30
DecTop SQL Joins Interview Questions You Need to Know
SQL Joins Questions You Must Know
If you're getting ready for an SQL interview, it's important to understand SQL joins. You might be asked to explain how different types of SQL joins work or write queries during the interview. Knowing how to use INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL OUTER JOIN is very helpful.
In this interview tutorial, we will go through common SQL joins interview questions with easy examples and clear explanations. By the end, you'll feel more confident and ready to answer any join question. These skills will also help you with real-world database tasks. Get ready to succeed in your SQL interview!
What can you expect in SQL joins interview questions?
Join interviews, which are designed to test your understanding of databases and how well you solve problems. It is common for you to be asked about different types of joins like INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL OUTER JOIN and how to use them in real-world situations. Whether you are a fresher or have experience, it is likely that you will be asked to write SQL queries, explain join concepts, and improve query performance. It is important for you to be well-prepared for these questions, including tricky cases, to increase your chances of success.
Read More: SQL Queries Interview Questions and Answers. |
Top 20 SQL joins interview questions for freshers
Example Tables:
Student:
ROLL_NO | NAME | AGE | CITY |
101 | Ravi Kumar | 21 | Delhi |
102 | Priya Patel | 22 | Mumbai |
103 | Arjun Singh | 20 | Bangalore |
Course:
COURSE_ID | COURSE_NAME | ROLL_NO |
C101 | Data Science | 101 |
C102 | Machine Learning | 102 |
C103 | Cloud Computing | 103 |
C104 | Web Development | 101 |
1. What is a JOIN in SQL?
A JOIN in SQL is used to combine rows from two or more tables based on a related column. For example, in the Student
and Course
tables, we can use ROLL_NO
to match rows in each table and view which student is taking which course.
2. Can you explain an INNER JOIN?
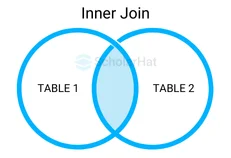
An INNER JOIN
selects records that have matching values in both tables. So, if we want to find students who are enrolled in a course, we can use an INNER JOIN
on ROLL_NO
:
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
INNER JOIN Course
ON Student.ROLL_NO = Course.ROLL_NO;
3. What is a LEFT JOIN?
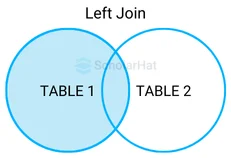
A LEFT JOIN
returns all records from the left table (Student) and the matched records from the right table (Course). If there is no match, NULL values are returned for columns from the right table. For example:
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
LEFT JOIN Course
ON Student.ROLL_NO = Course.ROLL_NO;
4. What is a RIGHT JOIN?
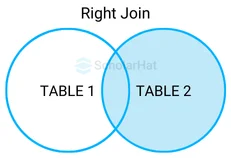
A RIGHT JOIN
returns all records from the right table (Course) and the matched records from the left table (Student). If no match is found, NULL values appear in columns from the left table. For example:
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
RIGHT JOIN Course
ON Student.ROLL_NO = Course.ROLL_NO;
5. What is a FULL JOIN?
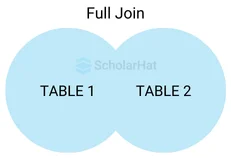
A FULL JOIN
returns all records when there is a match in either the left or right table. If there is no match, NULLs are returned for the unmatched rows. For example:
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
FULL JOIN Course
ON Student.ROLL_NO = Course.ROLL_NO;
6. Can we use multiple JOINs in a single query?
Yes, multiple JOINs can be used to combine data from more than two tables. Each JOIN needs to specify the matching column for the tables being joined.
7. What is a CROSS JOIN?
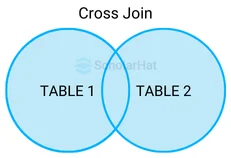
A CROSS JOIN
returns the Cartesian product of both tables. This means every row in the Student
table is combined with every row in the Course
table.
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
CROSS JOIN Course;
8. How do you perform a SELF JOIN?
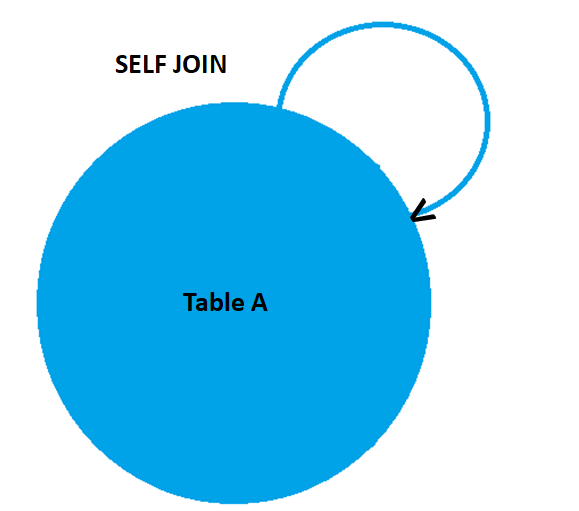
A SELF JOIN in sql
is a join of a table with itself. It's helpful for comparing rows within the same table. You use table aliases to differentiate the instances.
9. What’s the difference between Inner join and Outer join?
An INNER JOIN
returns only the matching rows from both tables, while an OUTER JOIN
(LEFT, RIGHT, or FULL) returns all rows from one or both tables, even if there’s no match.
10. How does a NATURAL JOIN work?
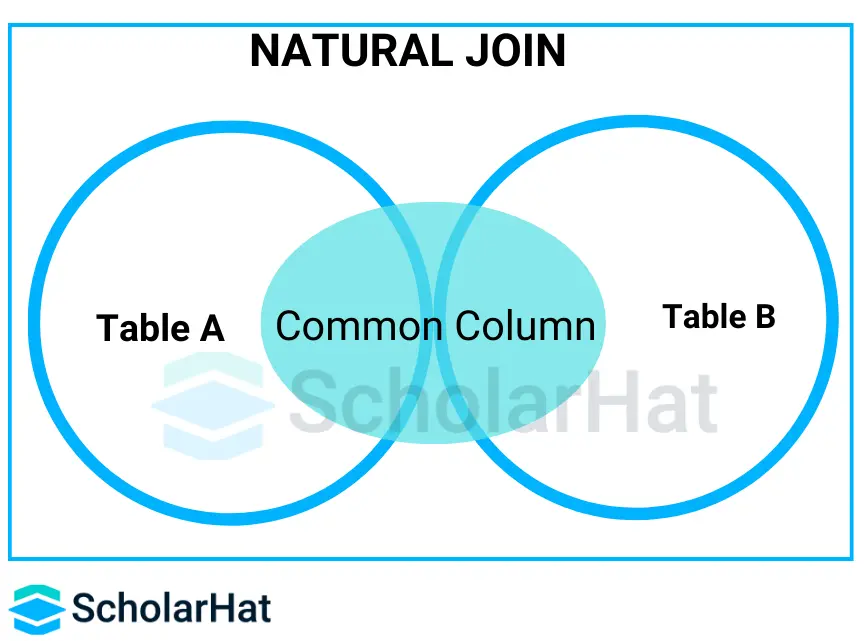
A NATURAL JOIN
automatically joins tables based on columns with the same name and datatype. It’s a shortcut but can cause issues if there are unexpected columns with the same name in both tables.
11. What is the purpose of using table aliases in JOINs?
Table aliases make queries easier to read, especially in JOINs with multiple tables or a SELF JOIN. For example:
SELECT S.NAME, C.COURSE_NAME
FROM Student AS S
INNER JOIN Course AS C
ON S.ROLL_NO = C.ROLL_NO;
12. How can you join more than two tables?
You can join multiple tables by adding additional JOIN statements. Each JOIN specifies which tables and columns should match.
13. What is the difference between JOIN and UNION?
A JOIN
combines columns from different tables, while a UNION
combines rows from different queries or tables, making it a vertical combination.
14. How do you filter results in a JOIN using WHERE clause?
Use the WHERE
clause to apply conditions after performing the JOIN. For instance, to find students from Delhi in a course:
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
INNER JOIN Course
ON Student.ROLL_NO = Course.ROLL_NO
WHERE Student.CITY = 'Delhi';
15. Can JOINs be used with aggregate functions?
Yes, JOINs can be used with aggregate functions like SUM
, AVG
, etc., allowing you to calculate grouped results across joined tables.
16. What’s the impact of indexing on JOIN performance?
Indexes on the joined columns (e.g., ROLL_NO
) improve JOIN performance by speeding up data retrieval, especially for large tables.
17. Can we join a table with a subquery?
Yes, you can join a table with a subquery by treating the subquery as a temporary table. It’s helpful in combining filtered data with other tables.
18. What is an equi join?
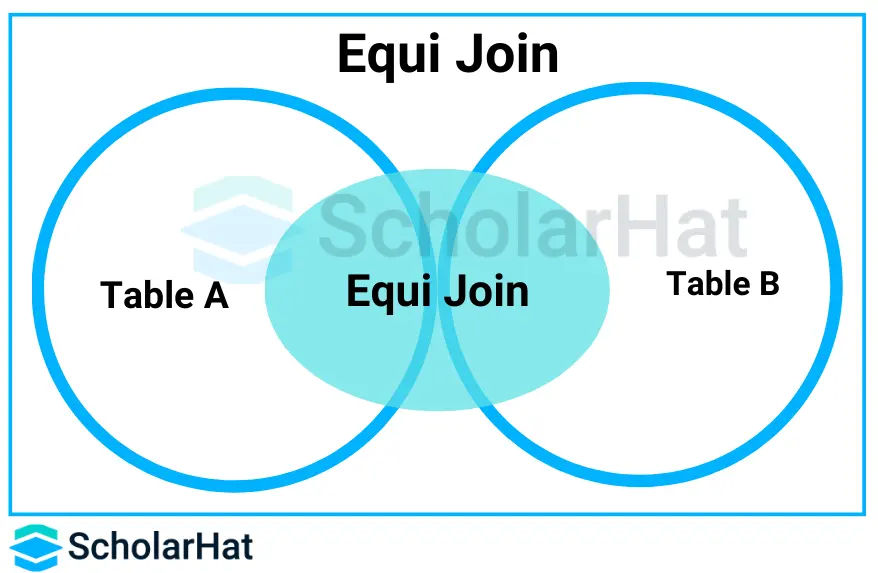
An equi join
is a type of join where we match rows based on equality conditions, usually with =
. Most joins are equi joins.
19. How can you exclude unmatched rows in a JOIN?
To exclude unmatched rows, use an INNER JOIN
. It only returns rows with matching values in both tables.
20. How do you handle NULL values in JOINs?
NULL values can cause mismatches in JOINs. Using IS NULL
or COALESCE
can help handle NULLs effectively, especially in OUTER JOINs.
NAME | COURSE_NAME |
---|---|
Ravi Kumar | Data Science |
Priya Patel | Machine Learning |
Arjun Singh | Cloud Computing |
Ravi Kumar | Web Development |
SQL joins interview questions for experienced
21. What is a NATURAL JOIN?
A NATURAL JOIN
automatically joins tables based on columns with the same name and data type in both tables. Unlike an inner join, you don’t explicitly specify the condition for the join.
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
NATURAL JOIN Course;
NAME | COURSE_NAME |
---|---|
Ravi Kumar | Data Science |
Priya Patel | Machine Learning |
Arjun Singh | Cloud Computing |
22. How would you use multiple JOINs in a query?
You can join multiple tables in a query by chaining JOIN
statements. This allows you to fetch related data from different tables. Each JOIN
should have an appropriate condition.
SELECT Student.NAME, Course.COURSE_NAME, Instructor.INSTRUCTOR_NAME
FROM Student
INNER JOIN Course ON Student.ROLL_NO = Course.ROLL_NO
INNER JOIN Instructor ON Course.COURSE_ID = Instructor.COURSE_ID;
NAME | COURSE_NAME | INSTRUCTOR_NAME |
---|---|---|
Ravi Kumar | Data Science | Dr. Sharma |
Priya Patel | Machine Learning | Prof. Verma |
Arjun Singh | Cloud Computing | Dr. Kumar |
23. Can you explain the concept of using aliases in joins?
Aliases are used to give temporary names to tables and columns, especially when working with self-joins or when the same table is involved multiple times in the query. This makes the query more readable.
SELECT S1.NAME AS Student_Name, S2.NAME AS Friend_Name
FROM Student S1
JOIN Student S2 ON S1.ROLL_NO = S2.ROLL_NO;
Student_Name | Friend_Name |
---|---|
Ravi Kumar | Priya Patel |
Arjun Singh | Arjun Singh |
24. What is the difference between JOIN and UNION?
While both JOIN
and UNION
combine data from multiple tables. The key difference is that JOIN
combines rows based on a condition while UNION
appends the result sets of two queries, ensuring no duplicates (use UNION ALL
to include duplicates).
SELECT Student.NAME FROM Student
UNION
SELECT Teacher.NAME FROM Teacher;
NAME |
---|
Ravi Kumar |
Priya Patel |
Prof. Sharma |
25. What is a CROSS JOIN?
A CROSS JOIN
returns the Cartesian product of both tables. Every row in the first table is joined with all rows in the second table. This can result in a very large result set.
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
CROSS JOIN Course;
NAME | COURSE_NAME |
---|---|
Ravi Kumar | Data Science |
Ravi Kumar | Machine Learning |
Ravi Kumar | Cloud Computing |
Priya Patel | Data Science |
Priya Patel | Machine Learning |
Priya Patel | Cloud Computing |
26. How do you prevent duplicate rows in a JOIN query?
To prevent duplicate rows in a JOIN query, use the DISTINCT
keyword. This eliminates duplicate records from the result set.
SELECT DISTINCT Student.NAME, Course.COURSE_NAME
FROM Student
INNER JOIN Course ON Student.ROLL_NO = Course.ROLL_NO;
NAME | COURSE_NAME |
---|---|
Ravi Kumar | Data Science |
Priya Patel | Machine Learning |
27. How would you find rows with matching values in only one table?
Use an OUTER JOIN
(left or right) and check for NULL
in the joined table to identify rows from the main table that do not have matching rows in the second table.
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
LEFT JOIN Course ON Student.ROLL_NO = Course.ROLL_NO
WHERE Course.COURSE_NAME IS NULL;
NAME | COURSE_NAME |
---|---|
Rajesh Kumar | NULL |
28. How do you handle NULL values in JOIN operations?
In SQL joins, NULL
values can affect the results. You can handle them by using the COALESCE()
function to replace NULL with a default value or handle them in the WHERE
clause.
SELECT Student.NAME, COALESCE(Course.COURSE_NAME, 'No Course') AS COURSE_NAME
FROM Student
LEFT JOIN Course ON Student.ROLL_NO = Course.ROLL_NO;
NAME | COURSE_NAME |
---|---|
Ravi Kumar | Data Science |
Rajesh Kumar | No Course |
29. What is a JOIN with multiple conditions?
You can use multiple conditions in the ON
clause of a JOIN to specify more complex matching logic, such as combining multiple columns for the join condition.
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
JOIN Course
ON Student.ROLL_NO = Course.ROLL_NO AND Course.YEAR = 2024;
NAME | COURSE_NAME |
---|---|
Ravi Kumar | Data Science |
Priya Patel | Machine Learning |
30. What is an example of using JOIN with a subquery?
JOINs can be combined with subqueries to retrieve data that meets specific criteria from another query.
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
INNER JOIN Course ON Student.ROLL_NO = Course.ROLL_NO
WHERE Course.COURSE_NAME IN (SELECT COURSE_NAME FROM Course WHERE YEAR = 2024);
NAME | COURSE_NAME |
---|---|
Ravi Kumar | Data Science |
Priya Patel | Machine Learning |
31. How would you JOIN three or more tables?
To join three or more tables, chain multiple JOINs, each with an appropriate condition. The process remains the same as joining two tables, but you repeat the JOIN operation for each additional table.
SELECT Student.NAME, Course.COURSE_NAME, Instructor.NAME
FROM Student
JOIN Course ON Student.ROLL_NO = Course.ROLL_NO
JOIN Instructor ON Course.COURSE_ID = Instructor.COURSE_ID;
NAME | COURSE_NAME | INSTRUCTOR_NAME |
---|---|---|
Ravi Kumar | Data Science | Dr. Sharma |
Priya Patel | Machine Learning | Prof. Verma |
32. What is a FULL OUTER JOIN?
A FULL OUTER JOIN
returns all records when there is a match in either the left or right table. It returns NULL
for non-matching rows from both tables.
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
FULL OUTER JOIN Course ON Student.ROLL_NO = Course.ROLL_NO;
NAME | COURSE_NAME |
---|---|
Ravi Kumar | Data Science |
Priya Patel | Machine Learning |
Arjun Singh | Cloud Computing |
Rajesh Kumar | NULL |
33. What is the difference between LEFT JOIN and RIGHT JOIN?
Both LEFT JOIN
and RIGHT JOIN
are types of OUTER JOINs, but:
LEFT JOINin SQL
returns all records from the left table and the matched records from the right table.RIGHT JOIN in SQL
returns all records from the right table and the matched records from the left table.
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
LEFT JOIN Course ON Student.ROLL_NO = Course.ROLL_NO;
NAME | COURSE_NAME |
---|---|
Ravi Kumar | Data Science |
Priya Patel | Machine Learning |
Arjun Singh | Cloud Computing |
Rajesh Kumar | NULL |
34. How do you write a JOIN query to get data from two tables with conditions on both?
To join two tables with conditions on both, you specify multiple conditions in the ON
clause or use the WHERE
clause to filter rows after joining.
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
JOIN Course ON Student.ROLL_NO = Course.ROLL_NO
WHERE Course.YEAR = 2024 AND Student.AGE > 20;
NAME | COURSE_NAME |
---|---|
Ravi Kumar | Data Science |
Priya Patel | Machine Learning |
35. What is the difference between a JOIN and a UNION in SQL?
JOIN
combines data from two or more tables based on a common column while UNION
combines results from two queries, stacking one result set below the other. UNION
Also removes duplicate rows by default.
SELECT Student.NAME FROM Student
UNION
SELECT Teacher.NAME FROM Teacher;
NAME |
---|
Ravi Kumar |
Priya Patel |
Prof. Sharma |
36. How would you perform a JOIN between more than two tables?
To perform a JOIN with multiple tables, chain the JOIN
conditions one after the other, specifying how each table is related to the others.
SELECT Student.NAME, Course.COURSE_NAME, Instructor.NAME
FROM Student
JOIN Course ON Student.ROLL_NO = Course.ROLL_NO
JOIN Instructor ON Course.COURSE_ID = Instructor.COURSE_ID;
NAME | COURSE_NAME | INSTRUCTOR_NAME |
---|---|---|
Ravi Kumar | Data Science | Dr. Sharma |
Priya Patel | Machine Learning | Prof. Verma |
37. What is the role of the ON
clause in a JOIN?
The ON
clause in a JOIN specifies the condition that defines how two tables are related. It is used to filter rows based on matching columns from both tables.
SELECT Student.NAME, Course.COURSE_NAME
FROM Student
INNER JOIN Course ON Student.ROLL_NO = Course.ROLL_NO;
NAME | COURSE_NAME |
---|---|
Ravi Kumar | Data Science |
Priya Patel | Machine Learning |
38. What is the impact of using DISTINCT
with JOIN?
Using DISTINCT
in a JOIN query ensures that the result set does not contain duplicate rows. It’s useful when you expect multiple matching rows from one table and only want to return unique combinations.
SELECT DISTINCT Student.NAME, Course.COURSE_NAME
FROM Student
INNER JOIN Course ON Student.ROLL_NO = Course.ROLL_NO;
NAME | COURSE_NAME |
---|---|
Ravi Kumar | Data Science |
Priya Patel | Machine Learning |
39. How would you handle multiple NULL values in JOIN operations?
In JOIN operations, NULL values in one or both tables can cause rows to be excluded. Use COALESCE()
or IFNULL()
functions to replace NULLs with a default value.
SELECT Student.NAME, COALESCE(Course.COURSE_NAME, 'No Course') AS COURSE_NAME
FROM Student
LEFT JOIN Course ON Student.ROLL_NO = Course.ROLL_NO;
NAME | COURSE_NAME |
---|---|
Ravi Kumar | Data Science |
Rajesh Kumar | No Course |
40. How can you join a table with a subquery in SQL?
You can join a table with a subquery in the FROM
clause. The subquery acts as a derived table that is treated like a regular table in the JOIN operation.
SELECT Student.NAME, SubQuery.COURSE_NAME
FROM Student
JOIN (SELECT COURSE_NAME, ROLL_NO FROM Course WHERE YEAR = 2024) AS SubQuery
ON Student.ROLL_NO = SubQuery.ROLL_NO;
NAME | COURSE_NAME |
---|---|
Ravi Kumar | Data Science |
Priya Patel | Machine Learning |
Summary
SQL join interview questions often focus on understanding different types of joins, such as INNER, LEFT, RIGHT, and FULL OUTER JOIN, as well as their use cases. Candidates should be prepared to explain how joins combine data from multiple tables based on related columns and demonstrate writing queries to illustrate these concepts. Practical knowledge of handling NULL values and complex join conditions can also be essential.FAQs
- INNER JOIN – returns only matching rows between tables.
- LEFT JOIN (or LEFT OUTER JOIN) – returns all rows from the left table and matched rows from the right.
- RIGHT JOIN (or RIGHT OUTER JOIN) – returns all rows from the right table and matching rows from the left.
- FULL OUTER JOIN – returns all rows from both tables, with NULLs for non-matching rows.
- INNER JOIN is ideal when you only need matching rows from the tables.
- LEFT JOIN is best when you need all rows from the left table and matching data from the right or to identify unmatched data.
- RIGHT JOIN is useful when the focus is on preserving all rows from the right table.
- FULL OUTER JOIN works well when you need all data from both tables, with NULLs for non-matching rows.
SELECT a.column1, b.column2 FROM table1 a, table2 b WHERE a.id = b.id;
Take our Interview skill challenge to evaluate yourself!
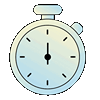
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.