandler(nextHandler: SupportHandler): void;
handleRequest(issue: string): void;
}
// Concrete Handler 1 - FrontLine Support
class FrontLineSupportHandler implements SupportHandler {
private nextHandler: SupportHandler | null = null;
setNextHandler(nextHandler: SupportHandler): void {
this.nextHandler = nextHandler;
}
handleRequest(issue: string): void {
if (issue === "Basic") {
console.log("FrontLine Support: Handling basic issue.");
} else {
console.log("FrontLine Support: Forwarding to next level.");
if (this.nextHandler) {
this.nextHandler.handleRequest(issue);
}
}
}
}
// Concrete Handler 2 - Technical Support
class TechnicalSupportHandler implements SupportHandler {
private nextHandler: SupportHandler | null = null;
setNextHandler(nextHandler: SupportHandler): void {
this.nextHandler = nextHandler;
}
handleRequest(issue: string): void {
if (issue === "Technical") {
console.log("Technical Support: Handling technical issue.");
} else {
console.log("Technical Support: Forwarding to next level.");
if (this.nextHandler) {
this.nextHandler.handleRequest(issue);
}
}
}
}
// Concrete Handler 3 - Admin Support
class AdminSupportHandler implements SupportHandler {
private nextHandler: SupportHandler | null = null;
setNextHandler(nextHandler: SupportHandler): void {
this.nextHandler = nextHandler;
}
handleRequest(issue: string): void {
if (issue === "Admin") {
console.log("Admin Support: Handling admin issue.");
} else {
console.log("Admin Support: No further handler, unable to resolve.");
}
}
}
// Client
const frontLineSupport = new FrontLineSupportHandler();
const technicalSupport = new TechnicalSupportHandler();
const adminSupport = new AdminSupportHandler();
frontLineSupport.setNextHandler(technicalSupport);
technicalSupport.setNextHandler(adminSupport);
// Client sends requests
console.log("Issue: Basic");
frontLineSupport.handleRequest("Basic");
console.log("\nIssue: Technical");
frontLineSupport.handleRequest("Technical");
console.log("\nIssue: Admin");
frontLineSupport.handleRequest("Admin");
console.log("\nIssue: Unknown");
frontLineSupport.handleRequest("Unknown");
// Handler Interface
class SupportHandler {
setNextHandler(nextHandler) {
throw new Error("Method not implemented.");
}
handleRequest(issue) {
throw new Error("Method not implemented.");
}
}
// Concrete Handler 1 - FrontLine Support
class FrontLineSupportHandler extends SupportHandler {
constructor() {
super();
this.nextHandler = null;
}
setNextHandler(nextHandler) {
this.nextHandler = nextHandler;
}
handleRequest(issue) {
if (issue === "Basic") {
console.log("FrontLine Support: Handling basic issue.");
} else {
console.log("FrontLine Support: Forwarding to next level.");
if (this.nextHandler) {
this.nextHandler.handleRequest(issue);
}
}
}
}
// Concrete Handler 2 - Technical Support
class TechnicalSupportHandler extends SupportHandler {
constructor() {
super();
this.nextHandler = null;
}
setNextHandler(nextHandler) {
this.nextHandler = nextHandler;
}
handleRequest(issue) {
if (issue === "Technical") {
console.log("Technical Support: Handling technical issue.");
} else {
console.log("Technical Support: Forwarding to next level.");
if (this.nextHandler) {
this.nextHandler.handleRequest(issue);
}
}
}
}
// Concrete Handler 3 - Admin Support
class AdminSupportHandler extends SupportHandler {
constructor() {
super();
this.nextHandler = null;
}
setNextHandler(nextHandler) {
this.nextHandler = nextHandler;
}
handleRequest(issue) {
if (issue === "Admin") {
console.log("Admin Support: Handling admin issue.");
} else {
console.log("Admin Support: No further handler, unable to resolve.");
}
}
}
// Client
const frontLineSupport = new FrontLineSupportHandler();
const technicalSupport = new TechnicalSupportHandler();
const adminSupport = new AdminSupportHandler();
frontLineSupport.setNextHandler(technicalSupport);
technicalSupport.setNextHandler(adminSupport);
// Client sends requests
console.log("Issue: Basic");
frontLineSupport.handleRequest("Basic");
console.log("\nIssue: Technical");
frontLineSupport.handleRequest("Technical");
console.log("\nIssue: Admin");
frontLineSupport.handleRequest("Admin");
console.log("\nIssue: Unknown");
frontLineSupport.handleRequest("Unknown");
Output
Issue: Basic
FrontLine Support: Handling basic issue.
Issue: Technical
FrontLine Support: Forwarding to next level.
Technical Support: Handling technical issue.
Issue: Admin
FrontLine Support: Forwarding to next level.
Technical Support: Forwarding to next level.
Admin Support: Handling admin issue.
Issue: Unknown
FrontLine Support: Forwarding to next level.
Technical Support: Forwarding to next level.
Admin Support: No further handler, unable to resolve.
Explanation
- The program demonstrates the Chain of Responsibility pattern by setting up a sequence of support handlers for different issue types.
- Each handler processes specific issues or forwards them to the next handler in the chain.
- For issues it cannot handle, the last handler reports that it cannot resolve the issue.
Application of Chain of Responsibility Design Pattern
1. Use the Chain of Responsibility design when your program is expected to handle many types of requests in different ways, but the specific types of requests and their sequencing are unknown ahead of time.
- The pattern allows you to chain numerous handlers together and "ask" each handler whether it can process a given request.
- This gives all handlers an opportunity to process the request.
2. Use the pattern when it is critical to execute many handlers in a specific order.
- Because you can join the handlers in the chain in any sequence, all requests will be routed exactly how you intended.
3. Use the CoR pattern when the set of handlers and their order are expected to change during runtime.
- If you include setters for a reference field in the handler classes, you will be able to insert, remove, or reorder handlers dynamically.
Why Do We Need the Chain of Responsibility Pattern?
1. Handling Requests with Different Levels of Responsibility
- Scenario: You need to process requests that may require different levels of handling, such as customer service issues that range from simple to complex.
- Need: The Chain of Responsibility pattern allows each handler to process a request or pass it along the chain, providing a flexible way to manage different levels of responsibility without tightly coupling the request sender to specific handlers.
2. Adhering to the Single Responsibility Principle
- Scenario: You want to keep the logic of handling different types of requests separated.
- Need: The Chain of Responsibility pattern helps adhere to the single responsibility principle by allowing each handler to focus on a specific type of request while delegating others to the next handler in the chain.
3. Decoupling Request Senders and Handlers
- Scenario: You have a system where different types of requests need to be handled, but you want to avoid hardcoding which handler should process each request.
- Need: The Chain of Responsibility pattern decouples the sender of the request from the actual handler, allowing for more flexible and maintainable code by changing the chain configuration or adding new handlers without modifying existing code.
4. Flexibility in Adding or Removing Handlers
- Scenario: You need the ability to add or remove request handlers dynamically.
- Need: The Chain of Responsibility pattern makes it easy to modify the chain of handlers, providing flexibility to adjust the handling process as requirements change without affecting other parts of the system.
5. Handling Multiple Types of Requests
- Scenario: You have a variety of request types, each requiring different handling logic.
- Need: The Chain of Responsibility pattern allows for multiple types of requests to be handled by different handlers in a chain, making it easy to process different requests in a structured manner.
6. Streamlining Request Processing
- Scenario: You want to ensure that each request is processed in a systematic way without requiring extensive condition checks in the code.
- Need: The Chain of Responsibility pattern streamlines request processing by passing the request through a chain of handlers, each of which decides whether to handle the request or pass it along, reducing complex condition checks.
When Not to Use the Chain of Responsibility Pattern?
1. When Request Handling is Simple and Direct
- Scenario: If all requests can be handled by a single handler or a straightforward logic.
- Reason: The Chain of Responsibility pattern introduces complexity that might be unnecessary if the request handling is simple and doesn’t require multiple levels of processing.
2. When Performance is Critical
- Scenario: In performance-sensitive applications where minimizing processing time is crucial.
- Reason: The Chain of Responsibility pattern can introduce overhead due to multiple handler calls and may not be suitable for performance-critical applications where every millisecond counts.
3. When the Chain is Fixed and Known
- Scenario: If the order and type of request handling are known and fixed in advance.
- Reason: If the chain of handlers is predetermined and won’t change, the pattern might add unnecessary abstraction, making the design more complex without significant benefits.
4. When Request Handling Logic is Highly Interdependent
- Scenario: If handlers have complex dependencies or need to interact closely with one another.
- Reason: The Chain of Responsibility pattern is best for loosely coupled handlers; highly interdependent logic might be better served by a different pattern where handlers can directly interact.
5. When Memory Usage is a Concern
- Scenario: In systems with strict memory constraints.
- Reason: The Chain of Responsibility pattern can increase memory usage due to maintaining multiple handler objects, which might not be ideal in memory-constrained environments.
Relationship with Other Patterns
- Mediator Pattern: The Chain of Responsibility pattern differs from the Mediator pattern. While the Chain of Responsibility manages a sequence of handlers that process a request, the Mediator pattern centralizes communication between objects, reducing direct dependencies and interactions among them.
- Adapter Pattern: The Chain of Responsibility pattern is not the same as the Adapter pattern. The Chain of Responsibility deals with processing requests through a chain of handlers, whereas the Adapter pattern changes one interface to make it compatible with another interface.
- Composite Pattern: It is common to use the Chain of Responsibility pattern with the Composite pattern. When dealing with complex structures like trees or hierarchies, the Chain of Responsibility can help manage different levels of processing within the composite structure, allowing for flexible handling of various requests.
- Factory Method Pattern: The Chain of Responsibility pattern works well with the Factory Method pattern. The Factory Method can be used to create different handlers for the chain, allowing for the dynamic configuration of handling logic based on specific needs or conditions.
- Singleton Pattern: The Chain of Responsibility pattern can be used in conjunction with the Singleton pattern when you need a single, consistent chain of handlers throughout the application. The Singleton pattern ensures that only one instance of the chain exists, maintaining control and consistency in request processing.