09
NovIterator Design Pattern
Understanding the Iterator Design Pattern
Understanding the Iterator Design Pattern is essential for providing a uniform way to access elements in a collection without exposing the underlying representation. It allows the traversal of a collection’s elements sequentially without needing to know how the collection is structured, thus promoting clean, organized code.
In this design pattern tutorial, we will explore the Iterator Design Pattern including, "What is the Iterator Design Pattern?" We’ll also discuss "When should I use the Iterator Design Pattern?" and provide examples to illustrate its use. Let’s begin by addressing the question, "What is the Iterator Design Pattern?"
What is the Iterator Design Pattern?
- The Iterator Design Pattern is a behavioral design pattern that allows you to access pieces of a collection carefully without exposing the underlying implementation.
- It defines a standard method for traversing a collection's elements, regardless of how the collection is organized.
- This approach separates the traversal logic from the collection by utilizing an iterator object to keep track of the current position within the collection.
- The iterator pattern ensures that the client just needs to understand how to access elements from the collection, not its internal structure.
- It encourages the use of iterator objects to access elements rather than directly modifying the collection, which improves encapsulation.
Why do we need the Iterator Design Pattern?
Simplified Traversal
- Provides a standard way to iterate over different types of collections (arrays, lists, trees) using the same interface, without exposing the internal details of the collection.
- Clients do not need to know the specifics of how the collection is structured to traverse through it.
Decouples Code from Collection Implementations
- The Iterator Design Pattern decouples client code from the specific implementation of collections.
- Whether the collection is a simple array or a complex tree, the client interacts with it using the iterator.
- Abstracting the traversal mechanism allows collections to be easily modified or replaced without affecting the code that uses them.
Code Reusability and Flexibility
- Encourages reuse by allowing the same traversal logic for different types of collections.
- The pattern follows the open/closed principle by letting you extend collections with new iterator functionality without modifying the existing collection code.
Flexibility in Iteration
- Allows multiple ways to iterate through a collection. For example, you can implement different iterators for traversing forward, backward, or even filtering specific elements.
- Enhances flexibility by supporting multiple iterators for the same collection simultaneously.
Consistent Interface
- Provides a consistent interface for traversing collections, simplifying client code, and reducing complexity.
- Clients interact with the iterator through a uniform API without worrying about the collection’s structure or traversal logic.
Supports Complex Data Structures
- It makes traversing through complex data structures like trees or graphs easier by hiding the internal details and providing a simple way to access each element.
Real-world illustration of Iterator Design Pattern
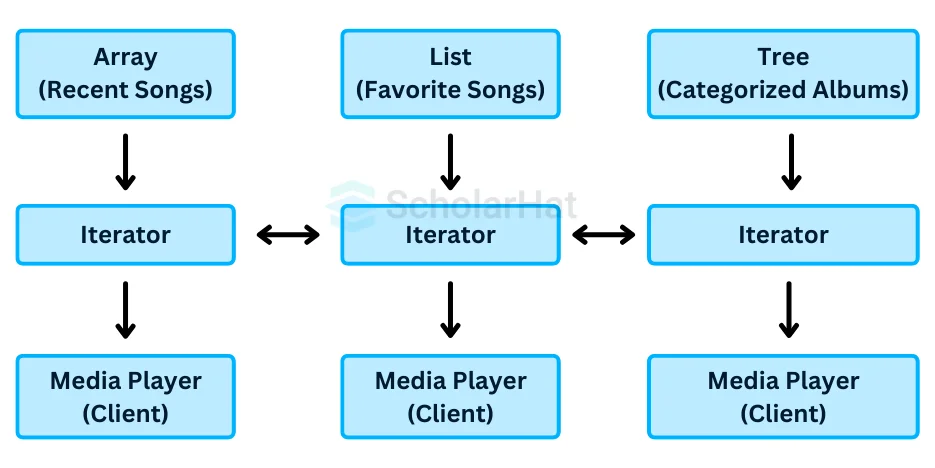
- Imagine a media application that needs to process different types of playlists stored in various formats, such as arrays (for recently played songs), lists (for favorite songs), and trees (for categorized albums).
- The media application expects to access songs sequentially through a specific interface, but each playlist format uses a completely different internal structure.
- An iterator can bridge the gap between the media application and the diverse playlist formats by providing a consistent way to traverse through each collection, enabling seamless access to the songs without modifying the media application or the playlist structures.
Iterator Design Pattern Basic Structure and Implementation
The structure for the implementation of the Iterator Design Pattern includes the following key components:
Iterator Interface
- Defines the methods required for traversing through a collection.
- This interface specifies operations like hasNext() to check if more elements are available and next() to retrieve the next element in the collection.
- For example, in a media application, the SongIterator interface might have methods like hasNextSong() and nextSong() for traversing a playlist.
Concrete Iterator
- Implements the Iterator interface and provides the actual traversal logic for a specific collection.
- This class keeps track of the current position in the collection and defines how to navigate through it.
- For instance, a ListIterator might iterate over a list of favorite songs, providing the logic to move from one song to the next.
Collection (or Aggregate) Interface
- Defines the method to create an iterator for the collection.
- This interface specifies the createIterator() method, which returns an instance of the iterator that can be used to traverse the collection.
- In a media app, the Playlist interface might include a createSongIterator() method to provide an iterator for accessing songs.
Concrete Collection (or Aggregate)
- Implements the collection interface and returns a concrete iterator for its elements.
- This class stores the collection of elements and creates an iterator specific to its internal structure.
- For example, a SongPlaylist class might store songs in an array or list and return a SongIterator to allow traversal through the songs.
Real-Life Example
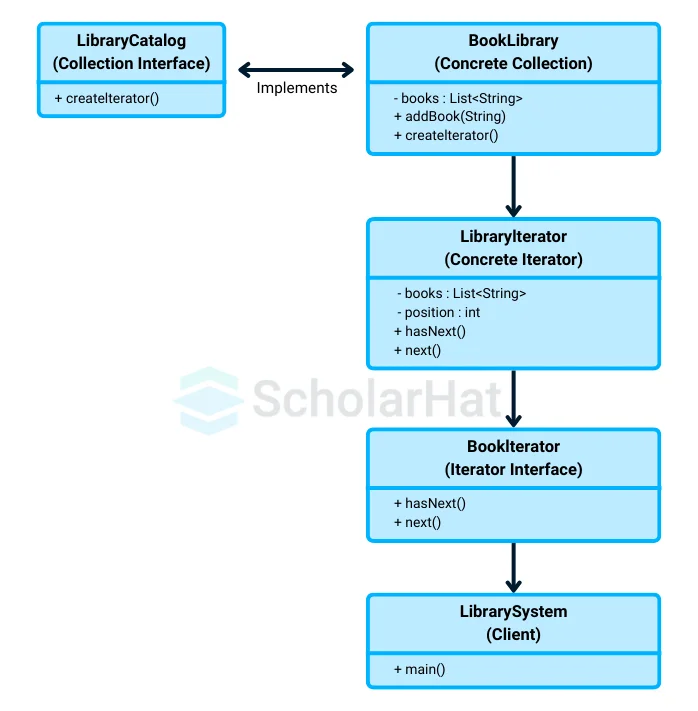
Subsystems (Library Collections and Access Methods)
- Books: These are the items in the library, stored in various formats and locations. They can be organized in different ways, such as by genre, author, or publication date.
- Library Collections: Different sections of the library may use distinct organizational systems. For example, one section might use shelves while another uses digital catalogs.
Iterator (Library Catalog)
- Library Catalog: It is used to access and browse through the books in different sections. It provides a uniform way to look through the collection, regardless of how the books are stored or organized.
Operation Flow
- Unified Access: When you use the library catalog, it allows you to browse through books in various sections of the library. You can find books by title, author, or genre without worrying about the organizational method.
- Access Management: The library catalog manages the process of finding and retrieving books, regardless of their physical or digital storage method.
- Consistent Browsing: Whether you are looking through physical shelves or digital lists, the library catalog ensures a consistent and straightforward way to access the books.
How the Iterator Helps
- Unified Structure: It provides a single interface to access different types of book collections, making it easy to find and manage books across various sections of the library.
- Simplified Management: Using the library catalog means you do not need to adapt to different organizational methods or storage systems. It simplifies the browsing experience.
- Consistent Access: Whether dealing with physical or digital books, the library catalog ensures a smooth and consistent browsing experience, regardless of how the books are organized.
Example
using System;
using System.Collections;
using System.Collections.Generic;
// Book class to store details of each book
public class Book
{
public string Title { get; }
public Book(string title)
{
Title = title;
}
}
// Iterator for the library catalog
public class LibraryCatalog : IEnumerable<Book>
{
private List<Book> _books = new List<Book>();
// Method to add books to the catalog
public void AddBook(Book book)
{
_books.Add(book);
}
public IEnumerator<Book> GetEnumerator()
{
return _books.GetEnumerator();
}
IEnumerator IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
}
// Main class to demonstrate the library catalog using Iterator
public class Program
{
public static void Main(string[] args)
{
// Create a library catalog
var catalog = new LibraryCatalog();
// Add books to the catalog
catalog.AddBook(new Book("The Great Gatsby"));
catalog.AddBook(new Book("1984"));
catalog.AddBook(new Book("To Kill a Mockingbird"));
// Display the library catalog
Console.WriteLine("Library Catalog:");
foreach (var book in catalog)
{
Console.WriteLine(book.Title);
}
}
}
# Python Code Placeholder
# Implementing the same pattern in Python can be done using iterators
class LibraryIterator:
def __init__(self, books):
self.books = books
self.position = 0
def has_next(self):
return self.position < len(self.books)
def next(self):
book = self.books[self.position] if self.has_next() else None
self.position += 1
return book
class BookLibrary:
def __init__(self):
self.books = []
def add_book(self, book):
self.books.append(book)
def create_iterator(self):
return LibraryIterator(self.books)
# Client code
library = BookLibrary()
library.add_book("The Great Gatsby")
library.add_book("1984")
library.add_book("To Kill a Mockingbird")
iterator = library.create_iterator()
print("Library Catalog:")
while iterator.has_next():
print(iterator.next())
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
// Book class to store details of each book
class Book {
private String title;
public Book(String title) {
this.title = title;
}
public String getTitle() {
return title;
}
}
// Iterator for the library catalog
class LibraryCatalog implements Iterable<Book> {
private List<Book> books = new ArrayList<>();
// Method to add books to the catalog
public void addBook(Book book) {
books.add(book);
}
@Override
public Iterator<Book> iterator() {
return books.iterator();
}
}
// Main class to demonstrate the library catalog using Iterator
public class Main {
public static void main(String[] args) {
// Create a library catalog
LibraryCatalog catalog = new LibraryCatalog();
// Add books to the catalog
catalog.addBook(new Book("The Great Gatsby"));
catalog.addBook(new Book("1984"));
catalog.addBook(new Book("To Kill a Mockingbird"));
// Display the library catalog
System.out.println("Library Catalog:");
for (Book book : catalog) {
System.out.println(book.getTitle());
}
}
}
// TypeScript Code Placeholder
interface IBookIterator {
hasNext(): boolean;
next(): string | null;
}
class LibraryIterator implements IBookIterator {
private books: string[];
private position: number = 0;
constructor(books: string[]) {
this.books = books;
}
hasNext(): boolean {
return this.position < this.books.length;
}
next(): string | null {
return this.hasNext() ? this.books[this.position++] : null;
}
}
class BookLibrary {
private books: string[] = [];
addBook(book: string): void {
this.books.push(book);
}
createIterator(): IBookIterator {
return new LibraryIterator(this.books);
}
}
// Client code
const library = new BookLibrary();
library.addBook("The Great Gatsby");
library.addBook("1984");
library.addBook("To Kill a Mockingbird");
const iterator = library.createIterator();
console.log("Library Catalog:");
while (iterator.hasNext()) {
console.log(iterator.next());
}
// JavaScript Code Placeholder
class LibraryIterator {
constructor(books) {
this.books = books;
this.position = 0;
}
hasNext() {
return this.position < this.books.length;
}
next() {
return this.hasNext() ? this.books[this.position++] : null;
}
}
class BookLibrary {
constructor() {
this.books = [];
}
addBook(book) {
this.books.push(book);
}
createIterator() {
return new LibraryIterator(this.books);
}
}
// Client code
const library = new BookLibrary();
library.addBook("The Great Gatsby");
library.addBook("1984");
library.addBook("To Kill a Mockingbird");
const iterator = library.createIterator();
console.log("Library Catalog:");
while (iterator.hasNext()) {
console.log(iterator.next());
}
Output
Library Catalog:
The Great Gatsby
1984
To Kill a Mockingbird
Explanation
- This code demonstrates the Iterator Pattern with a library catalog system.
- The IBookIterator interface defines how to iterate through a list of books, while the LibraryIterator class implements it, allowing traversal over the book collection.
- The ILibraryCatalog interface includes a method to create an iterator, and the BookLibrary class implements this interface, adding books and returning an iterator to access them.
- The client uses this iterator to go through the library and print each book in the catalog one by one.
Applications of Iterator Design Pattern
Scenario 1. Use the Iterator pattern when your collection has a sophisticated data structure that you want to hide from clients (for convenience or security reasons).
- The iterator encapsulates the complexities of working with a complicated data structure, giving the client various straightforward ways to access the collection members.
- While this approach is incredibly handy for the client, it also safeguards the collection from careless or evil activities that the client could take if he or she worked directly with the collection.
Scenario 2. Use the pattern to reduce duplication of traversal code throughout your app.
- Non-trivial iteration algorithms typically have large amounts of code.
- When embedded in an app's business logic, it may obscure the original code's responsibility and render it less maintainable.
- Moving the traversal logic to specified iterators can help you make the application's code more streamlined and clean.
Scenario 3. Use the Iterator when you wish your code to be able to traverse various data structures or when the kinds of these structures are unknown ahead of time.
- The pattern defines a pair of generic interfaces for collections and iterators.
- Given that your code now uses these interfaces, it will continue to operate if you pass it to various types of collections and iterators that implement them.
Why Do We Need the Iterator Design Pattern?
Simplifying Traversal Logic
- Scenario: You need to traverse through complex data structures without exposing their internal details.
- Need: The Iterator pattern provides a uniform way to iterate over different types of collections, hiding the internal complexity and simplifying traversal logic.
Adhering to the Single Responsibility Principle
- Scenario: You want to separate the responsibility of data structure management from the logic of traversing it.
- Need: The iterator pattern helps adhere to the single responsibility principle by moving the iteration logic out of the collection class and keeping responsibilities distinct.
Uniform Access to Different Collections
- Scenario: You have multiple types of collections (e.g., arrays, lists, trees) but want to iterate over them in a consistent manner.
- Need: The Iterator pattern provides a common interface for iterating over different types of collections, making it easier for clients to access elements uniformly.
Supporting Multiple Traversals
- Scenario: You need to support multiple traversal algorithms, such as forward, backward, or even custom traversal of the collection.
- Need: The Iterator pattern allows you to implement different traversal strategies without altering the collection itself, enhancing flexibility.
Avoiding Code Duplication
- Scenario: You want to avoid duplicating the iteration code for each collection type.
- Need: The Iterator pattern centralizes the traversal logic, eliminating code duplication and ensuring consistency across different collections.
Simplifying Client Code
- Scenario: You want to keep client code simple while still allowing it to traverse various collections.
- Need: The Iterator pattern provides a clean interface that simplifies client code by abstracting the collection's complexity, allowing the client to focus only on iteration.
When Not to Use the Iterator Design Pattern?
When Direct Access is Sufficient
- Scenario: If the collection is simple, and direct access methods like loops are efficient and clear.
- Reason: The Iterator pattern adds abstraction, which may be unnecessary if direct access methods provide a more straightforward solution.
When You Don’t Need Multiple Traversal Strategies
- Scenario: If you don’t need to implement different ways to iterate through the collection.
- Reason: If a single traversal method suffices, introducing an iterator may add unnecessary complexity.
When Performance is a Concern
- Scenario: In performance-critical applications, minimizing object creation is important.
- Reason: Iterators create additional objects and abstractions, which can slow down performance, making it less suitable for high-performance requirements.
When the Collection is Not Meant to be Iterated
- Scenario: If the collection is small or not intended for frequent iteration.
- Reason: If there is no need for structured traversal, adding an iterator may complicate the design without providing significant benefits.
When Object Overhead is Undesirable
- Scenario: In systems where memory usage is a concern, creating iterator objects would add unnecessary overhead.
- Reason: The Iterator pattern can increase memory usage by creating additional objects, making it unsuitable in memory-constrained environments.
Relationship with Other Patterns
1. Mediator Pattern: The Iterator pattern is different from the Mediator pattern. It is about accessing elements one by one in a collection, while the Mediator pattern simplifies how objects interact with each other by centralizing their communication.
2. Adapter Pattern: The Iterator pattern is not like the Adapter pattern. It is used to move through a collection of elements while the Adapter pattern changes one interface to make it compatible with another interface.
3. Composite Pattern:It is common to use the Iterator pattern with the Composite pattern. It is because the Iterator pattern helps you go through complex structures like trees, allowing you to access each component easily, regardless of the structure.
4. Factory Method Pattern: The Iterator pattern works well with the Factory Method pattern. It is useful for creating different types of iterators for various collections, allowing you to customize how you traverse each collection.
5. Singleton Pattern: The Iterator pattern can be used with the Singleton pattern when you need only one instance of the iterator to access a collection. It is useful for maintaining control and consistency while managing resources efficiently.
Summary
The Iterator Design Pattern provides a consistent method for traversing over many sorts of collections without revealing their underlying features. It simplifies traversal logic, provides many traversal strategies, and helps in the adherence to concepts such as Single Responsibility and Open/Closed. Iterators make code clearer and more adaptable, providing consistent access to various collection types while minimizing code duplication and complexity. To master design patterns, enroll in ScholarHat's Master Software Architecture and Design Certification Training.