24
JanTop OOPS JavaScript Interview Questions You Should Know!
OOPS JavaScript Interview Questions
Understanding OOPS (Object-Oriented Programming) principles in JavaScript is important for developers preparing for technical interviews. You might face questions about key ideas like classes, objects, Different Types of Inheritance, encapsulation, and polymorphism. You’ll also need to explain how JavaScript uses these concepts, which can be different because of its prototype-based inheritance.
This Interview tutorial covers common OOPS questions in JavaScript interviews, with simple explanations and examples to help you learn. By the end, you'll feel ready to answer questions confidently and use OOPS principles to write clear and organized JavaScript code.
Read More: Object-Oriented Programming Concepts |
What to Expect in OOPS JavaScript Interview Questions
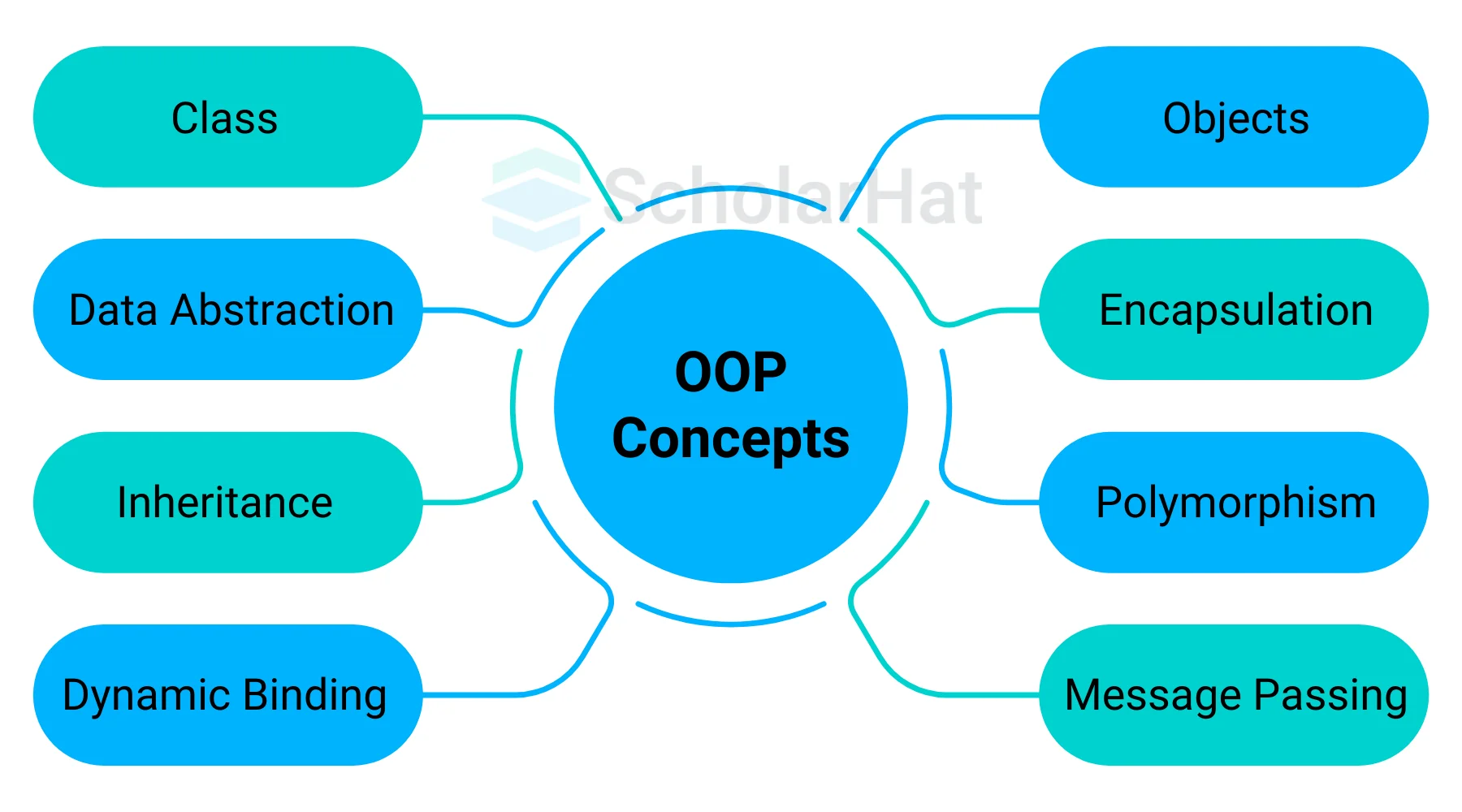
OOPS JavaScript Interview Questions for Freshers
1. What is Object-Oriented Programming (OOP)?
Ans: Object-Oriented Programming (OOP) is a programming paradigm that organizes data and behavior into reusable structures called objects. It focuses on four main principles: encapsulation, inheritance, polymorphism, and abstraction. OOP helps make code more modular, maintainable, and scalable.
2. What is a class in JavaScript?
Ans:
A class in JavaScript is a blueprint for creating objects with shared properties and methods. It is defined using the class
keyword. For example:
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
greet() {
console.log(`Hello, my name is ${this.name}`);
}
}
Output
No direct output. Run the code to see results.
3. What is an object in JavaScript?
Ans: An object in JavaScript is a standalone entity that contains properties (key-value pairs) and methods. It is like a real-world entity with attributes and behaviors.
let car = {
brand: "Toyota",
model: "Corolla",
drive: function() {
console.log("The car is driving");
}
};
console.log(car.brand); // Toyota
Output
Toyota
4. What is encapsulation in OOP?
Ans:
Encapsulation is the concept of wrapping data (properties) and behavior (methods) into a single unit (class). It restricts direct access to some components, providing controlled access through methods.
5. Explain inheritance in JavaScript.
Ans:
Inheritance allows one class (child class) to acquire the properties and methods of another class (parent class). It is implemented using the extends
keyword.
6. What is polymorphism in JavaScript?
Ans:
Polymorphism means "many forms." In JavaScript, it allows different objects to respond uniquely to the same method call, often using method overriding or function overloading.
Read More: Difference Between Method Overloading and Method Overriding in Java |
7. What is an abstraction in JavaScript?
Ans: Abstraction involves hiding the complex implementation details of functionality and showing only the necessary features. In JavaScript, this can be achieved through classes and objects.
8. What is the difference between class and object?
Ans: A class is a blueprint for creating objects. An object is an instance of a class containing actual values for the properties and methods defined in the class.
9. How do you create a class in JavaScript?
Ans: You create a class in JavaScript using the class
keyword. For example:
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a noise`);
}
}
Output
No direct output. Run the code to see results.
10. What are getters and setters in JavaScript?
Ans: Getters and setters are special methods in JavaScript that allow you to define custom logic when accessing or updating a property.
class Circle {
constructor(radius) {
this._radius = radius;
}
get radius() {
return this._radius;
}
set radius(value) {
if (value > 0) {
this._radius = value;
} else {
console.log("Radius must be positive");
}
}
}
Output
No direct output. Run the code to see results.
11. What is the prototype in JavaScript?
Ans: In JavaScript, every object has a built-in property called Prototype in JavaScript. It allows you to add methods and properties to objects, enabling inheritance.
function Person(name) {
this.name = name;
}
Person.prototype.greet = function() {
console.log("Hello, " + this.name);
};
let john = new Person("John");
john.greet(); // Hello, John
Output
Hello, John
12. What is a constructor in JavaScript?
Ans: A constructor is a special method that initializes an object when a class is called.
class Person {
constructor(name) {
this.name = name;
}
greet() {
console.log("Hi, I'm " + this.name);
}
}
let alice = new Person("Alice");
alice.greet(); // Hi, I'm Alice
Output
Hi, I'm Alice
Read More: Java Constructor |
13. What is method overriding in JavaScript?
Ans: Method overriding occurs when a subclass provides a specific implementation of a method already defined in its parent class.
class Animal {
sound() {
console.log("Animals make sound");
}
}
class Dog extends Animal {
sound() {
console.log("Dogs bark");
}
}
let dog = new Dog();
dog.sound(); // Dogs bark
Output
Dogs bark
14. How do you create an object in JavaScript?
Ans: You can create an object using curly braces {}
, the new Object()
method, or by using a class.
let car = { brand: "Tesla", color: "Blue" };
console.log(car.brand); // Tesla
Output
Tesla
15. What is the difference between static and instance methods?
Ans: Static methods belong to the class and can be called without creating an object. Instance methods belong to objects and require an object to be called.
class MathUtils {
static add(a, b) {
return a + b;
}
}
console.log(MathUtils.add(3, 5)); // 8
Output
8
Read More: Static Keyword in Java |
16. What is a "this" keyword in JavaScript?
Ans: The this
keyword refers to the object it belongs to. Its value depends on how the function is called.
let user = {
name: "Sam",
greet: function() {
console.log("Hi, " + this.name);
}
};
user.greet(); // Hi, Sam
Output
Hi, Sam
Read More: This Keyword in Java |
17. What are access modifiers in JavaScript?
Ans: Access modifiers control access to class properties or methods. JavaScript uses #
for private properties.
class Circle {
#radius;
constructor(radius) {
this.#radius = radius;
}
getRadius() {
return this.#radius;
}
}
let circle = new Circle(5);
console.log(circle.getRadius()); // 5
Output
5
Read More: Java Access Modifiers: Default, Private, Protected, Public |
18. How does inheritance work in JavaScript?
Ans: Inheritance allows one class to use properties and methods of another class using the extends
keyword.
class Vehicle {
start() {
console.log("Vehicle is starting");
}
}
class Car extends Vehicle {
start() {
console.log("Car is starting");
}
}
let car = new Car();
car.start(); // Car is starting
Output
Car is starting
19. What is the difference between "==" and "==="?
Ans: The ==
operator checks value equality, ignoring type. The ===
operator checks both value and type equality.
console.log(5 == "5"); // true
console.log(5 === "5"); // false
Output
true
false
20. What is the purpose of the Object.create()
method?
Ans: The Object.create()
method creates a new object using an existing object as its prototype.
let person = {
greet: function() {
console.log("Hello!");
}
};
let friend = Object.create(person);
friend.greet(); // Hello!
Output
Hello!
OOPS JavaScript Interview Questions for Intermediate
21. What is the difference between function declaration and function expression in JavaScript?
Ans: A function declaration is hoisted, so you can call it before it's defined. A function expression is not hoisted, so you can only call it after it's defined.
// Function Declaration
greet();
function greet() {
console.log("Hello!");
}
// Function Expression
// greetMe(); // Error: Cannot access before initialization
const greetMe = function () {
console.log("Hi!");
};
greetMe();
Output
Hello!
Hi!
22. What are closures in JavaScript?
Ans: Closures allow a function to remember and access its scope even when the function is executed outside that scope.
function greet(name) {
return function () {
console.log("Hello, " + name);
};
}
let greetRaj = greet("Raj");
greetRaj(); // Hello, Raj
Output
Hello, Raj
23. What is the difference between call, apply, and bind methods in JavaScript?
Ans: These methods are used to control the value of this
in a function:
call()
: Calls a function with arguments passed individually.apply()
: Calls a function with arguments passed as an array.bind()
: Returns a new function with a specificthis
value.
let user = {
name: "Priya",
};
function greet(greeting, punctuation) {
console.log(greeting + ", " + this.name + punctuation);
}
greet.call(user, "Hello", "!"); // Hello, Priya!
greet.apply(user, ["Hi", "!"]); // Hi, Priya!
let boundGreet = greet.bind(user, "Hey");
boundGreet("?"); // Hey, Priya?
Output
Hello, Priya!
Hi, Priya!
Hey, Priya?
24. How do you create a class in JavaScript?
Ans: You can create a class using the class
keyword. Classes can have a constructor and methods.
class Student {
constructor(name, grade) {
this.name = name;
this.grade = grade;
}
displayInfo() {
console.log(this.name + " is in grade " + this.grade);
}
}
let ramesh = new Student("Ramesh", 10);
ramesh.displayInfo(); // Ramesh is in grade 10
Output
Ramesh is in grade 10
25. What is the use of the spread operator?
Ans: The spread operator (...
) allows you to expand arrays or objects into individual elements or properties.
let numbers = [1, 2, 3];
let newNumbers = [...numbers, 4, 5];
console.log(newNumbers); // [1, 2, 3, 4, 5]
Output
[1, 2, 3, 4, 5]
26. What are promises in JavaScript?
Ans: Promises are used to handle asynchronous operations. A promise can be in one of three states: pending
, fulfilled
, or rejected
.
let promise = new Promise((resolve, reject) => {
setTimeout(() => resolve("Data received"), 2000);
});
promise.then((data) => console.log(data)); // Data received (after 2 seconds)
Output
Data received
27. What is destructuring in JavaScript?
Ans: Destructuring allows you to extract values from arrays or objects into variables.
let person = { name: "Anita", age: 25 };
let { name, age } = person;
console.log(name); // Anita
console.log(age); // 25
Output
Anita
25
28. How does the async/await
syntax work in JavaScript?
Ans: The async
keyword makes a function return a promise and await
pauses execution until the promise resolves.
async function fetchData() {
let data = await Promise.resolve("Data loaded");
console.log(data);
}
fetchData(); // Data loaded
Output
Data loaded
29. What are arrow functions in JavaScript?
Ans: Arrow functions are a shorter syntax for writing functions. They do not have their own this
.
let add = (a, b) => a + b;
console.log(add(5, 3)); // 8
Output
8
30. How can you handle errors in JavaScript?
Ans: You can handle errors using try
, catch
, and finally
blocks.
try {
throw new Error("Something went wrong");
} catch (error) {
console.log(error.message);
} finally {
console.log("Cleanup done");
}
Output
Something went wrong
Cleanup done
Read More: Exception Handling in Java |
31. What is the prototype in JavaScript?
Ans: A prototype is an object from which other objects inherit properties. Every JavaScript object has a prototype that links to other objects for property sharing.
function Person(name) {
this.name = name;
}
Person.prototype.greet = function () {
console.log("Hello, " + this.name);
};
let neeraj = new Person("Neeraj");
neeraj.greet(); // Hello, Neeraj
Output
Hello, Neeraj
32. What is event delegation in JavaScript?
Ans: Event delegation is a technique where you attach a single event listener to a parent element to manage events for its child elements.
document.getElementById("parent").addEventListener("click", function (event) {
if (event.target.tagName === "BUTTON") {
console.log("Button clicked: " + event.target.textContent);
}
});
Output
Button clicked: Button Text
33. How does Object.create()
work in JavaScript?
Ans: The Object.create()
method creates a new object with the specified prototype object.
let parent = {
greet: function () {
console.log("Hello from parent");
},
};
let child = Object.create(parent);
child.greet(); // Hello from parent
Output
Hello from parent
34. What is the difference between synchronous and asynchronous code?
Ans: Synchronous code executes line by line, blocking further execution until the current task is completed. Asynchronous code runs tasks in the background, allowing other operations to continue.
// Synchronous code
console.log("Start");
console.log("End");
// Asynchronous code
console.log("Start");
setTimeout(() => console.log("Async Task"), 1000);
console.log("End");
Output
Start
End
Async Task
35. What is a module in JavaScript?
Ans: A module is a reusable piece of JavaScript code that can be imported or exported between files using import
and export
.
// File: math.js
export function add(a, b) {
return a + b;
}
// File: main.js
import { add } from "./math.js";
console.log(add(3, 5)); // 8
Output
8
OOPS JavaScript Interview Questions for Experienced
36. What is the difference between deep copy and shallow copy?
Ans: A shallow copy copies the reference of objects, so changes in one affect the other. A deep copy creates an independent duplicate.
// Shallow Copy
let obj1 = { name: "Aman" };
let shallowCopy = Object.assign({}, obj1);
shallowCopy.name = "Ravi";
console.log(obj1.name); // Aman
// Deep Copy
let obj2 = { age: 30 };
let deepCopy = JSON.parse(JSON.stringify(obj2));
deepCopy.age = 25;
console.log(obj2.age); // 30
Output
Aman
30
37. How does the Map
object differ from a plain object?
Ans: Map
is better for storing key-value pairs because it preserves order and allows any JavaScript Data Typesas keys.
let map = new Map();
map.set(1, "One");
map.set("two", 2);
console.log(map.get(1)); // One
console.log(map.get("two")); // 2
Output
One
2
38. What is the difference between var
, let
, and const
?
Ans: var
has function scope and is hoisted. let
and const
have block scope. const
cannot be reassigned.
var a = 10;
let b = 20;
const c = 30;
// Reassigning
a = 15; // OK
b = 25; // OK
// c = 35; // Error
console.log(a, b, c); // 15 25 30
Output
15 25 30
39. What are generators in JavaScript?
Ans: Generators are special functions that can pause and resume their execution using yield
.
function* generatorFunction() {
yield "First";
yield "Second";
return "Done";
}
let gen = generatorFunction();
console.log(gen.next().value); // First
console.log(gen.next().value); // Second
console.log(gen.next().value); // Done
Output
First
Second
Done
40. What is memoization, and how is it implemented?
Ans: Memoization is a technique to optimize functions by caching their results for specific inputs.
function memoize(fn) {
let cache = {};
return function (n) {
if (cache[n]) {
return cache[n];
}
cache[n] = fn(n);
return cache[n];
};
}
function square(n) {
return n * n;
}
let memoizedSquare = memoize(square);
console.log(memoizedSquare(5)); // 25 (calculated)
console.log(memoizedSquare(5)); // 25 (cached)
Output
25
25
41. What are WeakMaps and WeakSets in JavaScript?
Ans: WeakMaps and WeakSets are collections where keys and values are weakly referenced. They are useful for caching without preventing garbage collection.
let weakMap = new WeakMap();
let obj = { name: "Arjun" };
weakMap.set(obj, "Developer");
console.log(weakMap.get(obj)); // Developer
Output
Developer
Read More: Garbage Collection in C# |
42. How does this
work in arrow functions?
Ans: In arrow functions, this
is lexically bound to the enclosing context and does not change.
let obj = {
name: "Maya",
greet: () => console.log("Hello, " + this.name),
};
obj.greet(); // Hello, undefined
Output
Hello, undefined
43. What is currying in JavaScript?
Ans: Currying is the process of transforming a function with multiple arguments into a series of functions that each take one argument.
function curry(a) {
return function (b) {
return function (c) {
return a + b + c;
};
};
}
console.log(curry(1)(2)(3)); // 6
Output
6
44. How do you debounce a function?
Ans: Debouncing delays a function's execution until after a specified time has passed since the last invocation.
function debounce(func, delay) {
let timer;
return function (...args) {
clearTimeout(timer);
timer = setTimeout(() => func(...args), delay);
};
}
const log = debounce(() => console.log("Debounced"), 1000);
log();
log();
log(); // Only executes once after 1 second
Output
Debounced
45. What is the purpose of the Symbol
type in JavaScript?
Ans: Symbol
is used to create unique identifiers that avoid property name collisions in objects.
let sym = Symbol("unique");
let obj = { [sym]: "Hello" };
console.log(obj[sym]); // Hello
Output
Hello
46. What is a JavaScript Proxy, and how is it used?
Ans: A Proxy allows you to create custom behavior for basic operations on objects like getting or setting properties.
let handler = {
get: function (target, property) {
return property in target ? target[property] : "Property not found";
},
};
let obj = { name: "Ravi" };
let proxy = new Proxy(obj, handler);
console.log(proxy.name); // Ravi
console.log(proxy.age); // Property not found
Output
Ravi
Property not found
47. What are async/await in JavaScript?
Ans: async
and await
simplify working with promises, making asynchronous code look like synchronous code.
async function fetchData() {
let promise = new Promise((resolve) => setTimeout(() => resolve("Data fetched"), 1000));
let result = await promise;
console.log(result);
}
fetchData();
Output
Data fetched
48. What is the difference between call, apply, and bind?
Ans: call()
invokes a function with arguments passed individually. apply()
passes arguments as an array. bind()
creates a new function with a specific this
.
function greet(greeting) {
console.log(greeting + ", " + this.name);
}
let user = { name: "Vikram" };
// Using call
greet.call(user, "Hello"); // Hello, Vikram
// Using apply
greet.apply(user, ["Hi"]); // Hi, Vikram
// Using bind
let boundGreet = greet.bind(user, "Namaste");
boundGreet(); // Namaste, Vikram
Output
Hello, Vikram
Hi, Vikram
Namaste, Vikram
49. How do you handle errors in promises?
Ans: Errors in promises are handled using .catch()
or try...catch
with async/await.
// Using .catch()
let promise = new Promise((_, reject) => reject("Error occurred"));
promise.catch((error) => console.log(error)); // Error occurred
// Using try...catch
async function fetchData() {
try {
let promise = Promise.reject("Failed to fetch");
await promise;
} catch (error) {
console.log(error); // Failed to fetch
}
}
fetchData();
Output
Error occurred
Failed to fetch
50. What is a Service Worker in JavaScript?
Ans: A Service Worker is a script that runs in the background, separate from the web page, enabling features like offline support and caching.
// Registering a Service Worker
if ("serviceWorker" in navigator) {
navigator.serviceWorker
.register("/sw.js")
.then(() => console.log("Service Worker Registered"))
.catch((error) => console.log("Registration failed:", error));
}
Output
Service Worker Registered
Read More: JavaScript Interview Questions & Answers |
Summary
This tutorial covers the top 50 OOPS JavaScript interview questions and answers, organized by experience levels: fresher, intermediate, and experienced. It provides a clear understanding of core OOPS concepts in JavaScript, such as classes, objects, inheritance, encapsulation, and polymorphism, along with practical examples. By reviewing these questions, you’ll be ready to excel in interviews and demonstrate your expertise in OOPS using JavaScript.Boost your JavaScript knowledge with Scholarhat's JavaScript Course! Enroll now and master OOPS principles to build clean and maintainable applications.