18
AprLearn Method Overloading in Java (With Examples)
Method Overloading in Java
Method overloading in Java is important for learning its object-oriented programming capabilities. Method Overloading in Java enables you to declare multiple methods with the same name but distinct parameters, resulting in different implementations depending on the input. This makes your code simpler and more manageable because you may use the same method name for many tasks.
In this Java tutorial, we will look at method overloading in Java and cover what it is. And when should we utilize method overloading? We'll also look at Method Overloading in Java with examples. So, let us begin by examining "What is Method Overloading?"
What is Method Overloading in Java?
Let's understand the Method Overloading in Java:
- Method Overloading in Java lets you create several methods with the same name but different parameters.
- This means you can have multiple methods that do similar tasks, but each works with different types or numbers of inputs.
- It makes your code easier to understand and use because you can call the same method name in different situations.
For example, method overloading is when a calculated method can compute the area of different shapes, such as a circle (using radius) or a rectangle (using length and width), by using the same method name with different parameters.
Why is Method Overloading Important?
Let's understand the key importance of Method Overloading in Java:
- Method Overloading is important because it lets you use the same method name for similar tasks but with different inputs.
- This makes your code cleaner and easier to understand, and it avoids repeating code.
- It also helps handle different scenarios without needing extra method names.
Advantages of Method Overloading in Java
Here are the key advantages of Method overloading in Java:
- Method overloading improves the readability and reusability of the code.
- Method overloading decreases the program's complexity.
- Method overloading allows programmers to complete a task efficiently and effectively.
- Method overloading allows you to access methods that perform related functions but use slightly different arguments and types.
- Objects of a class can also be initialized in various ways using constructors.
Different Ways to Implement Method Overloading In Java
Here are various techniques to implement method overloading.
1. Changing the Number of Parameters
- One of the simplest ways to implement method overloading is by changing the number of parameters.
- A method may vary depending on how many parameters are supplied.
- The following example demonstrates how to implement method overloading with varying numbers of parameters in method declaration and definition.
Example
class Calculator {
// Method to sum two integers
int sum(int a, int b) {
return a + b;
}
// Overloaded method to sum three integers
int sum(int a, int b, int c) {
return a + b + c;
}
}
public class Main {
public static void main(String[] args) {
Calculator calc = new Calculator();
System.out.println("Sum of 2 numbers: " + calc.sum(10, 20)); // Output: 30
System.out.println("Sum of 3 numbers: " + calc.sum(10, 20, 30)); // Output: 60
}
}
Output
Sum of 2 numbers: 30
Sum of 3 numbers: 60
Explanation
- The Calculator class has two sum methods: one for summing two integers and one for summing three integers (method overloading).
- In the Main class, the sum methods are called with different numbers of arguments to demonstrate method overloading.
Real-Life Example
class ShoppingCart {
// Method to calculate total cost without discount
double calculateTotal(double price1, double price2) {
return price1 + price2;
}
// Overloaded method to calculate total cost with a discount on one item
double calculateTotal(double price1, double price2, double discount) {
return price1 + (price2 - discount);
}
}
public class Main {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
System.out.println("Total cost without discount: " + cart.calculateTotal(50.0, 30.0)); // Output: 80.0
System.out.println("Total cost with discount: " + cart.calculateTotal(50.0, 30.0, 5.0)); // Output: 75.0
}
}
Output
Total cost without discount: 80.0
Total cost with discount: 75.0
Explanation
- The ShoppingCart class has two calculateTotal methods: one adds two prices, and the other applies a discount to the second price before adding.
- In the Main class, both methods are used to show the total cost with and without a discount, demonstrating method overloading.
2. Changing the Data Type of Parameters
One more way to perform method overloading is to change the data types of method parameters. The following example demonstrates how to perform method overloading in method declaration and definition using various parameter data types.
Example
class Printer {
// Method to print an integer
void print(int value) {
System.out.println("Printing integer: " + value);
}
// Overloaded method to print a double
void print(double value) {
System.out.println("Printing double: " + value);
}
}
public class Main {
public static void main(String[] args) {
Printer printer = new Printer();
printer.print(10);
printer.print(10.5);
}
}
Output
Printing integer: 10
Printing double: 10.5
Explanation
- The Printer class has two print methods: one for printing integers and another for printing doubles, demonstrating method overloading.
- In the Main class, both methods are called to show how the Printer handles different data types.
Real-Life Example
class AreaCalculator {
// Method to calculate the area of a circle (using double)
double area(double radius) {
return Math.PI * radius * radius;
}
// Method to calculate the area of a rectangle (using int)
int area(int length, int width) {
return length * width;
}
}
public class Main {
public static void main(String[] args) {
AreaCalculator areaCalc = new AreaCalculator();
System.out.println("Area of circle: " + areaCalc.area(7.5)); // Output: Area of circle: 176.71
System.out.println("Area of rectangle: " + areaCalc.area(5, 10)); // Output: Area of rectangle: 50
}
}
Output
Area of circle: 176.71458676442586
Area of rectangle: 50
Explanation
- The AreaCalculator class has two area methods: one calculates the area of a circle (using a double radius), and the other calculates the area of a rectangle (using int length and width), showcasing method overloading.
- In the Main class, both methods are called with appropriate arguments to compute and display the areas of a circle and a rectangle.
3. Changing the Order of Parameters
- Method overloading can also be performed by arranging the arguments of two or more overloaded methods.
- For example, if method 1's arguments are (String f_name, int roll_no) and the other methods are (int roll_no, String f_name), but they both have the same name, these two methods are regarded as overloaded with distinct parameter sequences.
Example
class Display {
// Method to display string first, then integer
void show(String s, int a) {
System.out.println("String: " + s + ", Integer: " + a);
}
// Overloaded method to display integer first, then string
void show(int a, String s) {
System.out.println("Integer: " + a + ", String: " + s);
}
}
public class Main {
public static void main(String[] args) {
Display display = new Display();
display.show("Hello", 10); // Output: String: Hello, Integer: 10
display.show(20, "World"); // Output: Integer: 20, String: World
}
}
Output
String: Hello, Integer: 10
Integer: 20, String: World
Explanation
- The Display class has two show methods: one prints a string followed by an integer, and the other prints an integer followed by a string, demonstrating method overloading.
- In the Main class, both methods are called with different argument orders to display the outputs.
Real-Life Example
class Logger {
// Method to log a message with a string priority
void log(String priority, int code) {
System.out.println("Priority: " + priority + ", Code: " + code);
}
// Overloaded method to log a message with an integer priority
void log(int code, String priority) {
System.out.println("Code: " + code + ", Priority: " + priority);
}
}
public class Main {
public static void main(String[] args) {
Logger logger = new Logger();
logger.log("High", 101); // Output: Priority: High, Code: 101
logger.log(102, "Low"); // Output: Code: 102, Priority: Low
}
}
Output
Priority: High, Code: 101
Code: 102, Priority: Low
Explanation
- The Logger class has two log methods: one logs a string priority followed by a code, and the other logs a code followed by a string priority, demonstrating method overloading.
- In the Main class, both methods are called with different argument orders to display log messages.
Method Overloading Considerations
Q 1. Why is Method Overloading Not Possible by Changing the Return Type Only?
Example
class Example {
// Method 1: An integer version of the method
int add(int a, int b) {
return a + b;
}
// Method 2: A double version of the method
// This will cause a compile-time error!
double add(int a, int b) {
return a + b + 0.0;
}
public static void main(String[] args) {
Example obj = new Example();
int sum1 = obj.add(10, 20); // This calls the first add method
// double sum2 = obj.add(10, 20); // Compiler error: cannot resolve method
}
}
Output
// Compiler Error: Method add(int,int) is already defined in class Example
Explanation
- The Example class has two methods named add(),both with the same parameter types (int and int), butone is supposed to return an int and the other a double.
- In Java, method overloading is based on the method signature, which includes the method name and parameter types. The return type is not considered in method overloading.
- Because both methods have the same name and parameter types, the compiler cannot distinguish between them, leading to a compile-time error.
- To fix this, you could change the parameter types or the number of parameters to make the method signatures unique.
Q 2. When the Java Compiler Decides: Compile-Time vs Run-Time Errors
Example
class CompileTimeExample {
// Method 1: int version
int multiply(int a, int b) {
return a * b;
}
// Method 2: double version (will cause a compile-time error)
// double multiply(int a, int b) {
// return a * b * 1.0;
// }
public static void main(String[] args) {
CompileTimeExample obj = new CompileTimeExample();
int result = obj.multiply(10, 5); // This is fine
// double result2 = obj.multiply(10, 5); // Compiler will catch this error if method 2 is uncommented
}
}
Output
50
// Note: If Method 2 is uncommented, a compiler error will occur:
// Method multiply(int,int) is already defined in class CompileTimeExample
Explanation
- The CompileTimeExample class contains two methods named multiply(), both with the same parameters (int a and int b)but with different return types (int for one, double for the other).
- In Java, method overloading depends on the method signature, which includes the method name and the parameter types. The return type is not part of the method signature for overloading.
- Uncommenting the second method would cause a compile-time error because the compiler cannot differentiate between the two methods based on their parameter list alone.
- The correct way to overload the method would be to change the parameter list, for example, by changing the parameter types or adding an additional parameter.
Q 3. Can We Overload Static Methods?
Example
class StaticMethodExample {
// Static method with one parameter
static void display(int a) {
System.out.println("Integer: " + a);
}
// Overloaded static method with two parameters
static void display(int a, int b) {
System.out.println("Sum: " + (a + b));
}
// Overloaded static method with different parameter types
static void display(String a) {
System.out.println("String: " + a);
}
public static void main(String[] args) {
// Calling the overloaded static methods
StaticMethodExample.display(10); // Calls the first method
StaticMethodExample.display(10, 20); // Calls the second method
StaticMethodExample.display("Hello"); // Calls the third method
}
}
Output
Integer: 10
Sum: 30
String: Hello
Explanation
- The StaticMethodExample class demonstrates method overloading with static methods. The display method is overloaded three times with different parameter lists: one with a single integer, one with two integers, and one with a string.
- The first method is called with a single integer, which prints the integer value. The second method is called with two integers, which adds the integers and prints their sum. The third method is called with a string, which prints the string value.
- Overloading static methods follows the same rules as overloading instance methods, allowing multiple methods with the same name but different parameter lists within the same class.
Q 4. Can We Overload the main() Method?
Example
class MainMethodOverload {
// Standard main method called by JVM
public static void main(String[] args) {
System.out.println("Standard main method");
// Calling overloaded main methods explicitly
main(10);
main("Hello");
}
// Overloaded main method with an integer parameter
public static void main(int a) {
System.out.println("Overloaded main method with int: " + a);
}
// Overloaded main method with a String parameter
public static void main(String a) {
System.out.println("Overloaded main method with String: " + a);
}
}
Output
Standard main method
Overloaded main method with int: 10
Overloaded main method with String: Hello
Explanation
- The Main Method Overloadclassdemonstrates method overloading with the main method. Although the mainmethod is typically used as the entry point for a Java application, it can be overloaded with different parameter lists.
- The standard main (String[] args)JVM calls the method to start the application. Within this method, two overloaded versions of themainmethod are explicitly called: one with an integer parameter and one with a string parameter.
- The overloaded main (int a) and main (String a) methods are invoked from the standard main method, demonstrating that you can define multiple main methods with different parameter types. However, only the standard main method is used to start the Java application.
Q 5. What if the exact prototype does not match the arguments?
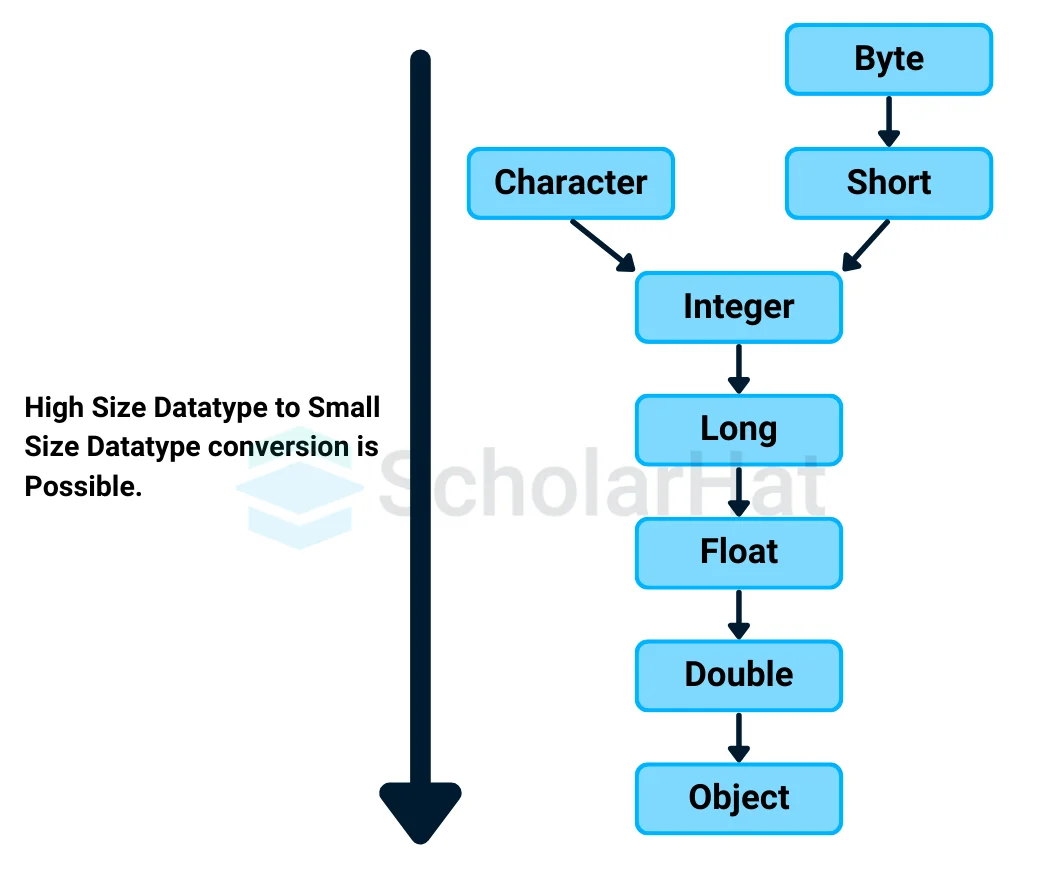
- Type conversion to a higher type (in terms of range) within the same family.
- Type conversion to a subsequent higher family (for example, if no long data type is available for an int data type, it will look for a float data type).
Example
class TypeConversionExample {
// Method with an int parameter
void show(int a) {
System.out.println("int: " + a);
}
// Method with a double parameter
void show(double a) {
System.out.println("double: " + a);
}
// Method with a String parameter
void show(String a) {
System.out.println("String: " + a);
}
public static void main(String[] args) {
TypeConversionExample obj = new TypeConversionExample();
obj.show(10); // Exact match with int
obj.show(10L); // No long method, so int method is used (widening to int)
obj.show(10.5f); // float is widened to double
obj.show("Hello"); // Exact match with String
}
}
Output
int: 10
double: 10.0
double: 10.5
String: Hello
Explanation
- The TypeConversionExample class has three show methods: one for int, one for double, and one for String.
- In the main method, different types of arguments are passed to demonstrate method overloading and type conversion (e.g., long is converted to int, and float to double).
Difference between Method Overloading and Method Overriding in Java
Factor | Method Overloading | Method Overriding |
Definition | Defining multiple methods with the same name but different parameter lists (types, numbers, or both). | Redefining a method in a subclass that is already defined in the superclass with the same signature. |
Method Signature | It must differ in the number or type of parameters. | It must have the same method signature (name, return type, and parameters) as the superclass method. |
Return Type | It can have different return types for overloaded methods. | It must have the same return type or a subtype (covariant return type) as the method in the superclass. |
Inheritance | Methods can be overloaded within the same class. | Overriding requires inheritance (a subclass overriding a superclass method). |
Binding | Static binding (resolved at compile-time). | Dynamic binding (resolved at runtime). |
Polymorphism | Not a type of polymorphism. | A key feature of runtime polymorphism. |
Access Modifier | It can have different access modifiers for overloaded methods. | To modify or extend the behavior of an inherited method. |
Summary
Further Read Articles: |
Method-Overriding In Java |
"while" Loop in Java |
Parameterized Constructor in Java |
File Handling in Java Complete Guide |
Practice MCQs for a Better Understanding
Q 1: What is method overloading in Java?
- (a) When a method has the same name but different return types.
- (b) When a method has the same name but different parameter types.
- (c) When a method is called multiple times in the program.
- (d) When a method is overloaded with different access modifiers.
Q 2: Which of the following is true about method overloading?
- (a) Method overloading is determined at runtime.
- (b) Method overloading is determined at compile time.
- (c) Method overloading can be achieved using different return types.
- (d) Method overloading is not possible in Java.
Q 3: Which of the following is NOT a valid method overloading scenario in Java?
- (a) Methods with the same name but different number of parameters.
- (b) Methods with the same name and same number of parameters but different return types.
- (c) Methods with the same name and same number of parameters but different types of arguments.
- (d) Methods with the same name and same type of parameters but different access modifiers.
Q 4: What will be the output of the following code?
class MethodOverloading {
public static void display(int a) {
System.out.println("Integer: " + a);
}
public static void display(double a) {
System.out.println("Double: " + a);
}
public static void main(String[] args) {
display(5); // Will call the display(int)
display(5.5); // Will call the display(double)
}
}
- (a) Integer: 5
- (b) Double: 5.5
- (c) Integer: 5, Double: 5.5
- (d) Compilation Error
Q 5: Which of the following is an advantage of method overloading in Java?
- (a) It allows the same method name to perform different tasks based on the input parameters.
- (b) It reduces memory usage by reusing method names.
- (c) It increases the complexity of the program.
- (d) It makes the code more difficult to maintain.