18
JulforEach() in JavaScript
What is JavaScript forEach()?
The forEach() method in JavaScript loops through the array elements. This iterative method executes a predefined function once for each of the array elements in ascending index order, known as a callback function. You cannot execute the forEach() for empty array elements. forEach() always returns undefined; hence, it is not chainable like other iterative methods such as filter(), map(), etc.
In this JavaScript tutorial, we'll learn the forEach() function, including its syntax, parameters, examples, forEach() with arrays, map and sets, comparison of forEach() and for loop and more. To dive deeper into these concepts and enhance your JavaScript skills, be sure to enroll in our Free Javascript Course for a complete learning experience.
Read More: JavaScript Interview Questions and Answers (Fresher + Experience)
Syntax of forEach()
array.forEach(callbackFunction);
array.forEach(callbackFunction, thisValue);
or
array.forEach(function(currentValue, index, array));
Read More: Functions in JavaScript: User-Defined and Standard Library Functions
Parameters:
The forEach() method mainly takes two parameters; one is necessary, and the other is optional:
- Callback function: It holds the function to be executed once on each array element. It can take up to three parameters:
- currentElement: is the current array element being processed at the time the loop occurs. It is the only compulsory parameter.
- index: It is the index of the current element being processed.
- array: The original array forEach() was called upon.
- thisValue: It holds the context to be passed as this is to be used while executing the callback function in Javascript. This is an optional parameter.
forEach() with Arrays
We know that forEach() method iterates over array elements.
Example of displaying the array elements using forEach()
let employee = ['Sourav', 'Sakshi', 'Pradnya'];
// using forEach
employee.forEach(myFunction);
function myFunction(item) {
console.log(item);
}
In the above code, the forEach() method takes the myFunction() function that displays each element of the employee array.
Output
Sourav
Sakshi
Pradnya
forEach() with Arrow Function
The arrow function in Javascript can be used with the forEach() method.
Example of forEach() with Arrow Function
let employee = ['Sourav', 'Sakshi', 'Pradnya'];
employee.forEach(element => {
console.log(element);
});
You can observe that the callback function is defined using an arrow function syntax. It takes one parameter element denoting the current element of the array being processed.
Output
Sourav
Sakshi
Pradnya
Usage of Parameters passed to the Callback Function
1. Using the currentElement parameter
const employeesDetails = [
{ name: "Shailendra Chauhan", age: 50, salary: 40000, designation: "CEO" },
{ name: "Sourav Kumar", age: 30, salary: 30000, designation: "SEO" },
{ name: "Sakshi Dhameja", age: 37, salary: 3700, designation: "Mentor" },
{ name: "Pradnya", age: 27, salary: 20000, designation: "Content Writer" }
];
employeesDetails.forEach((employeeDetail) => {
let sentence = `I am ${employeeDetail.name} an employee of ScholarHat.`;
console.log(sentence);
});
The above JavaScript program iterates over an array of employeeDetails and logs a sentence for each employee to the console.
Output
I am Shailendra Chauhan an employee of ScholarHat.
I am Sourav Kumar an employee of ScholarHat.
I am Sakshi Dhameja an employee of ScholarHat.
I am Pradnya an employee of ScholarHat.
2. Using the index parameter
The index parameter of the callback function helps in getting the index of the array elements.
const employeesDetails = [
{ name: "Shailendra Chauhan", age: 50, salary: 40000, designation: "CEO" },
{ name: "Sourav Kumar", age: 30, salary: 30000, designation: "SEO" },
{ name: "Sakshi Dhameja", age: 37, salary: 3700, designation: "Mentor" },
{ name: "Pradnya", age: 27, salary: 20000, designation: "Content Writer" }
];
employeesDetails.forEach((employeeDetail, index) => {
let sentence = `index ${index} : I am ${employeeDetail.name} an employee of ScholarHat.`;
console.log(sentence);
});
The above JavaScript program iterates over an array of employeeDetails and logs a sentence for each employee along with their index to the console.
Output
index 0 : I am Shailendra Chauhan an employee of ScholarHat.
index 1 : I am Sourav Kumar an employee of ScholarHat.
index 2 : I am Sakshi Dhameja an employee of ScholarHat.
index 3 : I am Pradnya an employee of ScholarHat.
3. Using the array parameter
const employeesDetails = [
{ name: "Shailendra Chauhan", age: 50, salary: 40000, designation: "CEO" },
{ name: "Sourav Kumar", age: 30, salary: 30000, designation: "SEO" },
{ name: "Sakshi Dhameja", age: 37, salary: 3700, designation: "Mentor" },
{ name: "Pradnya", age: 27, salary: 20000, designation: "Content Writer" }
];
employeesDetails.forEach((employeeDetail, index, array) => {
console.log(array);
});
The array parameter outputs the entire array 4 times since we have four array elements, and the iteration occurs four times.
Output
[
{
name: 'Shailendra Chauhan',
age: 50,
salary: 40000,
designation: 'CEO'
},
{ name: 'Sourav Kumar', age: 30, salary: 30000, designation: 'SEO' },
{
name: 'Sakshi Dhameja',
age: 37,
salary: 3700,
designation: 'Mentor'
},
{
name: 'Pradnya',
age: 27,
salary: 20000,
designation: 'Content Writer'
}
]
[
{
name: 'Shailendra Chauhan',
age: 50,
salary: 40000,
designation: 'CEO'
},
{ name: 'Sourav Kumar', age: 30, salary: 30000, designation: 'SEO' },
{
name: 'Sakshi Dhameja',
age: 37,
salary: 3700,
designation: 'Mentor'
},
{
name: 'Pradnya',
age: 27,
salary: 20000,
designation: 'Content Writer'
}
]
[
{
name: 'Shailendra Chauhan',
age: 50,
salary: 40000,
designation: 'CEO'
},
{ name: 'Sourav Kumar', age: 30, salary: 30000, designation: 'SEO' },
{
name: 'Sakshi Dhameja',
age: 37,
salary: 3700,
designation: 'Mentor'
},
{
name: 'Pradnya',
age: 27,
salary: 20000,
designation: 'Content Writer'
}
]
[
{
name: 'Shailendra Chauhan',
age: 50,
salary: 40000,
designation: 'CEO'
},
{ name: 'Sourav Kumar', age: 30, salary: 30000, designation: 'SEO' },
{
name: 'Sakshi Dhameja',
age: 37,
salary: 3700,
designation: 'Mentor'
},
{
name: 'Pradnya',
age: 27,
salary: 20000,
designation: 'Content Writer'
}
]
Conditionals in a forEach() Callback Function
While iterating through arrays, we can check for specific conditions by passing these conditions into our callback function.
const employeesDetails = [
{ name: "Shailendra Chauhan", age: 50, salary: 40000, designation: "CEO" },
{ name: "Sourav Kumar", age: 30, salary: 30000, designation: "SEO" },
{ name: "Sakshi Dhameja", age: 37, salary: 37000, designation: "Mentor" },
{ name: "Pradnya", age: 27, salary: 20000, designation: "Content Writer" }
];
employeesDetails.forEach(({name, salary}) => {
if(salary >= 30000){
console.log(name);
}
});
The above code checks the salary of all the employees in the array and prints the one having a salary greater than 40000.
Output
Shailendra Chauhan
Sourav Kumar
Sakshi Dhameja
forEach() vs. for loop in JavaScript/for loop to forEach()
The forEach() and for loop in JavaScript resemble very much, except for some differences.
Example Illustrating for loop in JavaScript
const names = ["Sourav", "Sakshi", "Girdhar", "Amit"];
let text = "";
for (let i = 0, len = names.length; i < len; i++) {
text += names[i] + " ";
}
console.log(`text: ${text}`);
In the above code, the for loop iterates over the names array, appending the current element to the text string in each iteration.
Output
text: Sourav Sakshi Girdhar Amit
Now, we'll convert the for loop in the above code into forEach().
const names = ["Sourav", "Sakshi", "Girdhar", "Amit"];
let text = "";
names.forEach(name => {
text += name + " ";
});
console.log(`text: ${text}`);
The forEach method iterates over the elements in the names array, and the arrow function appends each name to the text variable.
Output
text: Sourav Sakshi Girdhar Amit
In the forEach(), you cannot use the break or continue statement to control the loop like for loop. To terminate the loop in the forEach() method, you must throw an exception inside the callback function.
forEach() with Sets
const set = new Set(["Sourav", "Sakshi", "Girdhar", "Amit"]);
set.forEach(myFunction);
function myFunction(item) {
console.log(item);
}
Output
Sourav
Sakshi
Girdhar
Amit
forEach() with Maps
Read More: Map in Javascript
let map = new Map();
// inserting elements
map.set('name', 'Sakshi');
map.set('designation', 'Mentor');
// looping through Map
map.forEach (myFunction);
function myFunction(value, key) {
console.log(key + ': ' + value);
}
Output
name: Sakshi
designation: Mentor
Summary
We saw the forEach() function in quite detail and understood its applications through various examples. forEach() uses callback functions, which is a fundamental concept in JavaScript asynchronous programming. You need to be through with it to understand the forEach() smoothly. You can explore this topic much more in our JavaScript Programming Course.
FAQs
Take our Javascript skill challenge to evaluate yourself!
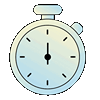
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.