04
JulAssignment operator in Java
Assignment Operators in Java: An Overview
We already discussed the Types of Operators in the previous tutorial Java. In this Java tutorial, we will delve into the different types of assignment operators in Java, and their syntax, and provide examples for better understanding. Because Java is a flexible and widely used programming language. Assignment operators play a crucial role in manipulating and assigning values to variables. To further enhance your understanding and application of Java assignment operator's concepts, consider enrolling in the best Java Online Course Free With Certificate.
What are the Assignment Operators in Java?
Assignment operators in Java are used to assign values to variables. They are classified into two main types: simple assignment operator and compound assignment operator.
Syntax:
The general syntax for a simple assignment statement is:
variable = expression;
And for a compound assignment statement:
variable operator= expression;
Read More -
Join ScholarHat’s Java Full-Stack program and start coding like a pro. | |
Training Name | Price |
Full-Stack Java Developer Training (Guaranteed Path to ₹3 - ₹9 LPA* Jobs) | Book a FREE Live Demo! |
Types of Assignment Operators in Java
- Simple Assignment Operator: The Simple Assignment Operator is used with the "=" sign, where the operand is on the left side and the value is on the right. The right-side value must be of the same data type as that defined on the left side.
- Compound Assignment Operator: Compound assignment operators combine arithmetic operations with assignments. They provide a concise way to perform an operation and assign the result to the variable in one step. The Compound Operator is utilized when +,-,*, and / are used in conjunction with the = operator.
1. Simple Assignment Operator (=):
The equal sign (=) is the basic assignment operator in Java. It is used to assign the value on the right-hand side to the variable on the left-hand side.
Syntax
operator1 = operator2;
Example
import java.io.*;
class AssignmentOperator {
public static void main(String[] args)
{
int value;
String data;
value = 2024;
data = "ScholarHat";
System.out.println("value is assigned: " + value);
System.out.println("name is assigned: " + data);
}
}
Explanation
Output
value is assigned: 2024
name is assigned: ScholarHat
2. Addition Assignment Operator (+=) :
Syntax
operator1 += operator2;
This means,
operator1 = operator1 + operator2;
Example
import java.io.*;
class AssignmentOperator {
public static void main(String[] args)
{
int value1 = 12, value2 = 24;
System.out.println("value1 is assigned: " + value1);
System.out.println("value2 is assigned: " + value2);
value1 += value2;
System.out.println("value1 = " + value1);
}
}
Explanation
Output
value1 is assigned: 12
value2 is assigned: 24
value1 = 36
3. Subtraction Operator (-=):
Syntax
operator1 -= operator2;
This means,
operator1 = operator1 - operator2;
Example
import java.io.*;
class AssignmentOperator {
public static void main(String[] args)
{
int value1 = 20, value2 = 10;
System.out.println("value1 is assigned: " + value1);
System.out.println("value2 is assigned: " + value2);
value1 -= value2;
System.out.println("value1 = " + value1);
}
}
Explanation
Output
value1 is assigned: 20
value2 is assigned: 10
value1 = 10
4. Multiplication Operator (*=):
Syntax
operator1 *= operator2;
This means,
operator1 = operator1 * operator2;
Example
import java.io.*;
class AssignmentOperator {
public static void main(String[] args)
{
int value1 = 20, value2 = 10;
System.out.println("value1 is assigned: " + value1);
System.out.println("value2 is assigned: " + value2);
value1 *= value2;
System.out.println("value1 = " + value1);
}
}
Explanation
Output
value1 is assigned: 20
value2 is assigned: 10
value1 = 200
Read More - Java Developer Salary
5. Division Operator (/=):
Syntax
operator1 /= operator2;
This means,
operator1 = operator1 / operator2;
Example
import java.io.*;
class AssignmentOperator {
public static void main(String[] args)
{
int value1 = 20, value2 = 10;
System.out.println("value1 is assigned: " + value1);
System.out.println("value2 is assigned: " + value2);
value1 /= value2;
System.out.println("value1 = " + value1);
}
}
Explanation
Output
value1 is assigned: 20
value2 is assigned: 10
value1 = 2
6. Modulus Assignment Operator (%=):
Syntax
operator1 %= operator2;
This means,
operator1 = operator1 % operator2;
Example
import java.io.*;
class AssignmentOperator {
public static void main(String[] args)
{
int value1 = 20, value2 = 7;
System.out.println("value1 is assigned: " + value1);
System.out.println("value2 is assigned: " + value2);
value1 %= value2;
System.out.println("value1 = " + value1);
}
}
Explanation
Output
value1 is assigned: 20
value2 is assigned: 7
value1 = 6
Example of Assignment Operator in Java
Let's look at a few examples in our Java Playground to illustrate the usage of assignment operators in Java:
public class AssignmentOperatorsExample {
public static void main(String[] args) {
// Simple Assignment Operator
int a = 10;
// Compound Assignment Operator
int b = 5;
b += 3; // b is now 8
int c = 12;
c -= 4; // c is now 8
int d = 6;
d *= 2; // d is now 12
int e = 20;
e /= 5; // e is now 4
int f = 17;
f %= 4; // f is now 1
// Displaying the results
System.out.println("= Operator : " + a);
System.out.println("+= Operator : " + b);
System.out.println("-= Operator : " + c);
System.out.println("*= Operator : " + d);
System.out.println("/= Operator : " + e);
System.out.println("%= Operator : " + f);
}
}
Explanation
Output
= Operator : 10
+= Operator : 8
-= Operator : 8
*= Operator : 12
/= Operator : 4
%= Operator : 1
Read More
- Unary Operator in Java
- Arithmetic Operators in Java
- Relational Operators in Java
- Logical Operators in Java
- Ternary Operator in Java
Summary
FAQs
1. Simple Assignment Operator
- 1. (=) operator
- 1. (+=) operator
- 2. (-=) operator
- 3. (*=) operator
- 4. (/=) operator
- 5. (%=) operator
Take our Java skill challenge to evaluate yourself!
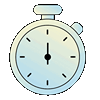
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.