13
SepVariables in JavaScript: Declaration, Types, Scope
What is a Variable in JavaScript Programming?
A variable is a named storage location for data in memory. The data stored in the variable can later be modified.
Example
var x = 8;
In this JavaScript tutorial, we'll learn about JavaScript variables, the declaration, and initialization of variables in JavaScript using var, let, and const keywords, JavaScript variables' scope, dynamic typing, and more.
Read More: JavaScript Interview Questions and Answers (Fresher + Experience)
Rules for Naming JavaScript Variables
Variable names are called as identifiers. Identifiers must be unique.
- Names can consist of letters (both uppercase and lowercase), digits, and underscores (_).
- The first character must be either an alphabet, an underscore (_), or a dollar sign ($).
- Variable names are case sensitive, i.e., num and Numare are two different variable names.
- A keyword cannot be your variable name. e.g. var, function, return.
Declaration of Variables in JavaScript
We can declare variables in JavaScript in three ways:
1. Using the var keyword
Initially, all variables in JavaScript were declared using the var keyword until ES6 was introduced. It declares variables with function scope or globally.
Syntax
var variablename;
Example
var name;
2. Using the let keyword
The let keyword was introduced in the ES6 version of JavaScript. You cannot declare a duplicate variable using the let keyword with the same name and case, and JavaScript will throw a syntax error.
Syntax
let variablename;
Example
let name;
3. Using the const keyword
The const keyword in JavaScript defines variables that cannot be modified once they’ve been assigned a value.
Syntax
const const_name;
Example
const x;
Initialization of Variables in JavaScript
The assignment operator "=" is used to assign a value to a variable when you declare it or after the declaration and before accessing it.
Example of Variable Declaration using let keyword
// variable declaration
let num;
// initialization of the declared variable
num = 50;
console.log(num)
Output
50
Example of Variable Declaration using the var keyword
var str = "Welcome to ScholarHat";
var x = 14;
var y = 20;
var z = x + y;
console.log(str);
console.log(x);
console.log(y);
console.log(z);
Output
Welcome to ScholarHat
14
20
34
Example of Variable Declaration and Initialization in the same line
let name = "ScholarHat"; //assigned string value
let num = 150; //assigned numeric value
let isCompleted = true; //assigned boolean value
console.log(name)
console.log(num)
console.log(isCompleted)
Output
ScholarHat
150
true
Example of Multiple Variables Declaration and Initialization in the same line
let name = "ScholarHat", num = 150, isCompleted = true;
console.log(name)
console.log(num)
console.log(isCompleted)
Output
ScholarHat
150
true
Variable Declaration without Initialization
The default value of variables that do not have any value is undefined.
let num;
console.log(num);
Output
undefined
Change the Value of Variables
We can change the value of a variable after assigning it.
let marks = 500;
console.log(marks);
// change the value of marks
marks = 300;
console.log(marks);
Output
500
300
Types of Variables/Scope of Variables in JavaScript
1. JavaScript global variables
Global variables in JavaScript are variables declared outside of any function or block scope. They are declared at the beginning of the program and can be accessed from any part of the program. Variables declared without the var keyword inside any function become global variables automatically.
Example of Global Variables in JavaScript
// Declaring global variables using var keyword
var globalVar1 = "Welcome";
var globalVar2 = "Students";
// Declaring global variables using let keyword
let globalVar3 = "to the";
let globalVar4 = "JavaScript tutorial";
// Declaring global variables using const keyword
const PI = 3.14;
const CompanyName = "ScholarHat";
// Accessing and printing global variables
console.log(globalVar1 + " " + globalVar2);
console.log(globalVar3 + " " + globalVar4);
console.log("The value of PI is " + PI);
console.log("Company Name: " + CompanyName);
Output
Welcome Students
to the JavaScript tutorial
The value of PI is 3.14
Company Name: ScholarHat
2. JavaScript local variable
Local variables are declared and initialized at the start of a function or block and allocated memory inside that execution scope.
Example of Local Variables in JavaScript
function addNumbers(a, b) {
// Local variable 'result' declared within the function
let result = a + b;
// Local variable 'message' declared within the function
let message = "The sum is: " + result;
console.log(message);
}
addNumbers(5, 7); // function call
console.log(result);
In the above code, the result and message are local variables declared inside the function addNumbers(). Hence, the variable result could not be accessible outside the addNumbers() function.
Output
The sum is: 12
/index.js:13
console.log(result);
^
ReferenceError: result is not defined
at Object. (/index.js:13:13)
at Module._compile (node:internal/modules/cjs/loader:1103:14)
at Object.Module._extensions..js (node:internal/modules/cjs/loader:1157:10)
at Module.load (node:internal/modules/cjs/loader:981:32)
at Function.Module._load (node:internal/modules/cjs/loader:822:12)
at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:77:12)
at node:internal/main/run_main_module:17:47
Variable Declaration without var and let keywords
You can declare or initialize a variable without using the var and let keywords. However, to do so, a value must be assigned to the variable. Such variables become global variables automatically, regardless of their declaration place.
function myfunction(){
msg = "Hello JavaScript!";
}
myfunction();
console.log(msg)
In the above code, the variable msg is declared inside the function myfunction() without using var or let keywords. Hence, the variable msg becomes a global variable. Therefore, it is accessed outside the function.
Output
Hello JavaScript!
Dynamic Typing
In JavaScript, there's no need to specify the type of data a variable will contain. You can update the value of any type after initialization. It is also called dynamic typing.
let variable = 10; //numeric value
variable = 'ScholarHat'; //string value
variable = 10.7; //decimal value
variable = true; //Boolean value
variable = null; //null value
console.log(variable)
In the above code, we declared the variable named "variable" with an integer value. After that, we initialized the variable with different values.
Output
null
Also read similar articles:
- Variables in C++ Programming
- Variables in C Programming - Types of Variables in C ( With Examples )
- Variables in Java
- What are Python Variables - Types of Variables in Python Language
- Variables in C#: Types of Variables with Examples
Summary
Becoming familiar with variables in JavaScript is essential for creating successful programming solutions. Understanding how to declare and use a variable correctly will help developers structure their code correctly. Experimenting with different techniques and researching the different types of variables can also give valuable insight into how each type of variable behaves. Take the time to practice and understand JavaScript variables, and consider pursuing a JavaScript Certification Course to demonstrate your skills to potential employers.
Take our Javascript skill challenge to evaluate yourself!
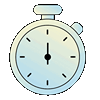
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.