18
AprFiltering Arrays in JavaScript: The Essential Guide
Filter in JavaScript
The filter() in JavaScript is a powerful tool that allows developers to easily create a new array in JavaScript by filtering out elements from an existing one based on specific conditions. Using filter() in JavaScript not only improves code readability but also makes it easier to handle and manipulate large sets of data. By applying filter() in JavaScript, developers can efficiently streamline their code and perform tasks like searching, sorting, and extracting elements without altering the original array.
In this JavaScript tutorial, we will clarify your concepts regarding filter() in JavaScript, including how the filter() method works, practical examples of using filter(), chaining filter() with other array methods, common use cases of the filter() method, advanced filtering techniques, limitations of the filter() method, and much more. To gain a deeper understanding and enhance your JavaScript skills, be sure to enroll in our Free Javascript Course for comprehensive learning.
What is the filter() Method in JavaScript?
The filter() method in JavaScript is used to create a new array containing only the elements that meet a specific condition. Think of it like sorting through a basket of fruits and picking only the ripe ones. It doesn’t change the original basket but gives you a new one with your selection. This makes filter() perfect for tasks like narrowing down data or finding specific values in arrays.
Syntax for filter() Method in JavaScript
array.filter(callbackFunction(element, index, array), thisArg)
Syntax Explanation of Parameters
- array: The array on which the filter() method is called.
- callbackFunction: A function executed on each element of the array to determine if it should be included in the new array.
- element: Represents the current item being processed in the array.
- index (optional): The index of the current element being processed.
- array (optional): The original array on which filter() was called.
- thisArg (optional): A value that can be used as this inside the callback function.
How the filter() Method Works
Step 1: Start with an Array: The filter() method works on arrays. You begin by calling it on an existing array like this:
const numbers = [1, 2, 3, 4, 5];
Step 2: Define a Callback Function: This function specifies the condition that each element must meet to be included in the new array. For example, you might want to filter elements greater than 3, which would look like this:
(element) => element > 3
Step 3: Iterate Through the Array: The filter() method automatically loops through every element in the array and applies the callback function to each one.
Step 4: Evaluate the Condition: For each element, the callback function returns true (include this element) or false (exclude this element). For example, for the callback (element) => element > 3:
- For 1: false (excluded)
- For 4: true (included)
Step 5: Create a New Array: The elements that pass the condition (where the callback function returns true) are added to a new array. The original array remains unchanged.
Step 6: Return the New Array: Once all elements are processed, the filter() method returns the newly created array, like this:
const filtered = numbers.filter((element) => element > 3);
console.log(filtered); // [4, 5]
Key Points to Remember
- Does Not Modify the Original Array: The filter() method creates a new array and leaves the original array unchanged.
- Callback Function is Mandatory: You must provide a valid function as a condition to filter the array. Without it, filter() it won’t work.
- Returns a New Array: If no elements pass the condition, it returns an empty array.
- Flexible and Dynamic: The callback function can be as simple or complex as needed, allowing for dynamic filtering logic.
- Works with Any Data Type: Although commonly used with numbers and strings, you can use filter() with objects, booleans, or even nested arrays.
Example with Step-by-Step Results
const words = ["apple", "banana", "cherry", "date", "fig"];
// Filter words that start with 'b' or 'c'
const filteredWords = words.filter((word) => word.startsWith("b") || word.startsWith("c"));
console.log(filteredWords);
Output
["banana", "cherry"]
Practical Examples of Using filter() in JavaScript
Let us learn the filter() method using some examples.
1. Filter Even Numbers
This example filters out only the even numbers from an array.
Example
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const evenNumbers = numbers.filter((num) => num % 2 === 0);
console.log(evenNumbers);
Output
[ 2, 4, 6, 8 ]
- Filters out even numbers (divisible by 2).
- Returns an array with only the even numbers: [2, 4, 6, 8].
2. Filter Strings with Specific Length
In this example, we filter out strings from an array that have more than 5 characters.
Example
const words = ["apple", "banana", "cherry", "date", "fig"];
const longWords = words.filter((word) => word.length > 5);
console.log(longWords);
Output
[ 'banana', 'cherry' ]
- Filters strings with more than 5 characters.
- Returns an array with the words: ["banana", "cherry"].
3. Filter Positive Numbers
Here, we filter out all negative numbers, leaving only the positive ones.
Example
const numbers = [-10, 20, 15, -5, 0, 30];
const positiveNumbers = numbers.filter((num) => num > 0);
console.log(positiveNumbers);
Output
[ 20, 15, 30 ]
- Filters out negative numbers and zeros.
- Returns only positive numbers: [20, 15, 30].
4. Filter Objects Based on a Property Value
In this example, we filter out objects based on a specific property value.
Example
const people = [
{ name: "John", age: 25 },
{ name: "Alice", age: 17 },
{ name: "Bob", age: 30 },
{ name: "Jane", age: 15 }
];
const adults = people.filter((person) => person.age > 18);
console.log(adults);
Output
[ { name: 'John', age: 25 }, { name: 'Bob', age: 30 } ]
5. Filter Array with Multiple Conditions
This example demonstrates how you can filter an array based on multiple conditions. We want to find even numbers greater than 5.
Example
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const filteredNumbers = numbers.filter((num) => num % 2 === 0 && num > 5);
console.log(filteredNumbers);
Output
[6, 8]
- Filters even numbers that are greater than 5.
- Returns [6, 8] as the output.
Chaining filter() with Other Array Methods
1. Combining filter() with map()
You can use filter() to refine your data and then apply map() to transform the filtered results.
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const squaredEvens = numbers
.filter((num) => num % 2 === 0) // Keep only even numbers
.map((num) => num ** 2); // Square each even number
console.log(squaredEvens);
Output
[ 4, 16, 36, 64 ]
- The filter() method selectsonly the even numbers from the array.
- The map() method transforms the filtered numbers by squaring them.
2. Combining filter() with reduce()
Use filter() to refine your array and then apply reduce() to combinethe filtered values into a single result.
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const sumOfOdds = numbers
.filter((num) => num % 2 !== 0) // Keep only odd numbers
.reduce((sum, num) => sum + num, 0); // Sum up the odd numbers
console.log(sumOfOdds);
Output
25
- The filter() method selects only the odd numbers from the array.
- The reduce() method calculates the sum of the filtered odd numbers.
3. Combining filter() with sort()
You can use filter() to refine your data and then apply sort() to arrange the filtered results in ascending or descending order.
const numbers = [10, 3, 45, 2, 18, 7, 12];
const sortedEvens = numbers
.filter((num) => num % 2 === 0) // Keep only even numbers
.sort((a, b) => a - b); // Sort the even numbers in ascending order
console.log(sortedEvens);
Output
[ 2, 10, 12, 18 ]
- The filter() method selects only the even numbers from the array.
- The sort() method arranges the filtered numbers in ascending order.
Advanced Filtering Techniques
1. Filtering Nested Arrays or Objects
Use filter() to extract elements from nested arrays or objects by accessing their properties.
const students = [
{ name: "Alice", scores: { math: 85, science: 90 } },
{ name: "Bob", scores: { math: 75, science: 65 } },
{ name: "Charlie", scores: { math: 95, science: 88 } },
];
const highMathScores = students.filter(student => student.scores.math > 80);
console.log(highMathScores);
Output
[
{ name: 'Alice', scores: { math: 85, science: 90 } },
{ name: 'Charlie', scores: { math: 95, science: 88 } }
]
- The filter() method evaluates each student's math score.
- It retains only those students whose math scores are greater than 80.
2. Filtering Unique Elements
Remove duplicates from an array using filter() combined with indexOf().
const numbers = [1, 2, 2, 3, 4, 4, 5];
const uniqueNumbers = numbers.filter((value, index, self) => self.indexOf(value) === index);
console.log(uniqueNumbers);
output
[ 1, 2, 3, 4, 5 ]
- he indexOf() method finds the first occurrence of an element.
- The filter() retains only the indexOf() method and finds the first occurrence of an element.
3. Using filter() with Asynchronous Operations
Combine filter() with Promise.all() to handle asynchronous filtering tasks.
const fetchData = async id => ({ id, value: id * 2 });
const ids = [1, 2, 3, 4, 5];
const filteredData = await Promise.all(
ids.map(async id => {
const data = await fetchData(id);
return data.value > 5 ? data : null;
})
).then(results => results.filter(item => item !== null));
console.log(filteredData);
Output
[ { id: 3, value: 6 }, { id: 4, value: 8 }, { id: 5, value: 10 } ]
- The map() method runs asynchronous tasks for each ID and fetches data.
- The filter() removes null values, retaining only data meeting the condition.
Performance Considerations for filter() in JavaScript
There are several performance considerations for filter() in JavaScript you should know:
- Iterates Through All Elements: The filter() method checks each element in the array, making it slower for large datasets if the callback logic is complex.
- Creates a New Array: It returns a new array without changing the original one, which increases memory usage, especially with large arrays.
- Multiple Passes in Chaining: Chaining filter() with other methods like map() or reduce() processes the array multiple times, reducing efficiency.
- Avoid Callback in Loops: Recreating the callback function in loops adds unnecessary overhead; instead, define the function once and reuse it.
- Handles Sparse Arrays: filter() checks all array indices, even for empty or undefined values, which can slightly impact performance.
Limitations of the filter() Method in JavaScript
There are several limitations to the filter() method in JavaScript. You should consider:
- Processes All Elements: The filter() method checks every element, even if some conditions are already met, which can waste time for large arrays.
- One Condition at a Time: It can handle only one condition per call, so combining multiple conditions might require extra steps or other methods.
- Uses More Memory: It creates a new array instead of modifying the original, which can use more memory for large datasets.
- Issues with Sparse Arrays: It skips empty array slots but still processes undefined values, which may cause unexpected results.
- No Built-in Async Support: The filter() method in JavaScript cannot directly handle asynchronous operations, so additional methods like Promise.all() are needed for such tasks.
Conclusion
The filter() method in JavaScript is a powerful tool for extracting array elements based on conditions. It simplifies filtering logic, but developers should be mindful of its limitations, like processing time for large arrays and no direct support for asynchronous tasks. Understanding these aspects helps maximize the benefits of using filter in JavaScript effectively.
FAQs
Take our Javascript skill challenge to evaluate yourself!
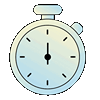
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.