21
FebJavaScript Events An Easy Learner
Events in JavaScript
An Events in JavaScriptare the occurrence that responds to an input from the user by producing an output. We may take into account various input formats, including mouse clicks, button pushes, and tab-press events that cause text boxes to alter. When a user presses a button in Javascript, the required action is carried out, and the user receives the answer as an output.
In the JavaScript tutorial, we will learn what events are in JavaScript, what common types of events are in JavaScript, what event handlers are in JavaScript, how to handle events in JavaScript, and many more. To master arrow functions and other JavaScript concepts, be sure to enroll in our Free Javascript Course for a complete learning experience.
What are the events in JavaScript?
The Event in Javascript is actionsor activities that take place in the web browser and are recognized and handled by the JavaScript code. These events can be brought about by browser functions like resizing a window or loading a page, as well as by user actions like clicking a button or pushing a key.
- Includes mouse events (click, mouseover), keyboard events (keydown, keyup), form events (submit, change), and window events (load, resize).
- Functions that respond to events, can be added directly in HTML or via JavaScript (onclick, addEventListener).
- More flexible than handlers, allowing multiple functions for the same event on an element.
- Automatically passed to event handlers, containing details like event type and target element.
- Events move through the DOM in capturing (down) and bubbling (up) phases; use event.stopPropagation() to stop it.
Syntax
<HTML-element Event-Type = "Action to be performed">
Read More: |
Introduction to JavaScript |
JavaScript Developer Salary |
How to Become a Full Stack JavaScript Developer? |
Why are Events in JavaScript important?
Events are essential in JavaScript to create interactive web applications. They provide features like form validation, dynamic content updates, and interactive user interface components by enabling you to make your web pages dynamic and responsive to user activities.
What are the Event Handlers?
Event handlers in JavaScript are functions or methods that are called in response to specific events in a program. In web development, they are commonly used to handle events like clicks, key presses, form submissions, and more. Event handlers allow you to define what should happen when an event occurs.
Types of Events in JavaScript
There are five types of events in JavaScript that are followed below with their event handlers:
- Mouse Events
- Keyboard Events
- Form Events
- Window Events
- Touch Events (for mobile devices)
1. Mouse Events
Events | Event-Handlers | Explanation |
click | onclick | when you click the mouse on an event. |
mouseout | onmouseout | When you take off the cursor on an element. |
mousedown | onmousedown | when you press the mouse button on an element. |
mouseup | onmouseup | when you release the mouse cursor on an element. |
mousemove | onmousemove | when a mouse movement occurs. |
mouseover | onmouseover | when you take the mouse cursor on an element. |
2. Keyboard Events
Events | Event-Handlers | Explanation |
Keyup | onkeyup | when the user presses any key on the keyboard. |
keydown | onkeydown | when a user releases the key on the keyboard. |
3. Form Events
Events | Event-Handlers | Explanation |
change | onchange | When a user updates or changes a form element's value. |
focus | onfocus | When the user focuses on an element. |
submit | onsubmit | When the user submits the form. |
blur | onblur | when a form element is not the center of attention |
4. Windows Event
Events | Event-Handlers | Explanation |
resize | onresize | When the user adjusts the browser's window size |
unload | onunload | The browser unloads the page when a user exits the current one. |
load | onload | when the browser completes the loading of the page. |
5. Touch Events
Events | Event-Handlers | Explanation |
touchstart | ontouchstart | when a touch point touches the screen. Used to detect the start of a touch interaction. |
touchmove | ontouchmove | when a touch point moves across the screen. Useful for detecting swipes or drags. |
touchend | ontouchend | when a touch point is lifted off the screen. Indicates the end of a touch event. |
touchcancel | ontouchcancel | when a touch event is interrupted. Occurs when the touch is canceled unexpectedly. |
touchenter | ontouchenter | when a touch point enters an element's area. Similar to mouseover but for touch interactions. |
touchleave | ontouchleave | when a touch point leaves an element's area. Similar to mouseout but for touch interactions. |
Some Common Examples of Events in JavaScript
1. Example of onClick event
<html>
<head> Javascript Events </head>
<body>
<script language="Javascript" type="text/Javascript">
<!--
function clickevent()
{
document.write("Welcome to ScholorHat!");
}
//-->
</script>
<form>
<input type="button" onclick="clickevent()" value="Who's this?"/>
</form>
</body>
</html>
Output
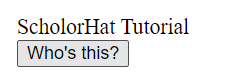
After clicking on the button, you will get:
2. Example of onsubmit Event
<html>
<head>
<title>Form Submit Example</title>
<script>
function validateForm(event) {
event.preventDefault(); // Prevent form submission
const name = document.forms["myForm"]["name"].value;
if (name === "") {
alert("Name must be filled out");
} else {
alert("Form submitted successfully!");
}
}
</script>
</head>
<body>
<form name="myForm" onsubmit="validateForm(event)">
Name: <input type="text" name="name">
<input type="submit" value="Submit">
</form>
</body>
</html>
Output

After filling in the name on the text box and clicking the submit button, you will get:
3. Example of onkeydown Event
<html>
<head>
<title>Keydown Event Example</title>
<script>
function logKey(event) {
console.log(`Key pressed: ${event.key}`);
}
</script>
</head>
<body>
<input type="text" onkeydown="logKey(event)" placeholder="Press any key">
</body>
</html>
Output
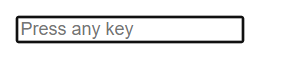
4. Example of unload Event
<html>
<head>
<title>Unload Event Example</title>
<script>
window.onunload = function() {
console.log("User is leaving the page");
// Cleanup code or analytics tracking can go here
};
</script>
</head>
<body>
<h1>Unload Event Example</h1>
<p>Check the console log when you leave this page.</p>
</body>
</html>
Output
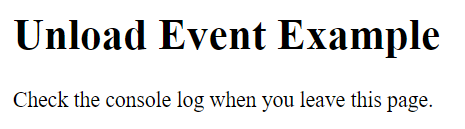
5. Example of ontouchstart Event
<html>
<head>
<title>Touchstart Event Example</title>
<script>
function handleTouchStart(event) {
document.getElementById("message").innerText = "Touch started!";
}
</script>
</head>
<body>
<div style="width: 100px; height: px; background-color: lightblue;"
ontouchstart="handleTouchStart(event)">
Touch here
</div>
<p id="message"></p>
</body>
</html>
Output
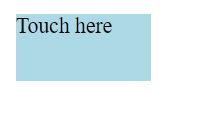
After touching on the Touch here you will get:
6. Example of load Event
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Load Event Example</title>
</head>
<body>
<h1>Page Load Event Example</h1>
<script>
// Attach the load event to the window object
window.addEventListener('load', function() {
alert('Page has fully loaded!');
console.log('All resources have been loaded.');
});
</script>
</body>
</html>
Output
Key Features and Benefits of Events in JavaScript
JavaScript events are essential to the interactive and dynamic nature of online applications:
Key Features of Events in JavaScript
- Various Event Types: Supports mouse, keyboard, form, and window events.
- Event Handling Methods: Use inline event handlers or addEventListener() for flexibility.
- Event Propagation: Events can bubble up or capture down through the DOM.
- Custom Events: Ability to create and trigger custom events.
- Event Object: Provides detailed information about the event.
Benefits of Using Events in JavaScript
- Interactivity: Enhances user experience by responding to user actions in real time.
- Modularity: Custom events and addEventListener() promote clean, maintainable code.
- Efficiency: Event delegation improves performance by reducing the number of event listeners needed.
- Control: The ability to prevent default actions and manage event propagation offers precise control over how events behave.
- Flexibility: A broad range of event types and customization options support diverse application needs.
Conclusion
In conclusion, we have explored the topic of Events in JavaSCript to build interactive, responsive web applications. Mastering event handling enables developers to create dynamic user experiences that react to various user actions, enhancing engagement and functionality. Understanding these events is essential for crafting seamless and effective web interfaces. Check out our JavaScript Programming Course for additional crucial ideas of this kind.
FAQs
Take our Javascript skill challenge to evaluate yourself!
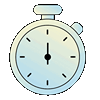
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.