13
SepData Types in JavaScript: Primitive & Non-Primitive
JavaScript Data Types
Data types in JavaScript represent the type of value stored in the JavaScript variables. They are one of the fundamental concepts of the JavaScript programming language.
In this JavaScript tutorial, we'll understand all the eight fundamental JavaScript data types with examples and the typeof operator in detail.
Read More: JavaScript Interview Questions and Answers (Fresher + Experience) |
Types of JavaScript Data Types
The ES6 version introduced in 2015 defines eight standard data types in JavaScript. The eight basic data types in JavaScript are classified into two categories:
1. JavaScript Primitive Data types
Primitive data types are predefined or built-in data types in JavaScript that can hold simple values.
2. JavaScript Non-Primitive Data types
Non-Primitive data types in JavaScript can hold multiple values and are derived from primitive ones. Hence, they are also known as derived data types or reference data types.
We'll understand all the primitive and non-primitive data types in detail in the below section.
Primitive Data Types in JavaScript
There are six primitive data types out of the eight basic data types in JavaScript. They are:
1. JavaScript String
Strings in JavaScript are a sequence of characters surrounded by quotes, single(' '), double(" "), or backticks (` `) representing textual data. For example, 'ScholarHat', "ScholarHat", `ScholarHat`.
Example of JavaScript String
// string enclosed within single quotes
let greetVar1 = 'Welcome to ScholarHat';
console.log(greetVar1)
// string enclosed within double quotes
let greetVar2 = "Welcome to ScholarHat";
console.log(greetVar2);
// string enclosed within backticks
let greetVar3 = `Welcome to ScholarHat`;
console.log(greetVar3);
In the above code, we've written the string in all three types of quotes. All print the same output.
Output
Welcome to ScholarHat
Welcome to ScholarHat
Welcome to ScholarHat
Read More: Strings in JavaScript
2. JavaScript Number
Numbers represent numeric values, both integer and floating values. There are also some special numbers like Infinity, -Infinity, and NaN (Not-a-Number).
Example of JavaScript String
// integer value
let int_number = 87;
console.log(int_number);
// floating-point value
let float_number = 9.56;
console.log(float_number);
let spnumber1 = 10 / 0;
console.log(spnumber1);
let spnumber2 = -10 / 0;
console.log(spnumber2);
let spnumber3 = "xyz" / 3;
console.log(spnumber3);
Output
87
9.56
Infinity
-Infinity
NaN
Read More: Numbers in JavaScript
3. JavaScript BigInt
BigInt is a number type for storing integers with arbitrary magnitudes beyond the range of the Number data type. It is created by appending n to the end of an integer or by calling the BigInt() function.
Example of JavaScript String
// BigInt value
let value1 = 900718825145740568n;
// add two big integers
let result = value1 + 1n;
console.log(result);
let value2 = 900719925124740998n + 9;
console.log(value2);
You cannot operate on BigInt numbers along with regular numbers. It results in type error.
Output
900718825145740569n
/index.js:8
let value2 = 900719925124740998n + 9;
^
TypeError: Cannot mix BigInt and other types, use explicit conversions
4. JavaScript Boolean
Boolean data type in JavaScript can have either true or false values.
Example of JavaScript String
let isCorrect = true;
console.log(isCorrect);
let isFalse = false;
console.log(isFalse);
Output
true
false
5. JavaScript undefined
A variable whose value is not assigned is called undefined. You can also explicitly assign "undefined" as a variable value.
Example of JavaScript undefined
let product;
console.log(product);
let sum = undefined;
console.log(sum);
Output
undefined
undefined
6. JavaScript null
null in JavaScript represents empty values or no values.
Example of JavaScript undefined
let sum = null;
console.log(sum);
Output
null
7. JavaScript Symbol
A JavaScript Symbol is a unique and immutable value. It is used to create unique identifiers for objects.
Example of JavaScript undefined
// two symbols with the same description
let value1 = Symbol("ScholarHat");
let value2 = Symbol("DotNetTricks");
console.log(value1 === value2);
In the above code, === compares vlue1 and value2. Though both value1 and value2 contain the exact string, "ScholarHat," JavaScript treats them as different since they are of the Symbol type.
Output
false
Non-Primitive Data Types in JavaScript
8. JavaScript Object
JavaScript objects store collections of data created using curly braces {} or the new keyword. They store data as key-value pairs.
Example of JavaScript Object
let CEO = {
firstName: "Shailendra",
lastName: null,
age: 50
};
console.log(CEO);
Key | Value |
firstName | Shailendra |
lastName | null |
age | 50 |
Output
{ firstName: 'Shailendra', lastName: null, age: 50 }
JavaScript typeof Operator
The typeof operator gives the data type of the variable. It can be used with or without parentheses().
let name = "sakshi";
console.log(typeof(name));
let number = 60;
console.log(typeof(number));
let isCorrect = true;
console.log(typeof(isCorrect));
let x = null;
console.log(typeof(x));
Output
string
number
boolean
object
JavaScript Types are Dynamic
JavaScript determines a variable's type based on its value. Variables are not directly associated with any particular data type, and any variable can be assigned values of all types. Hence, changing the value changes the variable's type.
let x;
console.log(typeof(x));
x = 5;
console.log(typeof(x));
x = "ScholarHat";
console.log(typeof(x));
In the above code, x is assigned values of different types.
Output
undefined
number
string
Summary
To learn JavaScript completely, you need to go step by step. Learning data types in JavaScript is one of the initial steps in the learning path. Understanding data types helps you deal with operators and variables properly. Follow the tutorial in sequence to learn in a structured way. Explore our JavaScript Programming Course to practice the learned concepts.
FAQs
Q1. How many types of JavaScript are there?
Q2. What is variable and data type?
Q3. How to define types in JS?
Take our Javascript skill challenge to evaluate yourself!
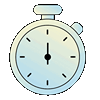
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.