21
FebTop 50 JavaScript Array Questions and Answer
JavaScript Array Interview Questions
If you're preparing for a JavaScript interview, having a strong understanding of JavaScript Arrays is essential. You might be asked to explain various Array Methods in JavaScript, their properties, and code implementations using JavaScript's built-in Array functionalities. Being familiar with array manipulation, iteration, filtering, and sorting, as well as understanding performance and immutability, is highly beneficial.
In this JavaScript tutorial, we’ll explore the top JavaScript Array interview questions with clear examples and explanations. By the end, you’ll be ready to confidently tackle any array-related question and apply these concepts in real-world scenarios. To further strengthen your understanding and prepare for interviews, you can enroll in a Free JavaScript Course With Certificate. This course will provide you with in-depth knowledge and practical experience in working with arrays and other JavaScript concepts.
What to Expect in JavaScript Array Interview Questions
JavaScript Array Interview Questions for Freshers
1. What does the concat()
method do in JavaScript?
Ans: The concat()
method is used to join two or more arrays together. It does not change the original arrays. Instead, it creates and returns a new array that contains all the elements of the arrays you passed to it.
let arr1 = [1, 2, 3];
let arr2 = [4, 5, 6];
let result = arr1.concat(arr2);
console.log(result);
Output
[1, 2, 3, 4, 5, 6]
2. What is the indexOf()
method used for?
Ans: The indexOf()
method helps you find the position of the first occurrence of a specified element in an array. If the element is not found, it will return -1
.
let arr = [10, 20, 30, 40];
let index = arr.indexOf(30);
console.log(index);
Output
2
3. How does the push()
method work?
Ans: The push()
method adds one or more elements to the end of an array and gives you back the new length of the array.
let arr = [1, 2, 3];
arr.push(4, 5);
console.log(arr);
Output
[1, 2, 3, 4, 5]
4. What does the pop()
method do?
Ans: The pop()
method removes the last element from an array and gives you that element. The array's length will also decrease.
let arr = [10, 20, 30];
let lastElement = arr.pop();
console.log(lastElement);
console.log(arr);
Output
30
[10, 20]
5. What is the shift()
method?
Ans: The shift()
method removes the first element from an array and gives you that element. It also reduces the size of the array.
let arr = [1, 2, 3];
let firstElement = arr.shift();
console.log(firstElement);
console.log(arr);
Output
1
[2, 3]
6. How does the unshift()
method work?
Ans: The unshift()
method adds one or more elements to the beginning of an array and gives back the new length of the array.
let arr = [2, 3, 4];
arr.unshift(1);
console.log(arr);
Output
[1, 2, 3, 4]
7. What is the slice()
method?
Ans: The slice()
method returns a new array that contains a portion of the original array. It does not modify the original array. You pass the start index and end index (exclusive) to select the portion you want.
let arr = [10, 20, 30, 40, 50];
let newArr = arr.slice(1, 4);
console.log(newArr);
Output
[20, 30, 40]
8. What does the splice()
method do?
Ans: The splice()
method changes the contents of an array. You can use it to remove, add, or replace elements at any position in the array. Unlike other methods, it directly modifies the original array.
let arr = [10, 20, 30, 40, 50];
arr.splice(2, 2, 100, 200);
console.log(arr);
Output
[10, 20, 100, 200, 50]
9. How does the reverse()
method work?
Ans: The reverse()
method reverses the order of the elements in the array. It changes the array in place and returns the reversed array.
let arr = [1, 2, 3, 4, 5];
arr.reverse();
console.log(arr);
Output
[5, 4, 3, 2, 1]
10. What is the sort()
method used for?
Ans: The sort()
method is used to arrange the elements of an array in ascending order. If you want a different order, you can provide a custom function to control how the sorting is done.
let arr = [40, 10, 100, 20];
arr.sort((a, b) => a - b);
console.log(arr);
Output
[10, 20, 40, 100]
11. What does the join()
method do?
Ans: The join()
method combines all elements of an array into a single string, using a separator like a comma, space, or custom string. If no separator is provided, it defaults to a comma.
let arr = [10, 20, 30];
let result = arr.join("-");
console.log(result);
Output
10-20-30
12. What is the difference between map()
and forEach()
?
Ans: map()
creates a new array by applying a function to each element, whereas forEach()
just executes a function on each element without returning a new array.
13. How does the filter()
method work?
Ans: The filter()
method creates a new array with elements that pass a test in a callback function. If no elements match, it returns an empty array.
let numbers = [1, 2, 3, 4, 5];
let result = numbers.filter(num => num % 2 === 0);
console.log(result);
Output
[2, 4]
14. What does the every()
method do?
Ans: every()
checks if all elements in an array satisfy a condition. It returns true
if all match, otherwise false
.
let numbers = [2, 4, 6];
let result = numbers.every(num => num % 2 === 0);
console.log(result);
Output
true
15. How does the some()
method work?
Ans: some()
checks if any element in the array satisfies a condition. It returns true
if any match, otherwise false
.
let numbers = [1, 3, 5];
let result = numbers.some(num => num % 2 === 0);
console.log(result);
Output
false
16. What is the reduce()
method used for?
Ans: The reduce()
method reduces an array to a single value by applying a function to an accumulator and each element.
let numbers = [1, 2, 3];
let sum = numbers.reduce((acc, num) => acc + num, 0);
console.log(sum);
Output
6
17. What is the reduceRight()
method?
Ans: reduceRight()
works like reduce()
, but it processes the array from right to left.
let numbers = [1, 2, 3];
let result = numbers.reduceRight((acc, num) => acc - num, 0);
console.log(result);
Output
-4
18. What is an array-like object in JavaScript?
Ans: An array-like object has a length
property and indexed elements but lacks array methods like push()
. Examples include NodeList
and the arguments
object.
function example() {
console.log(arguments);
}
example(1, 2, 3);
Output
[1, 2, 3]
19. What is the find()
method used for?
Ans: The find()
method returns the first element that satisfies a condition, or undefined
if no match is found.
let numbers = [1, 2, 3, 4];
let result = numbers.find(num => num > 2);
console.log(result);
Output
3
20. How does the from()
method work?
Ans: Array.from()
creates a new array from an array-like or iterable object, such as a string or NodeList
.
let str = "hello";
let result = Array.from(str);
console.log(result);
Output
["h", "e", "l", "l", "o"]
21. What is the difference between let
and var
?
Ans: let
is block-scoped, while var
is function-scoped. let
prevents redeclaration in the same scope.
let a = 10;
{
let a = 20;
console.log(a); // 20
}
console.log(a); // 10
Output
20
10
22. What is the purpose of const
in JavaScript?
Ans: const
defines a variable whose value cannot be reassigned, though the contents of objects or arrays can still be modified.
const numbers = [1, 2, 3];
numbers.push(4); // Allowed
// numbers = [4, 5, 6]; // Error: Assignment to constant variable
console.log(numbers);
Output
[1, 2, 3, 4]
23. What is the bind()
method in JavaScript?
Ans: The bind()
method allows you to create a new function with its this
context explicitly set to a specified object. This is particularly useful when passing functions as callbacks, ensuring that the function retains its original context regardless of how it is invoked.
let person = {
firstName: 'John',
lastName: 'Doe'
};
function greet() {
console.log('Hello, ' + this.firstName + ' ' + this.lastName);
}
let greetPerson = greet.bind(person);
greetPerson();
Output
Hello, John Doe
24. What are arrow functions in JavaScript?
Ans: Arrow functions in JavaScript provide a shorter syntax for writing functions. They also handle the this
keyword differently than regular functions, inheriting this
from the surrounding context.
let sum = (a, b) => a + b;
console.log(sum(3, 4));
Output
7
25. What is a promise in JavaScript?
Ans: A promise is an object representing the eventual completion or failure of an asynchronous operation. It can be in one of three states: pending, fulfilled, or rejected. Promises are commonly used to handle asynchronous operations like API calls.
let promise = new Promise((resolve, reject) => {
let success = true;
if (success) {
resolve('Operation was successful!');
} else {
reject('Operation failed');
}
});
promise.then(result => console.log(result)).catch(error => console.log(error));
Output
Operation was successful!
26. What is the async/await
syntax in JavaScript?
Ans: The async/await
syntax is used to work with asynchronous code in a more readable way. async
It makes a function return a promise and await
pauses the function execution until the promise is resolved.
async function fetchData() {
let response = await fetch('https://api.example.com/data');
let data = await response.json();
console.log(data);
}
fetchData();
27. What is the this
keyword in JavaScript?
Ans: The this
keyword refers to the context in which a function is called. In regular functions, this
refers to the global object (or undefined in strict mode), while in object methods, it refers to the object itself.
Read More: This Keyword in Java |
28. What is event delegation in JavaScript?
Ans: Event delegation is a technique for handling events at a higher level in the DOM rather than attaching event listeners to individual elements. This improves performance, especially when dealing with dynamically added elements.
document.getElementById('parent').addEventListener('click', function(event) {
if (event.target && event.target.matches('button.classname')) {
console.log('Button clicked!');
}
});
29. What is a closure in JavaScript?
Ans: A closure is a function that has access to its own scope, the outer function's scope, and the global scope. This allows functions to remember and access variables from their lexical environment even after the outer function has finished execution.
function outer() {
let counter = 0;
return function inner() {
counter++;
console.log(counter);
};
}
let increment = outer();
increment();
increment();
Output
1
2
30. What is the difference between ==
and ===
?
Ans: ==
is the equality operator that compares values, allowing type coercion. ===
is the strict equality operator that compares both values and types, without allowing type conversion.
Read More: Understanding Type Casting or Type Conversion in C# |
31. What is the Array.prototype.map()
method?
Ans: The map()
method creates a new array populated with the results of calling a provided function on every element in the calling array. It doesn’t change the original array.
let numbers = [1, 2, 3, 4];
let squares = numbers.map(num => num * num);
console.log(squares);
Output
[1, 4, 9, 16]
32. What is the Array.prototype.filter()
method?
Ans: The filter()
method creates a new array with all elements that pass the test implemented by the provided function. It doesn’t change the original array.
let numbers = [1, 2, 3, 4, 5];
let evenNumbers = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers);
Output
[2, 4]
33. What is the Array.prototype.reduce()
method?
Ans: The reduce()
method applies a function against an accumulator and each element in the array to reduce it to a single value.
let numbers = [1, 2, 3, 4];
let sum = numbers.reduce((acc, num) => acc + num, 0);
console.log(sum);
Output
10
34. What is the setTimeout()
function?
Ans: The setTimeout()
function is used to execute a function or code snippet after a specified delay (in milliseconds).
setTimeout(() => console.log('Hello, world!'), 2000);
Output
Hello, world! (after 2 seconds)
35. What is the setInterval()
function?
Ans: The setInterval()
function calls a function repeatedly with a fixed time delay between each call.
setInterval(() => console.log('Repeating message'), 1000);
Output
Repeating message (every second)
JavaScript Array Interview Questions for Experienced
36. What are JavaScript modules?
Ans: JavaScript modules allow you to split code into separate files, making it easier to manage and maintain. Modules can export and import Variables in JavaScript, functions, or objects from other files using the export
and import
keywords.
// file1.js
export const greeting = 'Hello World';
// file2.js
import { greeting } from './file1.js';
console.log(greeting);
Output
Hello World
37. What is the Event Loop
in JavaScript?
Ans: The event loop is a fundamental part of JavaScript's concurrency model. It allows JavaScript to perform non-blocking operations by executing the code, collecting events, and executing sub-tasks in the order they are received, allowing asynchronous tasks to be handled.
38. Explain the concept of hoisting
in JavaScript.
Ans: Hoisting is JavaScript’s behavior of moving declarations to the top of their containing scope before code execution. Variables declared with var
are hoisted, while those declared with let
and const
are hoisted but remain uninitialized until the code execution reaches them.
39. What are higher-order functions in JavaScript?
Ans: A higher-order function is a function that takes one or more functions as arguments or returns a function as its result. These are common in functional programming.
function multiplyBy(factor) {
return function (num) {
return num * factor;
};
}
const double = multiplyBy(2);
console.log(double(5)); // 10
Output
10
40. What is throttling
and debouncing
in JavaScript?
Ans: Throttling and debouncing are techniques used to limit the number of times a function is executed. Throttling ensures that a function is called no more than once at every specified time interval while debouncing ensures the function is only executed after a specified delay after the last call.
41. What is the Prototype Chain
in JavaScript?
Ans: The prototype chain is a mechanism that allows objects to inherit properties and methods from other objects. Every object in JavaScript has a prototype, and when you try to access a property or method, the search for it happens in the prototype chain if it doesn't exist in the object itself.
42. What is a WeakMap
in JavaScript?
Ans: A WeakMap
is a collection of key-value pairs where the keys must be objects, and the values can be any type. WeakMaps do not prevent garbage collection, meaning if there are no other references to the key object, it can be garbage collected.
Read More: Garbage Collection in C# |
43. What is the difference between null
and undefined
?
Ans: null
represents the intentional absence of any value, while undefined
means a variable has been declared but has not yet been assigned a value. In other words, undefined
is the default value of uninitialized variables.
44. What is the Map
object in JavaScript?
Ans: A Map
object holds key-value pairs where both the keys and values can be of any type. Map in JavaScriptremember the insertion order of their elements and offer better performance when frequent additions and removals are needed compared to regular objects.
let map = new Map();
map.set('name', 'John');
map.set('age', 30);
console.log(map.get('name')); // John
Output
John
45. What are async iterators in JavaScript?
Ans: Async iterators are a type of iterator that allows you to loop over asynchronous data. They work with the for-await-of
loop and can handle promises that resolve with asynchronous data streams.
46. What is requestAnimationFrame()
in JavaScript?
Ans: The requestAnimationFrame()
method tells the browser to call a specified function to update an animation before the next repaint, ensuring smooth animations. It’s more efficient than using setTimeout()
or setInterval()
for animations.
function animate() {
// Animation code
console.log('Animating...');
requestAnimationFrame(animate);
}
requestAnimationFrame(animate);
Output
Animating... (calls indefinitely)
47. What is a Service Worker
in JavaScript?
Ans: A Service Worker is a JavaScript script that runs in the background and manages caching, push notifications, and background sync for web apps, enabling offline functionality and improving performance.
48. How can you handle errors in JavaScript?
Ans: JavaScript provides the try...catch
block to handle exceptions. You can try executing a block of code, and if an error occurs, it can be caught and handled inside the catch
block.
try {
let result = riskyFunction();
} catch (error) {
console.error('Error:', error.message);
}
Output
Error: [Error message here]
49. What are Web Workers
in JavaScript?
Ans: Web Workers allow JavaScript to run scripts in background threads, enabling you to perform complex computations without blocking the main thread, improving the performance of web applications.
50. What is Object.freeze()
in JavaScript?
Ans: Object.freeze()
is used to make an object immutable, meaning its properties cannot be modified, added, or deleted. This can prevent accidental modifications to objects.
let obj = { name: 'John' };
Object.freeze(obj);
obj.name = 'Jane'; // This will not change the name
console.log(obj.name); // John
Output
John
Read More: JavaScript Interview Questions & Answers |
Summary
This tutorial covered the top 50 JavaScript Array interview questions and answers, categorized based on experience levels: fresher, intermediate, and experienced. It provides a comprehensive understanding of keyconcepts of JavaScript Array, from basic operations to advanced array methods and techniques. By reviewing these questions, you'll be well-prepared to excel in interviews and showcase your proficiency with arrays in JavaScript.
Unlock your JavaScript skills with Scholarhat's JavaScript Course! Enroll now and master JavaScript to create efficient, scalable web applications.
FAQs
if (array.length > 0) { console.log("Array has values"); } else { console.log("Array is empty"); }
Take our Javascript skill challenge to evaluate yourself!
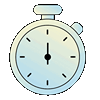
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.