13
JulUnderstanding MVC, MVP and MVVM Design Patterns
MVC, MVP, and MVVM Design Patterns
MVC, MVP, and MVVM design patterns are critical for designing user interfaces in software development. These patterns divide concerns, making applications easier to manage, scale, and test.
In this design pattern tutorial, we'll look at what MVC (Model-View-Controller), MVP (Model-View-Presenter), and MVVM (Model-View-ViewModel) design patterns are when to use them and give some examples. Let's start by talking about "What are MVC, MVP, and MVVM design patterns?"
MVC Pattern
MVC stands for Model-View-Controller. It is a software design pattern that was introduced in the 1970s. Also, the MVC pattern forces a separation of concerns, which means the domain model and controller logic are decoupled from the user interface (view). As a result, maintenance and testing of the application have become simpler and easier.
MVC design pattern splits an application into three main aspects: Model, View, and Controller
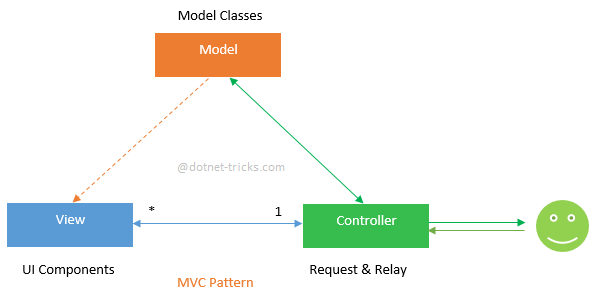
Model
- The Model component in the MVC design manages the application's data, business logic, and rules.
- It serves as the central repository for the data on which the program functions, encapsulating the application's basic functionality and behavior.
Purpose: manage the application's data and business logic.
Responsibilities:
- Manages data retrieval, storage, and manipulation.
- It contains the application's fundamental functionality and rules. Notifies the View whenever any data changes.
Real-Time example
In a blog application, the Model handles posts, comments, and user data.
View
- In the MVC pattern, the View component is responsible for presenting data to the user and managing the application's user interface (UI).
- It serves as a link between the user and the application's data, showing information in an understandable format and accepting user input.
Purpose: Communicate data from the model to the user. This is to record user input and send it to the controller.
Responsibilities:
- Display Data: Render data from the Model and dynamically update the user interface in response to Model changes.
- User Interaction: Capture user inputs such as clicks and form submissions and pass them to the Controller for processing.
Real-Time Example
Displaying a list of blog posts on a website and collecting user feedback.
Controller
- The Controller in the MVC Pattern serves as a bridge between the Model and the View.
- It accepts user input, modifies the Model, and selects the View to display.
- The Controller manages the flow of data within the program and ensures that user activities produce suitable answers.
Purpose: handle user input and update the model accordingly.To choose which View to display based on user actions and Model updates.
Responsibilities:
- Process Input: Record user actions in the View, like as clicks and form submissions, and use these inputs to update the model.
- Update Model: Use user input to perform Model operations such as data retrieval, change, and business logic execution.
Real-Time Example
Responding to a user's login request by validating credentials, updating the user session, and presenting the relevant dashboard.
Key points
- Intermediary Role: The Controller acts as a bridge between the View and the Model, ensuring that user actions result in data changes that are reflected in the UI.
- Control Flow: The Controller coordinates the program's flow, including user inputs, data processing, and UI updates.
- Decoupling of Logic: By separating input processing from data management and presentation, the Controller makes the program more modular and maintainable.
MVP pattern
- The MVP (Model-View-Presenter) pattern is an extension of the MVC pattern that aims to increase the separation of concerns and testability, especially in systems with sophisticated user interfaces.
- In MVP, the Presenter is responsible for handling the interaction between the Model and the View.
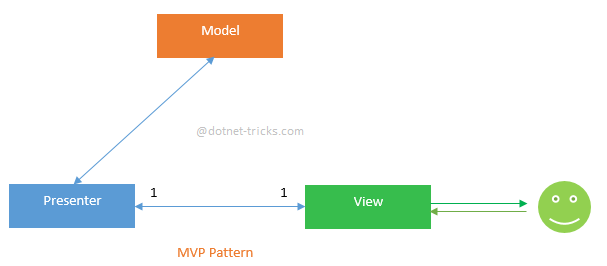
Model
Real-Time Example
View
Real-Time Example
In a task management program, the View would be the web pages or screens that display task and project information.
Presenter
Real-Time Example
- One-to-One Relationship: Typically, there is one Presenter for each View.
- View-Presenter Interaction: The View communicates with the Presenter, which updates the Model and then updates the View.
- Enhanced Testability: By separating the presentation logic into the Presenter, MVP makes it easier to write unit tests for the View and Presenter.
MVVM pattern
The MVVM (Model-View-ViewModel) Pattern is intended to allow a clear separation of concerns, especially in applications with complex user interfaces and data-binding capabilities. MVVM is widely used in modern user interface frameworks such as WPF, Xamarin, and Angular, where it uses data binding to synchronize the View and ViewModel.
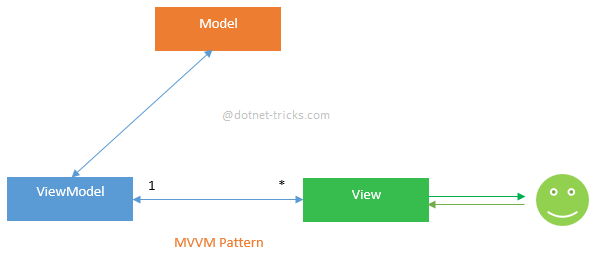
Model
Real-Time Example
View
Real-Time Example
ViewModel
Real-Time Example
Key Points about MVVM Pattern
- Data Binding: The View ties to the ViewModel, providing for automatic synchronization between the user interface and the underlying data.
- Separation of Concerns: The ViewModel separates presentation logic from UI, making the program more modular and testable.
- Two-Way Binding: Changes to the ViewModel are automatically reflected in the View and vice versa, eliminating the need for manual changes.
Difference Between MVC, MVP, and MVVM Design Patterns
MVC (MODEL VIEW CONTROLLER) | MVP (MODEL VIEW PRESENTER) | MVVM (MODEL VIEW VIEWMODEL) |
One of the earliest software architectural patterns. | Developed as a more advanced version of MVC. | An industry-recognized application architectural pattern. |
The UI (View) and data-access mechanism (Model) are bound together. | The presenter serves as a communication conduit, eliminating View reliance. | Data binding is used to segregate the underlying business logic from the view. |
Controllers and views have a one-to-many relationship. | A one-on-one relationship exists between the presenter and the viewer. | A one-to-many relationship exists between the ViewModel and numerous Views. |
The View has no awareness of the Controller. | The View contains references to the Presenter. | The View contains references to the ViewModel. |
Modifying is difficult due to the tightly connected code levels. | Loosely connected layers make the program easier to adapt. | Changes are simple to do, but sophisticated data binding might make debugging difficult. |
Perfect for small-scale projects only. | Suitable for both simple and complicated applications. | Not suitable for small-scale tasks. |
Note
Overall, the primary difference between these patterns is the involvement of the mediator component. MVC and MVP both use a Controller or Presenter to function as a go-between for the Model and the View, whereas MVVM uses a ViewModel to do the same.MVC is the simplest of these patterns, whereas MVP and MVVM are more versatile and allow for a clearer separation of concerns across the application's tiers.Summary
MVC, MVP, and MVVM are important design paradigms for organizing user interfaces. MVC, the earliest pattern, has tightly connected UI and data. MVP adds a Presenter to better segregate the View and Model, which improves testability. MVVM uses data binding and a ViewModel to decouple concerns and streamline updates. MVC is best for small projects, MVP is suitable for a wide range of applications, and MVVM is ideal for modern, data-driven systems. Also, consider our .NET design patterns training for a better understanding of other design patterns concepts.
Take our Designpatterns skill challenge to evaluate yourself!
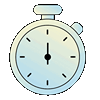
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.