18
AprMastering Loops in JavaScript: A Comprehensive Guide
Loops in JavaScript
A loop in JavaScript is a programming structure that repeats a block of code multiple times based on a condition. It helps save time and effort by automating repetitive tasks, like processing arrays or generating repetitive outputs. By using the loop in JavaScript, you can perform tasks efficiently, making your code more concise and reducing the number of error occurrences.
In the JavaScript Tutorial, let's understand some important concepts related to loops in JavaScript, including types of loops in javascript, for loop, while loop,do...while loop,using break statements, using continue statements, nesting loops in JavaScript, and a lot more. To master these concepts and more, you can enroll in a Free JavaScript Course With Certificate. This course will provide you with the knowledge and hands-on experience needed to excel in JavaScript loops and other essential programming techniques.
What is a loop in JavaScript?
A loop in JavaScript is like a worker repeating a task until it's finished, such as scanning items at a store. Loop in JavaScript helps avoid writing repetitive code by automating repetitive tasks. Loops are essential for iterating through data, processing lists, and running actions multiple times. Without them, code would be longer, less efficient, and harder to manage.
Let's go over some important points for loops in JavaScript:
- Loops save time by automating repetitive tasks.
- They help make your code shorter and easier to read.
- Loops can be controlled with conditions to stop at the right time.
- Common types of loops in JavaScript are for, while, and do...while.
Types of Loops in JavaScript
There are five types of loops in JavaScript:
- For Loop
- While Loop
- Do...While Loop
- For...In Loop
- For...Of Loop
1. for Loops in JavaScript
The for loop is one of the most common loops in JavaScript. for loop in JavaScript is used when you know in advance how many times you need to repeat a block of code. The syntax of a for loop consists of three main parts: initialization, condition, and increment/decrement.
Syntax
for (initialization; condition; increment/decrement) {
// Code to execute on each iteration
}
- Initialization: Sets up a counter variable (e.g., let i = 0).
- Condition: The condition is checked before each iteration (e.g., i < 10). The loop runs as long as this condition is true.
- Increment/Decrement: After each iteration, the counter is updated (e.g., i++ for increment).
Example 1: Iterating Over a Range of Numbers
for (let i = 1; i <= 5; i++) {
console.log(i);
}
This loop starts at i = 1 and increments by 1 until i exceeds 5.
Example 2: Using a For Loop with an Array
let fruits = ["apple", "banana", "cherry"];
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
output
apple
banana
cherry
In this example, the for loop iterates over an array of fruits, accessing each element using its index.
Common Use Cases
- Iterating over arrays or lists: The for loop is commonly used to access elements in an array by index.
- Repetitive tasks: It’s great for performing a task a specific number of times, like printing numbers or executing calculations.
Key Points to Remember
- Initialization: Defines the starting point (usually a counter variable).
- Condition: Determines when the loop should stop; if false, the loop ends.
- Increment/Decrement: Updates the counter variable after each iteration (e.g., i++ for increment or i-- for decrement).
2. While Loop in JavaScript
The while loop in JavaScript is used when you want to repeat a block of code as long as a specific condition is true. Unlike the for loop, you don't need to know the number of iterations in advance; the loop will continue as long as the condition remains true.
Syntax
while (condition) {
// Code to execute as long as the condition is true
}
- Condition: The condition is checked before each iteration. If the condition evaluates to true, the loop continues.
- The loop stops when the condition becomes false.
Example 1: Simple Counter Example
let i = 1;
while (i <= 5) {
console.log(i); // Output: 1, 2, 3, 4, 5
i++; // Increment counter to avoid infinite loop
}
Output
1
2
3
4
5
In this example, the loop starts with i = 1 and runs until i is greater than 5. The counter is incremented inside the loop to prevent the loop from running indefinitely.
Example 2: Infinite Loop Example (and How to Avoid It)
let i = 1;
while (i <= 5) {
console.log(i);
// Missing i++ would lead to an infinite loop
}
Output
1
1
1
1
1 ........
Without the i++, the value of i would never change, causing the loop to run infinitely. To avoid an infinite loop, always ensure that the loop's condition is met or that the loop variable is updated within the loop body.
When to Use While Loops Over Other Loops:
- Unknown number of iterations: Use a while loop when the number of iterations isn't known in advance and the loop should continue until a specific condition is met (e.g., waiting for a user input or a variable to reach a certain value).
- Event-driven or condition-based tasks: A while loop is ideal when you need to repeat actions based on dynamic conditions rather than a fixed number of times (like waiting for data or checking a sensor's status).
4. Do...While Loop in JavaScript
The do...while loop in JavaScript is similar to the while loop but with a key difference: it always executes the code block at least once, even if the condition is false. This is because the condition is checked after the code block executes, not before.
Syntax
do {
// Code to execute
} while (condition);
- Code Block: The code inside the do block runs first.
- Condition: After executing the code block, the condition is checked. If it's true, the loop runs again. If it's false, the loop stops.
Example: Ensuring the Loop Executes At Least Once
let i = 1;
do {
console.log(i); // Output: 1
i++;
} while (i <= 0); // The condition is false, but the code inside runs once
Output
1
In this example, the condition is false from the start (i <= 0), but the loop still executes once because the condition is checked after the code block runs.
Practical Use Cases of do...while Loops
- User Input Validation: Ensures that a block of code runs at least once, such as prompting a user for valid input. The code will keep running until the input meets the condition.
let userInput; do { userInput = prompt("Please enter a number greater than 10:"); } while (parseInt(userInput) <= 10);
- Menu Systems: In applications with menus where the user has to select an option, a do...while loop ensures the menu displays at least once and keeps showing it until the user chooses to exit.
- Repeating Actions with Conditions: When you need an action (e.g., updating a game score, sending requests) to be executed at least once, regardless of a condition.
5. For...In Loop in JavaScript
The for...in loop in JavaScript is used to iterate over the enumerable properties of an object. It allows you to loop through all the keys (or property names) of an object, making it useful for working with objects and their properties.
Syntax Breakdown and Explanation
for (let key in object) {
// Code to execute for each key in the object
}
- Key: In each iteration, key will represent the name of the current property in the object.
- Object: The loop will iterate over all enumerable properties of the specified object.
Example: Iterating Over Object Properties
let person = {
name: "John",
age: 30,
city: "New York"
};
for (let key in person) {
console.log(key + ": " + person[key]); // Output: name: John, age: 30, city: New York
}
Output
name: John
age: 30
city: New York
In this example, the for...in loop iterates over all the properties (name, age, city) of the person object, logging both the property name and its value.
Key Differences from Other Loops:
- Works with Objects: Unlike the for loop or while loop, which is typically used for arrays, the for...in the loop is designed for iterating over the keys (properties) of objects.
- Not for Array Indexes: It should not be used for iterating over arrays, as it may not iterate over array elements in a predictable order (use for...of or traditional for loop for arrays).
Common Pitfalls to Avoid
Iteration Over Inherited Properties: The for...in loop iterates over all enumerable properties, including inherited ones. You can avoid this by using hasOwnProperty() to check if the property is directly on the object.
Unintended Changes to Arrays: Using for...in on arrays can lead to unexpected behavior, such as iterating over non-numeric keys or prototype properties. Always use for...in with objects.
Not Suitable for Array Iteration: Although you can technically use for...in to loop over an array, it's not recommended. Use for...of for arrays instead, as it's designed to work with iterable objects like arrays.
5. For...Of Loop
The for...of loop in JavaScript is used to iterate over iterable objects like arrays, strings, and other data structures that have an iterable protocol. Unlike the for...in loop, which iterates over the keys of an object, the for...of loop iterates directly over the values of an iterable.
Syntax
for (let value of iterable) {
// Code to execute for each value in the iterable
}
- Value: In each iteration, the value represents the value of the current item in the iterable object.
- Iterable: The loop will iterate over all elements of the iterable object (e.g., array, string).
Example: Iterating Over Arrays, Strings, and Iterable Objects
//Iterating over an array
let numbers = [10, 20, 30];
for (let num of numbers) {
console.log(num); // Output: 10, 20, 30
}
//Iterating over a string
let text = "hello";
for (let char of text) {
console.log(char); // Output: h, e, l, l, o
}
//Iterating over a Set
let set = new Set([1, 2, 3, 4]);
for (let item of set) {
console.log(item); // Output: 1, 2, 3, 4
}
Output
10
20
30
h
e
l
l
o
1
2
3
4
Use Cases of for...of Loops
1. Iterating over Arrays and Strings: Access elements or characters directly without worrying about indexes.
Example
let fruits = ["apple", "banana", "cherry"];
for (let fruit of fruits) {
console.log(fruit); // Output: apple, banana, cherry
}
Output
apple
banana
cherry
2. Working with Iterables like Sets and Maps: Retrieve unique values or key-value pairs seamlessly.
Example
let userRoles = new Map([
["admin", "Alice"],
["editor", "Bob"],
]);
for (let [role, name] of userRoles) {
console.log(`${role}: ${name}`); // Output: admin: Alice, editor: Bob
}
Output
admin: Alice
editor: Bob
Advantages of for...of Loops
1. Simpler Syntax: Directly accesses values, making the code cleaner and easier to understand.
Example
let numbers = [10, 20, 30];
for (let num of numbers) {
console.log(num); // Cleaner than a traditional loop
}
Output
10
20
30
2. Compatible with All Iterables: Works with arrays, strings, sets, maps, and other iterable objects.
Example
let text = "hello";
for (let char of text) {
console.log(char);
}
Output
h
e
l
l
o
Comparison with for...in Loop
- for...in Loop: Iterates over the keys of an object or the indices of an array, which can be less predictable and should be avoided for arrays where order matters.
for...of Loop: This method directly accesses the values of iterable objects, making it more appropriate for arrays, strings, and otheriterable objects where the actual values are required.
Example
let arr = [10, 20, 30];
// `for...in` iterates over the indices (0, 1, 2)
for (let index in arr) {
console.log(index);
}
// `for...of` iterates over the values (10, 20, 30)
for (let value of arr) {
console.log(value);
}
Output
0
1
2
10
20
30
Breaking and Skipping Loop Iterations
Using break Statement
The break statement is used to terminate a loop immediately when a specific condition is met. It exits the loop entirely and resumes execution after the loop.
Practical Scenarios
- Exiting a loop early when a desired element is found.
- Stopping a loop when a condition occurs, such as encountering invalid data.
Example
for (let i = 0; i < 10; i++) {
if (i === 5) {
break; // Terminates the loop when i equals 5
}
console.log(i);
}
Output
0
1
2
3
4
Using continue Statement
The continue statement skips the current iteration of a loop when a specified condition is true and proceeds to the next iteration.
Practical Scenario
- Skipping specific elements, such as filtering out unwanted values.
- Avoid unnecessary processing during certain loop iterations.
Example
for (let i = 0; i < 10; i++) {
if (i % 2 === 0) {
continue; // Skips even numbers
}
console.log(i);
}
Output
1
3
5
7
9
Nesting Loops in JavaScript
Nested loops in JavaScript are loops placed inside another loop. The inner loop executes completely for each iteration of the outer loop. This is useful when dealing with multi-dimensional data structures or performing repetitive tasks within repetitive contexts.
Example 1: Generating a Multiplication Table
for (let i = 1; i <= 5; i++) {
let row = "";
for (let j = 1; j <= 5; j++) {
row += `${i * j}\t`; // Calculate product and format
}
console.log(row);
}
Output
1 2 3 4 5
2 4 6 8 10
3 6 9 12 15
4 8 12 16 20
5 10 15 20 25
Example 2: Iterating Over a 2D Array
let matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9],
];
for (let i = 0; i < matrix.length; i++) {
for (let j = 0; j < matrix[i].length; j++) {
console.log(`Element at [${i}][${j}]: ${matrix[i][j]}`);
}
}
Output
Element at [0][0]: 1
Element at [0][1]: 2
Element at [0][2]: 3
Element at [1][0]: 4
Element at [1][1]: 5
Element at [1][2]: 6
Element at [2][0]: 7
Element at [2][1]: 8
Element at [2][2]: 9
Performance Considerations with Nested Loops
- Time Complexity: The execution time increases with nested loops, often resulting in quadratic time complexity (O(n^2)) or worse.
- Optimization Tips:
- Minimize the depth of nesting wherever possible.
- Use efficient algorithms to reduce iterations, such as pre-computed results or avoiding redundant calculations.
- Be cautious when working with large datasets, as nested loops can quickly become computationally expensive.
Conclusion
Loops in JavaScript are essential tools for handling repetitive tasks efficiently. By using different types of loops in JavaScript, such as for, while, and for...of, developers can iterate through data structures, perform calculations, or manage dynamic operations. Mastering loops in JavaScript is crucial for writing optimized and readable code in real-world applications.
Dear learners, if you are still facing problems with your frontend design, we have come up with a new course, Frontend Foundations Certification Training. Don't be late and enroll now.
FAQs
- Use for when you know the number of iterations (e.g., iterating over an array with indexes).
- Use while when the number of iterations is uncertain and depends on a condition.
- Use do...while when the loop must run at least once.
- Use for...in for objects and for...of for iterable objects like arrays.
Take our Javascript skill challenge to evaluate yourself!
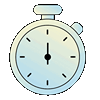
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.