11
JulMastering Arrow Functions in JavaScript
JavaScript Arrow Functions
Arrow functions in JavaScript are anonymous functions, i.e., unnamed functions often assigned to a variable. They were introduced in the ES6 version of JavaScript and are simple and concise alternatives to writing anonymous function expressions using =>. => indicates the beginning of an arrow function. They improve code readability.
In this JavaScript tutorial, we'll cover all the aspects of arrow functions in JavaScript, including their syntax, parameter variation, differences between traditional/regular functions and arrow functions, etc. To master arrow functions and other JavaScript concepts, be sure to enroll in our Free Javascript Course for a complete learning experience.
Read More: JavaScript Interview Questions and Answers (Fresher + Experience)
There are two ways to declare an arrow function:
- Without curly braces {}
JavaScript Single-line Arrow Function Syntax
let myFunction = (arg1, arg2, ...argN) => expression
Unlike traditional functions, this method doesn't require writing the return keyword. With the single-line arrow function, JavaScript implicitly adds the return statement.
Example of Single line Arrow Function in JavaScript
let multiply = (a, b) => a * b; console.log(multiply(8, 6));
multiply(8, 6) calls the multiply function with 8 and 6 as arguments. The function returns the product in the absence of the return statement.
Output
48
- With curly braces {}
Multi-line statements in the arrow function require the explicit return statement for returning values in response to the function call.
JavaScript Multi-line Arrow Function Syntax
let myFunction = (arg1, arg2, ...argN) => { statement(s) }
Example of Multi-line Arrow Function in JavaScript
let multiply = (a, b) => { return a * b; }; console.log(multiply(8, 6));
multiply(8, 6) calls the multiply function with 8 and 6 as arguments. The function returns the product in the presence of the return statement.
Output
48
Parameters of Arrow Functions
Arrow functions in JavaScript can have zero or multiple parameters. The parameters of the arrow functions are specified in parentheses ().
1. Arrow Function without Parameters
An empty parentheses () denotes an arrow function devoid of parameters.
Example of Arrow Function without Parameters
const greet = () => "Welcome to ScholarHat!";
console.log(greet());
The above single-line arrow function greet() takes no parameter.
Output
Welcome to ScholarHat!
2. Arrow Function with Single Parameters
If an arrow function has only one parameter, parentheses become optional.
Example of Arrow Function with only One Parameter
const product = x => x * 2;
console.log(product(8));
Output
16
3. Arrow Function with Multiple Parameters
Example of Arrow Function with more than One Parameter
const product = ( x, y, z ) => {
console.log( x * y * z )
}
product( 17, 3, 21);
Output
1071
4. Arrow Function with Default Parameters
You can specify predefined values in the () of the arrow function in the absence of the arguments passed to the function.
Example of Arrow Function with Default Parameters
const product = ( x, y = 60, z = 60 ) => {
console.log( x * y * z);
}
product( 42, 20 );
In the above code, y and z are the default parameters. In the function call, we've passed the value for the default parameter, z. In this case, the passed argument replaces the predefined values.
Output
50400
Regular Function vs. Arrow Function
The regular function is created using the function keyword followed by the function name.
Example of a Regular Function
function multiply(x, y) {
return x * y;
};
console.log(multiply(8, 6));
A function named multiply is defined with two parameters, x and y.
Output
48
Steps to Convert a Regular Function to an Arrow Function
- Replace the function keyword with the variable keyword const
- Add the = symbol after the function name and before the parentheses
- Add the => symbol after the parentheses
We can also replace the function keyword with the let keyword, but generally, a function is never changed after the declaration. Hence, you can preferably use the const keyword.
Example of a Regular function converted to an Arrow function
const multiply = (x, y) => x * y;
console.log(multiply(8, 6));
You can look at the implementation of the above three steps in the above code.
Output
48
You can remove the {} and the return keyword for single-line arrow functions and the () for arrow functions with no parameters, as we've seen above.
Now, we'll discuss the differentiating points between regular and arrow functions with examples.
1. this Keyword With Arrow Function
this in JavaScript is used to refer to the current object. The current object is the object on which the method is invoked. However, this is not the case with arrow functions.
Arrow functions use the lexical 'this'; that is, the value of 'this' is determined by the surrounding lexical scope. The 'this' keyword always represents the object that defines the arrow function.
Example of this keyword inside a Regular function
function Employee() {
this.name = "Jack",
this.designation = "SEO",
this.sayName = function () {
// this is accessible
console.log(this.designation);
function displayDesig() {
// this refers to the global object
console.log(this.designation);
console.log(this);
}
displayDesig();
}
}
let x = new Employee();
x.sayName();
In the above code, this.age inside this.sayName() is accessible because this.sayName() is an object method. However, displayDesig() is a normal function, and this.designation is not accessible because 'this' refers to the global object. Therefore, this.designation inside the displayDesig() function is undefined.
Output
SEO
undefined
Object [global]
Example of this keyword with Arrow function
function Employee() {
this.name = 'Sourav',
this.designation = 'SEO',
this.sayName = function () {
console.log(this.designation);
let displayDesig = () => {
console.log(this.designation);
}
displayDesig();
}
}
const x = new Employee();
x.sayName();
Here, the displayDesig() function is an arrow function. Inside the arrow function, this refers to the parent's scope, i.e., the scope of the Employee() function. Hence, this designation gives SEO.
Output
SEO
SEO
2. JavaScript arrow functions and the arguments object
An arrow function doesn’t have the arguments object. Attempting to access arguments within an arrow function will refer to the arguments object of the surrounding non-arrow function or will be undefined if there is no regular function around.
function add() {
return x => x + arguments[0];
}
let display = add(100, 30);
let result = display(5);
console.log(result);
The arrow function inside the add() function references the arguments object. However, this argument object belongs to the add() function, not the arrow function.
Output
105
3. Arrow Functions as a Constructor
An arrow function in JavaScript can never be used as a function constructor. Attempting to create a new object from an arrow function through the new keyword will result in an error.
let show = () => {};
let obj = new show();
Output
let obj = new show();
^
TypeError: show is not a constructor
4. Arrow functions and the prototype property
Regular functions have a prototype property for creating instances. Arrow functions don’t have the prototype property. As a result, the new keyword is not available, and you cannot construct an instance from the arrow function.
let text = message => console.log(message);
console.log(text.hasOwnProperty('prototype'));
Output
false
When Should You Not Use Arrow Functions?
Arrow functions should not be used to create methods inside objects. Arrow functions use the lexical 'this'.
let person = {
name: "Sourav",
designation: "SEO",
sayName: () => {
console.log(this.designation);
}
}
person.sayName();
Output
undefined
Summary
Arrow functions are a characteristic feature of JavaScript. They have distinct features and applications. We have studied this concept in great detail. You will now have a clear idea of when to use regular functions and when to use arrow functions. For more such detailed concepts, consider our JavaScript Programming Course.
FAQs
Take our Javascript skill challenge to evaluate yourself!
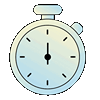
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.