09
Novfor Loop in JavaScript: A Detailed Discussion with Examples
for loop in JavaScript
A for loop in JavaScript is the first and most basic loop control structure. It executes a block of code for a certain number of times until a specified condition is evaluated as false. The JavaScript for loop is similar to the for loop in C, C++, or Java.
In this JavaScript tutorial, we'll explore the for loop control structure in JavaScript and understand its syntax, flowchart, nested, and infinite for loops with examples, etc.
Read More: JavaScript Interview Questions and Answers (Fresher + Experience) |
What is JavaScript for loop?
A for loop is a control structure that enables a set of instructions to get executed for a specified number of iterations until the condition holds. It is an entry-controlled loop.
JavaScript for Loop Flowchart
JavaScript for loop Syntax
for (expression 1; expression 2; expression 3) {
// code block to be executed
}
- Expression 1: Initialization of the Counter Variable
A counter variable keeps track of the number of iterations in the loop. There can be multiple counter-variables initialized in this expression separated by commas. You can also initialize the counter variable externally rather than in expression 1. Hence, this is an optional expression. This expression can also declare variables. We can leave the portion empty with a semicolon.
Example of Initialization of Counter Variables
const names = ["Sourav", "Sakshi", "Girdhar", "Amit"]; let text = ""; //Declare text outside of the loop for (let i = 0, len = names.length; i < len; i++) { text += names[i] + " "; } console.log(`text: ${text}`);
In the above code, we've declared and initialized two variables in the expression 1 of the for loop.
Output
text: Sourav Sakshi Girdhar Amit
Example of for loop without the initializer
let x = 1; for (; x <= 8; x++) { console.log("Value of x:" + x); }
In the above code, we've declared and initialized the counter variable before the for loop.
Output
Value of x:1 Value of x:2 Value of x:3 Value of x:4 Value of x:5 Value of x:6 Value of x:7 Value of x:8
- Expression 2: Test Condition
The test condition must return the true value for the loop to be executed. If the test condition is evaluated as false, the loop will end, and the code outside the loop will be executed. The for loop enters the body of the loop, and iteration starts based on the boolean true result of the test condition. On completion of one iteration of the loop, the condition is re-evaluated. This process will keep repeating until the condition is false.
This is also an optional expression like expression 1. If you do not specify any test condition, it is assumed to be true. But in such cases, you must specify the break keyword in the loop body; otherwise, it'll turn into an infinite for loop. This can crash your browser.
Example of for loop without the test condition
let x = 7; for (; ; x++) { console.log("Value of x:" + x); break; }
Output
Value of x:7
- Expression 3: Updation of the Counter Variable
This expression increments/decrements the value of the counter/initial variable. Hence, it's also called an update expression or iterator. On each update, the test condition is evaluated. The body of the for loop executes until the test condition is evaluated as false. The iterator stops once the test condition becomes false.
Again, this is an optional expression. You can update your counter variable inside the body of the for loop instead of putting it inside the parentheses () of the for loop.
Example of for loop without the update expression
const employees = ["Sourav", "Sakshi", "Girdhar", "Amit"]; let i = 0; let len = employees.length; let text = ""; for (; i < len;) { text += employees[i] + " "; //can be increased inside loop i++; } console.log(text)
In the above code, for (; i < len;) indicates omitted initialization and update expression, focusing only on the test condition.
Output
Sourav Sakshi Girdhar Amit
We saw that all the expressions in the for-loop syntax are optional. So, the syntax of the for loop without any statements is:
JavaScript for loop Syntax without Expressions/Statements
for ( ; ; ) {
// statements
}
Example of for loop Without any Expression
let i = 10;
for (;;) {
if (i > 20) {
break;
}
console.log(i);
i += 2;
}
As all expressions are optional, we've omitted them in the () of the for loop in the above code. Instead, we've initialized the counter variable above the for loop. The test condition and update expression are also mentioned in the body of the for loop.
Output
10
12
14
16
18
20
JavaScript program illustrating a simple for-loop
for (let i = 1; i < 11; i++) {
console.log(i);
}
The above code prints the numbers from 1 to 10 using a for loop in JavaScript.
Iteration | Variable | Condition: i < 11 | Action |
1 | i = 1 | true | 1 is printed. i is increased to 2. |
2 | i = 2 | true | 2 is printed. i is increased to 3. |
3 | i = 3 | true | 3 is printed. i is increased to 4. |
4 | i = 4 | true | 4 is printed. i is increased to 5. |
5 | i = 5 | true | 5 is printed. i is increased to 6 |
6 | i = 6 | true | 6 is printed. i is increased to 7 |
7 | i = 7 | true | 7 is printed. i is increased to 8 |
8 | i = 8 | true | 8 is printed. i is increased to 9 |
9 | i = 9 | true | 9 is printed. i is increased to 10 |
10 | i = 10 | true | 10 is printed. i is increased to 11. |
11 | i = 11 | false | The loop terminates |
Output
1
2
3
4
5
6
7
8
9
10
for loop in JavaScript without the loop body
In JavaScript, you can keep the for loop body empty, i.e., no statements inside the body of the loop. To do this, you need to put the semicolon (;) immediately after the for statement.
Example of JavaScript for loop Without loop Body
let sum = 0;
for (let i = 0; i < 11; i++, sum += i);
console.log(sum);
The above code contains the for-loop statement with initialized counter variable, test condition, and update expression. The program calculates the sum of integers from 0-10. The loop increments the sum within the loop header.
Output
66
Nested for Loops in JavaScript
In JavaScript, it is a for loop inside a for loop. For each cycle of the outer loop, the inner loop completes its entire sequence of iterations.
JavaScript Nested for Loop Flowchart
Example of Nested for Loop in JavaScript
for (let i = 1; i <= 5; i++) {
let line = "";
for (let j = 1; j <= i; j++) {
line += j + " ";
}
console.log(line.trim());
}
- This JavaScript code generates a pattern of numbers using nested loops.
- The inner loop j iterates from 1 to the current value of i, printing numbers and spaces.
- The outer loop i iterates from 1 to 5, regulating the number of rows.
- As a result, there is a pattern of numerals rising in each row, with a new line between each row.
JavaScript Infinite for loop
An infinite for loop gets created if the for loop condition remains true or if the programmer forgets to apply the test condition/terminating statement within the for loop statement.
Example of an infinite for loop in JavaScript
for (let i = 0; true; i++) {
console.log("This loop will run forever!");
}
In the above code, the test condition is explicitly set to true. Hence, this loop will continue executing indefinitely. To save resources, such an infinite loop must be avoided.
Output
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
This loop will run forever!
You can also read: |
Summary
In the above article, we covered the for loop from all angles. We've explained the minute aspects of the loop control structure with proper examples to make your learning smooth and easy. Remember that loop control structures are the fundamental concepts of any programming language, and so are JavaScript. Therefore, in order to learn JavaScript thoroughly, go step by step and clear your fundamentals in our JavaScript Programming Course.
ScholarHat provides various Training and Certification Courses to help you in your end-to-end product development: |
FAQs
Q1. What is a for loop in JavaScript?
Q2. What are the different types of loops in JavaScript?
Q3. How to use a for loop statement in JavaScript?
Take our Javascript skill challenge to evaluate yourself!
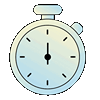
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.