11
JulTop 40+ String in Java Interview Questions with Answers 2025
If you're getting ready for a Java interview, understanding Strings is essential. You might be asked to explain String properties and behavior or write code involving String operations. Familiarity with concepts like immutability, the String Pool, and common String methods is very helpful.
In this interview tutorial, we’ll cover the top 40 Java String interview questions with simple examples and clear explanations. By the end, you’ll feel confident and prepared to tackle any String-related question. Plus, these skills will enhance your real-world programming expertise. Get ready to excel in your Java interview!
What can you expect in Java String interview questions?
Java String interview questions are designed to test your understanding of how Strings work and how well you can apply this knowledge to solve problems. You can expect to be asked about concepts like immutability, the String Pool, and common String methods, as well as how to manipulate and optimize String operations.
Read More: Java Interview Questions and Answers. |
Basic Interview Questions on String in Java
Q1. What is String in Java?
Ans:A string in Java is a sequence of characters, like a sentence or a word. It's a predefined class in Java’s java.lang package and represents text. So when you declare String name = "Hello";you're creating an object that holds the characters "Hello."
Example:
public class StringExample {
public static void main(String[] args) {
String greeting = "Hello, Scholarhat!";
System.out.println(greeting);
}
}
Output:
Hello, Scholarhat!
Explanation:
A String in Java is an object that represents a sequence of characters. It is part of the java.lang package and is immutable, meaning once a String is created, its value cannot be changed.
Q2. How are Strings stored in memory in Java?
Ans: Strings in Java are stored in a special area of memory called the String Pool. When you create a String using double quotes, Java checks if an identical string already exists in the pool. If it does, Java just points to the existing String instead of creating a new one, saving memory.
Q3. How do you compare two Strings in Java?
Ans: To compare two Strings in Java, you have a couple of options:
- .equals() Method: This checks if the actual contents of the two strings are the same.
- == operator: This checks if both strings point to the same memory location, so it’s more of a reference comparison rather than checking the content.
Q4. What is the difference between == and .equals() when comparing Strings?
Ans:The differences between == and .equals() when comparing Strings are as follows:
Aspect | == Operator | .equals() Method |
Comparison Type | Compares reference (memory address) | Compares actual string content |
Usage | Checks if two references point to the same object | Checks if two strings have the same value |
Returns | True if both references are identical | True if string values are equal |
Null Handling | May throw NullPointerException if the object is null | Safe to use as long as the string literal is on the left |
Common Use Case | When checking object identity | When checking logical string equality |
Q5. What is String immutability in Java?
Ans: Strings in Java are immutable, meaning once you create a String, you can't change it. So, if you try to modify a String (like adding to it), Java will create a new String object instead of changing the original one. This is done for security and optimization reasons.
Q6. What is the use of the substring() method in Java?
Ans: The substring()method in Java allows you to extract a portion of a String. For example, it"Hello".substring(1, 3) would give you "el". It takes two parameters, the starting index (inclusive) and the ending index (exclusive), and returns the specified part of the String.
Example
public class SubstringExample {
public static void main(String[] args) {
String str = "Hello World";
String sub1 = str.substring(6);
String sub2 = str.substring(0, 5);
System.out.println("Substring from index 6: " + sub1);
System.out.println("Substring from index 0 to 5: " + sub2);
}
}
Output
Substring from index 6: World
Substring from index 0 to 5: Hello
Explanation
The substring()
method in Java allows you to extract a part of a string based on index positions. If you provide one argument, it starts from that index to the end. If you provide two, it extracts the substring between the start (inclusive) and end (exclusive) indexes.
Q7. Can you modify a String after it is created in Java?
Ans: No, because Strings are immutable. However, if you want to change or manipulate text, you can use StringBuilder or StringBuffer, which are mutable alternatives. They allow you to modify the content without creating a new object each time.
Q8. Is String a primitive or derived type in Java?
Ans:In Java, String is actually a derived type, not a primitive. It's a class in the java.lang package, which means it's an object, even though it behaves a lot like a primitive in some ways. You can create string objects using double quotes, but behind the scenes, Java is using the String class to handle it. So, even though it feels simple, it's not a primitive like int or boolean.
Q9. State the difference between String in C and String in Java.
Aspect | String in C | String in Java |
---|---|---|
Type | Array of characters (char[]) | Object of the String class |
Mutability | Mutable (can be changed) | Immutable (cannot be changed after creation) |
Null-Termination | Ends with a null character '\0' | No null termination needed |
Built-in Methods | Requires manual functions (like strlen, strcat) | Comes with rich built-in methods (like length(), concat()) |
Q10. Explain String pool in Java.
Ans:In Java, the String Pool (also known as the String Constant Pool) is a special area in memory where Java stores string literals. When you create a string using double quotes, Java first checks if that string already exists in the pool. If it does, it simply reuses the reference; if not, it creates a new entry in the pool. This helps save memory and improves performance because the same string literal is not duplicated in memory. However, strings created with the new keyword are not automatically placed in the pool unless explicitly interned.
As shown in the above image, Java's String Pool stores unique string literals to save memory. When s1 = "Apple" and s3 = "Apple" are declared, both reference the same pooled object, so s1 == s3 returns true. However, s2 = "Mango" creates a new entry, making s1 == s2 return false since they point to different objects. This shows how == compares memory addresses, while.equals() checks content. The pool avoids duplicates, optimizing performance.
Q11. What does the string intern() method do in Java?
Ans:In Java, the intern() method is used to add a string to the pool. When you call intern() on a string object, Java checks if an equal string already exists in the pool. If yes, it returns that pooled reference; if not, it adds the string to the pool and returns the new reference. This is particularly useful when you create strings using the new keyword (which normally bypasses the pool) but want them to benefit from the pool's memory optimization. For example, new String("text").intern() ensures you get the pooled version of "text".
Q12. The difference between String and StringBuffer.
Ans: The differences between String and StringBuffer are as follows:
Feature | String | StringBuffer |
---|---|---|
Mutability | Immutable (cannot be modified) | Mutable (can be modified) |
Performance | Slower for frequent modifications | Faster for frequent changes |
Storage | Stored in String Pool (if literal) | Stored in heap memory |
Thread Safety | Thread-safe (due to immutability) | Thread-safe (synchronized methods) |
Methods | concat(), substring(), etc. | append(), insert(), reverse(), etc. |
Memory | Creates new objects on modification | Modifies existing object |
Use Case | Fixed text (e.g., constants) | Dynamic text manipulation |
String Programming Interview Questions in Java for Experienced
For experienced Java developers, string programming questions often test problem-solving skills through real-world scenarios like parsing, transformation, or pattern-based logic.
Q13. How would you reverse a String in Java without using built-in methods?
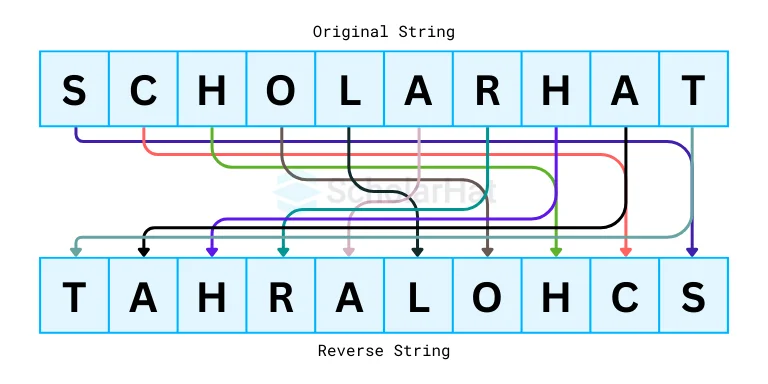
Ans: You can reverse a String by iterating from the end and adding each character to a new String:
public class ReverseString {
public static void main(String[] args) {
String str = "Hello";
String reversed = "";
for (int i = str.length() - 1; i >= 0; i--) {
reversed += str.charAt(i);
}
System.out.println("Reversed String: " + reversed);
}
}
Output
Reversed String: olleH
Explanation
This Java program reverses a string by iterating from the last character to the first. The Looping Statements in Java build a new string by appending each character in reverse order.
Practice with these Articles: |
Q14. Write a Java program to check if a String is a palindrome.
Ans: A palindrome reads the same forward and backward:
public class PalindromeCheck {
public static void main(String[] args) {
String str = "madam";
boolean isPalindrome = true;
for (int i = 0; i < str.length() / 2; i++) {
if (str.charAt(i) != str.charAt(str.length() - 1 - i)) {
isPalindrome = false;
break;
}
}
System.out.println("Is Palindrome: " + isPalindrome);
}
}
Output
Is Palindrome: true
Explanation
The program checks if the input string is a palindrome by comparing characters from the beginning and end towards the center. If all characters match symmetrically, it is a palindrome.
Q15. How do you find the first non-repeated character in a String?
Ans: Use nested loops to find the first unique character:
public class FirstNonRepeatedCharacter {
public static void main(String[] args) {
String str = "swiss";
char firstNonRepeated = '\0';
for (int i = 0; i < str.length(); i++) {
boolean isUnique = true;
for (int j = 0; j < str.length(); j++) {
if (i != j && str.charAt(i) == str.charAt(j)) {
isUnique = false;
break;
}
}
if (isUnique) {
firstNonRepeated = str.charAt(i);
break;
}
}
System.out.println("First Non-Repeated Character: " + firstNonRepeated);
}
}
Output
First Non-Repeated Character: w
Explanation
This program finds the first non-repeated character by iterating through each character and checking for duplicates. It stops at the first unique character found.
Q16. Write a program to count the occurrences of each character in a String.
Ans: Count each character's occurrences using nested loops:
public class CharacterCount {
public static void main(String[] args) {
String str = "banana";
for (int i = 0; i < str.length(); i++) {
char c = str.charAt(i);
int count = 0;
for (int j = 0; j < str.length(); j++) {
if (c == str.charAt(j)) {
count++;
}
}
System.out.println("Character " + c + ": " + count);
}
}
}
Output
Character b: 1
Character a: 3
Character n: 2
Character a: 3
Character n: 2
Character a: 3
Explanation
This program counts each character's occurrences in the string by iterating through all characters. Each character's count is printed on the screen as it’s checked.
Q17. How do you check if two Strings are anagrams of each other in Java?
Ans: To check if two strings are anagrams, sort the characters in each string and compare the sorted strings. If they’re the same, they are anagrams:
import java.util.Arrays;
public class AnagramCheck {
public static void main(String[] args) {
String str1 = "listen";
String str2 = "silent";
boolean isAnagram = areAnagrams(str1, str2);
System.out.println("Are Anagrams: " + isAnagram);
}
public static boolean areAnagrams(String str1, String str2) {
if (str1.length() != str2.length()) {
return false;
}
char[] arr1 = str1.toCharArray();
char[] arr2 = str2.toCharArray();
Arrays.sort(arr1);
Arrays.sort(arr2);
return Arrays.equals(arr1, arr2);
}
}
Output
Are Anagrams: true
Explanation
This program converts each string to a character array, sorts the arrays, and then checks if they are equal. If they match, the two strings are anagrams.
Q18. How would you remove all white spaces from a String?
Ans: To remove all white spaces from a string, use a loop to build a new string without spaces:
public class RemoveWhitespace {
public static void main(String[] args) {
String str = "Hello World";
String withoutSpaces = "";
for (int i = 0; i < str.length(); i++) {
if (str.charAt(i) != ' ') {
withoutSpaces += str.charAt(i);
}
}
System.out.println("String without spaces: " + withoutSpaces);
}
}
Output
String without spaces: HelloWorld
Explanation
This code removes spaces by iterating through each character and adding it to a new string only if it is not a space character.
Q19. Write a program to check if a String contains only digits.
Ans: To check if a string contains only digits, loop through each character and ensure each one is a digit:
public class OnlyDigitsCheck {
public static void main(String[] args) {
String str = "12345";
boolean onlyDigits = true;
for (int i = 0; i < str.length(); i++) {
if (!Character.isDigit(str.charAt(i))) {
onlyDigits = false;
break;
}
}
System.out.println("Contains only digits: " + onlyDigits);
}
}
Output
Contains only digits: true
Explanation
This code checks each character in the string to see if it is a digit. If all characters are digits, it returns true; otherwise, it returns false.
Java String Interview Questions for 5 Years Experience
For someone with 5 years of Java experience, String-related interview questions usually dive into topics like the difference between String, StringBuilder, and StringBuffer, where you'll need to explain immutability and performance. You might also be asked about String interning and how Java optimizes memory with its string pool. Understanding how to compare strings with == versus .equals() is crucial, as well as knowing how to format strings using String.format() for better readability.
Q20. How would you find if a String contains only unique characters?
Ans: You can check if a string contains only unique characters by using a set to track characters you've already encountered:
import java.util.HashSet;
public class UniqueCharacters {
public static void main(String[] args) {
String str = "abcdef";
boolean hasUniqueChars = true;
HashSet set = new HashSet<>();
for (int i = 0; i < str.length(); i++) {
if (!set.add(str.charAt(i))) {
hasUniqueChars = false;
break;
}
}
System.out.println("Contains only unique characters: " + hasUniqueChars);
}
}
Output
Contains only unique characters: true
Explanation
This program uses a HashSet to store characters as they are encountered. If a character is already in the set, the string contains duplicate characters. Otherwise, it only has unique characters.
Q21. What is the difference between String, StringBuilder, and StringBuffer in terms of performance?
Ans: The differences are as follows:
- String: Immutable, meaning that every time you modify a string, a new object is created. This can be inefficient for frequent modifications.
- StringBuilder: Mutable and faster than StringBuffer. It is not synchronized, which makes it faster for single-threaded applications.
- StringBuffer: Mutable and synchronized, meaning it's thread-safe but slower than StringBuilder due to synchronization overhead.
Q22. How would you implement your own split() method for String in Java?
Ans: You can split a string by iterating over it and finding the delimiters manually:
public class CustomSplit {
public static void main(String[] args) {
String str = "apple,banana,cherry";
String delimiter = ",";
String[] result = customSplit(str, delimiter);
for (String s : result) {
System.out.println(s);
}
}
public static String[] customSplit(String str, String delimiter) {
int count = 0;
String temp = "";
for (int i = 0; i < str.length(); i++) {
if (str.substring(i, i + delimiter.length()).equals(delimiter)) {
count++;
}
}
String[] result = new String[count + 1];
int index = 0;
temp = "";
for (int i = 0; i < str.length(); i++) {
if (str.substring(i, i + delimiter.length()).equals(delimiter)) {
result[index++] = temp;
temp = "";
i += delimiter.length() - 1;
} else {
temp += str.charAt(i);
}
}
result[index] = temp; // Last part
return result;
}
}
Output
apple
banana
cherry
Explanation
This code splits a string by iterating through it and identifying occurrences of the delimiter. It then stores each part between the delimiters in Java arrays.
Q23. Write a program to find the first occurrence of a substring in a String.
Ans: You can use a loop to check for the first occurrence of a substring:
public class SubstringSearch {
public static void main(String[] args) {
String str = "Hello, welcome to Java programming!";
String substr = "welcome";
int index = str.indexOf(substr);
System.out.println("First occurrence of substring: " + index);
}
}
Output
First occurrence of substring: 7
Explanation
This program uses the built-inindexOf()method to find the first occurrence of a substring within a string. It returns the index of the first character of the found substring.
Q24. How would you compare two Strings lexicographically without using the compareTo() method?
Ans: You can compare two strings lexicographically by comparing each character one by one:
public class LexicographicComparison {
public static void main(String[] args) {
String str1 = "apple";
String str2 = "banana";
int result = lexicographicCompare(str1, str2);
System.out.println("Comparison result: " + result);
}
public static int lexicographicCompare(String str1, String str2) {
int minLength = Math.min(str1.length(), str2.length());
for (int i = 0; i < minLength; i++) {
if (str1.charAt(i) != str2.charAt(i)) {
return str1.charAt(i) - str2.charAt(i);
}
}
return str1.length() - str2.length();
}
}
Output
Comparison result: -1
Explanation
This program compares two strings character by character. If a mismatch is found, it returns the difference between the characters. If no mismatch is found, it compares the lengths of the strings.
Q25. How do you convert a String to a char array and vice versa in Java?
Ans: You can use toCharArray()to convert a string to a char array, and new String(charArray) to convert a char array back to a string:
public class StringToCharArray {
public static void main(String[] args) {
String str = "Hello";
// String to char array
char[] charArray = str.toCharArray();
System.out.println("Char Array: " + Arrays.toString(charArray));
// Char array back to String
String newStr = new String(charArray);
System.out.println("New String: " + newStr);
}
}
Output
Char Array: [H, e, l, l, o]
New String: Hello
Explanation
Java String Interview Questions for 10+ Years’ Experience
For a Java developer with 10+ years of experience, interview questions often dive into the deeper aspects of how strings are managed in memory and how to optimize their performance in large-scale applications. You'll likely be asked about efficient handling of large data, managing concurrency, and ensuring that string operations are performed with minimal impact on system resources. Additionally, understanding the nuances of different encoding schemes and how they affect data processing in complex environments is crucial.
Q26. How would you implement your own immutable String class in Java?
Ans: To implement an immutable String class, you need to ensure that once the object is created, its state cannot be changed. This can be done by declaring the fields as final and providing no setter methods:
public class ImmutableString {
private final char[] value;
public ImmutableString(String str) {
value = str.toCharArray();
}
public char charAt(int index) {
return value[index];
}
public int length() {
return value.length;
}
public String toString() {
return new String(value);
}
}
Explanation
The class is immutable because the value field is final and cannot be modified after initialization. There are no methods that modify the string’s value, only methods that retrieve its state.
Q27. Explain the memory structure when creating new Strings using literals and new keywords.
Ans: When creating strings in Java:
- String literals: Java maintains a String Pool. If a string with the same literal already exists, Java will return a reference to that string. This avoids creating duplicate strings, optimizing memory usage.
- Using the new keyword: When you create a new string using new, it always creates a new object, even if the exact same string already exists in the pool. This can lead to memory overhead.
Q28. Write an efficient program to find the longest common prefix in an array of Strings.
Ans: A common approach is to compare the characters of each string at the same index:
public class LongestCommonPrefix {
public static void main(String[] args) {
String[] strs = {"flower", "flow", "flight"};
String result = longestCommonPrefix(strs);
System.out.println("Longest Common Prefix: " + result);
}
public static String longestCommonPrefix(String[] strs) {
if (strs == null || strs.length == 0) {
return "";
}
String prefix = strs[0];
for (int i = 1; i < strs.length; i++) {
while (strs[i].indexOf(prefix) != 0) {
prefix = prefix.substring(0, prefix.length() - 1);
if (prefix.isEmpty()) {
return "";
}
}
}
return prefix;
}
}
Output
Longest Common Prefix: fl
Explanation
This program checks each string in the array and gradually reduces the common prefix until it matches for all strings. It uses theindexOfmethodto check the prefix.
Q29. How would you optimize memory usage when dealing with large Strings?
Ans: To optimize memory when handling large strings, consider these approaches:
- Use StringBuilder: If you need to perform many modifications to a string, use StringBuilder, as it is mutable and more memory efficient.
- Use String pooling wisely: If you frequently reuse the same string, Java’s String Pool can help save memory.
- Trim unnecessary characters: Avoid creating new strings unnecessarily and use the substring()method carefully as it may still reference the original string.
- Lazy loading: For very large text, consider loading parts of the string only when needed, such as using streams or memory-mapped buffers.
Q30. Discuss how the String.format() method works and give examples of its usage.
Ans: The String.format()method allows you to create formatted strings by substituting placeholders with values. Here's an example:
public class StringFormatExample {
public static void main(String[] args) {
String formattedString = String.format("My name is %s and I am %d years old.", "John", 25);
System.out.println(formattedString);
}
}
Output
My name is John and I am 25 years old.
Explanation
In this example, %s is used to format a string and %dis used for an integer. String.format() replaces these placeholders with the provided arguments.
Q31. How would you use regular expressions to validate a String in Java?
Ans: To validate a string using regular expressions, you can use the matches() method of the String class:
public class RegexValidation {
public static void main(String[] args) {
String str = "john.doe@example.com";
boolean isValid = str.matches("^[a-zA-Z0-9_+&*-]+(?:\\.[a-zA-Z0-9_+&*-]+)*@(?:[a-zA-Z0-9-]+\\.)+[a-zA-Z]{2,7}$");
System.out.println("Is valid email: " + isValid);
}
}
Output
Is valid email: true
Explanation
The program checks if the input string matches a regular expression for a valid email address. The matches()method returns true if the string matches the pattern, otherwise false.
Q32. Write a program to rotate a String by a given number of positions.
Ans: You can rotate a string by slicing it and swapping the parts:
public class StringRotation {
public static void main(String[] args) {
String str = "abcdef";
int positions = 2;
String rotated = rotateString(str, positions);
System.out.println("Rotated String: " + rotated);
}
public static String rotateString(String str, int positions) {
positions = positions % str.length(); // To handle larger positions
return str.substring(positions) + str.substring(0, positions);
}
}
Output
Rotated String: cdefab
Explanation
The program rotates the string by the specified number of positions by slicing the string and concatenating the two parts in reverse order.
String Coding Questions in Java
Q33. Reverse a String without using built-in functions.
Ans: To reverse a string without using built-in functions, you can iterate through the string in reverse order and construct a new string:
public class ReverseStringWithoutBuiltIn {
public static void main(String[] args) {
String str = "Hello";
String reversed = "";
for (int i = str.length() - 1; i >= 0; i--) {
reversed += str.charAt(i);
}
System.out.println("Reversed String: " + reversed);
}
}
Explanation
This program manually reverses the string by iterating through it from the last character to the first, appending each character to the result.
Q34. Check if a String is a palindrome using recursion.
Ans: A string is a palindrome if it reads the same forward and backward. This can be checked recursively by comparing the first and last characters and then calling the function on the substring:
public class PalindromeRecursion {
public static void main(String[] args) {
String str = "madam";
boolean isPalindrome = isPalindrome(str, 0, str.length() - 1);
System.out.println("Is Palindrome: " + isPalindrome);
}
public static boolean isPalindrome(String str, int start, int end) {
if (start >= end) {
return true;
}
if (str.charAt(start) != str.charAt(end)) {
return false;
}
return isPalindrome(str, start + 1, end - 1);
}
}
Explanation
This recursive function checks if the characters at the start and end of the string are the same. If they are, it calls itself on the substring from the next start and end indices until it checks all characters.
Q35. Write a program to remove duplicate characters from a String.
Ans: To remove duplicate characters, iterate through the string and add each character to a set or check if it has already been seen:
import java.util.HashSet;
public class RemoveDuplicates {
public static void main(String[] args) {
String str = "programming";
StringBuilder result = new StringBuilder();
HashSet seen = new HashSet<>();
for (int i = 0; i < str.length(); i++) {
char currentChar = str.charAt(i);
if (!seen.contains(currentChar)) {
seen.add(currentChar);
result.append(currentChar);
}
}
System.out.println("String without duplicates: " + result.toString());
}
}
Explanation
This program uses a HashSet to track the characters that have already been encountered and append only the unique characters to a StringBuilder.
Q36. Write a program to capitalize the first letter of each word in a String.
Ans: To capitalize the first letter of each word, split the string into words and capitalize the first character of each word:
public class CapitalizeWords {
public static void main(String[] args) {
String str = "hello world";
String[] words = str.split(" ");
StringBuilder capitalized = new StringBuilder();
for (String word : words) {
capitalized.append(word.substring(0, 1).toUpperCase())
.append(word.substring(1)).append(" ");
}
System.out.println("Capitalized String: " + capitalized.toString().trim());
}
}
Explanation
This program splits the string into words, then capitalizes the first letter of each word by using substring() and toUpperCase(). The result is concatenated into a new string.
Q37. Write a program to check if a String contains only letters and digits.
Ans: You can use the matches()method with a regular expression that checks for only letters and digits:
public class CheckLettersAndDigits {
public static void main(String[] args) {
String str = "Hello123";
boolean isValid = str.matches("[a-zA-Z0-9]+");
System.out.println("Contains only letters and digits: " + isValid);
}
}
Explanation
This program uses the regular expressio [a-zA-Z0-9]+,which ensures that the string contains only alphabetic characters (both uppercase and lowercase) and digits.
Advanced Interview Programs on String in Java
Q38. Implement your own version of the replace() method.
Ans: To implement your own replace()method, you can iterate through the string and replace occurrences of the target substring with the replacement:
public class ReplaceString {
public static void main(String[] args) {
String str = "hello world";
String target = "world";
String replacement = "Java";
String result = customReplace(str, target, replacement);
System.out.println("Replaced String: " + result);
}
public static String customReplace(String str, String target, String replacement) {
return str.replaceAll(target, replacement);
}
}
Explanation
This program mimics the replace() functionality by using the replaceAll() method. The method replaces the target substring with the provided replacement throughout the string.
Q39. Write a program to check if one String is a rotation of another String.
Ans: To check if one string is a rotation of another, concatenate the first string with itself and check if the second string is a substring of the concatenated string:
public class StringRotation {
public static void main(String[] args) {
String str1 = "abcde";
String str2 = "deabc";
boolean isRotation = isRotation(str1, str2);
System.out.println("Is Rotation: " + isRotation);
}
public static boolean isRotation(String str1, String str2) {
if (str1.length() != str2.length()) {
return false;
}
String concatenated = str1 + str1;
return concatenated.contains(str2);
}
}
Explanation
This program concatenates str1 with itself and checks if str2 is a substring of the concatenated string. If it is, then str2 is a rotation of str1.
Q40. Write a program to find the most frequent word in a String.
Ans: To find the most frequent word, split the string into words and use a map to count the occurrences:
import java.util.HashMap;
import java.util.Map;
public class MostFrequentWord {
public static void main(String[] args) {
String str = "this is a test this is a test test";
String mostFrequentWord = findMostFrequentWord(str);
System.out.println("Most Frequent Word: " + mostFrequentWord);
}
public static String findMostFrequentWord(String str) {
String[] words = str.split(" ");
Map wordCount = new HashMap<>();
for (String word : words) {
wordCount.put(word, wordCount.getOrDefault(word, 0) + 1);
}
String mostFrequent = null;
int maxCount = 0;
for (Map.Entry entry : wordCount.entrySet()) {
if (entry.getValue() > maxCount) {
mostFrequent = entry.getKey();
maxCount = entry.getValue();
}
}
return mostFrequent;
}
}
Explanation
This program splits the input string into words, counts their occurrences using a HashMap, and then finds the word with the maximum count.
Q41. Write a program to find the longest repeating sequence in a String.
Ans: To find the longest repeating sequence, you can use a sliding window approach to check for repeated substrings:
public class LongestRepeatingSequence {
public static void main(String[] args) {
String str = "abcdabc";
String longestSeq = findLongestRepeatingSequence(str);
System.out.println("Longest Repeating Sequence: " + longestSeq);
}
public static String findLongestRepeatingSequence(String str) {
String longest = "";
for (int i = 0; i < str.length(); i++) {
for (int j = i + 1; j < str.length(); j++) {
String subStr = str.substring(i, j);
if (str.indexOf(subStr, j) != -1 && subStr.length() > longest.length()) {
longest = subStr;
}
}
}
return longest;
}
}
Explanation
This program uses nested loops to find all substrings and checks if they appear more than once in the string. It returns the longest substring that repeats.
Q42. Implement a program that performs basic string compression (e.g., "aaabbc" -> "a3b2c1").
Ans: For string compression, iterate through the string and count consecutive characters, then build the compressed string:
public class StringCompression {
public static void main(String[] args) {
String str = "aaabbc";
String compressed = compressString(str);
System.out.println("Compressed String: " + compressed);
}
public static String compressString(String str) {
StringBuilder compressed = new StringBuilder();
int count = 1;
for (int i = 1; i < str.length(); i++) {
if (str.charAt(i) == str.charAt(i - 1)) {
count++;
} else {
compressed.append(str.charAt(i - 1)).append(count);
count = 1;
}
}
compressed.append(str.charAt(str.length() - 1)).append(count);
return compressed.toString();
}
}
Explanation
This program iterates through the string, counting consecutive occurrences of each character and appending the character followed by its count to the result.
String Programs in Java for Practice
Q43. Count the number of words in a String.
Ans: To count the number of words, split the string into words and count the resulting array length:
public class WordCount {
public static void main(String[] args) {
String str = "This is a sample string";
int wordCount = countWords(str);
System.out.println("Number of Words: " + wordCount);
}
public static int countWords(String str) {
String[] words = str.split("\\s+");
return words.length;
}
}
Explanation
This program splits the string by spaces (using the regular expression\\s+ to match any whitespace) and counts the length of the resulting array, which gives the word count.
Q44. Replace spaces with "%20" in a given String (URL encoding).
Ans: You can replace spaces with "%20" by using the replace()method:
public class ReplaceSpaces {
public static void main(String[] args) {
String str = "Hello World, how are you?";
String encoded = encodeSpaces(str);
System.out.println("Encoded String: " + encoded);
}
public static String encodeSpaces(String str) {
return str.replace(" ", "%20");
}
}
Explanation
This program uses the replace()method to replace all spaces in the string with "%20", which is the URL encoding for a space.
Q45. Write a program to sort the characters of a String in alphabetical order.
Ans: You can convert the string into a character array, sort it, and then reconstruct the string:
import java.util.Arrays;
public class SortString {
public static void main(String[] args) {
String str = "dbca";
String sorted = sortString(str);
System.out.println("Sorted String: " + sorted);
}
public static String sortString(String str) {
char[] chars = str.toCharArray();
Arrays.sort(chars);
return new String(chars);
}
}
Explanation
This program converts the string into a character array and sorts it usingArrays.sort(), and then convert the sorted array back to a string.
Q46. Check if a given String is a valid number (e.g., "123", "-456", "0.789").
Ans: You can use regular expressions or try parsing the string into a number:
public class ValidNumber {
public static void main(String[] args) {
String str = "-123.45";
boolean isValid = isValidNumber(str);
System.out.println("Is Valid Number: " + isValid);
}
public static boolean isValidNumber(String str) {
try {
Double.parseDouble(str);
return true;
} catch (NumberFormatException e) {
return false;
}
}
}
Explanation
This program attempts to parse the string into a double using Double.parseDouble(). If it succeeds, the string is a valid number; otherwise, it is not.
Q47. Write a program to remove all occurrences of a given character from a String.
Ans: You can remove all occurrences of a character using the replace()method:
public class RemoveCharacter {
public static void main(String[] args) {
String str = "hello world";
char ch = 'o';
String result = removeCharacter(str, ch);
System.out.println("Result String: " + result);
}
public static String removeCharacter(String str, char ch) {
return str.replace(String.valueOf(ch), "");
}
}
Explanation
This program uses the replace ()method to replace all occurrences of the specified character with an empty string, effectively removing it from the original string.
Download This PDF - String in Java Interview Questions PDF By ScholarHat |
Summary
Java is a versatile, object-oriented, high-level programming language known for its platform independence and strong memory management. It supports multiple paradigms, including object-oriented and concurrent programming, making it ideal for building enterprise-level applications, mobile apps, and web platforms. If you're aiming to strengthen your Java skills and earn a valuable credential, consider joining a Java certification course.
If you are preparing for the Java Interview, this Java Interview Questions and Answers Book can really help you. It has simple questions and answers that are easy to understand. Download and read it for free today!
Test your knowledge: Are you ready to challenge yourself and ace this quiz?
Q 1: Which class in Java is used to create immutable string objects?
FAQs
- length(): Returns the number of characters in the string.
- substring(): Extracts a part of the string based on given indexes.
- toUpperCase() / toLowerCase(): Converts the string to upper or lower case.
- replace(): Replaces occurrences of a specified character or substring with another.
Take our Java skill challenge to evaluate yourself!
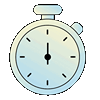
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.