18
Aprwhile Loop in Java
while loop in Java
Java while loop is one of the most commonly used loopsin Java. The while statement continually executes a block of statements while a particular condition is true. The test takes place before each iteration.
In this tutorial, we will learn What is while loop in Java, and while loop syntax in Java, and also look at some of the while loop programs in Java. To learn more about various concepts of Java Programming, do consider enrolling in our Java Full Stack Developer Courseright now!!
Read More: Top 50 Java Interview Questions and Answers
Read More - Java Multithreading Interview Questions
What is a while loop in Java?
In Java Programming, the while loop is used to repeat the execution of a specific block of code based on a Boolean condition. It evaluates the condition to either be 'True' or 'False' and based on the boolean result the execution takes place. If the condition is true, the block of code inside the while is executed. But if the condition is false, the loop terminates immediately to carry on to the next statement which is just after the while loop.
Syntax of while loop in Java
while (condition) {
// code to be executed
}
Parts of Java while Loop
1. Test Expression
In the Test Expression, the specified condition is checked. If the condition is evaluated to be 'True', the block of code inside the loop will be executed and it will move forward to the update expression. If the condition is false, the loop will terminate.
while (test_expression) {
// body of the loop
}
Example:
int i = 1;
while (i <= 5) {
// body of the loop
}
2. Update Expression
The Update Expression does an increment or decrement to the loop variable every time the execution of loop body occurs. It is written after the body of the loop. When the execution occurs and the loop comes to this expression the variable is incremented or decremented as specified in the expression. In short, it updates the value of the variable.
while (test_expression) {
// body of the loop
update_expression;
}
Example:
int i = 1;
while (i <= 5) {
System.out.println(i);
i++; // update expression
}
Read More: Java Developer Salary Guide in India – For Freshers & Experienced
Flowchart of Java while Loop
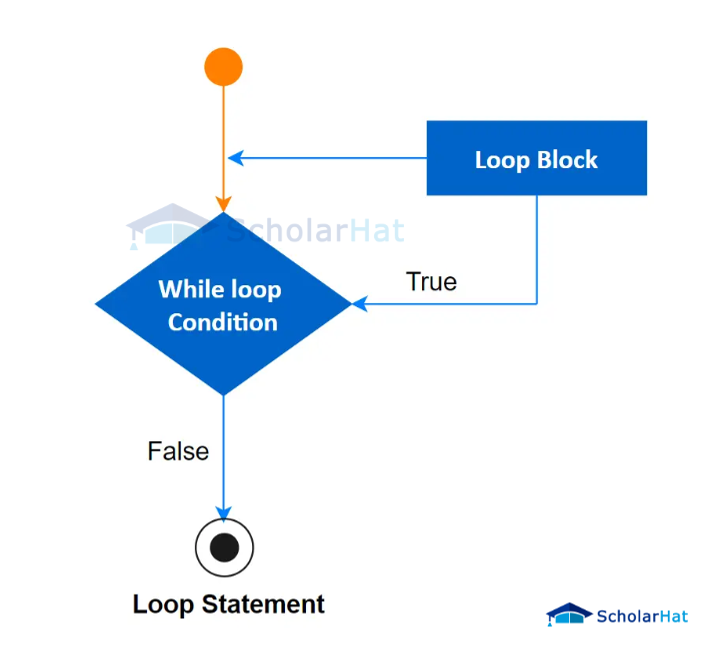
How Does a while loop execute?
Now let's try to understand how exactly a while loop is executed in a Java Program.
- Initialization Firstly, all the variables that are to be used inside the loop are initialized. This initialization is done before the while loop starts.
- Condition Checking Then comes the step where the condition inside the while loop is checked. If the condition is evaluated to be 'True', the loop body is executed. If it evaluates to 'False', the loop is terminated and the program carries on with the next statement which is just after the loop.
- Body Execution The body of the while loop is executed if the condition is 'True' and then, the loop returns to the second step where the condition is checked again.
- Iteration Every time the loop body is executed, the loop variables are updated. They either increment or decrement and the value is changed for the next iteration accordingly.
- Loop Termination The while checks the condition till it becomes 'False' and if it does, the loop is terminated and the program moves on to the next statement just after the loop.
Examples of Java while loop
1. Printing Numbers from 1 to 5
public class WhileLoopExample1 {
public static void main(String[] args) {
int i = 1; // Initialization
while (i <= 5) { // Test expression
System.out.println(i); // Body
i++; // Update expression
}
}
}
Output
1
2
3
4
5
2. Calculating Factorial of a Number
public class WhileLoopExample2 {
public static void main(String[] args) {
int num = 5;
int factorial = 1;
int i = 1; // Initialization
while (i <= num) { // Test expression
factorial *= i; // Body
i++; // Update expression
}
System.out.println("Factorial of " + num + " is: " + factorial);
}
}
Output
Factorial of 5 is: 120
3. Countdown from 5 to 1
public class WhileLoopExample4 {
public static void main(String[] args) {
int i = 5; // Initialization
while (i >= 1) { // Test expression
System.out.println(i); // Body
i--; // Update expression
}
System.out.println("Blast Off!");
}
}
Output
5
4
3
2
1
Blast Off!
Summary
Through the above article, we learnt about while loop in Java, its structure, flowchart and also while loop example in Java Program. Do have a look on other loops in Java to have a proper understanding of them all. And if you are a beginner to Java Programming, get enrolled in our Java Full Stack Developer Certificationto grab the comprehensive step by step guide to Java.
FAQs
count = 0 while count < 5: print(count) count += 1
do { // code block to be executed } while (condition);
- First, you need to initialize a variable outside of the loop.
- Use 'while' with a condition attached to it using the variable.
- Put an update expression after that so the loop eventually ends.