18
AprTop Features of JavaScript You Need to Know!
Features of Javascript
When you hear about JavaScript, you might wonder, "What makes it so special?" Well, JavaScript is like the magic behind all those interactive web pages you see. From clickable buttons to dynamic updates without refreshing the page, JavaScript does it all. But that’s just scratching the surface!
First, let’s start with what JavaScript is. In this JavaScript tutorial, we’ll explore how it works, why it’s so powerful, and how you can use it effectively. You’ll also learn aboutdesign patterns in JavaScript, when to use them, and see examples that will helpyou apply them to your projects. To dive deeper into JavaScript and gain hands-on experience, you can enroll in a Free Online JavaScript Course and start learning today! Let’s explore it!
What is Javascript?
- JavaScript is a programming language you use to make websites interactive. It is what makes things happen on a website, like buttons you can click, forms that check what you type, or animations you see.
- It is like the brain of a website that helps it do more than just show text and pictures. For example, if you see a dropdown menu or a pop-up, JavaScript is making it work.
- You can use JavaScript in your browser, and it is easy to learn. That is why it is so popular for making websites.
Read More: Different Types of Design Patterns |
Top features of JavaScript.
The table below summarizes the essential features of JavaScript, highlighting its versatility, asynchronous capabilities, and role in creating dynamic, interactive web content. These features make it a cornerstone of modern web development.
Serial Number | Feature | Explanation |
1 | Light-Weight Scripting Language | JavaScript is a lightweight language that runs directly in browsers without heavy resource requirements. |
2 | Interpreter | JavaScript is interpreted, so it executes code line by line without needing prior compilation. |
3 | Dynamic Typing | You don’t need to specify variable types; JavaScript assigns them automatically at runtime. |
4 | Event Handling | Handles user actions like clicks, key presses, and form submissions to make applications interactive. |
5 | Case Sensitive | JavaScript treats uppercase and lowercase letters differently in variable and function names. |
6 | Control Statements | Includes if-else, switch, loops, and more to control the flow of code execution. |
7 | Objects as First-Class Citizens | Objects can be created, modified, and passed as dynamic arguments in JavaScript. |
8 | Functions as First-Class Citizens | Functions can be assigned to variables, passed as arguments, or returned from other functions. |
9 | Client-side Validations | Validates user inputs on the browser before sending them to the server, improving user experience. |
10 | Cross-Browser Compatibility | JavaScript works across all modern browsers, ensuring consistent functionality. |
11 | Async Processing | Supports asynchronous operations like API calls using callbacks, promises, or async/await syntax. |
12 | Prototype-Based | JavaScript uses prototypes for inheritance instead of traditional classes. |
13 | Nullish Coalescing Operator (??) | Provides a concise way to handle null or undefined values in expressions. |
14 | Logical Nullish Assignment (??=) | Allows you to assign a value only if the variable is null or undefined. |
15 | Styling Console Log | Offers custom styles for console messages to make debugging visually organized. |
16 | Date and Time Handling | Provides built-in objects and methods to handle date and time operations. |
17 | HTML Content Generation | Dynamically generates and modifies HTML using the Document Object Model (DOM). |
18 | Browser and OS Detection | Identifies the user’s browser and operating system for customizing functionality. |
19 | Let & Const Keywords | Offers block-scoped variable declarations with better control over variable scope and immutability. |
20 | Arrow Functions | Provides a concise syntax for writing functions, with the added benefit of maintaining this context. |
21 | Template Literals | Allows multi-line strings and embedded expressions using backticks (`). |
22 | New Array Functions | Provides modern methods like map(), filter(), reduce(), and more for array manipulation. |
23 | Default Parameters | Enables setting default values for function parameters when no value is provided. |
24 | Property Shorthand | Simplifies object creation by using the same name for variables and their properties. |
25 | Similar Syntax to Java | Shares a familiar syntax with Java, making it easier for Java developers to learn. |
26 | If-Else Statement | Implements decision-making logic for executing different code blocks based on conditions. |
27 | Looping Statements | Supports loops like for, while, and forEach for iterative operations. |
28 | BigInt | Handles very large numbers that exceed the Number type’s limits. |
29 | Dynamic Import | Enables dynamically imported modules during runtime for modular programming. |
30 | Promise.allSettled | Returns results of all promises, whether they are fulfilled or rejected, as an array. |
31 | Object-Oriented Programming | Supports object-oriented concepts like classes, inheritance, and encapsulation. |
32 | DOM Manipulation | Directly interacts with and updates the HTML structure for dynamic web pages. |
33 | Libraries and Frameworks | Integrates seamlessly with popular tools like React, Angular, and Vue.js. |
34 | Template Literals | Makes string formatting simpler with embedded expressions and multi-line support. |
35 | Async/Await | Simplifies writing asynchronous code for improved readability and error handling. |
Now, let's explore the Features of Javascript one by one.
1. Light-Weight Scripting Language
JavaScript is a lightweight scripting language you can use to make websites interactive. It runs directly in your browser, so you don’t need special tools to see it in action. It’s easy to learn, and you can use it to add things like animations, buttons, or dynamic content to your website. You can also use it with frameworks like React or Angular for advanced web apps. Plus, it works with APIs to fetch and display data seamlessly.
2. Interpreter
JavaScript is interpreted directly by browsers without the need for compilation.
console.log("JavaScript is interpreted!");
Output:
JavaScript is interpreted!
3. Dynamic Typing
Variables in JavaScript can hold values of any type, and you don’t need to specify the type.
let value = 42;
console.log(typeof value);
value = "Hello";
console.log(typeof value);
Output:
number
string
4. Event Handling
You can respond to user actions, such as clicks, using event handling.
document.getElementById("eventButton").onclick = function () {
alert("Button clicked!");
};
Clicking the button shows an alert.
Output:
Button clicked!
5. Case Sensitive
JavaScript distinguishes between uppercase and lowercase letters.
let value = 10;
let Value = 20;
console.log(value, Value);
Output:
10 20
6. Control Statements
Control statements like if
, else
, and loops guide the program flow.
let age = 18;
if (age >= 18) {
console.log("You are an adult.");
} else {
console.log("You are not an adult yet.");
}
Output:
You are an adult.
Read More: Java Conditional Statements: if-else and switch |
7. Objects as First-Class Citizens
Objects are essential in JavaScript, and they can be passed around as values.
let person = { name: "Alice", age: 25 };
console.log(person.name);
Output:
Alice
8. Functions as First-Class Citizens
Functions in JavaScript can be assigned to variables in JavaScript, passed as arguments, and returned from other functions.
function greet(name) {
return "Hello, " + name;
}
let sayHi = greet;
console.log(sayHi("Alice"));
Output:
Hello, Alice
9. Client-side Validations
JavaScript can validate form inputs before sending them to the server.
function validate() {
let name = document.getElementById("name").value;
if (name === "") {
alert("Name cannot be empty.");
} else {
console.log("Form submitted.");
}
}
Output:
"Name cannot be empty"
10. Platform Independent
JavaScript works across all platforms as long as a browser is available.
console.log("JavaScript works on any platform!");
Output:
JavaScript works on any platform!
11. Async Processing
JavaScript can handle tasks in the background, allowing you to perform other actions simultaneously.
setTimeout(() => {
console.log("This runs after 2 seconds!");
}, 2000);
Output:
This runs after 2 seconds!
12. Prototype-Based
JavaScript uses prototypes for inheritance instead of traditional class-based inheritance.
function Person(name) {
this.name = name;
}
Person.prototype.greet = function () {
console.log("Hi, I'm " + this.name);
};
let john = new Person("John");
john.greet();
Output:
Hi, I'm John
Read More: Understanding Inheritance and Different Types of Inheritance |
13. Nullish Coalescing Operator (??)
The ??
operator returns the right-hand value if the left-hand value is null or undefined.
let user = null;
console.log(user ?? "Guest");
Output:
Guest
14. Logical Nullish Assignment (??=)
The ??=
operator assigns a value only if the variable is null or undefined.
let user = null;
user ??= "Guest";
console.log(user);
Output:
Guest
Read More: Java Operators: Arithmetic, Relational, Logical, and More |
15. Styling Console Log
You can add styles to your console log messages using CSS.
console.log("%cStyled Text!", "color: blue; font-size: 20px;");
Output:
The console displays styled text.
16. Date and Time Handling
JavaScript provides built-in support for working with dates and times through the Date
object.
let currentDate = new Date();
console.log("Current Date and Time:", currentDate);
Output:
Current Date and Time: [Current Date and Time]
17. HTML Content Generation
You can dynamically create and manipulate HTML content using JavaScript.
document.body.innerHTML = "Welcome to JavaScript";
Output:
The page now displays: Welcome to JavaScript
18. Browser and OS Detection
JavaScript can detect the user's browser and operating system using the navigator
object.
console.log("Browser Info:", navigator.userAgent);
Output:
Browser Info: [Details about the browser]
19. Let & Const Keywords
JavaScript uses let
for block-scoped variables and const
for constants.
let age = 25;
const pi = 3.14;
console.log("Age:", age, "Pi:", pi);
Output:
Age: 25 Pi: 3.14
20. Arrow Functions
Arrow functions in JavaScript provide a concise way to write functions in JavaScript.
let greet = (name) => console.log("Hello, " + name);
greet("Alice");
Output:
Hello, Alice
21. Template Literals
Template literals allow you to embed variables and expressions directly into Strings in JavaScript.
let name = "Alice";
console.log(`Hello, ${name}!`);
Output:
Hello, Alice!
22. New Array Functions
JavaScript provides useful array methods in JavaScript like map()
, filter()
, and reduce()
.
let numbers = [1, 2, 3];
let doubled = numbers.map(num => num * 2);
console.log(doubled);
Output:
[2, 4, 6]
23. Default Parameters
You can set default values for function parameters.
function greet(name = "Guest") {
console.log("Hello, " + name);
}
greet();
Output:
Hello, Guest
24. Property Shorthand
Object property shorthand reduces redundancy when the property name matches the variable name.
let name = "Alice";
let age = 30;
let person = { name, age };
console.log(person);
Output:
{ name: 'Alice', age: 30 }
25. Similar Syntax to Java
JavaScript has a syntax similar to Java, making it familiar to developers with a Java background.
class Person {
constructor(name) {
this.name = name;
}
greet() {
console.log("Hello, " + this.name);
}
}
let alice = new Person("Alice");
alice.greet();
Output:
Hello, Alice
26. If-Else Statement
JavaScript allows you to perform conditional checks using the if-else
statement.
let age = 20;
if (age >= 18) {
console.log("You are an adult.");
} else {
console.log("You are not an adult.");
}
Output:
You are an adult.
27. Looping Statements
JavaScript provides loops like for
, while
, and do-while
to execute repetitive tasks.
for (let i = 1; i <= 5; i++) {
console.log("Count:", i);
}
Output:
Count: 1
Count: 2
Count: 3
Count: 4
Count: 5
Read More: For Loop in JavaScript |
28. BigInt
The BigInt
type is used to handle numbers larger than the Number
type's limit.
let bigNumber = 123456789012345678901234567890n;
console.log(bigNumber);
Output:
123456789012345678901234567890
29. Dynamic Import
JavaScript allows importing modules dynamically at runtime using import()
.
// Assuming module.js exports a function named greet
import('./module.js').then(module => {
module.greet();
});
30. Promise.allSettled
Promise.allSettled
waits for all promises to settle (either fulfilled or rejected).
let promises = [
Promise.resolve("Success"),
Promise.reject("Error"),
Promise.resolve("Another Success"),
];
Promise.allSettled(promises).then(results => console.log(results));
Output:
[
{ status: 'fulfilled', value: 'Success' },
{ status: 'rejected', reason: 'Error' },
{ status: 'fulfilled', value: 'Another Success' }
]
31. Object-Oriented Programming
JavaScript supports object-oriented programming with class
and constructor
syntax.
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(this.name + " makes a sound.");
}
}
let dog = new Animal("Dog");
dog.speak();
Output:
Dog makes a sound.
Practice with these Articles: |
32. DOM Manipulation
JavaScript lets you manipulate the Document Object Model (DOM) to change HTML content.
document.body.style.backgroundColor = "lightblue";
Output:
The background color of the page changes to light blue.
33. Libraries and Frameworks
JavaScript has popular libraries and frameworks like React, Angular, and Vue for building modern applications.
Note: Examples require additional setups, so they're not runnable in this simple environment.
34. Template Literals
Template literals make string manipulation easier with embedded expressions and multiline strings.
let greeting = `Hello,
This is a multiline string.`;
console.log(greeting);
Output:
Hello,
This is a multiline string.
Read More: Java Strings: Operations and Methods |
35. Async/Await
The async/await
syntax simplifies working with asynchronous code.
async function fetchData() {
let data = await Promise.resolve("Fetched Data");
console.log(data);
}
fetchData();
Output:
Fetched Data
Summary
Further Read |
Strings in JavaScript |
Switch Statement in JavaScript |
for Loop in JavaScript |
Map in Javascript |
FAQs
- Dynamic typing: Variables can hold any type of data, and their type is determined at runtime.
- Object-oriented: JavaScript supports object-oriented programming, allowing the use of objects and prototypes.
- Asynchronous programming: With features like callbacks, promises, and async/await, JavaScript handles asynchronous operations efficiently.
- Event-driven: JavaScript is widely used for handling events like user actions (clicks, keypresses, etc.) in web development.
- First-class functions: Functions in JavaScript are treated as objects, meaning they can be assigned to variables, passed as arguments, and returned from other functions.
- Data interchange format: JSON is used to exchange data between a server and a client in a readable text format.
- Lightweight: It is easy to read and write, making it an efficient format for data transfer.
- JavaScript Object Notation: JSON is a subset of JavaScript and represents data as key-value pairs.
- Language-independent: Although it is based on JavaScript, JSON is language-independent and can be used in many programming languages.
- Supports objects and arrays: JSON allows you to represent data structures like objects, arrays, and nested data.
- Dynamic Typing: Variables in JavaScript can hold any type of data and their type is determined at runtime.
- Function Scope or Block Scope: Variables declared with var are function-scoped, while those declared with let or const are block-scoped.
- Hoisting: Variables declared with var are hoisted to the top of their scope, meaning they can be used before declaration (though their value will be undefined until initialized).
- Mutable: Variables (except those declared with const) can be reassigned new values.
- Global or Local: Depending on where they are declared, variables can be global (if declared outside functions) or local (if declared within functions).
- Global Scope: Variables declared outside of any function or block are globally accessible throughout the entire script.
- Local Scope: Variables declared within a function or block (using let, const, or var) are only accessible within that function or block.
Take our Javascript skill challenge to evaluate yourself!
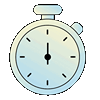
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.