18
JulArithmetic operators in Java
In Java, arithmetic operators are used to perform basic mathematical operations on numeric values. They can be applied to all primitive number types, including byte, short, int, long, float, and double.
We learned the Types of Operators in the previous Java tutorial. In this Java tutorial, we'll explore the syntax, types, and examples of arithmetic operators in Java. To further enhance your understanding and application of arithmetic operator concepts, consider enrolling in the best Java Certification Course Free, to gain knowledge about the effective utilization of arithmetic operators for improved problem-solving and time management.
What are the Arithmetic Operators in Java?
Operators in Java, a Java toolkit, are being used as a symbol that performs various operations according to the code. Arithmetic operators are fundamental components of Java that empower developers to perform mathematical operations and manipulate numeric values within their programs. Arithmetic operators are symbols or characters that perform mathematical operations on numeric operands. The primary arithmetic operators in Java include addition (+), subtraction (-), multiplication (*), division (/), and modulus (%).
Syntax:
The syntax for using arithmetic operators in Java is straightforward:
Here, operand1 and operand2 are numeric values or variables, and the operator is the arithmetic operator that defines the operation to be performed.
Read More -
Types of Arithmetic Operators
Arithmetic Operators in Java are particularly used for performing arithmetic operations on given data or variables. There are various types of operators in Java, such as
Operators | Operations |
+ | Addition |
- | Subtraction |
x | Multiplication |
/ | Division |
% | Modulus |
1. Addition Operator (+)
The addition operator (+) is used to add two numeric values. It can be applied to variables, literals, or a combination of both.
Example
int a = 5;
int b = 3;
int sum = a + b;
System.out.println("Sum: " + sum);
In this example, the variables a and b are added, and the result is stored in the variable sum. The output will be Sum: 8.
2. Subtraction Operator (-)
The subtraction operator (-) is used to subtract the right operand from the left operand.
Example
int x = 10;
int y = 7;
int difference = x - y;
System.out.println("Difference: " + difference);
The output of this code will be Difference: 3.
3. Multiplication Operator
The multiplication operator (*) is used to multiply two numeric values.
Example
int p = 4;
int q = 6;
int product = p * q;
System.out.println("Product: " + product);
This code will output Product: 24.
4. Division Operator (/)
Example
double numerator = 15.0;
int denominator = 3;
double result = numerator / denominator;
System.out.println("Result: " + result);
5. Modulus Operator (%)
Example
int num = 17;
int divisor = 5;
int remainder = num % divisor;
System.out.println("Remainder: " + remainder);
6. Operator Precedence
Example
int result = 10 + 2 * 5;
System.out.println("Result: " + result);
Read More - Java Programmer Salary In India
Example of Arithmetic Operators in Java
public class ArithmeticOperationsExample {
public static void main(String[] args) {
// Given values
int a = 10;
int b = 3;
// Arithmetic operations
int sum = a + b; // 13
int difference = a - b; // 7
int product = a * b; // 30
double quotient = (double) a / b; // 3.333...
int remainder = a % b; // 1
// Displaying results
System.out.println("Sum: " + sum);
System.out.println("Difference: " + difference);
System.out.println("Product: " + product);
System.out.println("Quotient: " + quotient);
System.out.println("Remainder: " + remainder);
}
}
This Java program initializes two variables a and b with values 10 and 3, respectively. It then performs the specified arithmetic operations and prints the results to the console. The explicit casting (double) is used to ensure that the division results in a floating-point value.
Output
Sum: 13
Difference: 7
Product: 30
Quotient: 3.3333333333333335
Remainder: 1
- Relational operators in Java
- Logical operators in Java
- Assignment operator in Java
- Unary operator in Java
- Bitwise operator in Java
- Ternary operator in Java
Why they are so important:
- Basic Building Block of Logic: You can't write meaningful programs without performing calculations. Arithmetic operators let you handle numbers efficiently.
- Used in Real-World Applications: From calculating totals in billing systems to updating game scores or processing sensor data in IoT, arithmetic operations are everywhere.
- Support for Problem Solving and Decision Making: You often need to compare values or compute results before making decisions (like if statements), and that usually involves arithmetic.
- Works with Different Data Types: Arithmetic operators can be used with integers, floating-point numbers, characters (as ASCII), and more—making them highly flexible.
- Foundation for Advanced Concepts: They are used in loops, algorithms, and data structures like arrays and matrices, where calculations are frequently required.
Common Mistakes to Avoid When Using Arithmetic Operators in Java
While arithmetic operators in Java are simple to use, beginners often make a few common mistakes. Understanding these will help you write accurate and bug-free programs.
1. Integer Division Confusion-In Java, if both operands are integers, division gives only the whole number (quotient) and discards the decimal part.
Example:
int a = 10, b = 3;
System.out.println(a / b); // Output: 3 (NOT 3.33)
2. Not Using Parentheses Properly in Expressions-Without parentheses, Java follows operator precedence rules, which may not give the expected result.
Example:
int result = 10 + 5 * 2; // Output: 20 (Multiplication happens first)
3. Using % (Modulus) Instead of / (Division)-Beginners often confuse the modulus operator % with division /./ gives the quotient, while % gives the remainder.
Example:
int a = 10, b = 3;
System.out.println(a / b); // Output: 3 (Quotient)
System.out.println(a % b); // Output: 1 (Remainder)
Summary
FAQs
Take our Java skill challenge to evaluate yourself!
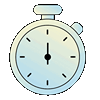
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.