11
JulTop Java Collections Interview Questions with Answers
Collections in Java Interview Questions
If you're preparing for a Java interview, having a solid grasp of Java Collections is crucial. You may be asked to explain various collection types, their properties, and code implementations using the Collections Framework in Java. Being familiar with Lists, Sets, Maps, and queues, as well as understanding performance and thread safety, is beneficial.
In this interview tutorial, we’ll review the top Java Collections interview questions with clear examples and explanations. By the end, you’ll be equipped to confidently address any collections-related question and apply these concepts in practical scenarios.
What to Expect in Java Collections Interview Questions
Java Collections interview questions test your knowledge of the Collections Framework and its real-world applications. You might encounter questions about the different types of collections (Lists, Sets, Maps), their specific use cases, and performance considerations. Interviewers may ask you to compare collections, explain synchronization and thread safety, or write code for data manipulation. Being ready to tackle questions on both basic concepts and tricky scenarios will help you stand out, regardless of your experience level.
Java Collections Interview Questions for Freshers
In this we a re gonna see
1. What is the Java Collections Framework?
Ans: The Java Collections Framework is like a toolkit that provides a set of classes and interfaces to manage groups of data. It includes common data structures like List
, Set
, Map
, and concrete classes like ArrayList
, HashSet
, and HashMap
. This framework makes it easier to store, access, and manipulate data efficiently in Java.
2. What are the main benefits of using collections in Java?
Ans: Collections in Java are incredibly useful for several reasons:
- They help you handle groups of data efficiently.
- They boost performance and offer flexibility in how you manage your data.
- With standardized data structures and interfaces, collections make it easy to work with data in a predictable way.
- They save you time by providing built-in methods for common tasks like sorting, searching, and filtering.
3. What is the difference between Collection and Collections in Java?
Ans: Collection
is an interface in the Java Collections Framework. It represents a group of objects and is the root interface for most collection types. On the other hand, Collections
is a utility class that provides a bunch of static methods you can use to operate on collections, such as sorting, reversing, or searching.
4. What is the difference between List and Set in Java?
Ans: Here’s how List
and Set
differ:
- List: A
List
allows duplicate elements and keeps them in the order in which they were added. Think of it as a shopping list where you can have multiple entries of the same item. Common examples areArrayList
andLinkedList
. - Set: A
Set
doesn’t allow duplicates, meaning each element must be unique. Depending on the implementation, it might or might not maintain insertion order. Examples includeHashSet
andTreeSet
.
Read More: Linked List in Data Structures |
5. How does ConcurrentHashMap ensure thread safety, and how is it different from Hashtable?
Ans:ConcurrentHashMap ensures thread safety by dividing its data into segments (or buckets). You can imagine it as multiple lockers where different threads can access different lockers at the same time without interfering with each other. This allows faster performance in multi-threaded environments compared to locking the entire map.
In contrast, Hashtable locks the entire structure for every read or write, which can slow things down when many threads are involved. So, ConcurrentHashMap is like multiple people accessing separate lockers, while Hashtable is like one person using a single locker room at a time.
6. What is the difference between HashSet and TreeSet?
Ans: Here’s what makes HashSet
and TreeSet
different:
- HashSet: It doesn’t preserve any order of elements. Operations like add, remove, and contains are usually very fast, typically taking constant time.
- TreeSet: Unlike
HashSet
, aTreeSet
keeps its elements sorted (either in natural order or according to a custom comparator). It provides slower performance for basic operations, but it guarantees that the elements will always be in order.
Read More: Differences Between Arrays and Linked Lists |
7. What is the purpose of the Map interface?
Ans: Themapinterface in Javais allabout storing key-value pairs. Each key is unique, and it maps to a single value. This is ideal when you need to store data that you’ll access by a unique identifier, like a dictionary where the word is the key and the definition is the value. Common implementations include HashMap
and TreeMap
.
8. What is the difference between HashMap and Hashtable?
Ans: Here’s how HashMap
and Hashtable
different from each other :
- HashMap:HashMap in Java because it doesn’t synchronize its methods, meaning it can be accessed by multiple threads without waiting. It also allows
null
keys and values. - Hashtable: It’s older and slower than the other
HashMap
because it synchronizes all its methods to make it thread-safe. However, this means you can’t usenull
it for keys or values.
Read More: Hash Table in Data Structures |
9. How is a LinkedHashMap different from a HashMap?
Ans: The big difference here is that LinkedHashMap
it maintains the order in which elements were inserted, unlike HashMap
, which doesn’t guarantee any specific order. This is made possible by adding a doubly-linked list to the map, which keeps track of the insertion order.
10. What is the difference between Iterator and ListIterator?
Ans: Let’s compare Iterator
and ListIterator
:
- Iterator: This is a more general-purpose interface that works with all types of collections. It only allows forward traversal of elements.
- ListIterator: This one is more powerful but works only with lists. It allows both forward and backward traversal and also provides extra features, like the ability to modify elements during iteration.
11. What are the key differences between an Array and an ArrayList?
Ans: An Array
is a fixed-size data structure, whereas an ArrayList
is a dynamic data structure that can grow or shrink as needed.
import java.util.ArrayList;
public class ArrayVsArrayList {
public static void main(String[] args) {
// Using an Array
int[] array = {1, 2, 3};
System.out.println("Array: ");
for (int num : array) {
System.out.print(num + " ");
}
// Using an ArrayList
ArrayList arrayList = new ArrayList<>();
arrayList.add(1);
arrayList.add(2);
arrayList.add(3);
System.out.println("\nArrayList: " + arrayList);
}
}
Output
Array: 1 2 3
ArrayList: [1, 2, 3]
Explanation
This example demonstrates the key differences:Java Arrays have a fixed size, while an ArrayList
can grow dynamically.
12. Explain the purpose of the Collections utility class.
Ans: The Collections
utility class provides static methods to operate on or return collections, such as sorting, reversing, shuffling, and more.
import java.util.ArrayList;
import java.util.Collections;
public class CollectionsExample {
public static void main(String[] args) {
ArrayList list = new ArrayList<>();
list.add(3);
list.add(1);
list.add(2);
// Sorting the list
Collections.sort(list);
System.out.println("Sorted List: " + list);
// Shuffling the list
Collections.shuffle(list);
System.out.println("Shuffled List: " + list);
}
}
Output
Sorted List: [1, 2, 3]
Shuffled List: [2, 1, 3]
Explanation
The Collections.sort()
method is used to sort the list and Collections.shuffle()
randomly shuffles the elements in the list.
13. How does the add() method work in Java Collections?
Ans: The add()
method in Java Collections adds an element to the collection. It returns true
if the collection changed as a result of the call.
import java.util.ArrayList;
public class AddMethodExample {
public static void main(String[] args) {
ArrayList list = new ArrayList<>();
list.add("apple");
list.add("banana");
System.out.println("ArrayList: " + list);
}
}
Output
ArrayList: [apple, banana]
Explanation
The add()
method adds elements to an ArrayList. In this case, "apple" and "banana" were added to the list.
14. What is the difference between the remove() and clear() methods in a Collection?
Ans: The remove()
method removes a specific element while clear()
removes all elements from the collection.
import java.util.ArrayList;
public class RemoveVsClear {
public static void main(String[] args) {
ArrayList list = new ArrayList<>();
list.add("apple");
list.add("banana");
list.add("cherry");
// Removing "banana"
list.remove("banana");
System.out.println("After remove: " + list);
// Clearing the list
list.clear();
System.out.println("After clear: " + list);
}
}
Output
After remove: [apple, cherry]
After clear: []
Explanation
The remove()
method removes a specific element and clear()
empties the entire list.
15. What is the difference between HashMap and TreeMap in Java?
Ans: A HashMap
stores elements in an unordered fashion and does not guarantee any specific order of elements, while a TreeMap
stores elements in a sorted order, according to the natural ordering or a comparator.
import java.util.HashMap;
import java.util.TreeMap;
public class MapExample {
public static void main(String[] args) {
// Using HashMap
HashMap<String, String> hashMap = new HashMap<>();
hashMap.put("A", "Apple");
hashMap.put("B", "Banana");
System.out.println("HashMap: " + hashMap);
// Using TreeMap
TreeMap<String, String> treeMap = new TreeMap<>();
treeMap.put("A", "Apple");
treeMap.put("B", "Banana");
System.out.println("TreeMap: " + treeMap);
}
}
Output
HashMap: {A=Apple, B=Banana}
TreeMap: {A=Apple, B=Banana}
Explanation
Although both store key-value pairs, a HashMap
does not guarantee any order, while a TreeMap
stores them in natural sorted order.
16. What are the differences between String, StringBuilder, and StringBuffer in Java?
Ans: The main difference between String
, StringBuilder
, and StringBuffer
is how they handle immutability and thread safety:
String
: Immutable, thread-safe.StringBuilder
: Mutable, not thread-safe.StringBuffer
: Mutable, thread-safe.
public class StringBuilderVsStringBuffer {
public static void main(String[] args) {
// Using String
String str = "Hello";
str = str + " World";
System.out.println("String: " + str);
// Using StringBuilder
StringBuilder sb = new StringBuilder("Hello");
sb.append(" World");
System.out.println("StringBuilder: " + sb);
// Using StringBuffer
StringBuffer sbf = new StringBuffer("Hello");
sbf.append(" World");
System.out.println("StringBuffer: " + sbf);
}
}
Output
String: Hello World
StringBuilder: Hello World
StringBuffer: Hello World
Explanation
StringBuilder
and StringBuffer
are mutable, allowing modifications, but String
is immutable. StringBuffer
is thread-safe, unlike StringBuilder
.
Read More: Java Strings: Operations and Methods |
17. What is the purpose of the transient keyword in Java?
Ans: The transient
keyword in Java is used to indicate that a field should not be serialized. When an object is serialized, transient fields are ignored, and their values are not saved.
import java.io.Serializable;
public class TransientExample implements Serializable {
transient int salary;
String name;
public TransientExample(String name, int salary) {
this.name = name;
this.salary = salary;
}
public String toString() {
return name + ": " + salary;
}
}
Explanation
The salary
field will not be serialized because it is marked as transient.
18. What is the difference between == and .equals() in Java?
Ans: The ==
operator checks for reference equality, i.e., whether two references point to the same object in memory. The .equals()
method checks for logical equality, i.e., whether two objects are logically equivalent based on their content.
public class EqualityCheck {
public static void main(String[] args) {
String str1 = "Hello";
String str2 = "Hello";
String str3 = new String("Hello");
// == checks reference equality
System.out.println("str1 == str2: " + (str1 == str2));
// .equals() checks value equality
System.out.println("str1.equals(str2): " + str1.equals(str2));
System.out.println("str1 == str3: " + (str1 == str3));
System.out.println("str1.equals(str3): " + str1.equals(str3));
}
}
Output
str1 == str2: true
str1.equals(str2): true
str1 == str3: false
str1.equals(str3): true
Explanation
The ==
operator compares object references while .equals()
compares the values within those objects.
Read More: Java Operators: Arithmetic, Relational, Logical, and More |
19. What is the difference between ArrayList and LinkedList in Java?
Ans: An ArrayList
uses a dynamic array to store elements, offering faster access but slower insertion/removal in the middle of the list. A LinkedList
uses a doubly linked list, offering faster insertion/removal but slower access time.
import java.util.ArrayList;
import java.util.LinkedList;
public class ListComparison {
public static void main(String[] args) {
ArrayList<String> arrayList = new ArrayList<>();
arrayList.add("apple");
arrayList.add("banana");
LinkedList<String> linkedList = new LinkedList<>();
linkedList.add("apple");
linkedList.add("banana");
System.out.println("ArrayList: " + arrayList);
System.out.println("LinkedList: " + linkedList);
}
}
Output
ArrayList: [apple, banana]
LinkedList: [apple, banana]
Explanation
Both ArrayList
and LinkedList
store the same data, but ArrayList
uses dynamic arrays and LinkedList
uses linked nodes.
Read More: Doubly Linked List in Data Structures |
20. What is an enum in Java?
Ans: An enum
is a special Java type used to define collections of constants. It provides a type-safe way to work with a fixed set of constants like days of the week, months, etc.
public enum Day {
SUNDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY
}
public class EnumExample {
public static void main(String[] args) {
Day day = Day.MONDAY;
System.out.println("Today is " + day);
}
}
Output
Today is MONDAY
Explanation
An enum
defines a set of constants, making the code more readable and type-safe compared to using plain constants.
21. How does HashMap work internally in Java?
Ans: A HashMap
works by using an array of buckets. Each bucket stores a list of entries (key-value pairs), and the key is hashed to determine the index. The hash function ensures efficient retrieval of values.
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
HashMap map = new HashMap<>();
map.put("apple", 1);
map.put("banana", 2);
map.put("cherry", 3);
System.out.println("HashMap: " + map);
}
}
Output
HashMap: {apple=1, banana=2, cherry=3}
Explanation
The HashMap
stores key-value pairs and uses a hash function to determine the index in the underlying array. This provides fast lookups and insertions.
Read More: Hashing in Data Structures |
22. What is the significance of the load factor in a HashMap?
Ans: The load factor determines when the HashMap should resize itself. It is the ratio of the number of elements to the current capacity. A higher load factor decreases memory usage but may degrade performance.
import java.util.HashMap;
public class LoadFactorExample {
public static void main(String[] args) {
// Creating a HashMap with initial capacity of 4 and load factor of 0.75
HashMap map = new HashMap<>(4, 0.75f);
map.put("apple", 1);
map.put("banana", 2);
map.put("cherry", 3);
System.out.println("HashMap: " + map);
}
}
Output
HashMap: {apple=1, banana=2, cherry=3}
Explanation
The load factor
determines how full the HashMap can get before it needs to resize. Here, a load factor of 0.75 means the HashMap will resize when 75% full.
23. What are the differences between ConcurrentHashMap and synchronized HashMap?
Ans: A ConcurrentHashMap
allows concurrent access to segments of the map, whereas a synchronized HashMap
locks the entire map during operations. ConcurrentHashMap
Provides better concurrency and scalability.
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentHashMapExample {
public static void main(String[] args) {
ConcurrentHashMap map = new ConcurrentHashMap<>();
map.put("apple", 1);
map.put("banana", 2);
map.put("cherry", 3);
System.out.println("ConcurrentHashMap: " + map);
}
}
Output
ConcurrentHashMap: {apple=1, banana=2, cherry=3}
Explanation
ConcurrentHashMap
provides higher concurrency by dividing the map into segments, allowing multiple threads to access different segments concurrently.
24. Explain the structure and usage of a LinkedHashSet.
Ans: A LinkedHashSet
is a collection that maintains insertion order while ensuring no duplicate elements. It combines the properties of a hash set and alinked list.
import java.util.LinkedHashSet;
public class LinkedHashSetExample {
public static void main(String[] args) {
LinkedHashSet set = new LinkedHashSet<>();
set.add("apple");
set.add("banana");
set.add("cherry");
System.out.println("LinkedHashSet: " + set);
}
}
Output
LinkedHashSet: [apple, banana, cherry]
Explanation
A LinkedHashSet
preserves the insertion order of elements while ensuring uniqueness, as shown in the example above.
25. How does a PriorityQueue work internally?
Ans: A PriorityQueue
Internally, it uses a heap data structure, which ensures that the element with the highest priority is always at the head of the queue.
import java.util.PriorityQueue;
public class PriorityQueueExample {
public static void main(String[] args) {
PriorityQueue queue = new PriorityQueue<>();
queue.add(10);
queue.add(5);
queue.add(20);
System.out.println("PriorityQueue: " + queue);
}
}
Output
PriorityQueue: [5, 10, 20]
Explanation
The PriorityQueue
automatically orders the elements based on their natural ordering (or a custom comparator), ensuring the smallest (or largest) element is always at the head.
Practice with these Articles: |
26. What is the difference between a WeakHashMap and a HashMap?
Ans: A WeakHashMap
stores weak references to its keys, meaning that if a key is no longer referenced elsewhere, it can be garbage collected. In contrast, a HashMap
keeps strong references to its keys.
import java.util.WeakHashMap;
public class WeakHashMapExample {
public static void main(String[] args) {
WeakHashMap map = new WeakHashMap<>();
String key = new String("apple");
map.put(key, 1);
System.out.println("WeakHashMap: " + map);
key = null; // Removing strong reference to key
System.gc(); // Request garbage collection
System.out.println("After GC: " + map);
}
}
Output
WeakHashMap: {apple=1}
After GC: {}
Explanation
The WeakHashMap
allows its keys to be collected as garbage when there are no strong references left. After garbage collection, the entry is removed from the map.
27. How is a CopyOnWriteArrayList different from an ArrayList?
Ans: A CopyOnWriteArrayList
creates a copy of the entire list on every write operation (add, set, remove), making it thread-safe for concurrent reads but less efficient for writes. In contrast, an ArrayList
allows for faster writes but is not thread-safe.
import java.util.concurrent.CopyOnWriteArrayList;
public class CopyOnWriteExample {
public static void main(String[] args) {
CopyOnWriteArrayList list = new CopyOnWriteArrayList<>();
list.add("apple");
list.add("banana");
// Writing to the list
list.add("cherry");
// Display the list
System.out.println("CopyOnWriteArrayList: " + list);
}
}
Output
CopyOnWriteArrayList: [apple, banana, cherry]
Explanation
The CopyOnWriteArrayList
is thread-safe and creates a copy of the list when it is modified, making it ideal for concurrent reads but inefficient for frequent writes.
28. What is a NavigableMap, and how is it different from a SortedMap?
Ans: A NavigableMap
is a subtype of SortedMap
that supports additional operations, such as retrieving the closest matches for given keys (e.g., lowerKey()
, higherKey()
, etc.). While both SortedMap
and NavigableMap
maintain sorting, and the latter provides more navigation methods.
import java.util.NavigableMap;
import java.util.TreeMap;
public class NavigableMapExample {
public static void main(String[] args) {
NavigableMap map = new TreeMap<>();
map.put(1, "apple");
map.put(2, "banana");
map.put(3, "cherry");
System.out.println("NavigableMap: " + map);
System.out.println("Lower Key: " + map.lowerKey(2));
}
}
Output
NavigableMap: {1=apple, 2=banana, 3=cherry}
Lower Key: 1
Explanation
The NavigableMap
provides methods like lowerKey()
and higherKey()
to navigate the map, which is not available in a basic SortedMap
.
29. How does the ConcurrentSkipListMap work internally?
Ans: The ConcurrentSkipListMap
is based on a skip list, a probabilistic data structure that allows for fast search, insertion, and deletion operations. It provides better concurrency compared to a TreeMap
by locking only portions of the map during updates.
import java.util.concurrent.ConcurrentSkipListMap;
public class ConcurrentSkipListMapExample {
public static void main(String[] args) {
ConcurrentSkipListMap map = new ConcurrentSkipListMap<>();
map.put(1, "apple");
map.put(2, "banana");
map.put(3, "cherry");
System.out.println("ConcurrentSkipListMap: " + map);
}
}
Output
ConcurrentSkipListMap: {1=apple, 2=banana, 3=cherry}
Explanation
The ConcurrentSkipListMap
supports concurrent access and modification, using a skip list for efficient searching, insertion, and deletion.
30. What is the difference between a TreeMap and a HashMap?
Ans: A TreeMap
stores key-value pairs in a sorted order (using the natural ordering or a comparator) and provides log(n) time complexity for operations, while a HashMap
stores key-value pairs in an unordered fashion and offers constant time complexity for most operations.
import java.util.TreeMap;
import java.util.HashMap;
public class TreeMapVsHashMap {
public static void main(String[] args) {
TreeMap treeMap = new TreeMap<>();
treeMap.put(1, "apple");
treeMap.put(3, "banana");
treeMap.put(2, "cherry");
HashMap hashMap = new HashMap<>();
hashMap.put(1, "apple");
hashMap.put(3, "banana");
hashMap.put(2, "cherry");
System.out.println("TreeMap: " + treeMap);
System.out.println("HashMap: " + hashMap);
}
}
Output
TreeMap: {1=apple, 2=cherry, 3=banana}
HashMap: {1=apple, 3=banana, 2=cherry}
Explanation
The TreeMap
maintains a sorted order based on keys, while the HashMap
does not guarantee any specific order of keys.
31. What is the difference between TreeSet and ConcurrentSkipListSet?
Ans: Both TreeSet
and ConcurrentSkipListSet
store elements in a sorted order. However, the key difference lies in concurrency. While TreeSet
is not thread-safe, ConcurrentSkipListSet
allows for safe concurrent access, using a skip list data structure to maintain order.
import java.util.concurrent.ConcurrentSkipListSet;
public class SetExample {
public static void main(String[] args) {
ConcurrentSkipListSet set = new ConcurrentSkipListSet<>();
set.add("apple");
set.add("banana");
set.add("cherry");
System.out.println("ConcurrentSkipListSet: " + set);
}
}
Output
ConcurrentSkipListSet: [apple, banana, cherry]
Explanation
ConcurrentSkipListSet
is thread-safe and uses a skip list for efficient navigation while TreeSet
would need to be synchronized externally for thread safety.
32. What are the advantages of using EnumMap in Java?
Ans: EnumMap
is a specialized map implementation designed specifically for enum keys. It provides better performance than regular HashMap
for enum keys because it is internally optimized to work with enums, using an array-based implementation rather than a hash table.
import java.util.EnumMap;
enum Fruit {
APPLE, BANANA, CHERRY
}
public class EnumMapExample {
public static void main(String[] args) {
EnumMap map = new EnumMap<>(Fruit.class);
map.put(Fruit.APPLE, 1);
map.put(Fruit.BANANA, 2);
map.put(Fruit.CHERRY, 3);
System.out.println("EnumMap: " + map);
}
}
Output
EnumMap: {APPLE=1, BANANA=2, CHERRY=3}
Explanation
EnumMap
is optimized for use with enum types, offering better performance and less memory overhead compared to other map implementations.
33. How does a Deque differ from a Queue in Java?
Ans: A Deque
(Double-Ended Queue) allows elements to be added or removed from both ends (front and back), while a Queue
typically allows elements to be added at the end and removed from the front, following FIFO (First-In-First-Out) order.
import java.util.ArrayDeque;
import java.util.Deque;
public class DequeExample {
public static void main(String[] args) {
Deque deque = new ArrayDeque<>();
deque.addFirst("apple");
deque.addLast("banana");
deque.addFirst("cherry");
System.out.println("Deque: " + deque);
}
}
Output
Deque: [cherry, apple, banana]
Explanation
Deque
allows adding and removing elements from both ends, unlike a regular Queue
, which is restricted to one end for insertion and the other for removal.
34. What is the purpose of the computeIfAbsent method in Map?
Ans: The computeIfAbsent
method is used to compute a value for a given key only if the key is not already associated with a value. It helps avoid unnecessary computation when the key is already present.
import java.util.HashMap;
import java.util.Map;
public class ComputeIfAbsentExample {
public static void main(String[] args) {
Map map = new HashMap<>();
map.put("apple", 1);
// Computes the value if absent
map.computeIfAbsent("banana", key -> key.length());
System.out.println("Map: " + map);
}
}
Output
Map: {apple=1, banana=6}
Explanation
When computeIfAbsent
is called the key "banana"; it computes the value based on the length of the key and inserts it into the map. If "banana" had been present already, the computation would have been skipped.
35. What is the difference between shallow cloning and deep cloning of a collection?
Ans: Shallow cloning copies the collection structure but not the objects contained within it. Any changes to the objects in the cloned collection affect the original. Deep cloning creates a completely independent copy of the collection and its objects.
import java.util.ArrayList;
import java.util.List;
public class ShallowVsDeepClone {
public static void main(String[] args) {
List originalList = new ArrayList<>();
originalList.add("apple");
// Shallow clone
List shallowCopy = new ArrayList<>(originalList);
// Deep clone
List deepCopy = new ArrayList<>();
for (String item : originalList) {
deepCopy.add(new String(item)); // Creates new object
}
shallowCopy.set(0, "banana");
System.out.println("Original List: " + originalList);
System.out.println("Shallow Copy: " + shallowCopy);
System.out.println("Deep Copy: " + deepCopy);
}
}
Output
Original List: [apple]
Shallow Copy: [banana]
Deep Copy: [apple]
Explanation
The shallow copy points to the same object as the original collection, while the deep copy creates new objects for each element in the collection.
36. How can you implement an LRU cache using LinkedHashMap?
Ans: You can implement an LRU (Least Recently Used) cache by using a LinkedHashMap
with access order enabled. This ensures that the map maintains the order of access, allowing easy eviction of the least recently accessed element.
import java.util.LinkedHashMap;
import java.util.Map;
public class LRUCacheExample {
public static void main(String[] args) {
Map cache = new LinkedHashMap<>(16, 0.75f, true);
// Add elements to the cache
cache.put(1, "apple");
cache.put(2, "banana");
cache.put(3, "cherry");
// Access an element to update its position
cache.get(2);
// Add a new element, evicting the least recently used element (1)
cache.put(4, "date");
System.out.println("Cache: " + cache);
}
}
Output
Cache: {2=banana, 3=cherry, 4=date}
Explanation
The LinkedHashMap
with access order ensures that the least recently used element (key 1) is evicted when a new element is added.
37. How do you create an immutable collection in Java?
Ans: You can create an immutable collection in Java by using methods from the Collections
class like Collections.unmodifiableList()
, or by using the List.of()
, Set.of()
, and Map.of()
methods introduced in Java 9 for creating immutable collections directly.
import java.util.List;
public class ImmutableCollectionExample {
public static void main(String[] args) {
List immutableList = List.of("apple", "banana", "cherry");
System.out.println("Immutable List: " + immutableList);
}
}
Output
Immutable List: [apple, banana, cherry]
Explanation
List.of()
creates an immutable collection that cannot be modified.
38. What is the purpose of Spliterator in Java Collections?
Ans: Spliterator
is an interface that allows for efficient splitting and iteration over a collection. It is particularly useful for parallel processing. It is designed to traverse and partition elements of a collection efficiently.
import java.util.List;
import java.util.Spliterator;
public class SpliteratorExample {
public static void main(String[] args) {
List list = List.of("apple", "banana", "cherry");
Spliterator spliterator = list.spliterator();
spliterator.forEachRemaining(System.out::println);
}
}
Output
apple
banana
cherry
Explanation
Spliterator
is used for efficient iteration over elements, supporting parallel processing and other optimized operations.
39. How can you merge two Maps efficiently in Java?
Ans: You can merge two maps efficiently by using the putAll()
method or the Java 8 merge()
method, which allows you to combine values for matching keys based on a given function.
import java.util.HashMap;
import java.util.Map;
public class MergeMapsExample {
public static void main(String[] args) {
Map map1 = new HashMap<>();
map1.put(1, "apple");
map1.put(2, "banana");
Map map2 = new HashMap<>();
map2.put(2, "cherry");
map2.put(3, "date");
map1.putAll(map2);
System.out.println("Merged Map: " + map1);
}
}
Output
Merged Map: {1=apple, 2=cherry, 3=date}
Explanation
putAll()
merges the maps by copying all entries from map2
into map1
, overwriting any duplicate keys.
40. What are the performance considerations of using different implementations of Set?
Ans: The performance of Set
implementations in Java depends on the underlying data structures. For example, a HashSet
offers constant-time performance for most operations, but it does not maintain any order. A TreeSet
guarantees sorted order but has a higher time complexity (log(n)) for most operations. A LinkedHashSet
maintains insertion order with a slightly slower performance than HashSet
, but it does not offer the sorting of TreeSet
.
Download This PDF - Collections in Java Interview Questions PDF By ScholarHat |
Summary
Java Collections Framework is a vital topic for Java interviews, covering concepts like List, Set, Map, and their implementations, such as ArrayList, HashMap, and TreeSet. This article explores key questions for both freshers and experienced candidates, including differences between collections, internal workings of data structures, and advanced topics like synchronization, concurrency, and performance optimization. It serves as a comprehensive guide for understanding and acing Java collections-related questions. Elevate your career with ScholarHat's Full-Stack Java Developer Certification Training and gain the skills to succeed in the tech industry!
FAQs
Take our Java skill challenge to evaluate yourself!
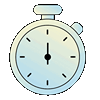
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.