21
NovUnderstanding the Composite Design Pattern
Composite Design Pattern
Composite Design Pattern is an important concept in software design. It allows individual objects and compositions of objects to be treated uniformly, enabling users to interact with complex tree structures as though they were dealing with single objects. This pattern simplifies the management of hierarchical structures and promotes flexibility in handling related objects.
In this design pattern tutorial, we will explore the Composite Design Pattern, including "What is the Composite Design Pattern?" and "When should I use the Composite Design Pattern?" We'll also look at examples of the Composite Design Pattern in action. So, let's begin with "What is the Composite Design Pattern?"
What is the Composite Design Pattern?
- The Composite Design Pattern is a structural design pattern that allows you to treat individual objects and compositions of objects uniformly.
- It simplifies the design of complex tree-like structures by organizing objects into a hierarchy where both individual and composite objects are treated the same way.
- Clients interact with these objects through a common interface, promoting flexibility, scalability, and easier management of hierarchies.
- This pattern reduces complexity in handling related objects and supports recursive composition.
Why do we need a Composite Design Pattern?
1. Uniform Interface
- The Composite Pattern ensures a consistent interface for both individual objects and compositions.
- This consistency simplifies client code, making it more readable and eliminating the need for conditional statements to distinguish between different sorts of objects.
- Other design patterns may not provide the same level of consistency in managing individual and composite items.
2. Hierarchical structures
- The Composite Pattern is primarily concerned with hierarchical systems in which items can be made of other elements.
- Other patterns address various types of problems, but the Composite Pattern focuses on scenarios involving tree-like structures.
3. Flexibility and Scalability
- The Composite Pattern enables the dynamic composition of items, resulting in the production of complex structures.
- It encourages flexibility and scalability, making it easy to add and delete parts from the hierarchy without changing the client code.
4. Common Operations
- The Composite Pattern eliminates code duplication by defining common operations at the component level, promoting a uniform approach to dealing with both leaf and composite objects.
- Other design patterns may not give as much support for typical operations in hierarchical systems.
5. Client Simplification
- The Composite Pattern makes client code simpler by offering a uniform mechanism to interface with individual and composite objects.
- This simplification is especially useful when dealing with complex structures like graphical user interfaces and organizational hierarchies.
Real-world Illustration of Composite Design Pattern
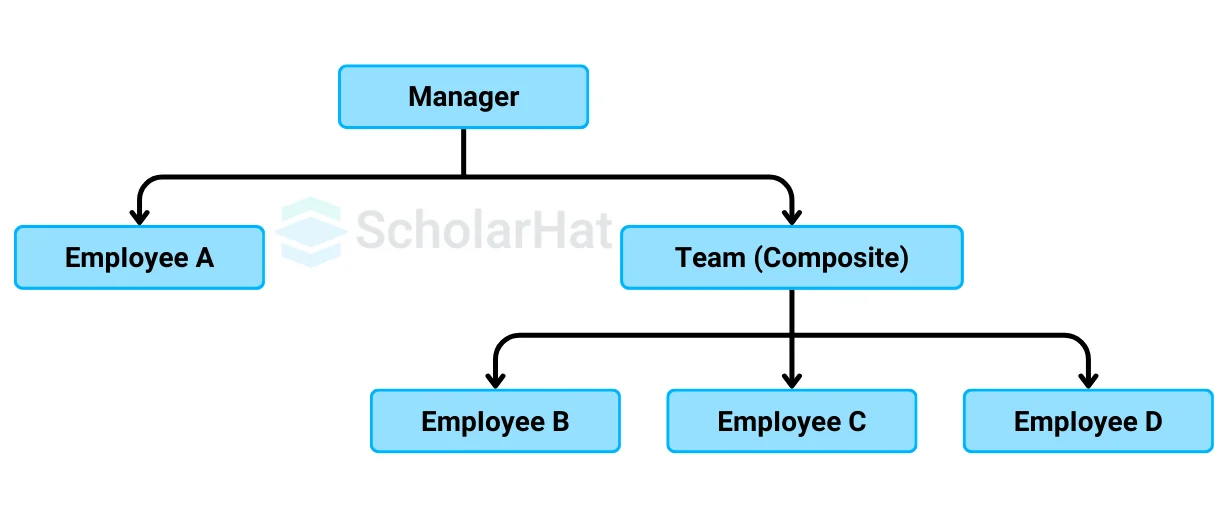
- Imagine an office where you have both individual employees and teams of employees.
- An individual employee can perform tasks on their own, and a team, composed of multiple employees, can also complete tasks together.
- When assigning work, you don’t have to treat them differently.
- You can assign tasks to a single employee or an entire team in the same way.
- This setup works like the Composite Pattern, where both individual and group objects are treated uniformly, simplifying task management without needing to differentiate between individual and composite workers.
Composite Design Pattern Basic Structure and Implementation
The structure for the implementation of the Composite Design Pattern is given below:
The classes, interfaces, and objects in the above structure are as follows:
1. Component
- This is an interface or abstract class that defines the common operations that both leaf objects (individual objects) and composite objects (groups of objects) must implement.
- It declares methods that allow the client to treat both individual objects and composites uniformly.
- By defining this contract, the client can interact with both single objects and collections of objects without knowing if it’s dealing with a single object or a group.
2. Leaf
- The Leaf class implements the Component interface and represents the individual object in the composition.
- It performs the core functionality that the client expects from a single object.
- For example, in a file system, a File class could be a leaf that performs actions like opening or reading a file.
- Leaf objects don’t contain any child objects and provide a concrete implementation of the operations defined in the Component interface.
3. Composite
- The Composite class also implements the Component interface but can hold child components, either leaves or other composite objects.
- It manages its child components and forwards requests to them, allowing recursive composition.
- For example, a Folder class can act as a composite, containing both File objects and other Folder objects.
- The composite performs operations by calling the same operation on its children, which could be either individual leaves or other composites.
Real-Life Example
1. Subsystems (Files and Folders in a Computer System)
- In a computer system, files and folders are organized hierarchically.
- A folder can contain multiple files and subfolders, and each of these subfolders can contain more files or folders.
2. Composite (File and Folder Structure)
- The folder structure itself acts as a composite.
- You can treat a single file or an entire folder the same way, allowing for uniform operations across both.
3. Operation Flow
- Unified Operation: When you want to delete a folder, the system deletes both the folder and all the files and subfolders inside it in one action.
- Recursive Handling: The folder (composite) manages all its contents. For example, if a folder contains multiple files and subfolders, the operation is passed down to each individual file or subfolder.
- Individual File Handling: Each file within the folder still behaves independently. If you open or modify a file, the system treats it as an individual entity.
4. How the Composite Helps
- Unified Structure: It allows you to treat a collection of files and folders as one unit. Whether you are moving, deleting, or renaming, the operation applies to the entire group.
- Simplified Management: By managing files and folders through a composite structure, you don’t have to interact with individual files separately. This reduces complexity, especially in large directories.
- Consistent Behavior: Whether you are working with a single file or an entire folder structure, the operations remain consistent, making it easier to handle large file systems.
Example
using System;
using System.Collections.Generic;
// Component Interface
public interface IFileSystemComponent {
void ShowDetails();
void Delete();
}
// Leaf class: File
public class File : IFileSystemComponent {
private string _name;
public File(string name) {
_name = name;
}
public void ShowDetails() {
Console.WriteLine($"File: {_name}");
}
public void Delete() {
Console.WriteLine($"Deleting file: {_name}");
}
}
// Composite class: Folder
public class Folder : IFileSystemComponent {
private string _name;
private List<IFileSystemComponent> _components = new List<IFileSystemComponent>();
public Folder(string name) {
_name = name;
}
public void AddComponent(IFileSystemComponent component) {
_components.Add(component);
}
public void RemoveComponent(IFileSystemComponent component) {
_components.Remove(component);
}
public void ShowDetails() {
Console.WriteLine($"Folder: {_name}");
foreach (var component in _components) {
component.ShowDetails();
}
}
public void Delete() {
Console.WriteLine($"Deleting folder: {_name}");
foreach (var component in _components) {
component.Delete();
}
}
}
// Client code
public class Program {
public static void Main() {
IFileSystemComponent file1 = new File("File1.txt");
IFileSystemComponent file2 = new File("File2.txt");
IFileSystemComponent file3 = new File("File3.txt");
Folder folder1 = new Folder("Folder1");
Folder folder2 = new Folder("Folder2");
folder1.AddComponent(file1);
folder1.AddComponent(file2);
folder2.AddComponent(folder1);
folder2.AddComponent(file3);
Console.WriteLine("Folder structure:");
folder2.ShowDetails();
Console.WriteLine("\nDeleting folder2:");
folder2.Delete();
}
}
import java.util.ArrayList;
import java.util.List;
// Component Interface
interface FileSystemComponent {
void showDetails();
void delete();
}
// Leaf class: File
class File implements FileSystemComponent {
private String name;
public File(String name) {
this.name = name;
}
@Override
public void showDetails() {
System.out.println("File: " + name);
}
@Override
public void delete() {
System.out.println("Deleting file: " + name);
}
}
// Composite class: Folder
class Folder implements FileSystemComponent {
private String name;
private List<FileSystemComponent> components = new ArrayList<>();
public Folder(String name) {
this.name = name;
}
public void addComponent(FileSystemComponent component) {
components.add(component);
}
public void removeComponent(FileSystemComponent component) {
components.remove(component);
}
@Override
public void showDetails() {
System.out.println("Folder: " + name);
for (FileSystemComponent component : components) {
component.showDetails();
}
}
@Override
public void delete() {
System.out.println("Deleting folder: " + name);
for (FileSystemComponent component : components) {
component.delete();
}
}
}
// Client code
public class Main {
public static void main(String[] args) {
FileSystemComponent file1 = new File("File1.txt");
FileSystemComponent file2 = new File("File2.txt");
FileSystemComponent file3 = new File("File3.txt");
Folder folder1 = new Folder("Folder1");
Folder folder2 = new Folder("Folder2");
folder1.addComponent(file1);
folder1.addComponent(file2);
folder2.addComponent(folder1);
folder2.addComponent(file3);
System.out.println("Folder structure:");
folder2.showDetails();
System.out.println("\nDeleting folder2:");
folder2.delete();
}
}
from typing import List
# Component Interface
class FileSystemComponent:
def show_details(self):
pass
def delete(self):
pass
# Leaf class: File
class File(FileSystemComponent):
def __init__(self, name: str):
self.name = name
def show_details(self):
print(f"File: {self.name}")
def delete(self):
print(f"Deleting file: {self.name}")
# Composite class: Folder
class Folder(FileSystemComponent):
def __init__(self, name: str):
self.name = name
self.components: List[FileSystemComponent] = []
def add_component(self, component: FileSystemComponent):
self.components.append(component)
def remove_component(self, component: FileSystemComponent):
self.components.remove(component)
def show_details(self):
print(f"Folder: {self.name}")
for component in self.components:
component.show_details()
def delete(self):
print(f"Deleting folder: {self.name}")
for component in self.components:
component.delete()
# Client code
file1 = File("File1.txt")
file2 = File("File2.txt")
file3 = File("File3.txt")
folder1 = Folder("Folder1")
folder2 = Folder("Folder2")
folder1.add_component(file1)
folder1.add_component(file2)
folder2.add_component(folder1)
folder2.add_component(file3)
print("Folder structure:")
folder2.show_details()
print("\nDeleting folder2:")
folder2.delete()
interface FileSystemComponent {
showDetails(): void;
delete(): void;
}
// Leaf class: File
class File implements FileSystemComponent {
private name: string;
constructor(name: string) {
this.name = name;
}
showDetails(): void {
console.log(`File: ${this.name}`);
}
delete(): void {
console.log(`Deleting file: ${this.name}`);
}
}
// Composite class: Folder
class Folder implements FileSystemComponent {
private name: string;
private components: FileSystemComponent[] = [];
constructor(name: string) {
this.name = name;
}
addComponent(component: FileSystemComponent): void {
this.components.push(component);
}
removeComponent(component: FileSystemComponent): void {
this.components = this.components.filter(c => c !== component);
}
showDetails(): void {
console.log(`Folder: ${this.name}`);
this.components.forEach(component => component.showDetails());
}
delete(): void {
console.log(`Deleting folder: ${this.name}`);
this.components.forEach(component => component.delete());
}
}
// Client code
const file1 = new File("File1.txt");
const file2 = new File("File2.txt");
const file3 = new File("File3.txt");
const folder1 = new Folder("Folder1");
const folder2 = new Folder("Folder2");
folder1.addComponent(file1);
folder1.addComponent(file2);
folder2.addComponent(folder1);
folder2.addComponent(file3);
console.log("Folder structure:");
folder2.showDetails();
console.log("\nDeleting folder2:");
folder2.delete();
class File {
constructor(name) {
this.name = name;
}
showDetails() {
console.log(`File: ${this.name}`);
}
delete() {
console.log(`Deleting file: ${this.name}`);
}
}
class Folder {
constructor(name) {
this.name = name;
this.components = [];
}
addComponent(component) {
this.components.push(component);
}
removeComponent(component) {
this.components = this.components.filter(c => c !== component);
}
showDetails() {
console.log(`Folder: ${this.name}`);
this.components.forEach(component => component.showDetails());
}
delete() {
console.log(`Deleting folder: ${this.name}`);
this.components.forEach(component => component.delete());
}
}
// Client code
const file1 = new File("File1.txt");
const file2 = new File("File2.txt");
const file3 = new File("File3.txt");
const folder1 = new Folder("Folder1");
const folder2 = new Folder("Folder2");
folder1.addComponent(file1);
folder1.addComponent(file2);
folder2.addComponent(folder1);
folder2.addComponent(file3);
console.log("Folder structure:");
folder2.showDetails();
console.log("\nDeleting folder2:");
folder2.delete();
Output
Folder structure:
Folder: Folder2
Folder: Folder1
File: File1.txt
File: File2.txt
File: File3.txt
Deleting folder2:
Deleting folder: Folder2
Deleting folder: Folder1
Deleting file: File1.txt
Deleting file: File2.txt
Deleting file: File3.txt
Explanation
- This program demonstrates the Composite Design Pattern, where objects are composed into tree-like structures to represent part-whole hierarchies.
- The FileSystemComponent interface represents both files and folders.
- The file is a leaf class, and the Folder is a composite class that can hold multiple FileSystemComponent objects, including other folders or files.
- The client can interact with both individual files and folders uniformly, and the folder structure is displayed recursively using the showDetails method.
Applications of Composite Design Pattern
1. Use the Composite pattern when you need to implement a tree-like object structure.
- The Composite pattern gives you two fundamental element kinds with a shared interface: simple leaves and sophisticated containers.
- A container can consist of both leaves and other containers. This allows you to create a nested recursive object structure that resembles a tree.
2. Use the pattern when you want the client code to treat both simple and complicated components consistently.
- All elements defined by the Composite pattern have a common interface.
- Using this interface, the client is not concerned about the concrete class of the objects with which it interacts.
When to use the Composite Design Pattern
- It is used when you need to represent part-whole hierarchies, where individual objects and compositions of objects should be treated uniformly.
- It is ideal when building tree-like structures, such as file systems, UI components, or organizational hierarchies.
- When you need to perform operations on both individual and composite objects in the same way without checking their types.
- It is useful when managing complex structures where components may be grouped into larger components, allowing simplified management and interaction.
When not to use the Composite Design Pattern
- It is unnecessary to use the Composite pattern if the structure is simple and doesn’t involve complex hierarchies or groupings.
- In cases where individual components have significantly different behaviors, forcing them into a uniform structure can add unnecessary complexity.
- It might violate the Single Responsibility Principle if the composite structure tries to manage too many concerns, such as both component creation and behavior.
- If there is no need to treat individual and composite objects uniformly, using the pattern could result in over-engineering.
Relationship with Other Patterns
- Decorator Pattern: The Composite pattern is similar to the Decorator pattern in that both deal with tree structures, but the Composite focuses on whole-part hierarchies, while the Decorator adds additional behavior to objects.
- Iterator Pattern: It can be used with the Composite pattern to traverse through components of a composite object, allowing for seamless iteration over a hierarchical structure.
- Flyweight Pattern: The Composite pattern can work with the Flyweight pattern to efficiently manage large hierarchies where shared components are used, reducing memory overhead.
- Builder Pattern: The Composite pattern can complement the Builder pattern when constructing complex tree structures, allowing for step-by-step creation of a composite object.