Different Types of Software Design Principles
Software Design Principles
Software Design Principles are recommendations that help you write code that is clear, manageable, and scalable. These principles give a foundation for developing software systems that are both robust and responsive to change.
In this Design patterns tutorial, we'll look at the fundamental Software Design Principles, including their definitions, applications, different types of design patterns. By following these guidelines, you may enhance code quality and make your projects more manageable. Let's start with an overview of "Software Design Principles."
What are Software design principles?
- Software design principles are essential recommendations that assist developers in writing effective and maintainable code.
- They incorporate ideas such as SOLID (Single Responsibility, Open/Closed, Liskov Substitution, Interface Separation, and Dependency Inversion) to ensure that code is modular, adaptable, and easy to understand.
- Adhering to these standards increases code quality and makes change management more effortless.
Read More: |
Different Types of Design Patterns |
Gang of Four Design Patterns |
Why Are Software Design Principles Important?
Software design principles are important because they guide developers in creating high-quality software that is efficient, maintainable, and scalable:
- Improves Code Quality: Following principles ensures the software is well-structured, making it easier to read, understand, and debug.
- Enhances Maintainability: Good design principles reduce complexity and improve code organization, making future updates and maintenance easier.
- Promotes Reusability: Principles like DRY (Don’t Repeat Yourself) encourage reusing code, saving time, and reducing redundancy.
- Increases Flexibility: Adopting principles like SOLID allows the software to adapt to changes with minimal impact, improving its lifespan.
- Encourages Collaboration: Well-designed software is easier for teams to work on, as it is modular and follows standard patterns.
- Prevents Errors and Bugs: Consistent design reduces the likelihood of introducing errors, leading to fewer bugs and a more stable system.
Different Types of Software Design Principles
Software design principles are a set of criteria that assist developers in creating good system designs. The most crucial premise is the SOLID one. The many kinds of software design principles are as follows:
1. SOLID
It is a combination of five basic designing principles:
- Single Responsibility Principle (SRP)
- Open/Closed Principle (OCP)
- Liscov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- 5. Dependency Inversion Principle (DIP)
1. Single Responsibility Principle (SRP)
- This principle states that there should never be more than one reason for a class to change.
- This means that you should design your classes in such a way that each class should have a single purpose.
2. Open/Closed Principle (OCP)
- This principle states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification.
- The "closed" part of the rule states that once a module has been developed and tested, the code should only be changed to correct bugs. The "open" part says that you should be able to extend existing code to introduce new functionality.
3. Liscov Substitution Principle (LSP)
- Subclasses should be able to replace their parent class without breaking the program.
- Subclasses must behave in a way that doesn’t change the expected results from the parent class.
4. Interface Segregation Principle (ISP)
- This principle states that Clients should not be forced to depend upon interfaces that they don’t use.
- This means minimizing the number of members in the interface visible to the dependent class.
5. Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that:
- The main parts of your code shouldn’t rely directly on the details; they should depend on general concepts.
- Details should follow these general concepts, not the other way around.
- This makes your code more flexible and easier to update without breaking other parts.
Read More: |
Singleton Design Pattern |
Prototype Design Pattern |
Understanding MVC, MVP, and MVVM Design Patterns |
2. DRY (Don’t Repeat Yourself)
- DRY (Don't Repeat Yourself) is a fundamental software design approach that aims to reduce code redundancy.
- It underlines the importance of having a single, unambiguous representation of all information or logic within a system.
- The DRY concept helps to eliminate errors by reducing code duplication and consolidating comparable code into reusable functions or components.
- It also simplifies maintenance and makes the codebase more cohesive and manageable.
3. KISS (Keep it simple, Stupid!)
- Keep It Simple, Stupid (KISS) is a software design guideline that encourages simplicity in code and design.
- It highlights the importance of keeping systems as simple as feasible while avoiding unnecessary complexity and over-engineering.
- The KISS principle encourages improved readability, easier maintenance, and a lower risk of errors, resulting in more resilient and understandable software.
4. YAGNI (You aren't going to need it)
- You Aren't Gonna Need It (YAGNI) is a software design theory that recommends not implementing capability until it is genuinely needed.
- It highlights that developers should avoid adding features or capabilities based on fictional demands or future requirements.
- By following YAGNI, developers can simplify their codebase, prevent over-engineering, and focus on solving existing problems, resulting in more manageable and efficient code.
5. Design by Contract
- Specify clear preconditions, postconditions, and invariants for components.
- Components must fulfill their contract obligations as specified.
- Both callers and implementers must meet their contractual obligations.
- Detect and handle contract violations to ensure reliable behavior.
6. Principle of Least Astonishment
- Ensure that software behaves in a way that users or developers expect, minimizing surprises or confusion.
- Follow established conventions and patterns to make the system predictable and easy to understand.
- Provide clear explanations and documentation to help users understand how the system should work and what to expect.
- Design features and interfaces to align with standard practices and user experiences to avoid unexpected behavior.
7. Composition Over Inheritance
- Build functionality by composing objects from other objects rather than using a rigid inheritance hierarchy. This allows for more flexible and reusable code.
- Reduces the problems associated with deep inheritance chains, such as tight coupling and inflexibility, by favoring composition.
- It simplifies changes and extensions by allowing objects to be composed in different ways rather than modifying or extending existing classes.
8. Fail Fast
- Identify and address errors as soon as they occur rather than allowing issues to propagate through the system.
- Validate inputs and conditions at the earliest possible point to prevent invalid data from causing problems later.
- By catching errors early, you simplify the debugging process and make it easier to locate and fix issues.
- Ensure the system behaves reliably by handling errors promptly, preventing unexpected behaviors, and improving overall stability.
9. Law of Demeter (LoD)
- Limit the knowledge that a class has about the internal details of other classes to reduce dependencies and promote encapsulation.
- A class should only interact with its direct collaborators and avoid reaching into the internals of other objects.
- Prevent method calls from being chained together (e.g., objectA.getB().getC().doSomething()) to reduce coupling and improve code maintainability.
- Design classes to have clear and simple interfaces, making the system easier to understand and less prone to errors.
Read More: OOPs Concepts in Java: Encapsulation, Abstraction, Inheritance, Polymorphism |
10. Separation of Concerns
- Break down a system into distinct sections, each handling a specific aspect of functionality. This helps manage complexity by keeping related tasks together.
- Ensure that each section or module works independently and does not overlap with others. Changes in one part should not affect others.
- Write code so that each part of the system does one job well, making it easier to understand, test, and maintain.
- By keeping different concerns separate, you can make updates or fixes to one part without worrying about unintended effects on other parts.
Advantages of Design Principles
Here are the advantages of applying design principles:
- Easier Maintenance: Simplifies code updates and fixes.
- Greater Flexibility: Facilitates changes and updates with minimal impact.
- Increased Reusability: Promotes reuse of components across projects.
- Enhanced Reliability: Helps catch and fix issues early.
- Simplified Testing: This makes it easier to write and run tests.
- Better Collaboration: Provides a clear and consistent approach for teams.
Read More: |
Most Frequently Asked Software Architect Interview Questions and Answers |
Top 50 Java Design Patterns Interview Questions and Answers |
Summary
In conclusion, we have explored design principles that guide the creation of robust and maintainable software. These principles, such as SRP, OCP, and LSP, focus on clear responsibilities and modularity, making code easier to understand, modify, and extend. Applying these principles improves code quality, scalability, and team collaboration. Also, consider our Software Architecture and Design Certification Training for a better understanding of other Java concepts.
FAQs
The design principle of Abstraction allows software to run on different platforms by defining general interfaces and hiding implementation details, enabling platform-independent code.
Take our Designpatterns skill challenge to evaluate yourself!
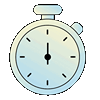
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.