11
JulMap in Javascript
JavaScript Map
The Map object is a strong and adaptable collection type that lets you store key-value pairs in JavaScript. In contrast to conventional objects, maps preserve the order in which their elements are inserted and can use any kind of data as a key. Because of this, Map is an adaptable tool for a wide range of programming situations where unique keys and ordered data storage are crucial.
In this JavaScript tutorial, We are going to explore more about the map in JavaScript including understanding JavaScript Map, examples of JavaScript map, and methods of JavaScript Map. To deepen your understanding and improve your skills, be sure to enroll in our Free Javascript Course for a thorough learning experience.
What is a JavaScript Map?
- In JavaScript, a Map is an integrated object that stores key-value pairs.
- The map is more flexible than objects since keys can be of any kind, unlike objects and functions, which can only have strings and symbols. Y
- You may also simply iterate over the elements in the order they were added because Map objects are iterable.
Examples of JavaScript Map
Here are a few examples to illustrate how Map works:
Example
// Creating a new Map
let map = new Map();
// Adding key-value pairs
map.set('name', 'Alice');
map.set('age', 25);
map.set(1, 'one');
// Accessing values
console.log(map.get('name')); // Alice
console.log(map.get(1)); // one
// Iterating over the map
for (let [key, value] of map) {
console.log(`${key} => ${value}`);
}
Output
Alice
one
name => Alice
age => 25
1 => one
JavaScript Map Methods
Map offers several ways for users to engage with its data:- set(key, value): Inserts a new element using the given value and key.
- get(key): Gives back the value connected to the given key.
- has(key): Determines if the map contains a key.
- delete(key): Eliminates the element linked to the given key.
- clear(): Clears the map of all elements. size: Gives the total number of items of the map.
Example of the map with the implementation of map methods
Example
map.set('city', 'New York');
console.log(map.has('city')); // true
map.delete('age');
console.log(map.size); // 3
map.clear();
console.log(map.size); // 0
Creating and Initializing a Map
Example
// Using the Map constructor
let map = new Map([
['name', 'Alice'],
['age', 25]
]);
// Adding elements
map.set('location', 'New York');
Advanced Operations with JavaScript Map
1. Cloning and Merging Maps
The spread operator and the new Map constructor can be used to duplicate a map. The set method can be used to merge maps.Example
let map1 = new Map([
['a', 1],
['b', 2]
]);
// Cloning map
let map2 = new Map(map1);
// Merging maps
let map3 = new Map([...map1, ...map2]);
2. Using Nested Maps
Map objects have the ability to hold additional maps, enabling intricate layered systems.Example
let nestedMap = new Map();
nestedMap.set('user', new Map([
['name', 'Alice'],
['age', 25]
]));
console.log(nestedMap.get('user').get('name')); // Alice
Performance-Related Issues
Take into account a map's performance attributes before using it:
- In general, insertion and lookup in a map operate more quickly than in an object.
- Maps are perfect for ordered data since they preserve insertion order.
Why and How to Use Maps in JavaScript?
- When you need keys of any kind, use Map instead of Unique Keys.
- When the sequence of the items is crucial, use the map.
- Iterability: To make iterating over key-value pairs simple, use maps.
- JavaScript Maps are an excellent tool for storing configuration parameters in cases when the keys are not strings.
- Caching Data: To store data for easy access, use a map.
- Graph Representation: Use maps to represent graphs with key-value pairs for nodes and edges.
Common Use Cases of JavaScript Map
1. Storing configuration setting
2. Caching Data
3. Graph Representation
Conclusion
FAQs
Take our Javascript skill challenge to evaluate yourself!
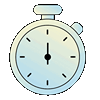
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.