04
JulTop 50 Core Java Interview Questions
Core Java Interview Questions
Java is a powerful programming language that opens up countless career opportunities, but preparing for interviews can feel overwhelming. If you're a student looking for a way to ease your nerves and boost your confidence, you’re in the right place! In this article, we’ll cover essential Core Java interview questions that will help you feel ready and relaxed as you step into your next interview. Let’s tackle this together!
In this Java Tutorial, For anyone preparing for a Java development role, mastering core Java interview questions is essential to demonstrate proficiency in the language. and this will guide you through some of the most frequently asked core Java interview questions, helping you prepare effectively.
Top 20 Core Java Interview Questions and Answers For Beginners
1. What is Java?
Java is a high-level, object-oriented programming language designed to be platform-independent. It allows developers to write code that can run on any machine with a Java Virtual Machine (JVM). Java is widely used for building desktop, mobile, web, and enterprise applications due to its robustness and scalability.
2. What are the features of Java?
Java has several key features, including:
- Object-Oriented: Everything in Java is treated as an object.
- Platform Independent: Java bytecode runs on any platform that has the JVM.
- Multithreaded: Java supports concurrent execution of multiple threads.
- Security: Java provides a secure environment through bytecode verification and the Security Manager.
3. What are JVM, JRE, and JDK?
The JVM (Java Virtual Machine) executes Java bytecode. JRE (Java Runtime Environment) includes the JVM and necessary libraries to run Java applications. JDK (Java Development Kit) is a complete toolkit for developing Java applications, including JRE, compiler, and development tools like debugger and profilers.
4. What is the difference between a local variable and an instance variable?
A local variable is declared inside a method, and its scope is limited to that method. It is destroyed once the method is completed. An instance variable is declared in a class but outside any method, and it belongs to the object of the class, persisting as long as the object exists.
5. What is a class in Java?
A Class in Javais a blueprint that defines the attributes and methods of objects. It encapsulates the state and behavior of the object and allows the creation of objects that share common properties and behaviors.
Example
class Car {
String model;
int year;
// Constructor
Car(String model, int year) {
this.model = model;
this.year = year;
}
void displayInfo() {
System.out.println("Car Model: " + model + ", Year: " + year);
}
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car("Toyota", 2020);
myCar.displayInfo();
}
}
Output
Car Model: Toyota, Year: 2020
Explanation
- In this example, the
Car
a class represents a real-world object with attributes likemodel
andyear
. An object of theCar
class is created, and its attributes are initialized using the constructor. - The
displayInfo()
method prints the car's information to the console.
6. What is an object in Java?
An object is an instance of a class. It represents a real-world entity with attributes (data) and behaviors (methods) defined by the class. Objects allow you to work with data and methods together in an organized way.
7. What is inheritance in Java?
- Inheritance is a mechanism in Java where one class (subclass) inherits properties and methods from another class (superclass).
- This promotes code reuse and allows for hierarchical classification.
- Inheritance also enables method overriding, allowing a subclass to provide specific implementations of methods from the superclass.
8. What is method overloading in Java?
Method Overloading InJava,a class canhave more than one method with the same name but different parameter lists (number, type, or both). It helps in defining methods that perform similar functions but take different inputs.
Example
class Calculator {
// Overloaded add method for integers
int add(int a, int b) {
return a + b;
}
// Overloaded add method for doubles
double add(double a, double b) {
return a + b;
}
}
public class Main {
public static void main(String[] args) {
Calculator calc = new Calculator();
System.out.println("Sum of integers: " + calc.add(10, 20));
System.out.println("Sum of doubles: " + calc.add(5.5, 6.5));
}
}
Output
Sum of integers: 30
Sum of doubles: 12.0
Explanation
- This program demonstrates method overloading in the
Calculator
class. The methodadd()
is overloaded to handle both integer and double inputs. - When the
add()
method is called with integers, the integer version is executed. When called with doubles, the double version is executed.
9. What is method overriding in Java?
Method overriding in Java occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. It allows the subclass to modify the behavior of the method for its own purposes while keeping the method name and parameters the same.
Example
class Animal {
// Superclass method
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
// Overriding the superclass method
@Override
void sound() {
System.out.println("Dog barks");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.sound(); // Calls the overridden method in Dog class
}
}
Output
Dog barks
Explanation
- In this example, the
Dog
class overrides thesound()
method of theAnimal
class. When thesound()
the method is called on aDog
object, the overridden method inDog
is executed. - This demonstrates runtime polymorphism, where the appropriate method is determined at runtime.
10. What is an abstraction in Java?
Abstraction is a process of hiding the implementation details and showing only the functionality to the user. In Java, abstraction is achieved using abstract classes and interfaces. It allows you to focus on what an object does rather than how it does it.
11. What is an interface in Java?
An interface in Java is a blueprint of a class that contains only abstract methods and static constants. It is used to achieve abstraction and multiple inheritance in Java. Classes that implement an interface must provide implementations for all the methods declared in the interface.
12. What is a package in Java?
A package in Java is a namespace that organizes a set of related classes and interfaces. It helps avoid name conflicts and provides access control. Commonly used packages include java.lang
(automatically imported) and java.util
(for utility classes).
13. What is the difference between static and non-static methods?
Static methods belong to the class and can be called without creating an object of the class. Non-static methods belong to an object and require an object to be instantiated before being called. Static methods cannot access instance variables directly.
14. What is encapsulation in Java?
Encapsulation is the process of wrapping the data (variables) and methods into a single unit, known as a class. It restricts direct access to some of the object's components, which is usually done by declaring fields as private and providing public getter and setter methods to access them.
15. What is the difference between a constructor and a method in Java?
A constructor is a special method that is used to initialize objects. It has the same name as the class and no return type. A method, on the other hand, performs a specific task and can have a return type. Methods can be invoked multiple times, while constructors are called only once when the object is created.
16. What is a static block in Java?
A static block in Java is a block of code inside a class that is executed when the class is first loaded into memory. It is used to initialize static variables or execute any required logic at the time of class loading before the main method or constructor is called.
17. What is a constructor in Java?
A constructor in Java is a special method that is called when an object is created. It initializes the object. Constructors have the same name as the class and do not have a return type.
18. What is the difference between '== operator' and 'equals() method' in Java?
The == the operator compares the references of two objects, while the equals() method compares the contents of the objects.
19. What is multithreading in Java?
Multithreading in Java is a process of executing multiple threads simultaneously. Threads are lightweight processes, and Java provides built-in support for multithreaded programming. This allows for improved application performance and responsiveness.
20. What are the exceptions in Java?
Exceptions in Javaare unwanted or unexpected events that disrupt the normal flow of a program. Java provides exception-handling mechanisms using try-catch
blocks and other constructs. Handling exceptions ensures that the program can continue executing or terminate gracefully.
Top 15 Core Java Interview Questions and Answers For Intermediate Learners
21. What is the difference between the ArrayList and LinkedList in Java?
ArrayList and LinkedList are two implementations of the List interface. ArrayList Uses a dynamic array for storage, providing faster access times but slower insertion and deletion. In contrast, LinkedList uses a doubly linked list structure, offering faster insertions and deletions at the cost of slower access times.
Example
import java.util.ArrayList;
import java.util.LinkedList;
public class Main {
public static void main(String[] args) {
// Using ArrayList
ArrayList arrayList = new ArrayList<>();
arrayList.add("Apple");
arrayList.add("Banana");
arrayList.add("Cherry");
// Using LinkedList
LinkedList linkedList = new LinkedList<>();
linkedList.add("Dog");
linkedList.add("Cat");
linkedList.add("Horse");
System.out.println("ArrayList: " + arrayList);
System.out.println("LinkedList: " + linkedList);
}
}
Output
ArrayList: [Apple, Banana, Cherry]
LinkedList: [Dog, Cat, Horse]
Explanation
- This example shows how to create and use an
ArrayList
and aLinkedList
in Java. Both are initialized, and elements are added. - The output demonstrates that both lists store their elements, but they use different underlying data structures.
22. What is the Java Collections Framework?
The Java Collections Framework is a unified architecture for managing and manipulating collections of objects. It provides interfaces (like List, Set, Map), implementations (like ArrayList, HashSet, HashMap), and algorithms for operations like sorting and searching.
23. What is the difference between HashMap and Hashtable?
HashMap in Java is unsynchronized and allows null keys and values, making it suitable for non-threaded applications.Hashtable in Java is synchronized, thread-safe, and does not allow null keys or values, making it slower but safer for concurrent use.
Example
import java.util.HashMap;
import java.util.Hashtable;
public class Main {
public static void main(String[] args) {
HashMap hashMap = new HashMap<>();
hashMap.put(null, "Value1");
hashMap.put("Key1", null);
Hashtable hashTable = new Hashtable<>();
// Uncommenting the following lines will throw NullPointerException
// hashTable.put(null, "Value1");
// hashTable.put("Key1", null);
System.out.println("HashMap: " + hashMap);
System.out.println("Hashtable: " + hashTable);
}
}
Output
HashMap: {null=Value1, Key1=null}
Hashtable: {}
Explanation
- This example illustrates the differences between
HashMap
andHashtable
. TheHashMap
allows null keys and values whileHashtable
does not. - By attempting to add a null key or value to a
Hashtable
, we demonstrate the limitations imposed by its design.
24. What is a thread pool in Java?
A thread pool is a collection of pre-initialized threads that can be reused to execute multiple tasks. This helps reduce the overhead of thread creation and destruction, improving performance in multi-threaded applications.
Example
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class Main {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(3);
for (int i = 0; i < 5; i++) {
final int taskId = i;
executor.submit(() -> {
System.out.println("Task " + taskId + " is running in thread: " + Thread.currentThread().getName());
});
}
executor.shutdown();
}
}
Output
Task 0 is running in thread: pool-1-thread-1
Task 1 is running in thread: pool-1-thread-2
Task 2 is running in thread: pool-1-thread-3
Task 3 is running in thread: pool-1-thread-1
Task 4 is running in thread: pool-1-thread-2
Explanation
- This example demonstrates the creation of a thread pool using
Executors.newFixedThreadPool()
. We submit multiple tasks to the pool. - The output shows that the tasks are executed using a limited number of threads, allowing for efficient resource management.
25. What is the difference between final, finally, and finalize in Java?
final
is a keyword used to declare constants, prevent method overriding, and stop inheritance. finally
is a block that executes after a try-catch block in Java, regardless of whether an exception was thrown. finalize()
is a method called by the garbage collector before an object is reclaimed.
Example
class FinalExample {
final int CONSTANT = 10; // final variable
void method() {
try {
System.out.println("Trying...");
int result = 10 / 0; // This will throw an exception
} catch (ArithmeticException e) {
System.out.println("Caught an exception: " + e.getMessage());
} finally {
System.out.println("Finally block executed.");
}
}
@Override
protected void finalize() throws Throwable {
System.out.println("Finalize method called.");
super.finalize();
}
}
public class Main {
public static void main(String[] args) {
FinalExample example = new FinalExample();
example.method();
example = null; // Set to null for garbage collection
System.gc(); // Suggest garbage collection
}
}
Output
Trying...
Caught an exception: / by zero
Finally block executed.
Finalize method called.
Explanation
- This example showcases the differences between
final
,finally
, andfinalize()
. - The
method()
demonstrates exception handling with afinally
block that executes regardless of an exception. - The
finalize()
method is called when the object is garbage collected, showing that it provides a final opportunity to release resources.
26. What is the purpose of the 'synchronized' keyword in Java?
The synchronized
keyword is used to restrict access to a method or block to only one thread at a time. This ensures that critical sections of code are executed by only one thread, preventing race conditions and ensuring thread safety.
Example
class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public int getCount() {
return count;
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
Counter counter = new Counter();
Thread t1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
counter.increment();
}
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
counter.increment();
}
});
t1.start();
t2.start();
t1.join();
t2.join();
System.out.println("Final Count: " + counter.getCount());
}
}
Output
Final Count: 2000
Explanation
- This example demonstrates how the
synchronized
the keyword is used to ensure that theincrement()
method is accessed by only one thread at a time. - Two threads increment the counter simultaneously, and the synchronized method guarantees the final count is correct.
27. What is an abstract class in Java?
An abstract class in Javais a class that cannot be instantiated on its own. It may contain abstract methods (without implementation) that subclasses must implement. Abstract classes provide a way to define a common interface for subclasses, allowing shared functionality.
Example
abstract class Animal {
abstract void sound(); // Abstract method
}
class Cat extends Animal {
void sound() {
System.out.println("Cat meows");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Dog barks");
}
}
public class Main {
public static void main(String[] args) {
Animal myCat = new Cat();
Animal myDog = new Dog();
myCat.sound();
myDog.sound();
}
}
Output
Cat meows
Dog barks
Explanation
- This example demonstrates the use of an abstract class
Animal
with an abstract methodsound()
. The subclassesCat
andDog
provide implementations for thesound()
method. - The
main
method creates instances ofCat
andDog
and calls their respectivesound()
methods.
28. What is an interface in Java?
An interface in Java is a reference type that defines a contract that classes can implement. It can contain abstract methods, default methods, static methods, and constants. Classes that implement an interface must provide implementations for all its abstract methods in Java.
Example
interface Animal {
void sound(); // Abstract method
}
class Cat implements Animal {
public void sound() {
System.out.println("Cat meows");
}
}
class Dog implements Animal {
public void sound() {
System.out.println("Dog barks");
}
}
public class Main {
public static void main(String[] args) {
Animal myCat = new Cat();
Animal myDog = new Dog();
myCat.sound();
myDog.sound();
}
}
Output
Cat meows
Dog barks
Explanation
- This example demonstrates how an interface
Animal
is defined with an abstract methodsound()
. The classesCat
andDog
implement this interface. - The
main
method creates instances ofCat
andDog
and calls theirsound()
methods.
29. What is the difference between 'throw' and 'throws' in Java?
throw
is used to explicitly throw an exception from a method or block of code, while throws
is used in a method declaration to specify that the method can throw exceptions. It informs the caller about the potential exceptions that may occur.
Example
public class Main {
public static void checkAge(int age) throws IllegalArgumentException {
if (age < 18) {
throw new IllegalArgumentException("Age must be at least 18.");
}
System.out.println("You are eligible.");
}
public static void main(String[] args) {
try {
checkAge(16); // This will throw an exception
} catch (IllegalArgumentException e) {
System.out.println("Caught an exception: " + e.getMessage());
}
}
}
Output
Caught an exception: Age must be at least 18.
Explanation
- This example shows how to use
throw
to explicitly throw an exception if the provided age is less than 18. - The
checkAge
method declares that it can throw anIllegalArgumentException
, and themain
method handles it using a try-catch block.
30. What is the Singleton design pattern?
The Singleton design pattern ensures that a class has only one instance and provides a global point of access to it. It is useful for managing shared resources or configurations. This can be implemented using private constructors and a static method to get the instance.
Example
class Singleton {
private static Singleton instance;
private Singleton() {} // Private constructor
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
public class Main {
public static void main(String[] args) {
Singleton singleton1 = Singleton.getInstance();
Singleton singleton2 = Singleton.getInstance();
System.out.println("Singleton Instance 1: " + singleton1);
System.out.println("Singleton Instance 2: " + singleton2);
System.out.println("Are both instances the same? " + (singleton1 == singleton2));
}
}
Output
Singleton Instance 1: Singleton@1a2b3c4
Singleton Instance 2: Singleton@1a2b3c4
Are both instances the same? true
Explanation
- This example demonstrates the Singleton pattern by ensuring that only one instance of the
Singleton
the class can be created. - Both
singleton1
andsingleton2
point to the same instance, confirming the Singleton behavior.
31. What is dependency injection in Java?
Dependency Injection (DI) is a design pattern used to implement IoC (Inversion of Control), allowing a class to receive its dependencies from an external source rather than creating them itself. This promotes loose coupling and easier testing.
Example
class Engine {
public void start() {
System.out.println("Engine started.");
}
}
class Car {
private Engine engine;
// Constructor injection
public Car(Engine engine) {
this.engine = engine;
}
public void drive() {
engine.start();
System.out.println("Car is driving.");
}
}
public class Main {
public static void main(String[] args) {
Engine engine = new Engine();
Car car = new Car(engine);
car.drive();
}
}
Output
Engine started.
Car is driving.
Explanation
- This example demonstrates dependency injection through constructor injection, where the
Car
class receives anEngine
instance. - This approach decouples the classes, allowing for easier testing and maintenance.
32. What is Java Reflection?
Java Reflection is an API that allows programs to inspect and manipulate classes, methods, and fields at runtime. It provides the ability to retrieve metadata about classes and can be used to create instances, invoke methods, and access fields dynamically.
Example
import java.lang.reflect.Method;
class Sample {
public void display() {
System.out.println("Display method called.");
}
}
public class Main {
public static void main(String[] args) {
try {
// Create an instance of Sample
Sample sample = new Sample();
// Use reflection to get the 'display' method from Sample class
Method method = Sample.class.getMethod("display");
// Invoke the 'display' method using reflection
method.invoke(sample);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output
Display method called.
Explanation
- This example demonstrates the use of Java Reflection to invoke a method dynamically.
- The
Method
class is used to retrieve thedisplay()
method, which is then invoked at runtime.
33. What is the purpose of the 'volatile' keyword in Java?
The volatile
keyword is used to indicate that different threads may change a variable's value. It ensures that the value of the variable is always read from main memory rather than from thread-local memory, providing visibility guarantees across threads.
Example
class VolatileExample {
private volatile boolean flag = false;
public void writer() {
flag = true; // Set flag to true
}
public void reader() {
if (flag) {
System.out.println("Flag is set to true.");
}
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
VolatileExample example = new VolatileExample();
// Writer Thread (sets flag to true)
Thread writerThread = new Thread(() -> {
example.writer();
});
// Reader Thread (checks if flag is true)
Thread readerThread = new Thread(() -> {
try {
// Add a short delay to simulate race conditions
Thread.sleep(100);
example.reader();
} catch (InterruptedException e) {
e.printStackTrace();
}
});
// Start both threads
readerThread.start();
writerThread.start();
// Join both threads to ensure they complete before the program exits
writerThread.join();
readerThread.join();
}
}
Output
Flag is set to true.
Explanation
- This example demonstrates the use of the
volatile
keyword to ensure that changes made by one thread are visible to other threads. - The
writer()
method sets theflag
, and thereader()
method checks the flag's value, showcasing thread visibility.
34. What are Java annotations?
Java annotations are metadata that provide data about a program but are not part of the program itself. They can be used for various purposes, such as providing information to the compiler, runtime processing, or for configuration in frameworks like Spring.
Example
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
// Define the custom annotation with runtime retention
@Retention(RetentionPolicy.RUNTIME)
@interface MyAnnotation {
String value();
}
@MyAnnotation(value = "This is a custom annotation")
public class Main {
public static void main(String[] args) {
// Get the annotation applied to the Main class
MyAnnotation annotation = Main.class.getAnnotation(MyAnnotation.class);
// Check if the annotation is present and then print the value
if (annotation != null) {
System.out.println("Annotation Value: " + annotation.value());
} else {
System.out.println("Annotation not found.");
}
}
}
Output
Annotation Value: This is a custom annotation
Explanation
- This example defines a custom annotation
MyAnnotation
and applies it to theMain
class. - The annotation's value is retrieved and displayed, demonstrating how annotations can be used for metadata.
35. What is the Observer design pattern?
The Observer design pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. This is often used in event-handling systems.
Example
import java.util.ArrayList;
import java.util.List;
// Observer interface
interface Observer {
void update(String message);
}
// Concrete implementation of Observer
class ConcreteObserver implements Observer {
private String name;
public ConcreteObserver(String name) {
this.name = name;
}
@Override
public void update(String message) {
System.out.println(name + " received: " + message);
}
}
// Subject class managing observers
class Subject {
private List observers = new ArrayList<>();
// Method to attach observers
public void attach(Observer observer) {
observers.add(observer);
}
// Notify all observers about the message
public void notifyObservers(String message) {
for (Observer observer : observers) {
observer.update(message);
}
}
}
// Main class to demonstrate the Observer pattern
public class Main {
public static void main(String[] args) {
Subject subject = new Subject();
// Creating observers
Observer observer1 = new ConcreteObserver("Observer 1");
Observer observer2 = new ConcreteObserver("Observer 2");
// Attaching observers to the subject
subject.attach(observer1);
subject.attach(observer2);
// Notifying observers with a message
subject.notifyObservers("Hello Observers!");
}
}
Output
Observer 1 received: Hello Observers!
Observer 2 received: Hello Observers!
Explanation
- This example demonstrates the Observer pattern by creating a
Subject
that maintains a list of observers. - When the
notifyObservers
method is called, and all attached observers are notified with the provided message.
Top 15 Core Java Interview Questions and Answers For Experienced
36. What is the difference between `==` and `equals()` in Java?
The ==
operator checks for reference equality, meaning it checks if two references point to the same object in memory. The equals()
method checks for value equality, meaning it checks if two objects are logically equivalent based on their attributes.
Example
class Person {
String name;
Person(String name) {
this.name = name;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Person person = (Person) obj;
return name.equals(person.name);
}
}
public class Main {
public static void main(String[] args) {
Person person1 = new Person("Alice");
Person person2 = new Person("Alice");
System.out.println("Using ==: " + (person1 == person2)); // false
System.out.println("Using equals(): " + person1.equals(person2)); // true
}
}
Output
Using ==: false
Using equals(): true
Explanation
- This example shows how
==
compares references whileequals()
compares the values of thename
attribute.
37. What is a Java `try-with-resources` statement?
The try-with-resources
statement is a feature introduced in Java 7 that allows you to declare one or more resources (such as files) that need to be closed after the program is finished using them. It ensures that each resource is closed at the end of the statement, which helps to prevent resource leaks.
Example
import java.io.BufferedReader;
import java.io.IOException;
import java.io.StringReader;
public class Main {
public static void main(String[] args) {
// Simulating the content of "file.txt" as a string
String simulatedFileContent = "This is line 1\nThis is line 2\nThis is line 3";
// Using StringReader to simulate reading from a file
try (BufferedReader br = new BufferedReader(new StringReader(simulatedFileContent))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line); // Same output as reading from file.txt
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output
// Contents of file.txt will be printed line by line.
Explanation
- This example demonstrates the use of the
try-with-resources
statement to automatically close theBufferedReader
after usage. - It eliminates the need for explicit
finally
blocks to close resources, thus simplifying the code.
38. What are the main principles of Object-Oriented Programming (OOP) in Java?
The main principles of OOP in Java are:
- Encapsulation:Bundling the data (attributes) and methods (functions) that operate on the data into a single unit or class and restricting access to some of the object's components.
- Inheritance: Mechanism by which one class can inherit fields and methods from another class, promoting code reuse.
- Polymorphism:The ability of different classes to be treated as instances of the same class through a common interface, allowing for method overriding and overloading.
- Abstraction: Hiding the complex implementation details and showing only the essential features of the object.
Example
// Encapsulation
class Vehicle {
private String brand;
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
}
// Inheritance
class Car extends Vehicle {
public void honk() {
System.out.println("Car honks!");
}
}
// Polymorphism
class Main {
public static void main(String[] args) {
Vehicle myCar = new Car();
myCar.setBrand("Toyota");
System.out.println("Brand: " + myCar.getBrand());
((Car) myCar).honk();
}
}
Output
Brand: Toyota
Car honks!
Explanation
- This example illustrates encapsulation with the
Vehicle
class, inheritance with theCar
class, and polymorphism by treating aCar
instance as aVehicle
.
39. What are Java annotations, and how are they used?
Java annotations are metadata that provide information about a program but are not part of the program itself. They can be used for various purposes, such as providing instructions to the compiler or runtime environment or for frameworks that process them to provide additional functionality.
Example
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
@Retention(RetentionPolicy.RUNTIME)
@interface MyAnnotation {
String value();
}
@MyAnnotation(value = "Hello Annotation")
class AnnotatedClass {
public void display() {
System.out.println("Annotated Class");
}
}
public class Main {
public static void main(String[] args) {
MyAnnotation annotation = AnnotatedClass.class.getAnnotation(MyAnnotation.class);
System.out.println("Annotation value: " + annotation.value());
}
}
Output
Annotation value: Hello Annotation
Explanation
- This example defines a custom annotation
MyAnnotation
and uses it to annotate a class. - At runtime, the annotation is retrieved, demonstrating how annotations can be processed.
40. What is the purpose of the `final` keyword in Java?
The final
keyword is used to restrict the user. It can be applied to variables, methods, and classes:
- If a variable is declared as
final
its value, it cannot be changed after initialization. - If a method is declared as
final
it cannot be overridden by subclasses. - If a class is declared as
final
it cannot be subclassed.
Example
final class FinalClass {
final int value;
FinalClass(int value) {
this.value = value;
}
final void display() {
System.out.println("Value: " + value);
}
}
public class Main {
public static void main(String[] args) {
FinalClass obj = new FinalClass(10);
obj.display();
}
}
Output
Value: 10
Explanation
- This example demonstrates the use of the
final
keyword by creating a final class with a final method and variable.
41. How does Java handle memory management and garbage collection?
Java manages memory through automatic garbage collection, which frees up memory by removing objects that are no longer reachable. The garbage collector (GC) runs in the background, identifying and disposing of these objects to reclaim memory.
Example
public class Main {
public static void main(String[] args) {
// Creating objects
for (int i = 0; i < 10000; i++) {
new Object();
}
// Suggesting garbage collection
System.gc();
System.out.println("Garbage collection suggested.");
}
}
Output
Garbage collection suggested.
Explanation
- This example illustrates that the Java runtime environment can create many objects and the
System.gc()
method suggests to the JVM that it should run the garbage collector. - Garbage collection is automatic and does not guarantee immediate cleanup of unreachable objects.
42. What is the Java Collections Framework?
The Java Collections Framework is a unified architecture for representing and manipulating collections of objects. It includes interfaces, implementations (classes), and algorithms for working with data structures like lists, sets, maps, and more.
Example
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
for (String fruit : list) {
System.out.println(fruit);
}
}
}
Output
Apple
Banana
Cherry
Explanation
- This example demonstrates the use of the Java Collections Framework by creating a list of fruits and iterating through it.
43. What is the difference between `ArrayList` and `LinkedList` in Java?
ArrayList
and LinkedList
are two commonly used implementations of the List
interface. The main differences are:
- Storage:
ArrayList
Uses a dynamic array to store elements whileLinkedList
using a doubly linked list. - Access Time:
ArrayList
provides fast random access (O(1)) to elements, whereasLinkedList
provides slower access (O(n)). - Insertion/Deletion:
LinkedList
is more efficient for insertions and deletions (O(1)) as it only requires updating references, whileArrayList
can be slower (O(n)) due to the need to shift elements.
Example
import java.util.ArrayList;
import java.util.LinkedList;
public class Main {
public static void main(String[] args) {
ArrayList arrayList = new ArrayList<>();
LinkedList linkedList = new LinkedList<>();
// Adding elements
arrayList.add("Apple");
linkedList.add("Banana");
// Accessing elements
System.out.println("ArrayList first element: " + arrayList.get(0));
System.out.println("LinkedList first element: " + linkedList.get(0));
}
}
Output
ArrayList first element: Apple
LinkedList first element: Banana
Explanation
- This example demonstrates the differences between
ArrayList
andLinkedList
by showcasing their methods and behaviors.
44. What is the purpose of the `synchronized` keyword in Java?
The synchronized
keyword is used to control access to a method or block of code by multiple threads. It ensures that only one thread can access the synchronized method or block at a time, preventing race conditions and maintaining thread safety.
Example
class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public int getCount() {
return count;
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
Counter counter = new Counter();
Thread t1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) counter.increment();
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) counter.increment();
});
t1.start();
t2.start();
t1.join();
t2.join();
System.out.println("Final count: " + counter.getCount());
}
}
Output
Final count: 2000
Explanation
- This example demonstrates how the
synchronized
keyword is used to prevent race conditions by ensuring that only one thread can increment the counter at a time.
45. What are lambda expressions in Java?
Lambda expressions in Javaare a feature introduced in Java 8 that provides a clear and concise way to represent one method interface using an expression. They allow you to implement functional interfaces in a more readable way.
Example
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
List names = Arrays.asList("Alice", "Bob", "Charlie");
// Using a lambda expression
names.forEach(name -> System.out.println(name));
}
}
Output
Alice
Bob
Charlie
Explanation
- This example demonstrates the use of a lambda expression with the
forEach
method to iterate over a list of names and print them.
46. What is the Stream API in Java?
The Stream API, introduced in Java 8, allows for functional-style operations on collections of objects. It provides a way to process sequences of elements (e.g., lists) in a more declarative manner, enabling operations like filtering, mapping, and reducing.
Example
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
List names = Arrays.asList("Alice", "Bob", "Charlie");
// Using Stream API to filter names starting with 'A'
names.stream()
.filter(name -> name.startsWith("A"))
.forEach(System.out::println);
}
}
Output
Alice
Explanation
- This example demonstrates how the Stream API is used to filter and print names starting with the letter 'A' from a list.
47. What is the purpose of the `volatile` keyword in Java?
The volatile
keyword is used to indicate that a variable's value may be changed by different threads. It ensures that the value of the variable is always read from main memory rather than from thread-local memory, providing visibility guarantees across threads.
Example
class VolatileExample {
private volatile boolean flag = false;
public void writer() {
flag = true; // Set flag to true
}
public void reader() {
if (flag) {
System.out.println("Flag is set to true.");
}
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
VolatileExample example = new VolatileExample();
Thread writerThread = new Thread(example::writer);
Thread readerThread = new Thread(example::reader);
readerThread.start();
writerThread.start();
writerThread.join();
readerThread.join();
}
}
Output
Flag is set to true.
Explanation
- This example demonstrates the use of the
volatile
keyword to ensure that changes made by one thread are visible to other threads. - The
writer()
method sets theflag
, and thereader()
method checks the flag's value, showcasing thread visibility.
48. What is a Java Future, and how is it used?
A Future
represents the result of an asynchronous computation in Java. It provides methods to check if the computation is complete, to wait for its completion, and to retrieve the result of the computation.
Example
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class Main {
public static void main(String[] args) {
ExecutorService executor = Executors.newSingleThreadExecutor();
Future future = executor.submit(new Callable() {
@Override
public Integer call() throws Exception {
Thread.sleep(2000); // Simulate long computation
return 42;
}
});
try {
// Waiting for the result
Integer result = future.get();
System.out.println("Result: " + result);
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
} finally {
executor.shutdown();
}
}
}
Output
Result: 42
Explanation
- This example demonstrates how to use a
Future
to submit a task for execution and retrieve its result after waiting for completion. - The
call()
method simulates a long computation, and the main thread waits for the result usingfuture.get()
.
49. What is the difference between `HashMap` and `Hashtable` in Java?
HashMap
and Hashtable
are both implementations of the Map
interface. The main differences are:
- Synchronization:
Hashtable
is synchronized and thread-safe, whileHashMap
is not, makingHashMap
more suitable for non-threaded applications. - Null Values:
HashMap
allows one null key and multiple null values, whereasHashtable
does not allow null keys or values. - Performance:
HashMap
Generally, it performs better due to the lack of synchronization.
Example
import java.util.HashMap;
import java.util.Hashtable;
public class Main {
public static void main(String[] args) {
HashMap hashMap = new HashMap<>();
Hashtable hashTable = new Hashtable<>();
// Adding elements
hashMap.put(null, "Value1");
hashTable.put("Key1", "Value2");
System.out.println("HashMap allows null key: " + hashMap.get(null));
// System.out.println("Hashtable allows null key: " + hashTable.get(null)); // Uncommenting this line will throw NullPointerException
}
}
Output
HashMap allows null key: Value1
Explanation
- This example highlights the differences between
HashMap
andHashtable
regarding null keys and synchronization.
50. What is the purpose of the `try-with-resources` statement in Java?
The try-with-resources
statement, introduced in Java 7, ensures that each resource is closed at the end of the statement. It is used to manage resources like files and sockets automatically, making code cleaner and less error-prone.
Example
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output
// Output depends on the contents of file.txt
Explanation
- This example demonstrates the use of the
try-with-resources
statement to automatically close theBufferedReader
resource after use, which helps to prevent resource leaks.
Summary
Core Java Interview Questions are important for assessing your understanding of Java basics. The article includes a list of 50 frequently asked questions that include important subjects, including inheritance, exception handling, and multithreading. Designed for both beginners and seasoned experts, it boosts confidence and guarantees you're ready for real-world programming problems. Whether you have a few or many years of experience, this approach will help you nail your next technical interview.To master Java concepts, enroll inScholarhat'sJava Free Certification Course.
To enhance your preparation further and gain certification, consider enrolling in our Java Online Course Free With Certificate, which provides in-depth learning and certification to boost your career prospects. Let’s jump in!
Q 1: What is the default value of a boolean variable in Java?
- (a) true
- (b) false
- (c) null
- (d) 0
Q 2: Which of the following is not a valid access modifier in Java?
- (a) public
- (b) private
- (c) protected
- (d) internal
Q 3: Which method is used to start a thread in Java?
- (a) run()
- (b) start()
- (c) init()
- (d) execute()
Q 4: Which of the following is not a primitive data type in Java?
- (a) int
- (b) char
- (c) String
- (d) boolean
Q 5: What will be the output of the following code?
int x = 5;
System.out.println(x++);
System.out.println(x);
- (a) 5 6
- (b) 6 6
- (c) 5 5
- (d) Compilation error
Download this PDF Now - Core Java Interview Questions PDF By ScholarHat |
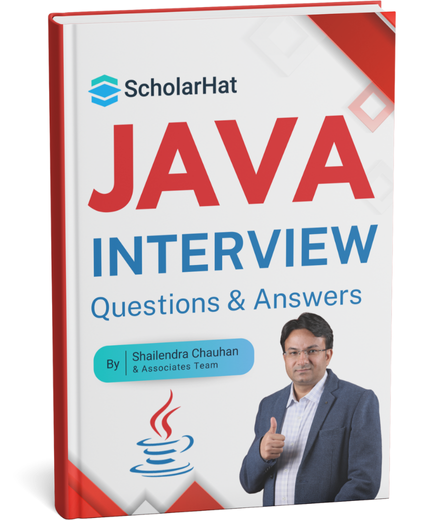
Crack Your Next Java Interview – Grab the Free Expert eBook!
Java Interview Questions and Answers Book Unlock expert-level Java interview preparation with our exclusive eBook! Get instant access to a curated collection of real-world interview questions, detailed answers, and professional insights — all designed to help you succeed.
No downloads needed — just quick, free access to the ultimate guide for Java interviews. Start your preparation today and move one step closer to your dream job!.
FAQs
Take our Java skill challenge to evaluate yourself!
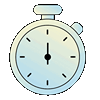
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.